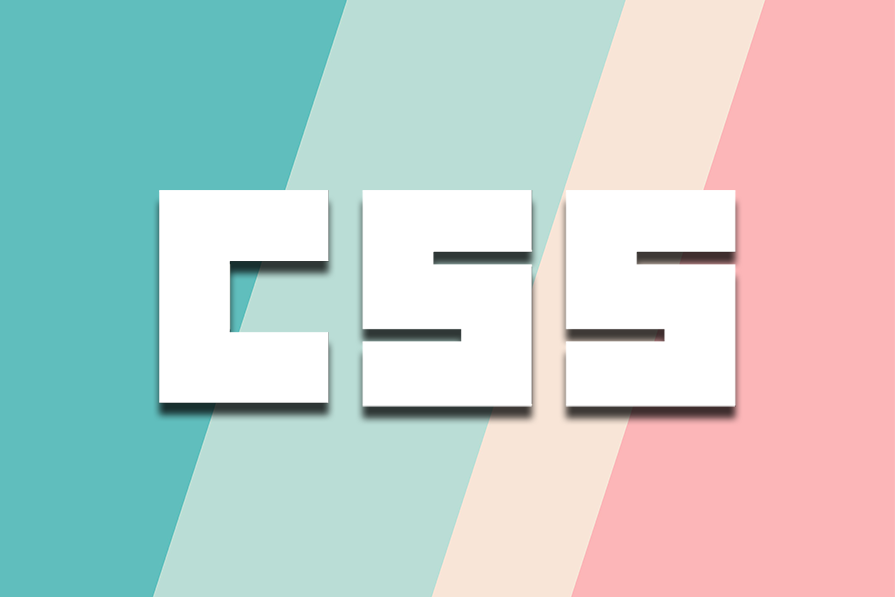
Project 3: Handling responsive design features
Project 3: Handling responsive design features 관련
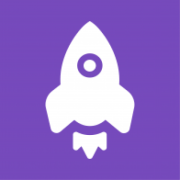

In our third project, we’ll build a responsive login form that loads some adjustment values from CSS variables. Like the media query feature dynamically switches standard CSS properties, it also switches custom properties, so we can assign different values for variables within different responsive breakpoints.
First, add the following content into a new HTML document:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<title>Responsive design with CSS variables</title>
</head>
<body>
<div class="form-box">
<input type="text" value="Username" />
<input type="password" value="Password" />
<button>Login</button>
</div>
</body>
</html>
Here we created a simple login form that consists of two input elements and a button. Add the following style tag into this HTML document to style it properly:
<style>
/* --- desktops and common --- */
:root {
--form-box-padding: 8px;
--form-box-flex-gap: 8px;
--form-input-font-size: 12px;
}
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
.form-box {
display: flex;
justify-content: flex-end;
gap: var(--form-box-flex-gap);
padding: var(--form-box-padding);
background-color: #333;
text-align: center;
}
.form-box input,
.form-box button {
font-size: var(--form-input-font-size);
padding: 8px;
margin-right: 4px;
}
.form-box input {
outline: none;
border: none;
}
.form-box button {
border: none;
background-color: #edae39;
}
</style>
The above CSS snippet styles the login form only for desktop devices, so it won’t adjust content responsively when you resize the browser, as shown in the following preview:
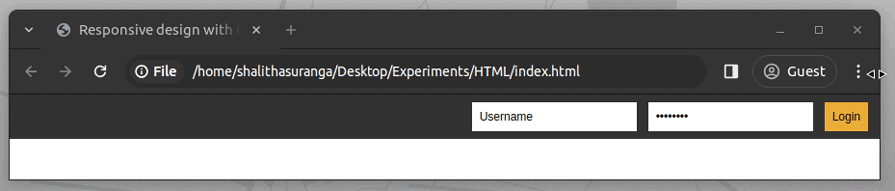
We can simply make this page responsive by writing some styling adjustments — i.e., changing flex-direction
— inside media query breakpoints. For padding
or font-size
-like values-based properties, we can use CSS variables instead of writing CSS properties repetitively to improve the readability and maintainability of CSS definitions.
Look at the previous CSS snippet: you will notice three CSS variables. Change those variables with media query blocks and complete the responsive screen handling code using the following code snippet:
/* --- tablets --- */
@media screen and (min-width: 601px) and (max-width: 900px) {
:root {
--form-box-padding: 20px 12px 20px 12px;
--form-box-flex-gap: 12px;
--form-input-font-size: 14px;
}
.form-box input,
.form-box button {
display: block;
width: 100%;
}
}
/* --- mobiles --- */
@media screen and (max-width: 600px) {
:root {
--form-box-padding: 24px;
--form-box-flex-gap: 12px;
--form-input-font-size: 20px;
}
.form-box {
flex-direction: column;
}
.form-box input,
.form-box button {
display: block;
}
}
The above code snippet adjusts the layout for mobile and tablet screens with some standard CSS properties and custom properties. For example, it uses a different flex-direction
mode, display
mode for several elements, and the following custom property values for mobile screens:
--form-box-padding: 24px;
--form-box-flex-gap: 12px;
--form-input-font-size: 20px;
Test this project by resizing the browser window:
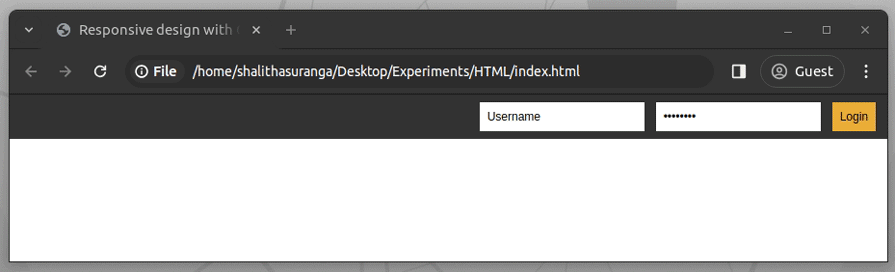
Try adjusting these CSS variables and creating new ones to improve this login screen further. You can use the same strategy to use CSS variables with container queries. Check the complete source code and see a live preview from this CodePen (shalithasuranga
).