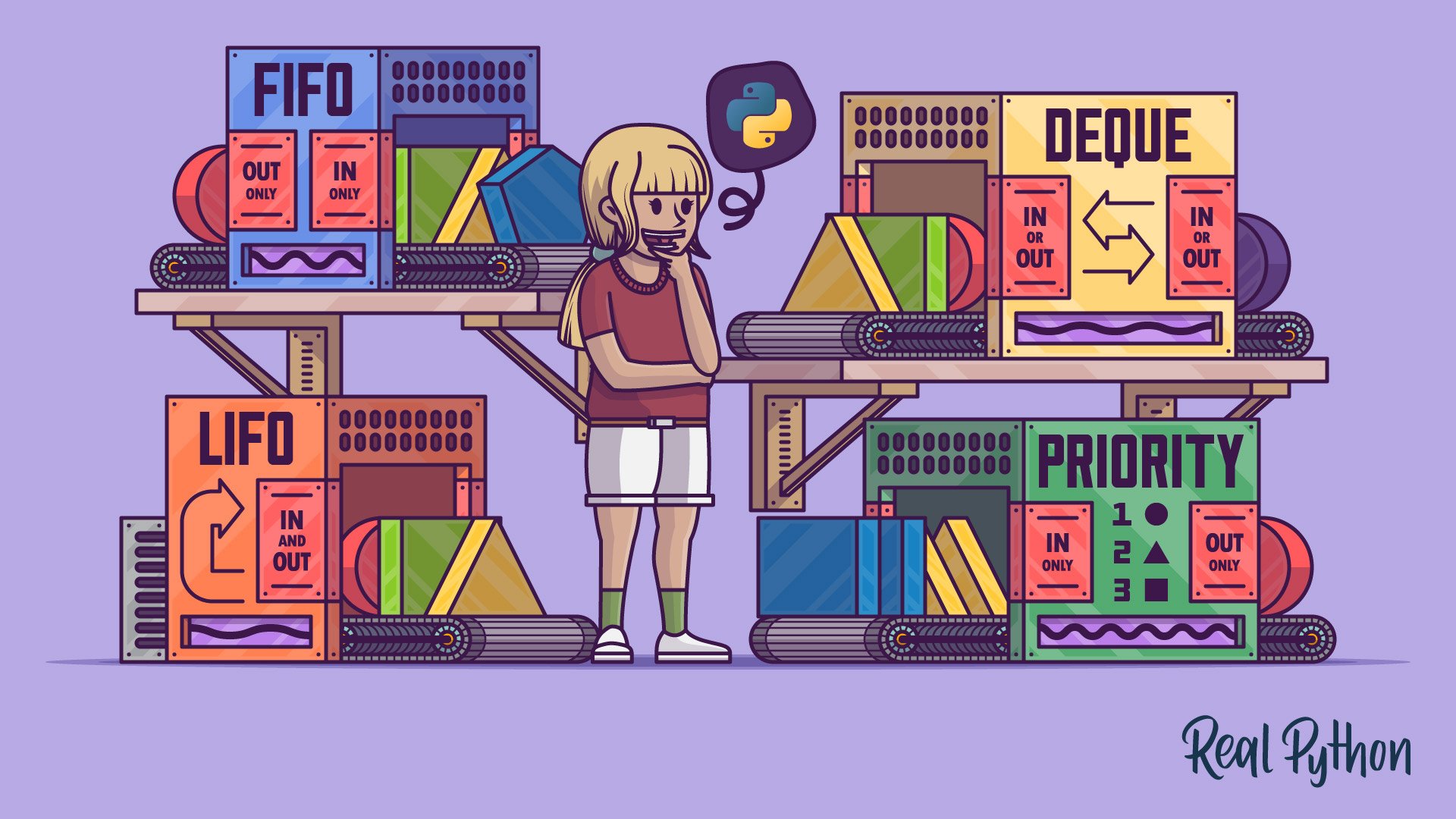
Python Stacks, Queues, and Priority Queues in Practice
Python Stacks, Queues, and Priority Queues in Practice 관련
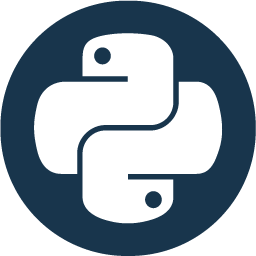
Queues are the backbone of numerous algorithms found in games, artificial intelligence, satellite navigation, and task scheduling. They’re among the top abstract data types that computer science students learn early in their education. At the same time, software engineers often leverage higher-level message queues to achieve better scalability of a microservice architecture. Plus, using queues in Python is simply fun!
Python provides a few built-in flavors of queues that you’ll see in action in this tutorial. You’re also going to get a quick primer on the theory of queues and their types. Finally, you’ll take a look at some external libraries for connecting to popular message brokers available on major cloud platform providers.
In this tutorial, you’ll learn how to
- Differentiate between various types of queues
- Implement the queue data type in Python
- Solve practical problems by applying the right queue
- Use Python’s thread-safe, asynchronous, and interprocess queues
- Integrate Python with distributed message queue brokers through libraries
To get the most out of this tutorial, you should be familiar with Python’s sequence types, such as lists and tuples, and the higher-level collections in the standard library.
You can download the complete source code for this tutorial with the associated sample data by clicking the link in the box below:
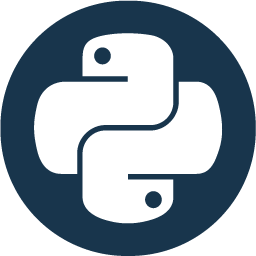
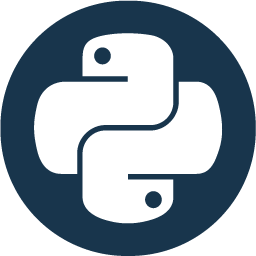
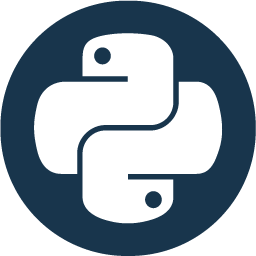
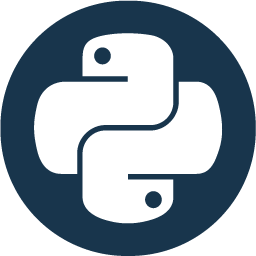
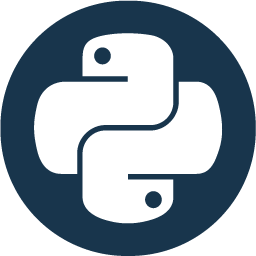
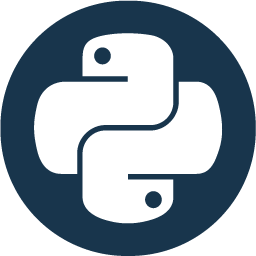
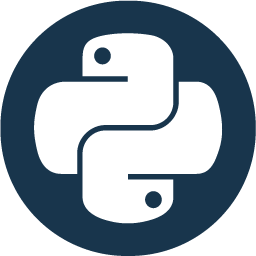
Conclusion
Now you have a solid understanding of the theory of queues in computer science and know their practical uses, ranging from finding the shortest path in a graph to synchronizing concurrent workers and decoupling distributed systems. You’re able to recognize problems that queues can elegantly solve.
You can implement FIFO, LIFO, and priority queues from scratch using different data structures in Python, understanding their trade-offs. At the same time, you know every queue built into the standard library, including thread-safe queues, asynchronous queues, and a queue for process-based parallelism. You’re also aware of the libraries allowing the integration of Python with popular message broker queues in the cloud.
In this tutorial, you learned how to
- Differentiate between various types of queues
- Implement the queue data type in Python
- Solve practical problems by applying the right queue
- Use Python’s thread-safe, asynchronous, and interprocess queues
- Integrate Python with distributed message queue brokers through libraries
Along the way, you’ve implemented breadth-first search (BFS), depth-first search (DFS), and Dijkstra’s shortest path algorithms. You’ve built a visual simulation of the multi-producer, multi-consumer problem, an asynchronous web crawler, and a parallel MD5 hash reversal program. To get the source code for these hands-on examples, follow the link below:
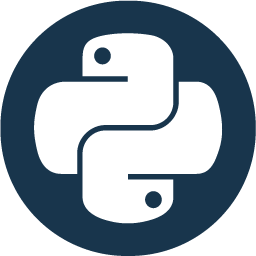