Rust to Assembly
About 2 min
Rust to Assembly ๊ด๋ จ
Understanding the Inner Workings of Rust
- Rust enum and match representation in assembly
- Assembly code generated when self is passed by value, reference or as a smart pointer
- Mapping Arrays, Tuples, Box and Option to assembly
- Map Rust vector iteration to assembly
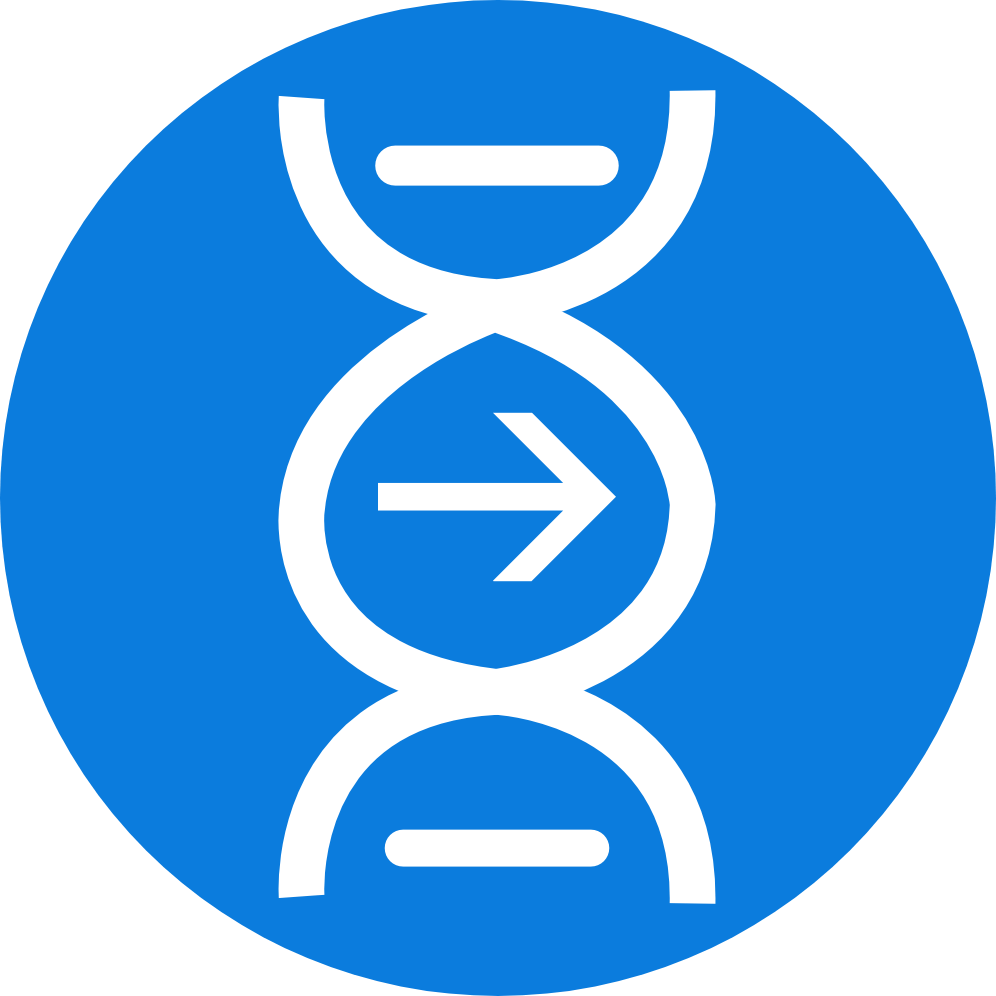
Mapping a bool vector to string slice vector
Understand the assembly code generated when mapping a Vec to a Vec<& 'static str> (static string slice vector). The allocations and de-allocations operations are also covered.
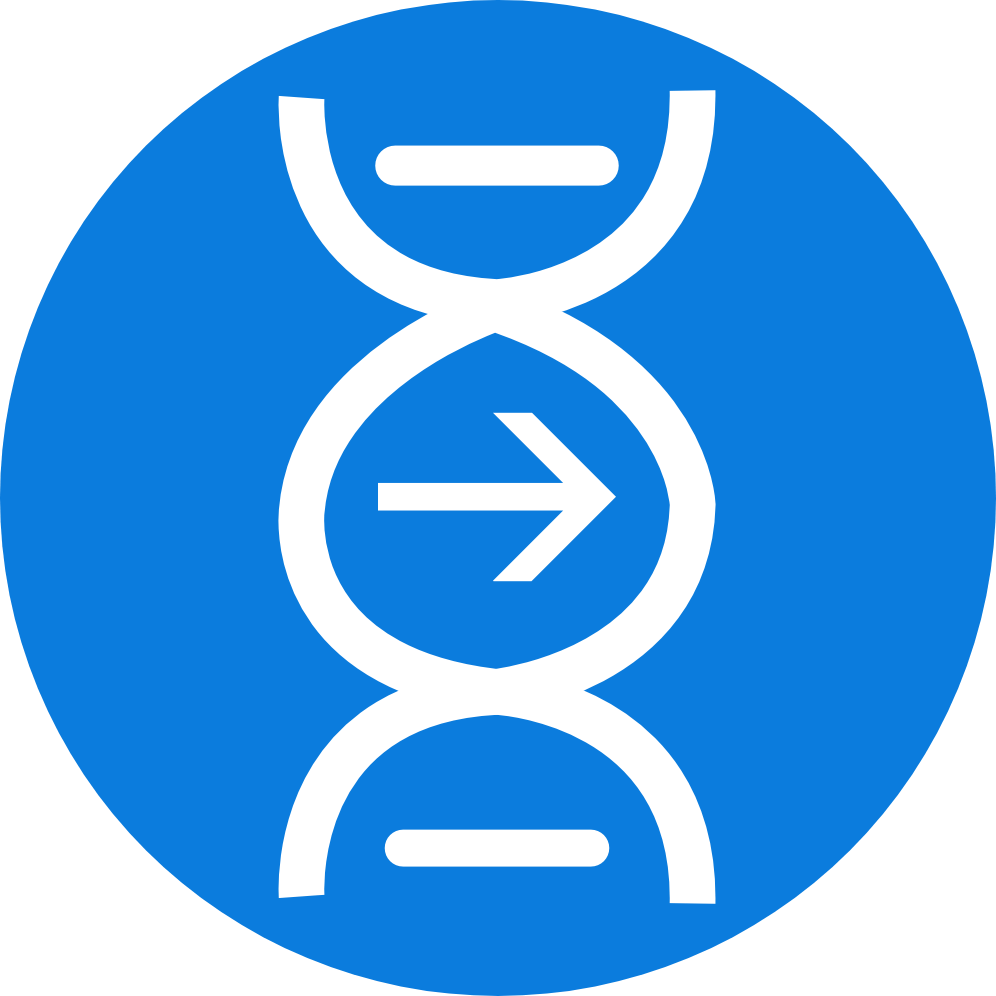
Mapping a bool vector to owned string vector
Understand the assembly code generated when mapping a Vec to a Vec (owned string). The allocations and de-allocations operations are also covered.
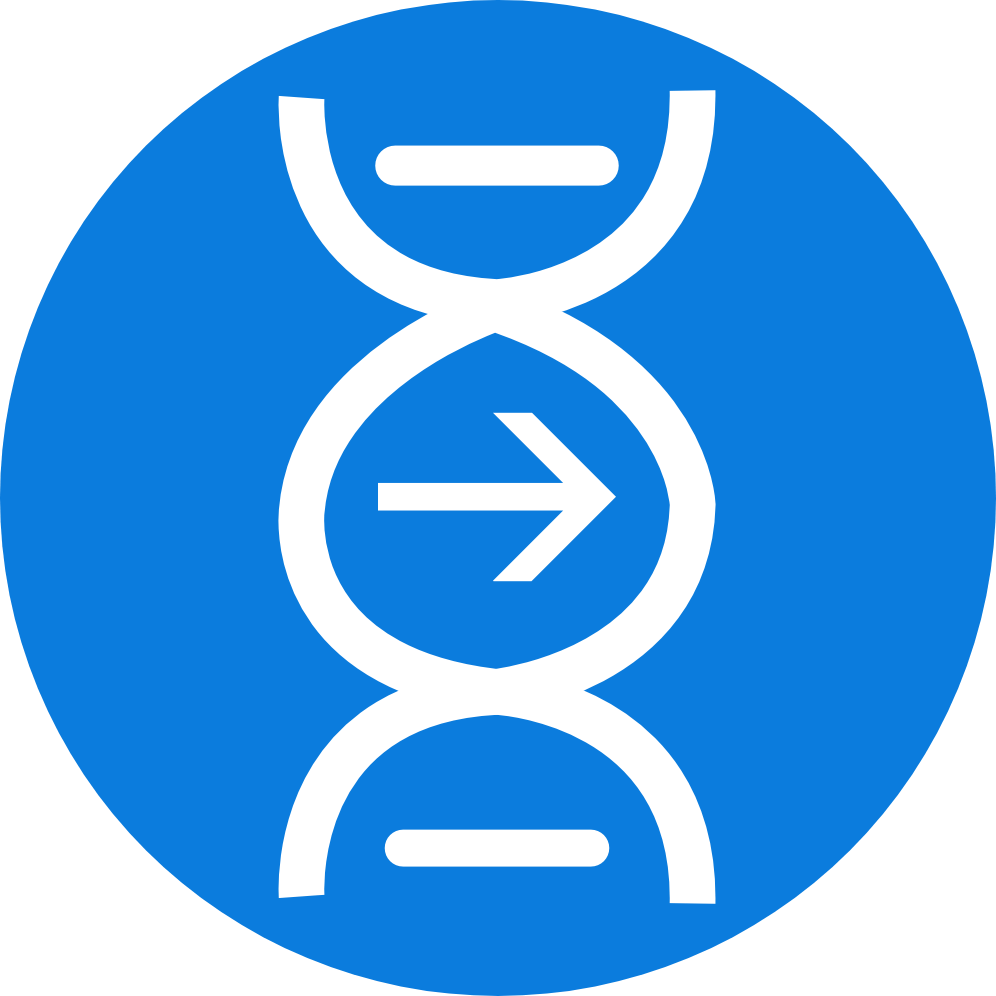
Compare static vs dynamic dispatch in Rust
Compare the assembly code generated for static vs dynamic dispatch for traits. Understand the performance implications of each approach.
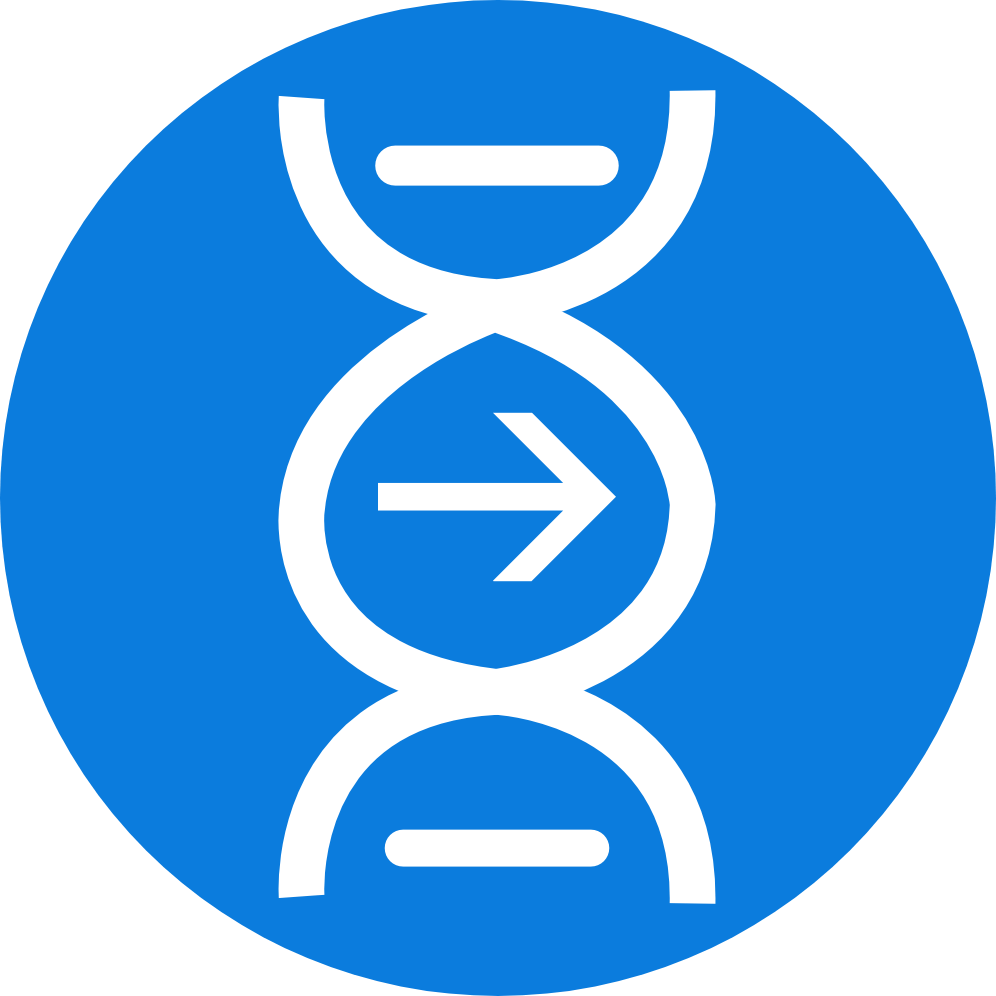
Understand tail call via vtable and freeing via a trait object
Learn how the compiler optimizes dynamically dispatched tail calls and how it frees memory when using trait objects.
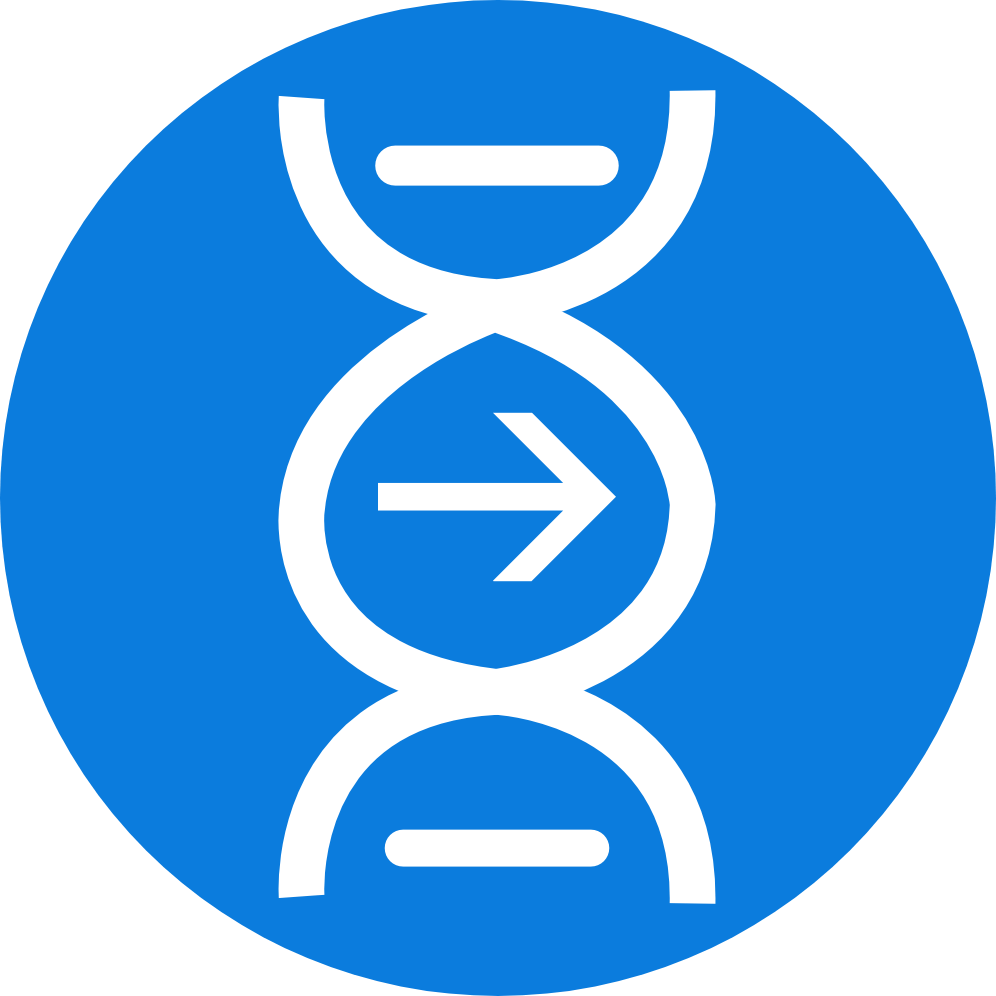
Rust Recursive Tree Traversal in Assembly
Learn how Rust compiles recursive tree traversal to assembly code and the optimization techniques used.
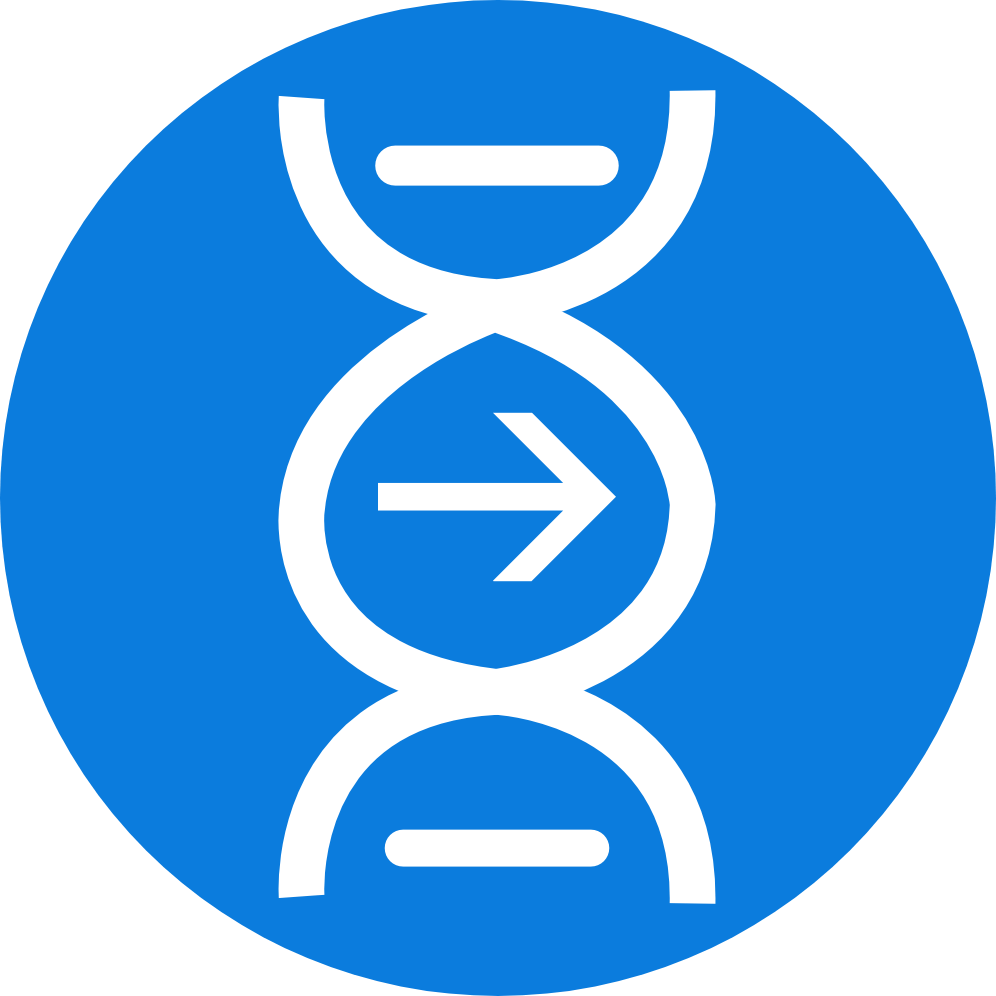
Rust Closures - impl Fn vs Box dyn Fn
Compare assembly code for Rust closures returned as impl Fn and Box
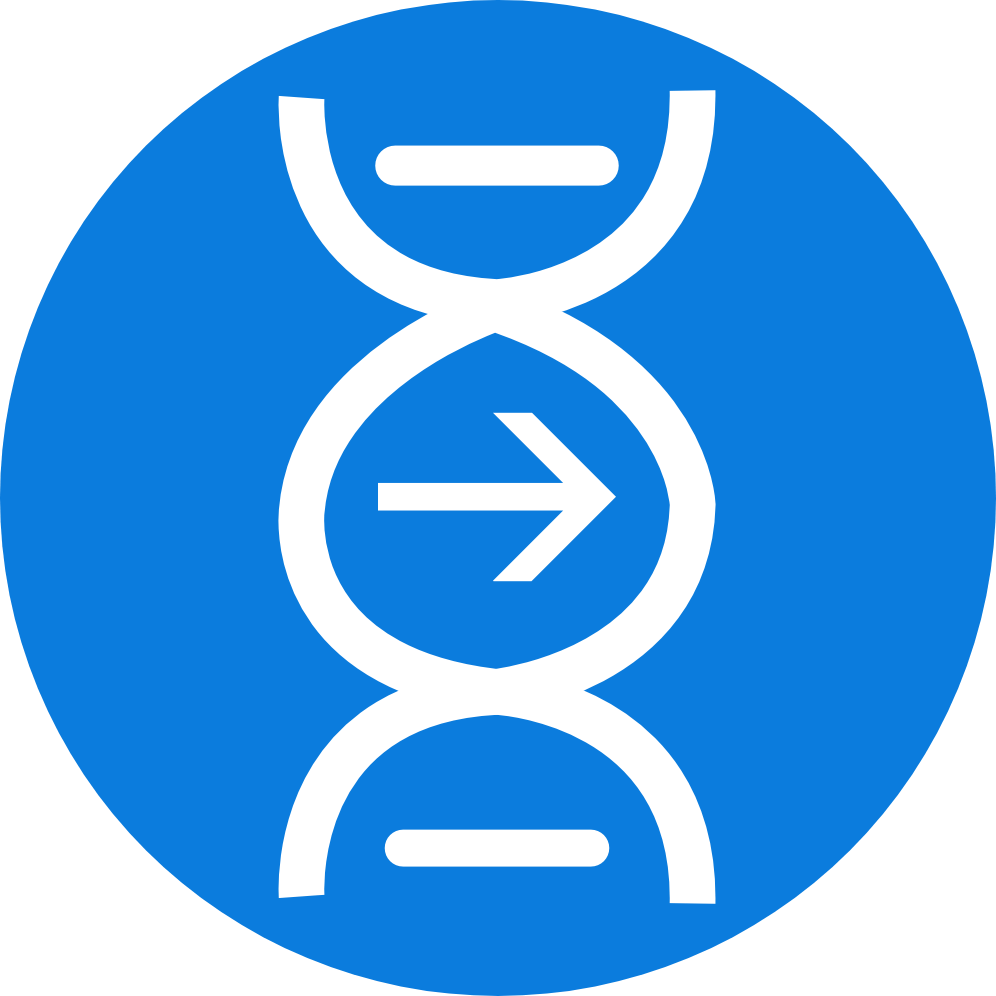
Rust async/await - State Machines and Assembly
Learn how Rust implements async functions using state machines and assembly code
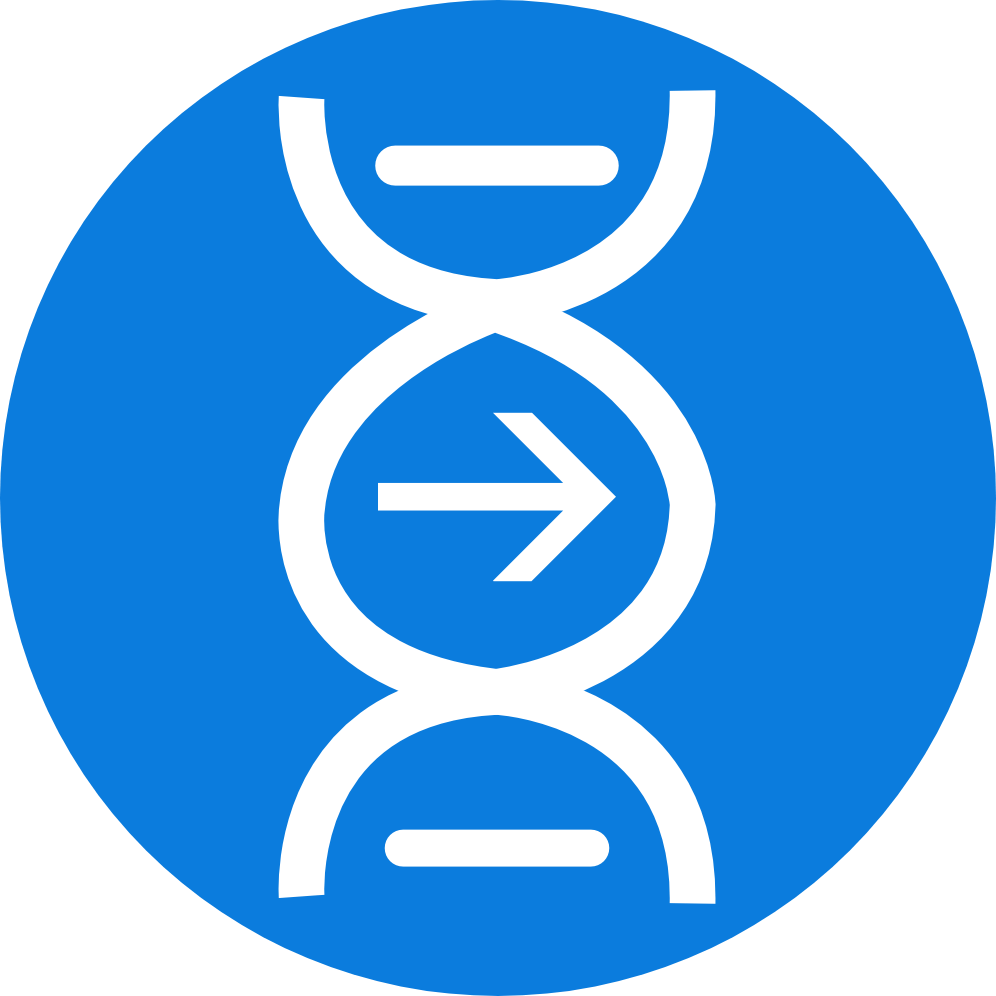
Rust async/await - Nested async function calls with loops
Desugaring and assembly code analysis for nested async function with a loop.
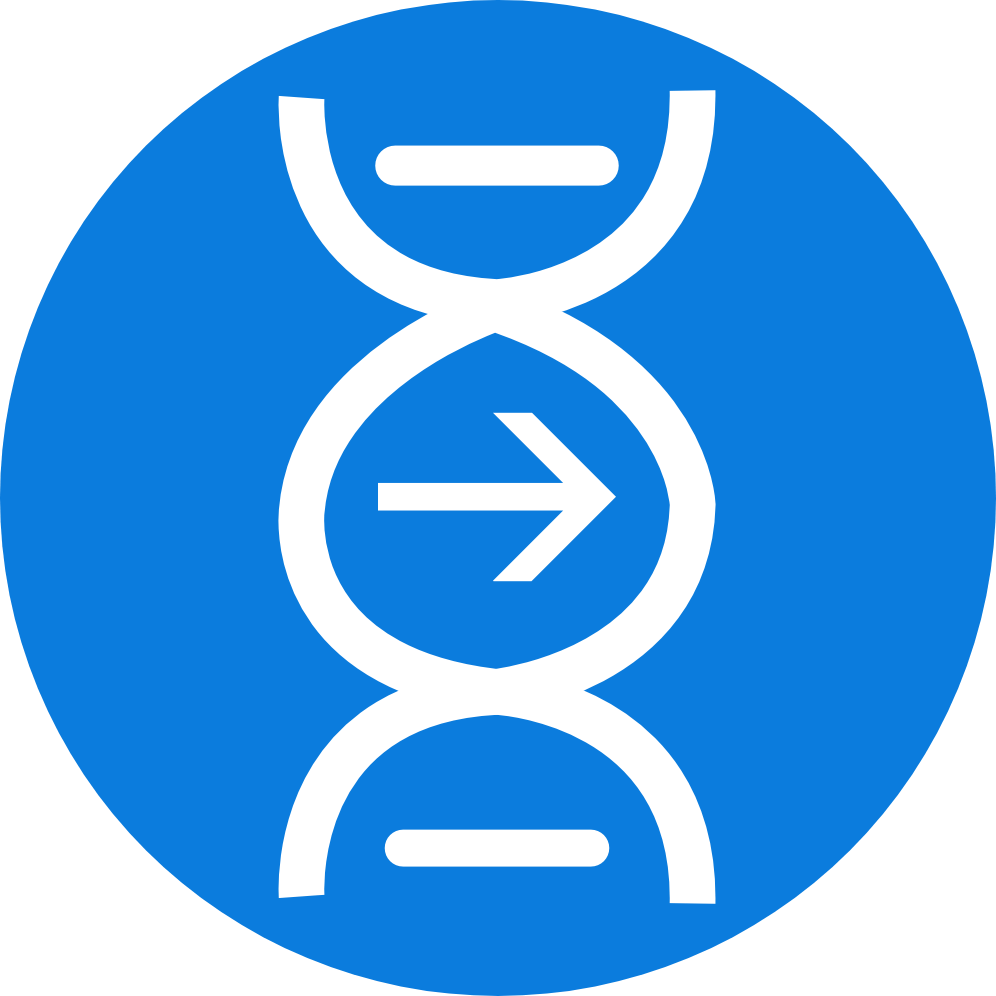
Rust async/await - Async executor
Learn how the async executor schedules async tasks in Rust. Understand the code of a simple async executor.