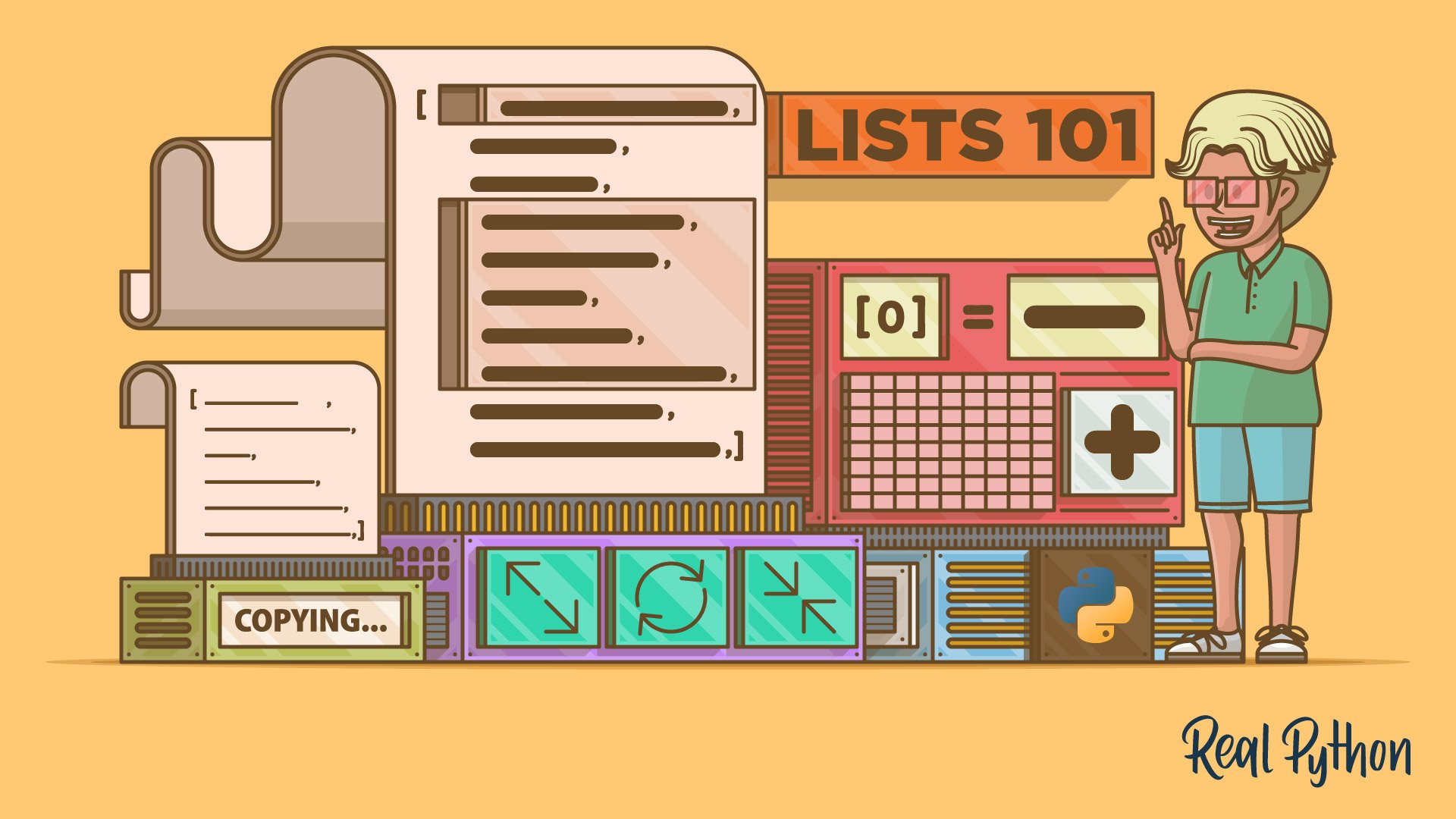
Common Gotchas of Python Lists
Common Gotchas of Python Lists 관련
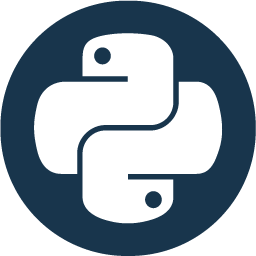
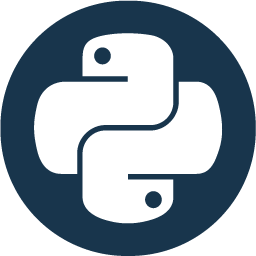
If you’re new to Python and are starting with lists, then you’ll want to be on the lookout for a few gotchas that can cause subtle issues in your code. Up to this point, you’ve learned what you need in order to understand most of these gotchas, so here’s a summary of the most common ones:
- Confusing aliases of a list with copies: This can cause issues because changes to one alias affect others. Take a look at the Aliases of a List section for practical examples of this issue.
- Forgetting that most
list
methods mutate the list in place and returnNone
rather than a new list: This commonly leads to issues when you assign the return value of a list method to a variable, thinking that you have a new list, but you really getNone
. Check out the Reversing and Sorting Lists section for practical examples of this gotcha. - Confusing
.append()
with.extend()
: This can cause issues because.append()
adds a single item to the end of the list, while the.extend()
method unpacks and adds multiple items. Have a look at the Growing and Shrinking Lists Dynamically section for details on how these methods work. - Using an empty list as a default argument value in function definitions: This can lead to unexpected behaviors because default argument values get defined when Python first parses the function.
You already know the explanation of the first three bullet points in this list. So, you only have to dive deeper into the last point. Why should you avoid using an empty list—or a list in general—as a default argument value? To answer this question, consider the following toy function:
def append_to(item, target=[]):
target.append(item)
return target
This function appends item
to the end of target
, which defaults to an empty list. At first glance, it may seem that consecutive calls to append_to()
will return single-item lists like in the following hypothetical example:
append_to(1)
#
# [1]
append_to(2)
#
# [2]
append_to(3)
#
# [3]
But because Python defines the default argument value when it first parses the function and doesn’t overwrite it in every call, you’ll be working with the same list object in every call. Therefore, you don’t get the above behavior. Instead, you get the following:
append_to(1)
#
# [1]
append_to(2)
#
# [1, 2]
append_to(3)
#
# [1, 2, 3]
The target
list remembers the data between calls. This happens because you’re using the same list object that appears as the default value in the function’s definition.
To prevent this issue, you can use None
as the default value:
def append_to(item, target=None):
if target is None:
target = []
target.append(item)
return target
append_to(1)
#
# [1]
append_to(2)
#
# [2]
append_to(3)
#
# [3]
Great! You’ve solved the issue. Now your function returns single-item lists as expected. That’s because the function doesn’t retain the state between calls.