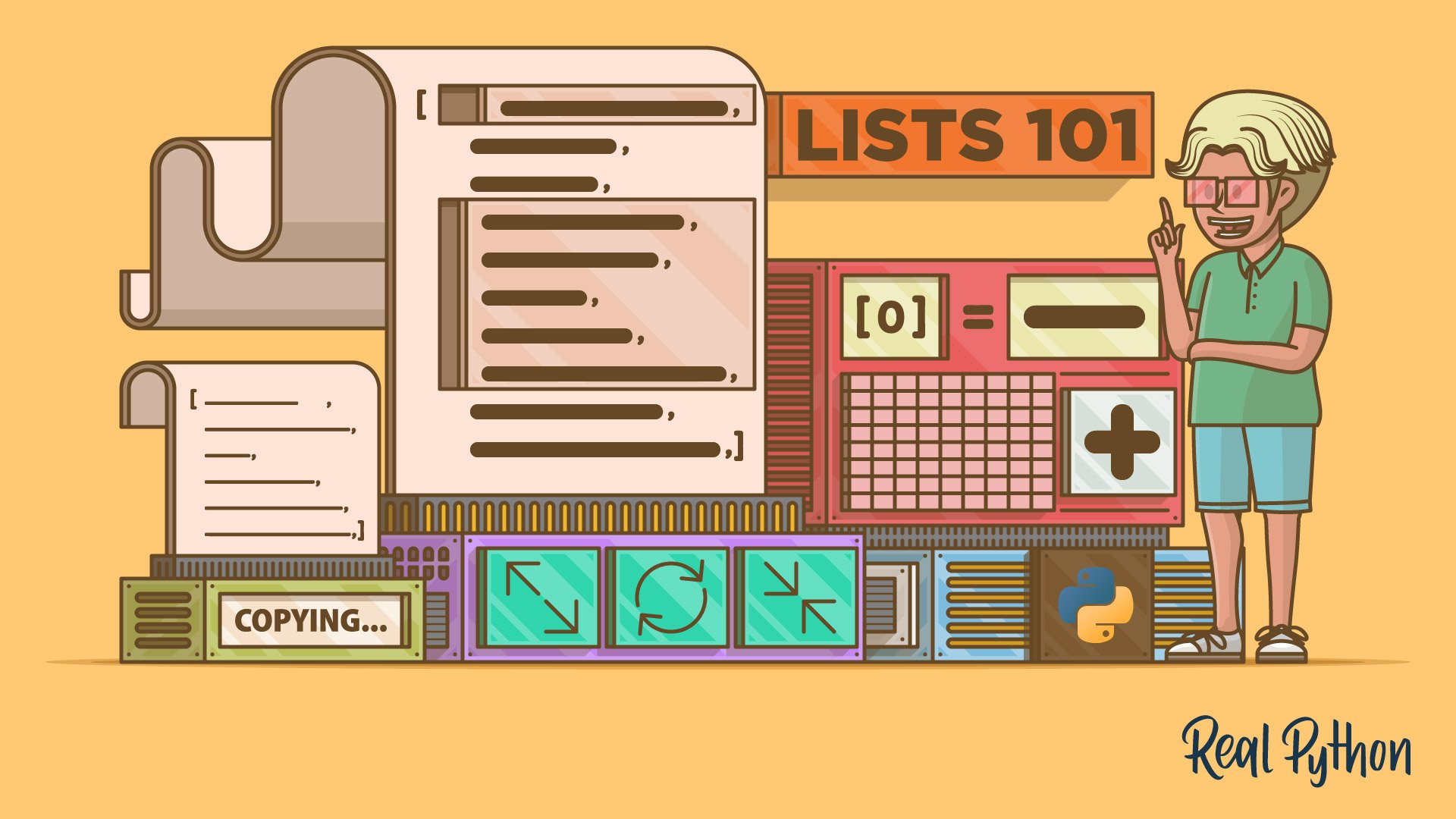
Deciding Whether to Use Lists
July 19, 2023About 2 min
Deciding Whether to Use Lists 관련
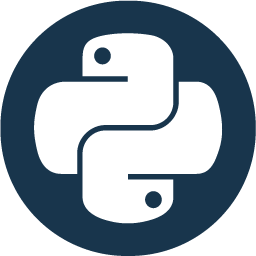
Python's list Data Type: A Deep Dive With Examples
In this tutorial, you'll dive deep into Python's lists. You'll learn how to create them, update their content, populate and grow them, and more. Along the way, you'll code practical examples that will help you strengthen your skills with this fundamental data type in Python.
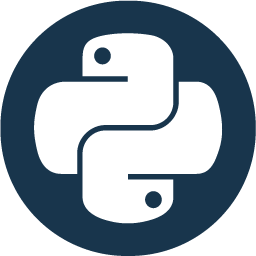
Python's list Data Type: A Deep Dive With Examples
In this tutorial, you'll dive deep into Python's lists. You'll learn how to create them, update their content, populate and grow them, and more. Along the way, you'll code practical examples that will help you strengthen your skills with this fundamental data type in Python.
As you’ve learned throughout this tutorial, lists are powerful, flexible, versatile, and full-featured data structures. Because of their characteristics, people tend to use and abuse them. Yes, they’re suitable for many use cases, but sometimes they aren’t the best available option.
In general, you should use lists when you need to:
- Keep your data ordered: Lists maintain the order of insertion of their items.
- Store a sequence of values: Lists are a great choice when you need to store a sequence of related values.
- Mutate your data: Lists are mutable data types that support multiple mutations.
- Access random values by index: Lists allow quick and easy access to elements based on their index.
In contrast, avoid using lists when you need to:
- Store immutable data: In this case, you should use a tuple. They’re immutable and more memory efficient.
- Represent database records: In this case, consider using a tuple or a data class.
- Store unique and unordered values: In this scenario, consider using a set or dictionary. Sets don’t allow duplicated values, and dictionaries can’t hold duplicated keys.
- Run many membership tests where item doesn’t matter: In this case, consider using a
set
. Sets are optimized for this type of operation. - Run advanced array and matrix operations: In these situations, consider using NumPy’s specialized data structures.
- Manipulate your data as a stack or queue: In those cases, consider using
deque
from thecollections
module orQueue
,LifoQueue
, orPriorityQueue
. These data types are thread-safe and optimized for fast inserting and removing on both ends.
Depending on your specific scenario, lists may or may not be the right tool for the job. Therefore, you must carefully evaluate your needs and consider advanced data structures like the ones listed above.