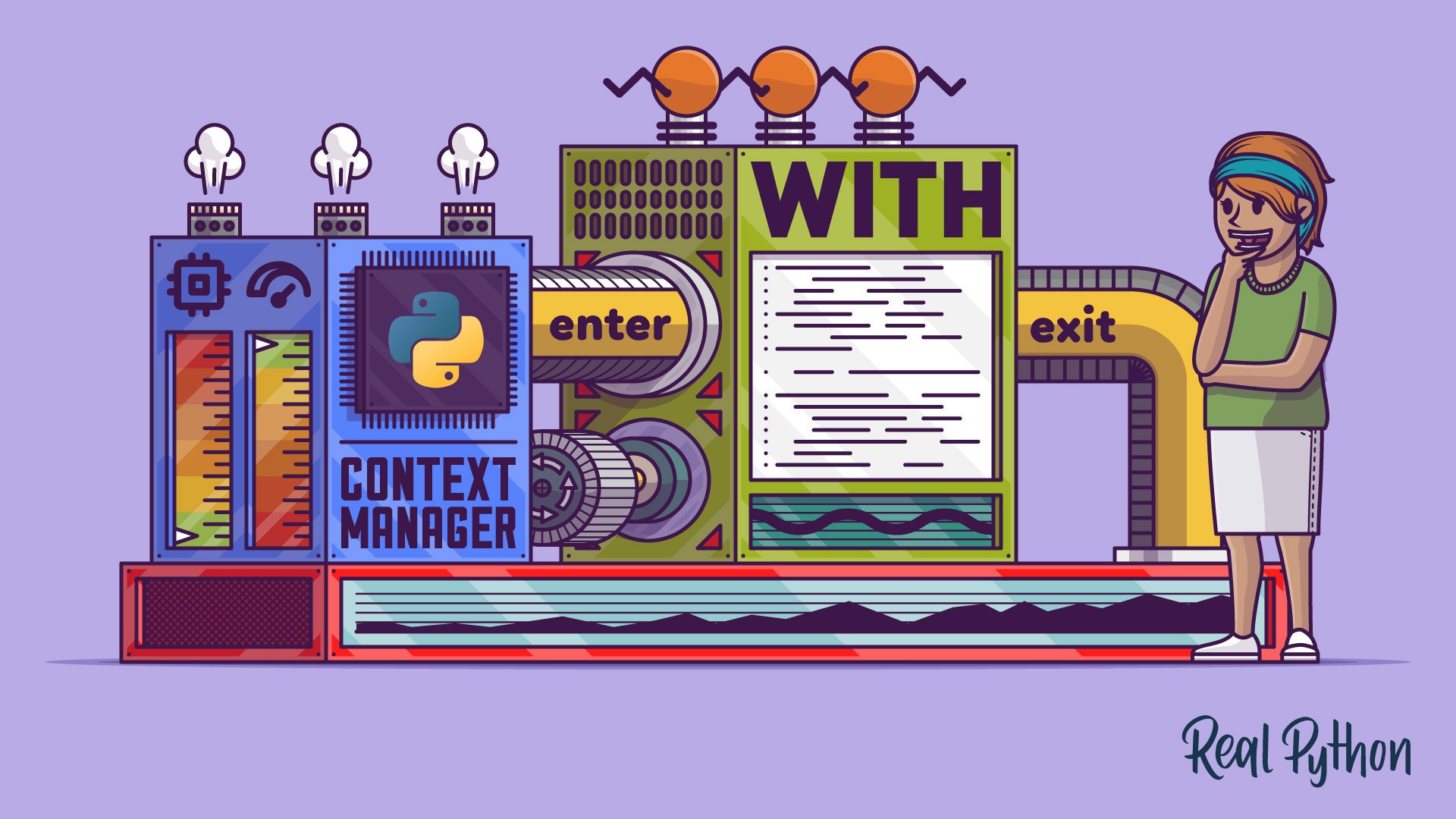
Creating Custom Context Managers
Creating Custom Context Managers 관련
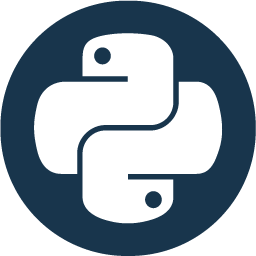
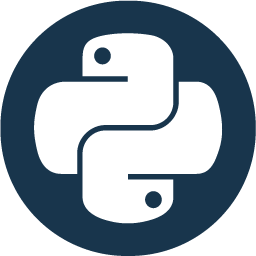
You’ve already worked with context managers from the standard library and third-party libraries. There’s nothing special or magical about open()
, threading.Lock
, decimal.localcontext()
, or the others. They just return objects that implement the context management protocol.
You can provide the same functionality by implementing both the .__enter__()
and the .__exit__()
special methods in your class-based context managers. You can also create custom function-based context managers using the contextlib.contextmanager
decorator from the standard library and an appropriately coded generator function.
In general, context managers and the with
statement aren’t limited to resource management. They allow you to provide and reuse common setup and teardown code. In other words, with context managers, you can perform any pair of operations that needs to be done before and after another operation or procedure, such as:
- Open and close
- Lock and release
- Change and reset
- Create and delete
- Enter and exit
- Start and stop
- Setup and teardown
You can provide code to safely manage any of these pairs of operations in a context manager. Then you can reuse that context manager in with
statements throughout your code. This prevents errors and reduces repetitive boilerplate code. It also makes your APIs safer, cleaner, and more user-friendly.
In the next two sections, you’ll learn the basics of creating class-based and function-based context managers.