Article(s)
About 12 min
Article(s) ๊ด๋ จ
freeCodeCamp Programming Tutorials: Python, JavaScript, Git & More
Browse thousands of programming tutorials written by experts. Learn Web Development, Data Science, DevOps, Security, and get developer career advice.
Frontend Masters Boost โ Helping Your Journey to Senior Developer
Helping Your Journey to Senior Developer
NHN Cloud Meetup - NHN ๊ธฐ์ ๋ธ๋ก๊ทธ
๊ธฐ์ ์ ๊ณต์ ํ๊ณ ํจ๊ป ์ฑ์ฅํด๊ฐ๋ ๊ฐ๋ฐ ๋ฌธํ, NHN์ด ์ถ๊ตฌํ๋ ๊ฐ์น์
๋๋ค.
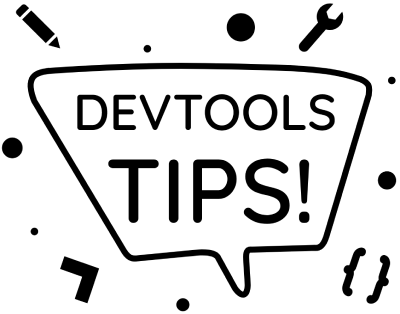
DevTools Tips
The collection of tips useful for web development.
freecodeCamp
freecodecamp.org
How to Loop Through Arrays in JavaScript
Looping through arrays in JavaScript is a fundamental concept that every JavaScript developer should understand. Whether you're a beginner or an experienced developer, understanding how to loop through an array is crucial for many programming tasks. In this article, we'll explore the different ways to loop through an array
How to Create an Interactive Terminal-Based Portfolio Website
In this article, you will learn how to create an interactive terminal-based portfolio and rรฉsumรฉ in JavaScript. We'll use the jQuery Terminal library (and a few other tools) to create a website that looks like a real terminal. This article will show more advanced usage of the jQuery Terminal
What are Higher Order Functions in JavaScript? Explained With Examples
JavaScript offers a powerful feature known as higher order functions (HOFs). These functions elevate your code by treating other functions as citizens of the language itself. In simpler terms, HOFs can accept functions as arguments and even return functions as results. This ability allows developers to write clean, reusable, and
How Do Closures Work in JavaScript? Explained with Code Examples
Sally and Joe are two love birds. They shared everything with each other and soon enough it was almost impossible to think that anything could come between them. One day, they had a quarrel which built up to a break up. It was hard for Joe and he wanted...
JavaScript Concatenate Strings โ How JS String Concatenation Works
When coding in JavaScript, you may need to combine multiple strings to create a new, longer string. This operation is known as concatenation. In this article, you will learn five ways to concatenate strings in JavaScript. How to Concatenate Strings i...
JS Remove Char from String โ How to Trim a Character from a String in JavaScript
Manipulating strings is a fundamental skill in programming. A common task you might encounter when coding in JavaScript is trimming characters from a string. Trimming involves removing specific characters from the beginning and/or end of a string. Th...
How to Create Objects in JavaScript
In programming, objects are fundamental building blocks used to represent real-world entities or concepts. JavaScript, a versatile and popular language, offers various ways to create these objects. This article dives deep into these methods, equipping you with the knowledge to craft objects tailored to your programming needs. We'll begin...
The JavaScript Class Handbook โ Complete Guide to Class Fields and the Super Keyword
Classes let you privatize your data while providing users indirect access to it. It is an excellent way to prevent direct access to your constructorโs data. This handbook aims to show you exactly how classes work in JavaScript. We will also discuss class fields and the super keyword. Table of...
The JavaScript Interview Prep Handbook โ Essential Topics to Know + Code Examples
JavaScript is a widely used language in web development and powers interactive features of virtually every website out there. JavaScript makes it possible to create dynamic web pages and is very versatile. JavaScript remains one of the most in-demand programming languages in 2024. Many companies are looking for proficiency in...
How to Manipulate Strings in JavaScript โ With Code Examples
String manipulation is a common task for programmers, whether it is extracting information from the string, converting letter cases, joining strings, or trimming extra white spaces. This tutorial covers various methods and techniques for manipulating strings using JavaScript, offering you a comprehensive guide on how to work with strings in...
What is Prototypal Inheritance in JavaScript? Explained with Code Examples
Prototypal inheritance can feel like a complex concept shrouded in technical jargon. But fear not! This guide will break it down using clear, relatable examples that go beyond the typical textbook explanations. We'll ditch the confusing terms and focus on real-world scenarios that you can easily understand. By the...
The JavaScript this Keyword Explained with Examples
All leading web browsers support JavaScript, a popular and versatile programming language. The this keyword is a very important concept to know in JavaScript. The this keyword is a reference to an object, but the object varies based on where and how it is called. In this article, you'll learn...
How to Use WeakMap and WeakSet in JavaScript
JavaScript offers a number of tools for organizing and managing data. And while developers often use widely recognized tools like Maps and Sets, they may often overlook certain other valuable resources. For example, are you familiar with WeakMap and WeakSet? They're special tools in JavaScript that help store and...
Scope, Closures, and Hoisting in JavaScript โ Explained with Code Examples
In the dynamic world of JavaScript, understanding the intricacies of scope, closures, and hoisting is fundamental for mastering the language and building robust applications. These concepts, though often misunderstood, play a crucial role in determining how variables and functions behave within the code. Scope dictates the accessibility of variables, closures...
How to Change Background Color with JavaScript โ BG Color in JS and HTML
You can style elements with JavaScript using the element's style property. In this article, you'll learn how to change background color using JavaScript. Here's what the mini project you'll build looks like: In the image above, each button changes t...
JavaScript GameDev with Kaboom.js
Creating a game can be one of the most rewarding experiences in programming, and building a Metroidvania-style game takes that excitement to another level. These games are known for their intricate maps, challenging enemies, and complex mechanics tha...
How to Use Callback Functions in JavaScript
When you're building dynamic applications with JavaScript that display real-time data โ like a weather app or live sports dashboard โ you'll need a way to automatically fetch new data from an external source without disrupting the user experience. You can do this using JavaScript's callback functions, which showcase...
How to Use Callback Functions in JavaScript
Nowadays, the interaction between web applications relies on HTTP. For instance, let's say you have an online shop application and you want to create a new product. You have to fill in all the necessary information and probably click on a button that says 'Create'. This action will send...
Learn Asynchronous JavaScript
Asynchronous programming is a critical skill in modern JavaScript development, enabling developers to create more efficient and responsive applications. Whether you're a seasoned developer looking to brush up on your skills or a beginner eager to lea...
How to Build a Simple Bitcoin-to-USD Calculator
Welcome to this fun and hands-on project where we'll build a calculator that converts Bitcoin to USD. You'll learn about API fetching, DOM manipulation, and localStorage, and you'll use some basic math along the way. By the end of this tutorial, you'll have a functioning Bitcoin price calculator that...
How to Match Parentheses in JavaScript without Using Regex
While writing my Lisp interpreter (for the Scheme dialect, to be precise), I decided to include support for square brackets. I did this because some of the Scheme books use them interchangeably with parentheses. But I didn't want to make the parser t...
What is a Component Library? When to Build Your Own and When to Use Someone Else's
If you've built a frontend project in the last five years, you will have likely written some components, and maybe even used a component library. Components and libraries have been an important part of the web development landscape for multiple decad...
How to Create A Color Picker Tool Using HTML, CSS, and JavaScript
Have you ever wanted to create your own interactive tools using just HTML, CSS, and JavaScript? In this article, we'll create a fun and straightforward project: a color picker tool. This handy little tool will let users select any color they like and...
How to Use Variables and Data Types in JavaScript โ Explained With Code Examples
A variable is like a box where you can store data or a reference to data. In this article, you will learn how to create and use variables. You'll also learn about the different data types in JavaScript and how to use them. Let's get started! Table of...
How to Create Interactive HTML Prototypes โ How Far Can You Go Without JavaScript?
Interactivity is what makes a website come alive. Whether it's a button that reveals more content or a form that responds to your input, these little touches keep users engaged. Traditionally, we've relied heavily on JavaScript to make websites inter...
How to Build an Expense Tracker with HTML, CSS, and JavaScript
Building projects is a great way to practice and improve your web development skills. And that's what we'll do in this in-depth tutorial: build a practical project using HTML, CSS, and JavaScript. If you often find yourself wondering where all your m...
How to Use Chart js for Interactive Data Visualization
Data surrounds us, but its raw form can be overwhelming and difficult to interpret. That's where data visualization comes in. It can help you take your data and turn it into charts and graphs that make sense at a glance. Among the many data visualiza...
How to Use the JavaScript Selection API: Build a Rich Text Editor and Real-Time Element Detection
When you interact with web pages, a common task youโll perform often is selecting text. Whether it's highlighting a section of a paragraph to copy, marking important parts of a document, or working with interactive features like note-taking or text e...
JavaScript Timer โ How to Set a Timer Function in JS
In Javascript, the timer function prevents your code from running everything at once when an event triggers or the page loads. This gives you more control over the timing of your program's actions and can enhance the user experience by creating smoot...
New JavaScript Array Methods to Help You Write Better, Cleaner Code
JavaScript is always improving, and every year, new features are added to make coding easier and more efficient. These updates help developers write cleaner code and work faster. If you want to stay ahead as a developer, it's important to learn about...
Learn HTTP Methods like GET, POST, and DELETE โ a Handbook with Code Examples
When you interact with websites or apps, a lot happens behind the scenes. A key part of this process is how your browser or app talks to a server. HTTPS methods define what action needs to happen โ it could be fetching data, sending information, or m...
Frontend Masters Boost โ Helping Your Journey to Senior Developer
frontendmasters.com
Five Basic Things About JavaScript That Will Help Non JavaScript-Focused Web Designers
Let's say you don't know JavaScript. You're a web designer and you're focused largely on UI and UX. Let's look at some things you could learn in a day that will give you that bang for the buck.
Control JavaScript Promises from Anywhere Using Promise.withResolvers()
This method enhances flexibility by allowing promises to be resolved or rejected remotely, simplifying and streamlining asynchronous code.
Playing with the Speculation Rules API in the Console
This new API enables client-side prerendering, improving performance for users who are likely to visit a new page.
Popovers Work Pretty Nicely as Slide-Out Drawers
Especially on mobile, the slide-out drawer UI/UX seems like a perfect fit for a popover, and works fine on desktop too.
Browser Support Tests in JavaScript for Modern Web Features
Sometimes it's good to know when a browser feature is supported or not so you can do something. Perhaps load a polyfill or just choose a slightly different approach. This post looks at newish features in browsers and shows the test
Script Integrity
Polyfill.io recently served malicious code, redirecting users to inappropriate sites. Subresource Integrity (SRI) can help prevent such issues by verifying script integrity.
Clip Pathing Color Changes
Let's look at a cool animated nav effect (from a recent post by Emil Kowalski) that uses CSS `clip-path` to move the highlighted nav item around. It's an interesting look at this CSS feature and adds a lot of polish to a simple idea.
Patterns for Memory Efficient DOM Manipulation with Modern Vanilla JavaScript
JavaScript Frameworks generally do a lot of DOM handling for you, but doing it yourself can be the most performant option, and there are quite a few best practices.
Reading from the Clipboard in JavaScript
While it's a bit more common to *write* to the clipboard, JavaScript can also read from it. Plain text is pretty simple, while multimedia content is a bit more complex.
์์ฆIT
yozm.wishket.com
ํ๋ก๊ทธ๋๋ฐ ์ฉ์ด โ๋ฉฑ๋ฑ์ฑ(Idempotent)โ ์์๋ณด๊ธฐ | ์์ฆIT
ํ๋ก๊ทธ๋๋ฐ ์ฉ์ด โ๋ฉฑ๋ฑ์ฑ(Idempotent)โ ์์๋ณด๊ธฐ
์์ํ๋ ๊ฐ๋ฐ์๋ฅผ ์ํ JS ๋งค๊ฐ ๋ณ์ ํ์ฉ๋ฒ 2๊ฐ์ง | ์์ฆIT
์์ํ๋ ๊ฐ๋ฐ์๋ฅผ ์ํ JS ๋งค๊ฐ ๋ณ์ ํ์ฉ๋ฒ 2๊ฐ์ง
์์ํ๋ ๊ฐ๋ฐ์๋ฅผ ์ํ JS ์ ๊ฐ ๊ตฌ๋ฌธ ํ์ฉ๋ฒ 3๊ฐ์ง | ์์ฆIT
์์ํ๋ ๊ฐ๋ฐ์๋ฅผ ์ํ JS ์ ๊ฐ ๊ตฌ๋ฌธ ํ์ฉ๋ฒ 3๊ฐ์ง
์์ํ๋ ๊ฐ๋ฐ์๋ฅผ ์ํ JS ๊ตฌ์กฐ ๋ถํด ํ์ฉ๋ฒ 3๊ฐ์ง | ์์ฆIT
์์ํ๋ ๊ฐ๋ฐ์๋ฅผ ์ํ JS ๊ตฌ์กฐ ๋ถํด ํ์ฉ๋ฒ 3๊ฐ์ง
NHN Cloud Meetup - NHN ๊ธฐ์ ๋ธ๋ก๊ทธ
meetup.nhncloud.com
CodeSnippet๊ณผ ํจ๊ปํ๋ JavaScript ํ๋ก๊ทธ๋๋ฐ | NHN Cloud Meetup
CodeSnippet๊ณผ ํจ๊ปํ๋ JavaScript ํ๋ก๊ทธ๋๋ฐ
์บ์ ์ฑ๋ฅ ํฅ์๊ธฐ (Improving Cache Speed at Scale) | NHN Cloud Meetup
์บ์ ์ฑ๋ฅ ํฅ์๊ธฐ (Improving Cache Speed at Scale)
PLT Hook ์ฒดํฌ๋ฅผ ์ํ Android so ํ์ผ ํ์ฑ | NHN Cloud Meetup
PLT Hook ์ฒดํฌ๋ฅผ ์ํ Android so ํ์ผ ํ์ฑ
NAVER D2
d2.naver.com
RTCS ์ค์๊ฐ ์น ์๋น์ค๋ฅผ ์ํ ๋์ | NAVER D2
RTCS ์ค์๊ฐ ์น ์๋น์ค๋ฅผ ์ํ ๋์
Devtool Tips
devtoolstips.org
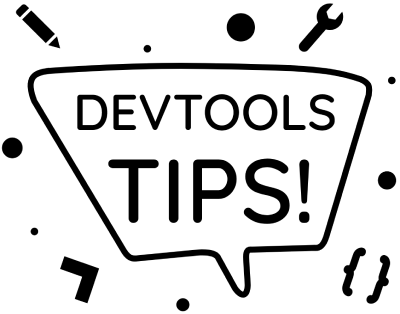
See the accessibility tree
Devtools Tips > See the accessibility tree
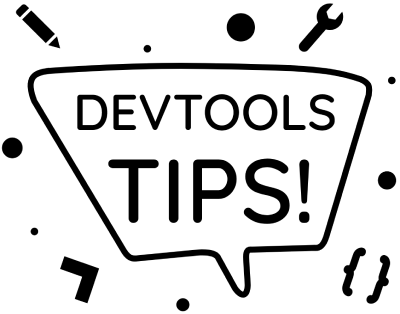
Name evaluated files with the sourceURL pragma
Devtools Tips > Name evaluated files with the sourceURL pragma
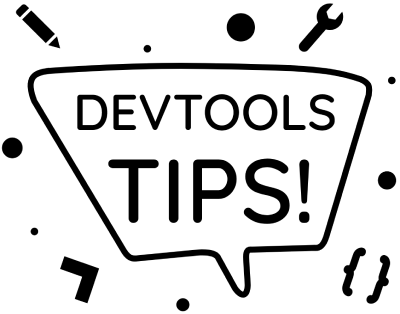
Simulate the Window Controls Overlay feature for PWA
Devtools Tips > Simulate the Window Controls Overlay feature for PWA
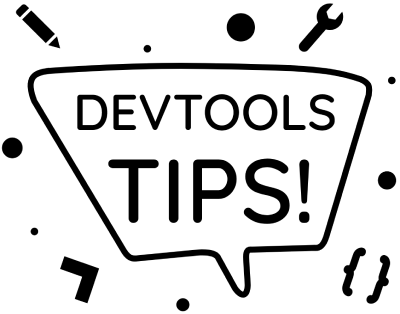
Inspect the user-agent DOM
Devtools Tips > Inspect the user-agent DOM
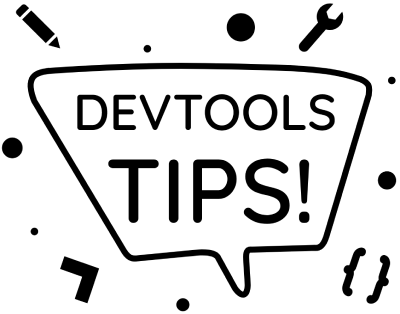
Explain console errors by using artificial intelligence
Devtools Tips > Explain console errors by using artificial intelligence
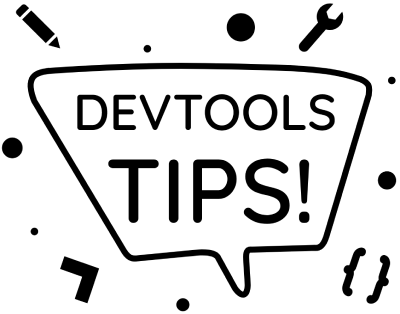
Enable DevTools in Safari
Devtools Tips > Enable DevTools in Safari
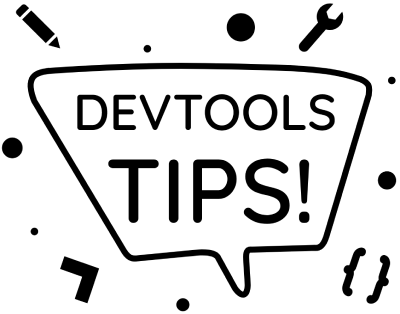
Block DevTools
Devtools Tips > Block DevTools
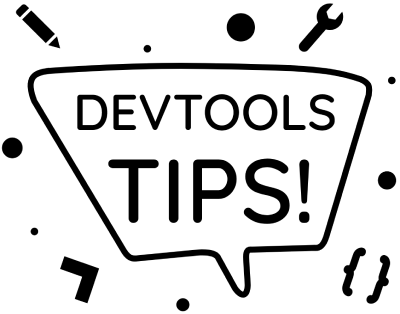
List all event listeners on the entire page
Devtools Tips > List all event listeners on the entire page
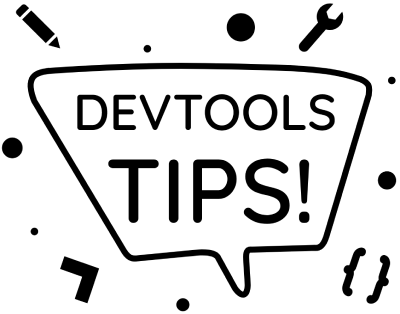
Debug popups that appear on hover
Devtools Tips > Debug popups that appear on hover
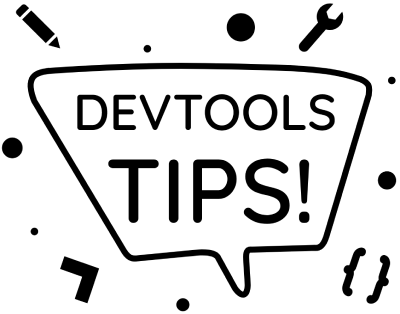
Force execution, skipping breakpoints, when paused
Devtools Tips > Force execution, skipping breakpoints, when paused
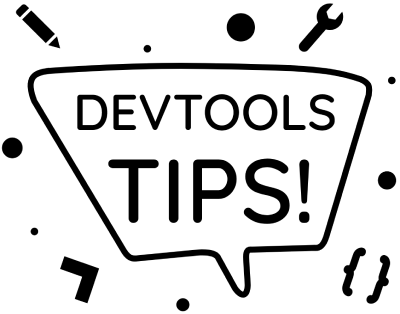
See the viewport size
Devtools Tips > See the viewport size