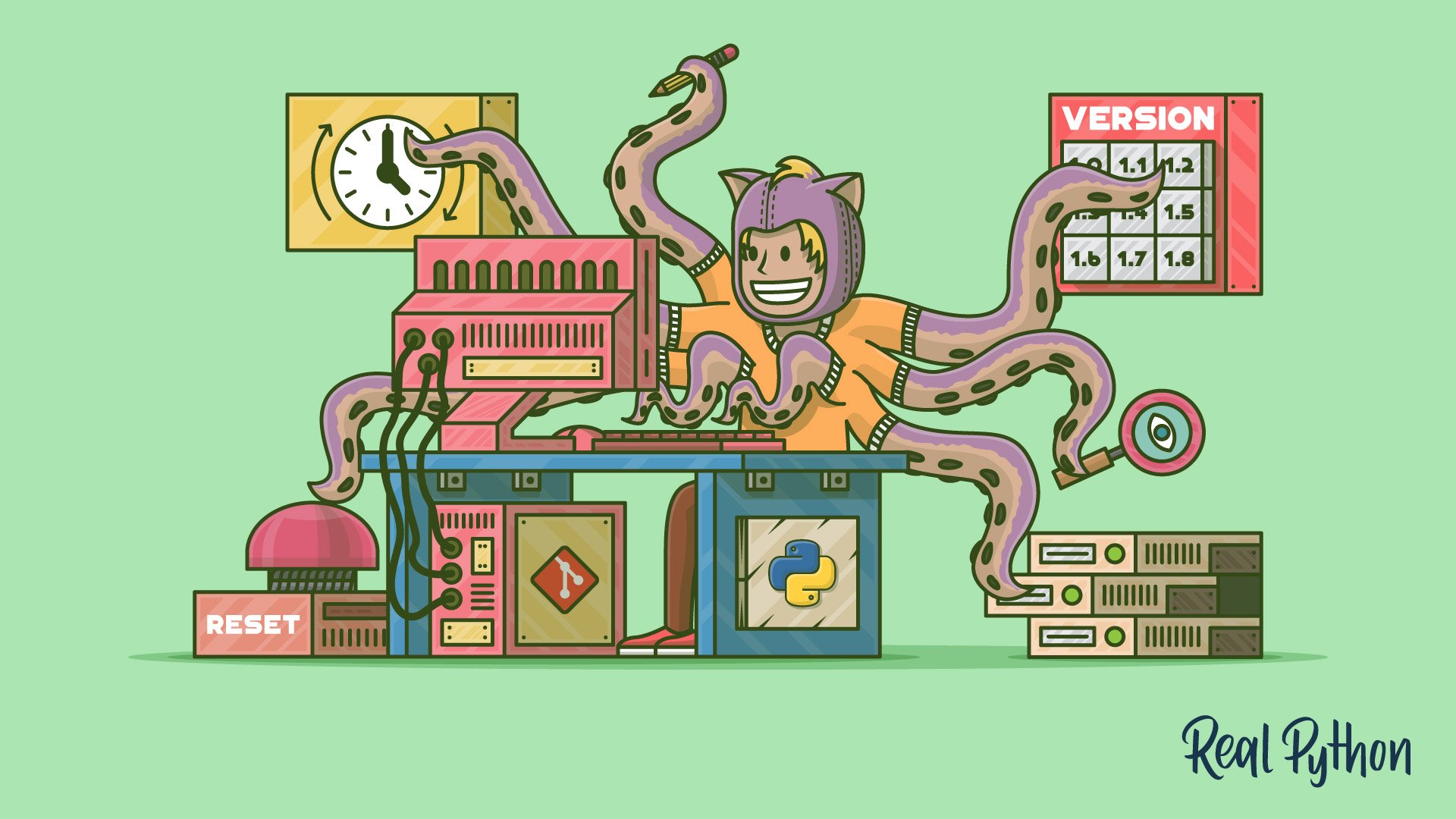
Resolving Merge Conflicts
Resolving Merge Conflicts êŽë š
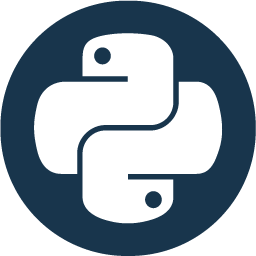
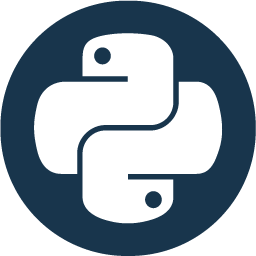
When youâre new to Git, merge conflicts seem like a scary thing, but with a little practice and a few tricks, they can become much easier to deal with.
Letâs start with some of the tricks that can make this easier. The first one changes the format of how conflicts are shown.
diff3
Format
Weâll walk through a simple example to see what Git does by default and what options we have to make it easier. To do this, create a new file, merge.py
, that looks like this:
def display():
print("Welcome to my project!")
Add and commit this file to your branch master
, and this will be your baseline commit. Youâll create branches that modify this file in different ways, and then youâll see how to resolve the merge conflict.
You now need to create separate branches that will have conflicting changes. Youâve seen how this is done before, so I wonât describe it in detail:
git checkout -b mergebranch
#
# Switched to a new branch 'mergebranch'
vi merge.py # edit file to change 'project' to 'program'
git add merge.py
git commit -m "change project to program"
#
# [mergebranch a775c38] change project to program
# 1 file changed, 1 insertion(+), 1 deletion(-)
git status
#
# On branch mergebranch
# nothing to commit, working directory clean
git checkout master
#
# Switched to branch 'master'
vi merge.py # edit file to add 'very cool' before project
git add merge.py
git commit -m "added description of project"
#
# [master ab41ed2] added description of project
# 1 file changed, 1 insertion(+), 1 deletion(-)
git show-branch master mergebranch
#
# * [master] added description of project
# ! [mergebranch] change project to program
# --
# * [master] added description of project
# + [mergebranch] change project to program
# *+ [master^] baseline for merging
At this point you have conflicting changes on mergebranch
and master
. Using the show-branch
command we learned in our Intro tutorial, you can see this visually on the command line:
git show-branch master mergebranch
#
# * [master] added description of project
# ! [mergebranch] change project to program
# --
# * [master] added description of project
# + [mergebranch] change project to program
# *+ [master^] baseline for merging
Youâre on branch master
, so letâs try to merge in mergebranch
. Since youâve made the changes with the intent of creating a merge conflict, lets hope that happens:
git merge mergebranch
#
# Auto-merging merge.py
# CONFLICT (content): Merge conflict in merge.py
# Automatic merge failed; fix conflicts and then commit the result.
As you expected, thereâs a merge conflict. If you look at status, thereâs a good deal of useful information there. Not only does it say that youâre in the middle of a merge, You have unmerged paths
, but it also shows you which files are modified, merge.py
:
git status
#
# On branch master
# You have unmerged paths.
# (fix conflicts and run "git commit")
#
# Unmerged paths:
# (use "git add <file>..." to mark resolution)
#
# both modified: merge.py
#
# no changes added to commit (use "git add" and/or "git commit -a")
You have done all that work to get to the point of having a merge conflict. Now you can start learning about how to resolve it! For this first part, youâll be working with the command line tools and your editor. After that, youâll get fancy and look at using visual diff tools to solve the problem.
When you open merge.py
in your editor, you can see what Git produced:
def display():
<<<<<<< HEAD
print("Welcome to my very cool project!")
=======
print("Welcome to my program!")
>>>>>>> mergebranch
Git uses diff
syntax from Linux to display the conflict. The top portion, between <<<<<<< HEAD
and =======
, are from HEAD, which in your case is master
. The bottom portion, between =======
and >>>>>>> mergebranch
are from, you guessed it, mergebranch
.
Now, in this very simple example, itâs pretty easy to remember which changes came from where and how we should merge this, but thereâs a setting you can enable which will make this easier.
The diff3
setting modifies the output of merge conflicts to more closely approximate a three-way merge, meaning in this case that it will show you whatâs in master
, followed by what it looked like in the common ancestor, followed by what it looks like in mergebranch
:
def display():
<<<<<<< HEAD
print("Welcome to my very cool project!")
||||||| merged common ancestors
print("Welcome to my project!")
=======
print("Welcome to my program!")
>>>>>>> mergebranch
Now that you can see the starting point, âWelcome to my project!â, you can see exactly what change was made on master
and what change was made on mergebranch
. This might not seem like a big deal on such a simple example, but it can make a huge difference on large conflicts, especially merges where someone else made some of the changes.
You can set this option in Git globally by issuing the following command:
git config --global merge.conflictstyle diff3
Okay, so you understand how to see the conflict. Letâs go through how to fix it. Start by editing the file, removing all of the markers Git added, and making the one conflicting line correct:
def display():
print("Welcome to my very cool program!")
You then add your modified file to the index and commit your merge. This will finish the merge process and create the new node:
git add merge.py
git commit
#
# [master a56a01e] Merge branch 'mergebranch'
git log --oneline
#
# a56a01e Merge branch 'mergebranch'
# ab41ed2 added description of project
# a775c38 change project to program
# f29b775 baseline for merging
Merge conflicts can happen while youâre cherry-picking, too. The process when you are cherry-picking is slightly different. Instead of using the git commit
command, you use the git cherry-pick --continue
command. Donât worry, Git will tell you in the status message which command you need to use. You can always go back and check that to be sure.
git mergetool
Similar to git difftool
, Git will allow you to configure a visual diff tool to deal with three-way merges. It knows about several different tools on different operating systems. You can see the list of tools it knows about on your system by using the command below. On my Linux machine, it shows the following:
git mergetool --tool-help
#
# 'git mergetool --tool=<tool>' may be set to one of the following:
# araxis
# gvimdiff
# gvimdiff2
# gvimdiff3
# meld
# vimdiff
# vimdiff2
# vimdiff3
#
# The following tools are valid, but not currently available:
# bc
# bc3
# codecompare
# deltawalker
# diffmerge
# diffuse
# ecmerge
# emerge
# kdiff3
# opendiff
# p4merge
# tkdiff
# tortoisemerge
# winmerge
# xxdiff
#
# Some of the tools listed above only work in a windowed
# environment. If run in a terminal-only session, they will fail.
Also similar to difftool
, you can configure the mergetool
options globally to make it easier to use:
git config --global merge.tool meld
git config --global mergetool.prompt false
The final option, mergetool.prompt
, tells Git not to prompt you each time it opens a window. This might not sound annoying, but when your merge involves several files it will prompt you between each of them.