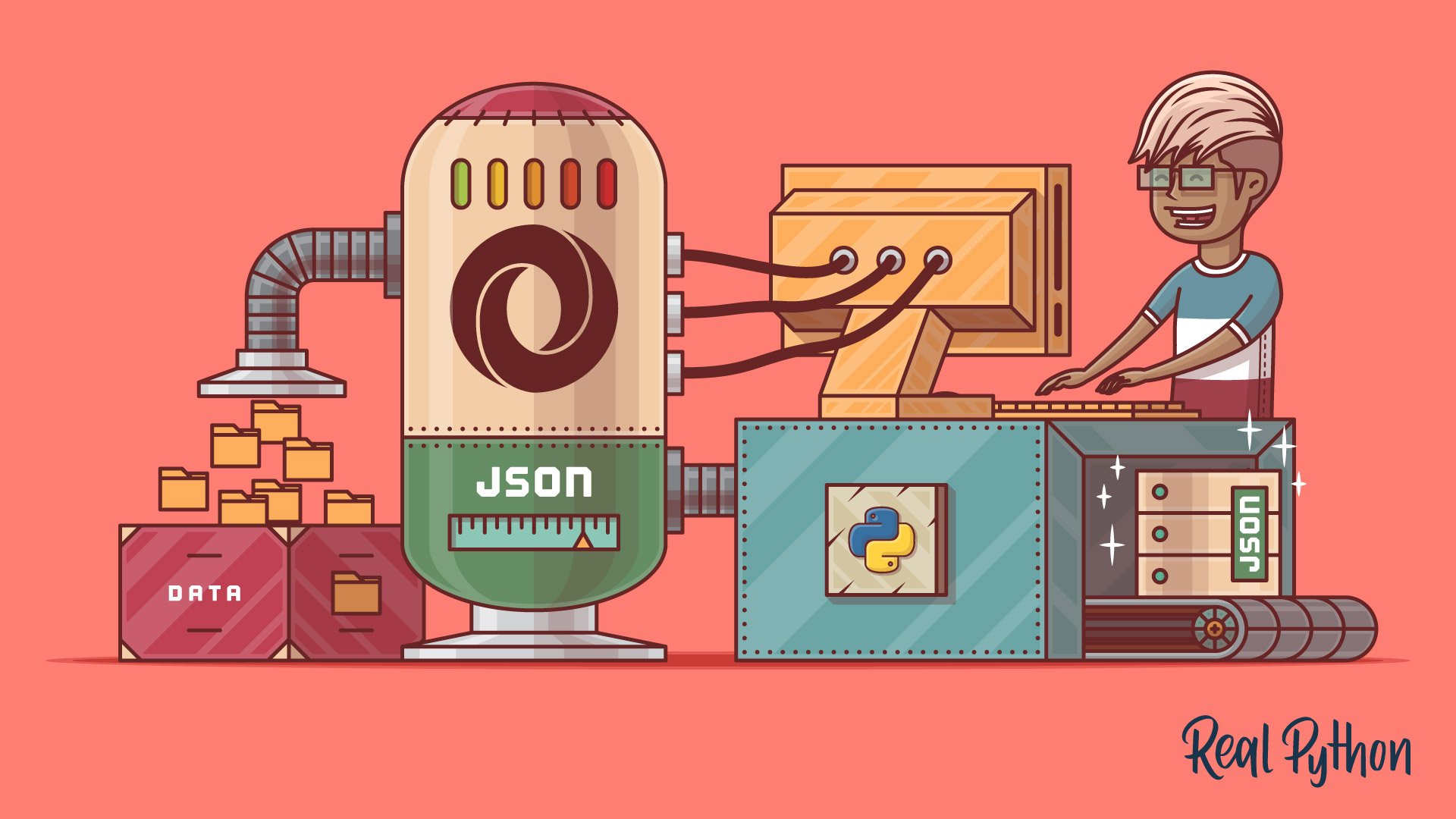
Writing JSON With Python
Writing JSON With Python êŽë š
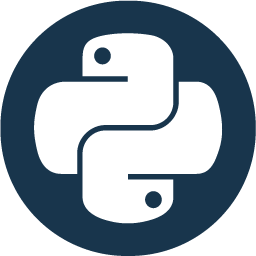
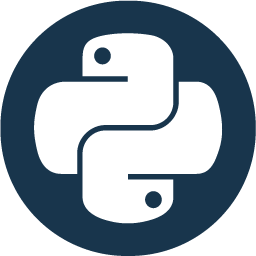
Python supports the JSON format through the built-in module named json
. The json
module is specifically designed for reading and writing strings formatted as JSON. That means you can conveniently convert Python data types into JSON data and the other way around.
The act of converting data into the JSON format is referred to as serialization. This process involves transforming data into a series of bytes for storage or transmission over a network. The opposite process, deserialization, involves decoding data from the JSON format back into a usable form within Python.
Youâll start with the serialization of Python code into JSON data with the help of the json
module.
Convert Python Dictionaries to JSON
One of the most common actions when working with JSON in Python is to convert a Python dictionary into a JSON object. To get an impression of how this works, hop over to your Python REPL and follow along with the code below:
import json
food_ratings = {"organic dog food": 2, "human food": 10}
json.dumps(food_ratings)
#
# '{"organic dog food": 2, "human food": 10}'
After importing the json
module, you can use .dumps()
to convert a Python dictionary to a JSON-formatted string, which represents a JSON object.
Itâs important to understand that when you use .dumps()
, you get a Python string in return. In other words, you donât create any kind of JSON data type. The result is similar to what youâd get if you used Pythonâs built-in str()
function:
str(food_ratings)
#
# "{'organic dog food': 2, 'human food': 10}"
Using json.dumps()
gets more interesting when your Python dictionary doesnât contain strings as keys or when values donât directly translate to a JSON format:
numbers_present = {1: True, 2: True, 3: False}
json.dumps(numbers_present)
#
# '{"1": true, "2": true, "3": false}'
In the numbers_present
dictionary, the keys 1
, 2
, and 3
are numbers. Once you use .dumps()
, the dictionary keys become strings in the JSON-formatted string.
Note: When you convert a dictionary to JSON, the dictionary keys will always be strings in JSON.
The Boolean Python values of your dictionary become JSON Booleans. As mentioned before, the tiny but significant difference between JSON Booleans and Python Booleans is that JSON Booleans are lowercase.
The cool thing about Pythonâs json
module is that it takes care of the conversion for you. This can come in handy when youâre using variables as dictionary keys:
dog_id = 1
dog_name = "Frieda"
dog_registry = {dog_id: {"name": dog_name}}
json.dumps(dog_registry)
#
# '{"1": {"name": "Frieda"}}'
When converting Python data types into JSON, the json
module receives the evaluated values. While doing so, json
sticks tightly to the JSON standard. For example, when converting integer keys like 1
to the string "1"
.
Serialize Other Python Data Types to JSON
The json
module allows you to convert common Python data types to JSON. Hereâs an overview of all Python data types and values that you can convert to JSON values:
Python | JSON |
---|---|
dict | object |
list | array |
tuple | array |
str | string |
int | number |
float | number |
True | true |
False | false |
None | null |
Note that different Python data types like lists and tuples serialize to the same JSON array
data type. This can cause problems when you convert JSON data back to Python, as the data type may not be the same as before. Youâll explore this pitfall later in this tutorial when you learn how to read JSON.
Dictionaries are probably the most common Python data type that youâll use as a top-level value in JSON. But you can convert the data types listed above just as smoothly as dictionaries using json.dumps()
. Take a Boolean or a list, for example:
>>> json.dumps(True)
'true'
>>> json.dumps(["eating", "sleeping", "barking"])
'["eating", "sleeping", "barking"]'
A JSON document may contain a single scalar value, like a number, at the top level. Thatâs still valid JSON. But more often than not, you want to work with a collection of key-value pairs. Similar to how not every data type can be used as a dictionary key in Python, not all keys can be converted into JSON key strings:
Python Data Type | Allowed as JSON Key |
---|---|
dict | â |
list | â |
tuple | â |
str | â |
int | â |
float | â |
bool | â |
None | â |
You canât use dictionaries, lists, or tuples as JSON keys. For dictionaries and lists, this rule makes sense as theyâre not hashable. But even when a tuple is hashable and allowed as a key in a dictionary, youâll get a TypeError
when you try to use a tuple as a JSON key:
available_nums = {(1, 2): True, 3: False}
json.dumps(available_nums)
#
# Traceback (most recent call last):
# ...
# TypeError: keys must be str, int, float, bool or None, not tuple
By providing the skipkeys
argument, you can prevent getting a TypeError
when creating JSON data with unsupported Python keys:
json.dumps(available_nums, skipkeys=True)
#
# '{"3": false}'
When you set skipkeys
in json.dumps()
to True
, then Python skips the keys that are not supported and would otherwise raise a TypeError
. The result is a JSON-formatted string that only contains a subset of the input dictionary. In practice, you usually want your JSON data to resemble the input object as close as possible. So, you must use skipkeys
with caution to not lose information when calling json.dumps()
.
Note
If youâre ever in a situation where you need to convert an unsupported object into JSON, then you can consider creating a subclass of the JSONEncoder
and implementing a .default()
method.
When you use json.dumps()
, you can use additional arguments to control the look of the resulting JSON-formatted string. For example, you can sort the dictionary keys by setting the sort_keys
parameter to True
:
toy_conditions = {"chew bone": 7, "ball": 3, "sock": -1}
json.dumps(toy_conditions, sort_keys=True)
#
# '{"ball": 3, "chew bone": 7, "sock": -1}'
When you set sort_keys
to True
, then Python sorts the keys alphabetically for you when serializing a dictionary. Sorting the keys of a JSON object can come in handy when your dictionary keys formerly represented the column names of a database, and you want to display them in an organized fashion to the user.
Another notable parameter of json.dumps()
is indent
, which youâll probably use the most when serializing JSON data. Youâll explore indent
later in this tutorial in the prettify JSON section.
When you convert Python data types into the JSON format, you usually have a goal in mind. Most commonly, youâll use JSON to persist and exchange data. To do so, you need to save your JSON data outside of your running Python program. Conveniently, youâll explore saving JSON data to a file next.
Write a JSON File With Python
The JSON format can come in handy when you want to save data outside of your Python program. Instead of spinning up a database, you may decide to use a JSON file to store data for your workflows. Again, Python has got you covered.
To write Python data into an external JSON file, you use json.dump()
. This is a similar function to the one you saw earlier, but without the s at the end of its name:
import json
dog_data = {
"name": "Frieda",
"is_dog": True,
"hobbies": ["eating", "sleeping", "barking",],
"age": 8,
"address": {
"work": None,
"home": ("Berlin", "Germany",),
},
"friends": [
{
"name": "Philipp",
"hobbies": ["eating", "sleeping", "reading",],
}, {
"name": "Mitch",
"hobbies": ["running", "snacking",],
},
],
}
with open("hello_frieda.json", mode="w", encoding="utf-8") as write_file:
json.dump(dog_data, write_file)
In lines 3 to 22, you define a dog_data
dictionary that you write to a JSON file in line 25 using a context manager. To properly indicate that the file contains JSON data, you set the file extension to .json
.
When you use open()
, then itâs good practice to define the encoding. For JSON, you commonly want to use "utf-8"
as the encoding when reading and writing files:
Source (docs.python.org
)
The RFC requires that JSON be represented using either UTF-8, UTF-16, or UTF-32, with UTF-8 being the recommended default for maximum interoperability.
The json.dump()
function has two required arguments:
- The object you want to write
- The file you want to write into
Other than that, there are a bunch of optional parameters for json.dump()
. The optional parameters of json.dump()
are the same as for json.dumps()
. Youâll investigate some of them later in this tutorial when you prettify and minify JSON files.