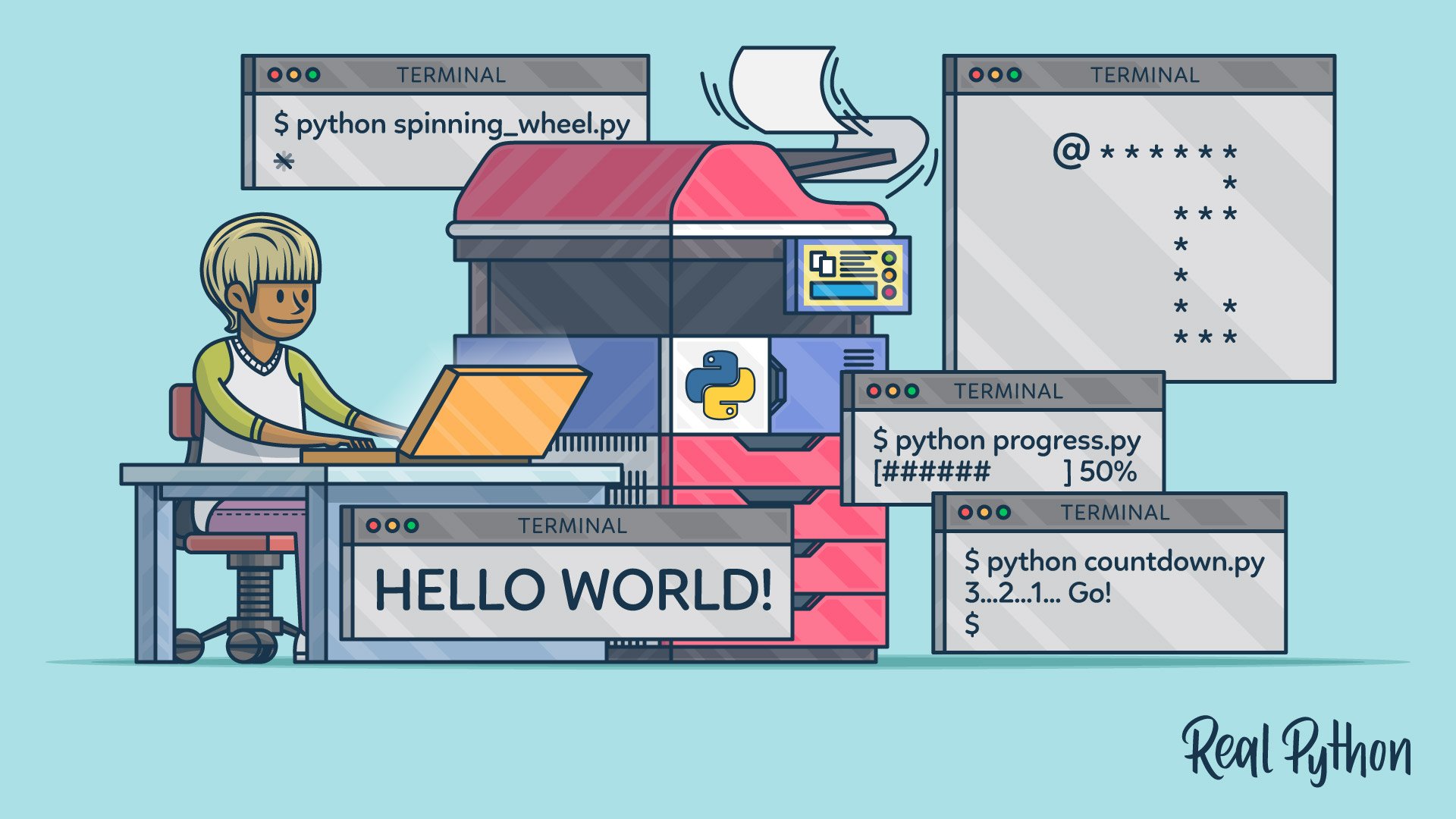
Python Print Counterparts
Python Print Counterparts êŽë š
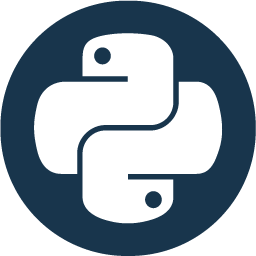
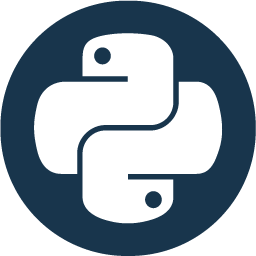
By now, you know a lot of what there is to know about print()
! The subject, however, wouldnât be complete without talking about its counterparts a little bit. While print()
is about the output, there are functions and libraries for the input.
Built-In
Python comes with a built-in function for accepting input from the user, predictably called input()
. It accepts data from the standard input stream, which is usually the keyboard:
name = input('Enter your name: ')
#
# Enter your name: jdoe
print(name)
#
# jdoe
The function always returns a string, so you might need to parse it accordingly:
try:
age = int(input('How old are you? '))
except ValueError:
pass
The prompt parameter is completely optional, so nothing will show if you skip it, but the function will still work:
x = input()
#
# hello world
print(x)
#
# hello world
Nevertheless, throwing in a descriptive call to action makes the user experience so much better.
Note
To read from the standard input in Python 2, you have to call raw_input()
instead, which is yet another built-in. Unfortunately, thereâs also a misleadingly named input()
function, which does a slightly different thing.
In fact, it also takes the input from the standard stream, but then it tries to evaluate it as if it was Python code. Because thatâs a potential security vulnerability, this function was completely removed from Python 3, while raw_input()
got renamed to input()
.
Hereâs a quick comparison of the available functions and what they do:
Python 2 | Python 3 |
---|---|
raw_input() | input() |
input() | eval(input()) |
As you can tell, itâs still possible to simulate the old behavior in Python 3.
Asking the user for a password with input()
is a bad idea because itâll show up in plaintext as theyâre typing it. In this case, you should be using the getpass()
function instead, which masks typed characters. This function is defined in a module under the same name, which is also available in the standard library:
from getpass import getpass
password = getpass()
#
# Password:
print(password)
#
# s3cret
The getpass
module has another function for getting the userâs name from an environment variable:
from getpass import getuser
getuser()
#
# 'jdoe'
Pythonâs built-in functions for handling the standard input are quite limited. At the same time, there are plenty of third-party packages, which offer much more sophisticated tools.
Third-Party
There are external Python packages out there that allow for building complex graphical interfaces specifically to collect data from the user. Some of their features include:
- Advanced formatting and styling
- Automated parsing, validation, and sanitization of user data
- A declarative style of defining layouts
- Interactive autocompletion
- Mouse support
- Predefined widgets such as checklists or menus
- Searchable history of typed commands
- Syntax highlighting
Demonstrating such tools is outside of the scope of this article, but you may want to try them out. I personally got to know about some of those through the Python Bytes Podcast. Here they are:
Nonetheless, itâs worth mentioning a command line tool called rlwrap
that adds powerful line editing capabilities to your Python scripts for free. You donât have to do anything for it to work!
Letâs assume you wrote a command-line interface that understands three instructions, including one for adding numbers:
print('Type "help", "exit", "add a [b [c ...]]"')
while True:
command, *arguments = input('~ ').split(' ')
if len(command) > 0:
if command.lower() == 'exit':
break
elif command.lower() == 'help':
print('This is help.')
elif command.lower() == 'add':
print(sum(map(int, arguments)))
else:
print('Unknown command')
At first glance, it seems like a typical prompt when you run it:
python calculator.py
#
# Type "help", "exit", "add a [b [c ...]]"
add 1 2 3 4
# 10
aad 2 3
# Unknown command
exit
But as soon as you make a mistake and want to fix it, youâll see that none of the function keys work as expected. Hitting the Left arrow, for example, results in this instead of moving the cursor back:
python calculator.py
#
# Type "help", "exit", "add a [b [c ...]]"
aad^[[D
Now, you can wrap the same script with the rlwrap
command. Not only will you get the arrow keys working, but youâll also be able to search through the persistent history of your custom commands, use autocompletion, and edit the line with shortcuts:
rlwrap python calculator.py
#
# Type "help", "exit", "add a [b [c ...]]"
# (reverse-i-search)`a': add 1 2 3 4`
Isnât that great?