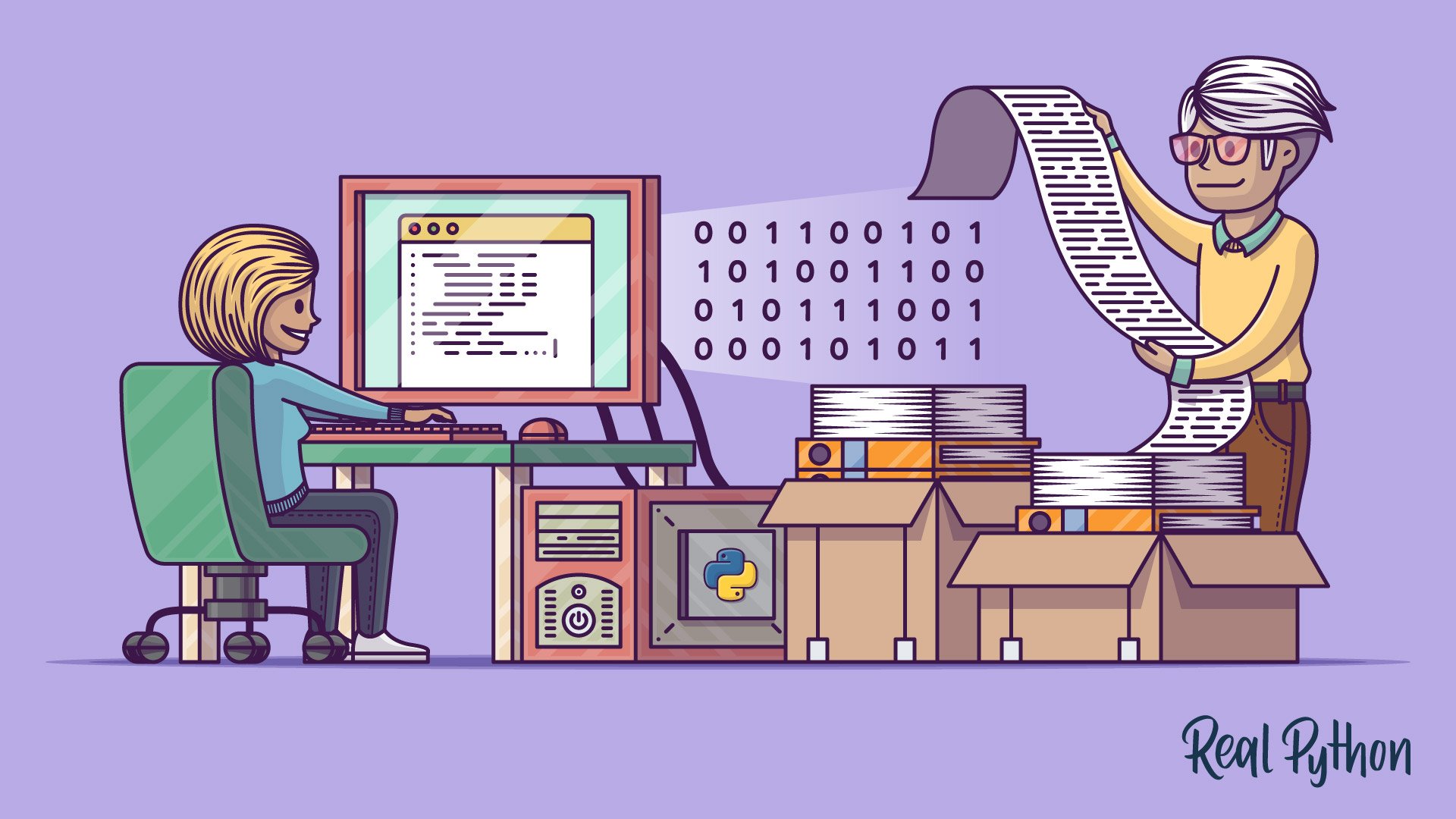
Opening and Closing a File in Python
Opening and Closing a File in Python 관련
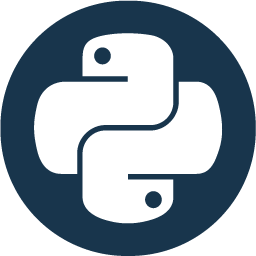
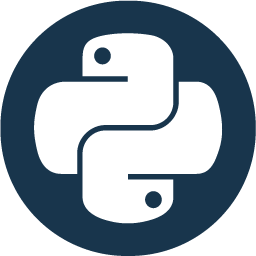
When you want to work with a file, the first thing to do is to open it. This is done by invoking the open()
built-in function. open()
has a single required argument that is the path to the file. open()
has a single return, the file object:
file = open('dog_breeds.txt')
After you open a file, the next thing to learn is how to close it.
Warning
You should always make sure that an open file is properly closed. To learn why, check out the Why Is It Important to Close Files in Python? tutorial.
It’s important to remember that it’s your responsibility to close the file. In most cases, upon termination of an application or script, a file will be closed eventually. However, there is no guarantee when exactly that will happen. This can lead to unwanted behavior including resource leaks. It’s also a best practice within Python (Pythonic) to make sure that your code behaves in a way that is well defined and reduces any unwanted behavior.
When you’re manipulating a file, there are two ways that you can use to ensure that a file is closed properly, even when encountering an error. The first way to close a file is to use the try-finally
block:
reader = open('dog_breeds.txt')
try:
# Further file processing goes here
finally:
reader.close()
If you’re unfamiliar with what the try-finally
block is, check out Python Exceptions: An Introduction.
The second way to close a file is to use the with
statement:
with open('dog_breeds.txt') as reader:
# Further file processing goes here
The with
statement automatically takes care of closing the file once it leaves the with
block, even in cases of error. I highly recommend that you use the with
statement as much as possible, as it allows for cleaner code and makes handling any unexpected errors easier for you.
Most likely, you’ll also want to use the second positional argument, mode
. This argument is a string that contains multiple characters to represent how you want to open the file. The default and most common is 'r'
, which represents opening the file in read-only mode as a text file:
with open('dog_breeds.txt', 'r') as reader:
# Further file processing goes here
Other options for modes are fully documented online, but the most commonly used ones are the following:
Character | Meaning |
---|---|
'r' | Open for reading (default) |
'w' | Open for writing, truncating (overwriting) the file first |
'rb' or 'wb' | Open in binary mode (read/write using byte data) |
Let’s go back and talk a little about file objects. A file object is:
Glossary — Python 3.13.2 documentation (docs.python.org
)
“an object exposing a file-oriented API (with methods such as
read()
orwrite()
) to an underlying resource.”
There are three different categories of file objects:
- Text files
- Buffered binary files
- Raw binary files
Each of these file types are defined in the io
module. Here’s a quick rundown of how everything lines up.
Text File Types
A text file is the most common file that you’ll encounter. Here are some examples of how these files are opened:
open('abc.txt')
open('abc.txt', 'r')
open('abc.txt', 'w')
With these types of files, open()
will return a TextIOWrapper
file object:
file = open('dog_breeds.txt')
type(file)
#
# <class '_io.TextIOWrapper'>
This is the default file object returned by open()
.
Buffered Binary File Types
A buffered binary file type is used for reading and writing binary files. Here are some examples of how these files are opened:
open('abc.txt', 'rb')
open('abc.txt', 'wb')
With these types of files, open()
will return either a BufferedReader
or BufferedWriter
file object:
file = open('dog_breeds.txt', 'rb')
type(file)
#
# <class '_io.BufferedReader'>
file = open('dog_breeds.txt', 'wb')
type(file)
#
# <class '_io.BufferedWriter'>
Raw File Types
A raw file type is:
io — Core tools for working with streams — Python 3.7.17 documentation (docs.python.org
)
“generally used as a low-level building-block for binary and text streams.”
It is therefore not typically used.
Here’s an example of how these files are opened:
open('abc.txt', 'rb', buffering=0)
With these types of files, open()
will return a FileIO
file object:
file = open('dog_breeds.txt', 'rb', buffering=0)
type(file)
#
# <class '_io.FileIO'>