How to show an action sheet
How to show an action sheet 관련
Updated for Xcode 15
Updated in iOS 15
SwiftUI allows us to show a selection of options to the user with using its confirmationDialog()
modifier, but if you’re targeting iOS 14 or earlier you need to use ActionSheet
instead. I’ll show you both here, but if you’re targeting iOS 15 or later, or if you want to support macOS, you should use confirmationDialog()
.
To create your action sheet using confirmationDialog()
, provide some title text, a binding to determine when the sheet should be shown, and optionally also whether you want the title text to appear – if you don’t specify this, SwiftUI will decide for you based on context.
So, this shows a sheet asking the user to select a paint color from three options:
struct ContentView: View {
@State private var showingOptions = false
@State private var selection = "None"
var body: some View {
VStack {
Text(selection)
Button("Confirm paint color") {
showingOptions = true
}
.confirmationDialog("Select a color", isPresented: $showingOptions, titleVisibility: .visible) {
Button("Red") {
selection = "Red"
}
Button("Green") {
selection = "Green"
}
Button("Blue") {
selection = "Blue"
}
}
}
}
}
Tips
This API uses a standard SwiftUI Button
for each action, so you can attach a role such as .destructive
to have SwiftUI color it appropriately.
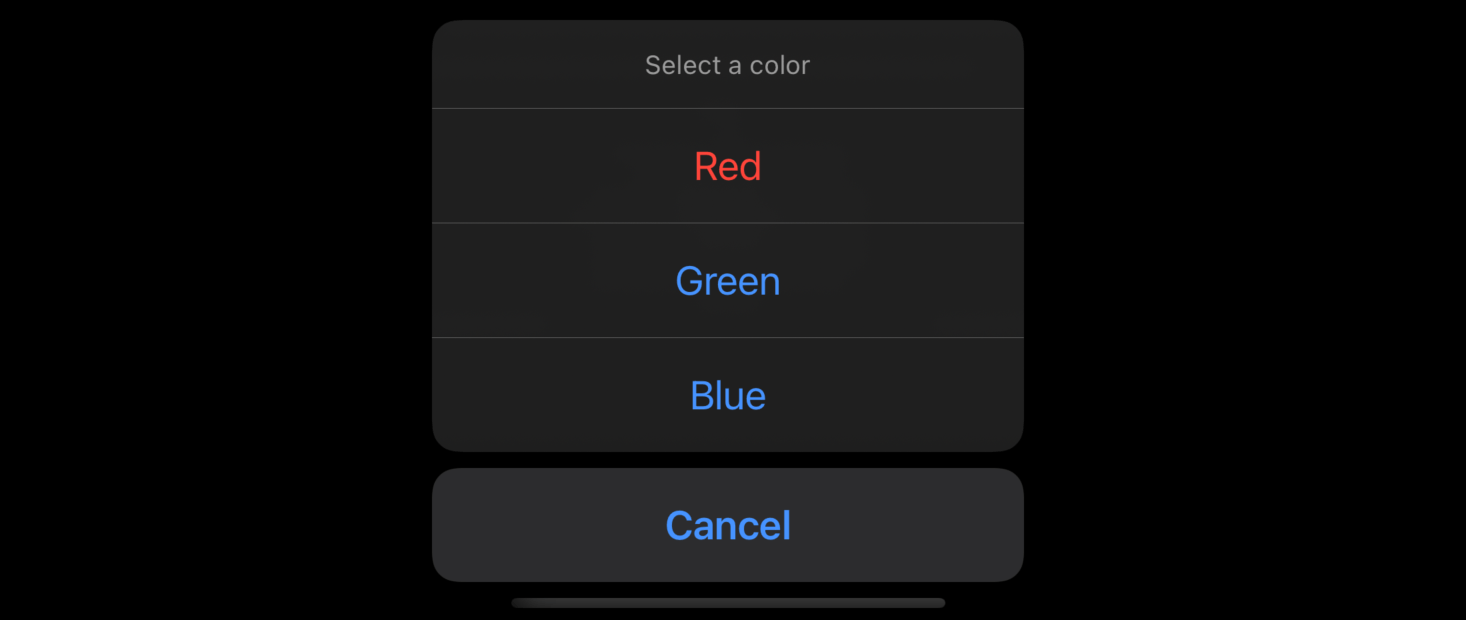
Because this new API is more flexible, we can actually collapse down those actions into a simple loop using ForEach
:
struct ContentView: View {
@State private var showingOptions = false
@State private var selection = "None"
var body: some View {
VStack {
Text(selection)
Button("Confirm paint color") {
showingOptions = true
}
.confirmationDialog("Select a color", isPresented: $showingOptions, titleVisibility: .visible) {
ForEach(["Red", "Green", "Blue"], id: \.self) { color in
Button(color) {
selection = color
}
}
}
}
}
}
If you need to target iOS 14 or below you should use the older ActionSheet
approach to achieve the same result. This also works by defining a property to track whether the action sheet should be visible or not.
For example, here is an example view that triggers an action sheet when a button is tapped:
struct ContentView: View {
@State private var showingOptions = false
@State private var selection = "None"
var body: some View {
VStack {
Text(selection)
Button("Show Options") {
showingOptions = true
}
.actionSheet(isPresented: $showingOptions) {
ActionSheet(
title: Text("Select a color"),
buttons: [
.default(Text("Red")) {
selection = "Red"
},
.default(Text("Green")) {
selection = "Green"
},
.default(Text("Blue")) {
selection = "Blue"
},
]
)
}
}
}
}