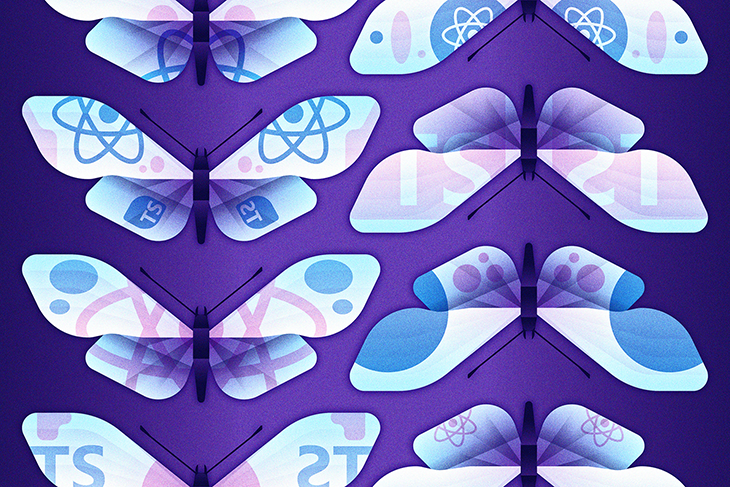
Building a simple polymorphic component
May 25, 2022About 1 min
Building a simple polymorphic component 관련
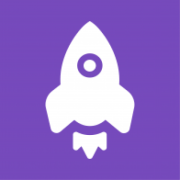
Build strongly typed polymorphic components with React and TypeScript
Learn how to build strongly typed polymorphic React components with TypeScript, using familiar Chakra UI and MUI component props as guides.

Build strongly typed polymorphic components with React and TypeScript
Learn how to build strongly typed polymorphic React components with TypeScript, using familiar Chakra UI and MUI component props as guides.
Contrary to what you may think, building your first polymorphic component is quite straightforward. Here’s a basic implementation:
const MyComponent = ({ as, children }) => {
const Component = as || "span";
return <Component>{children}</Component>;
};
Note here that the polymorphic prop as
is similar to Chakra UI’s. This is the prop we expose to control the render element of the polymorphic component.
Secondly, note that the as
prop isn’t rendered directly. The following would be wrong:
const MyComponent = ({ as, children }) => {
// wrong render below 👇
return <as>{children}</as>;
};
When rendering an element type at runtime, you must first assign it to a capitalized variable, and then render the capitalized variable.
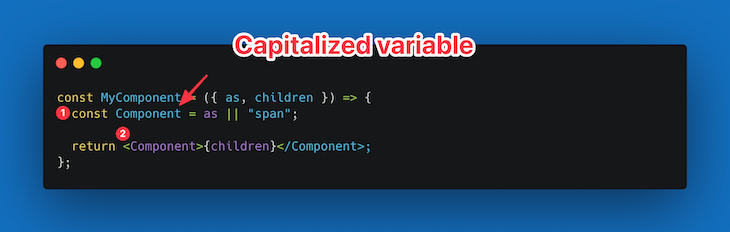
Now you can go ahead and use this component as follows:
<MyComponent as="button">Hello Polymorphic!<MyComponent>
<MyComponent as="div">Hello Polymorphic!</MyComponent>
<MyComponent as="span">Hello Polymorphic!</MyComponent>
<MyComponent as="em">Hello Polymorphic!</MyComponent>
Note that the different as
prop is passed to the rendered components above.
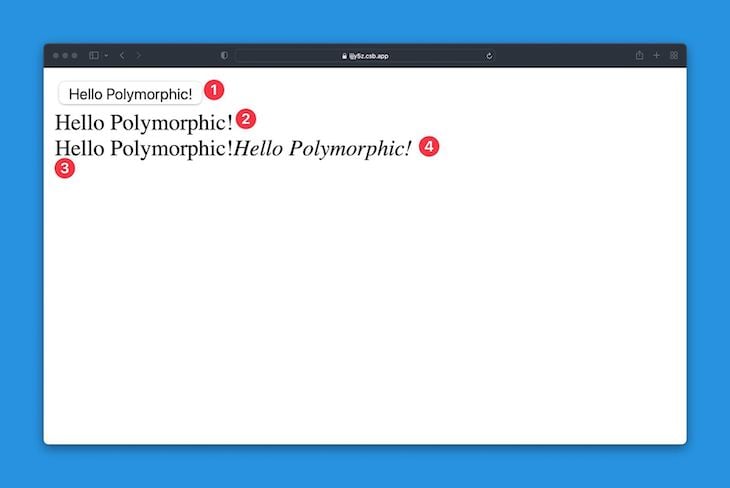