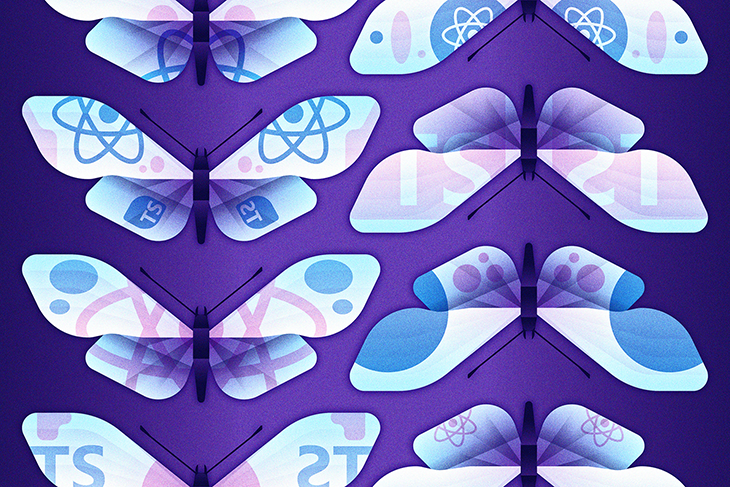
How to use TypeScript to build strongly typed polymorphic components in React
How to use TypeScript to build strongly typed polymorphic components in React êŽë š
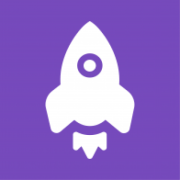

If youâre reading this, a prerequisite is that you already know some TypeScript â at least the basics. If you have no clue what TypeScript is, I strongly recommend giving this document a read first.
In this section, we will use TypeScript to solve the aforementioned concerns and build strongly typed polymorphic components.
The first two requirements we will start off with include:
- The
as
prop should not receive invalid HTML element strings - Wrong attributes should not be passed for valid elements
In the following section, we will introduce TypeScript generics to make our solution more robust, developer-friendly, and production-worthy.
Ensuring the as
prop only receives valid HTML element strings
Hereâs our current solution:
const MyComponent = ({ as, children }) => {
const Component = as || "span";
return <Component>{children}</Component>;
};
To make the next sections of this guide practical, weâll change the name of the component from MyComponent
to Text
and assume weâre building a polymorphic Text
component.
const Text = ({ as, children }) => {
const Component = as || "span";
return <Component>{children}</Component>;
};
Now, with your knowledge of generics, it becomes obvious that weâre better off representing as
with a generic type, i.e., a variable type based on whatever the user passes in.
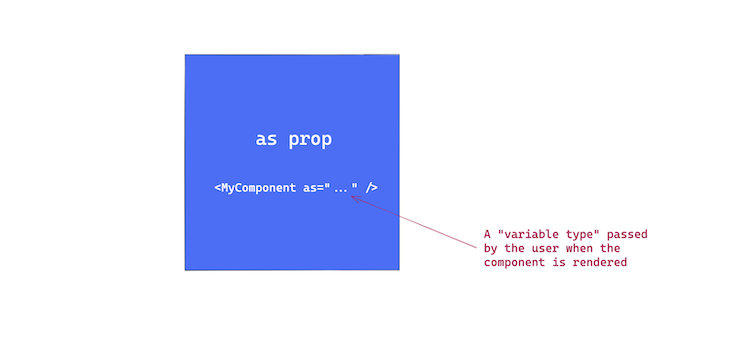
Letâs go ahead and take the first step as follows:
export const Text = <C>({
as,
children,
}: {
as?: C;
children: React.ReactNode;
}) => {
const Component = as || "span";
return <Component>{children}</Component>;
};
Note how the generic C
is defined and then passed on in the type definition for the prop as
.
However, if you wrote this seemingly perfect code, youâll have TypeScript yelling out numerous errors with more squiggly red lines than youâd like đ€·ââïž
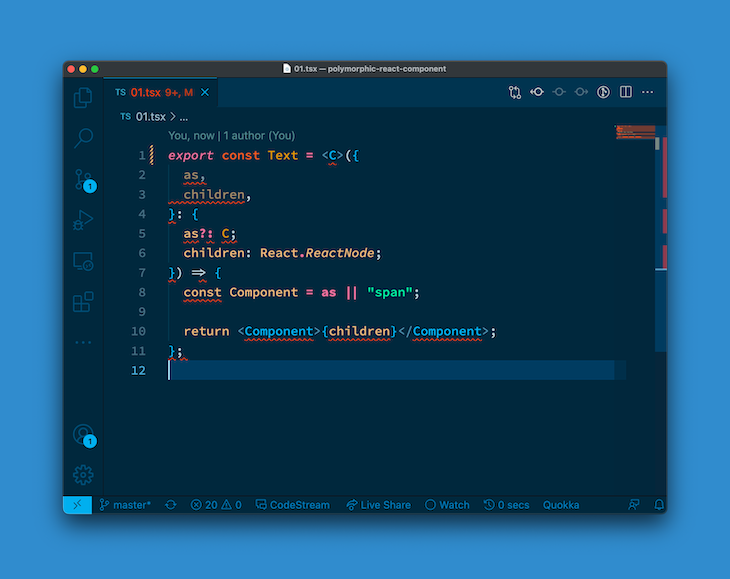
Whatâs going on here is a flaw in the syntax for generics in .tsx
files. There are two ways to solve this.
1. Add a comma after the generic declaration
This is the syntax for declaring multiple generics. Once you do this, the TypeScript compiler clearly understands your intent and the errors are banished.
// note the comma after "C" below đ
export const Text = <C,>({
as,
children,
}: {
as?: C;
children: React.ReactNode;
}) => {
const Component = as || "span";
return <Component>{children}</Component>;
};
2. Constrain the generic
The second option is to constrain the generic as you see fit. For starters, you can just use the unknown
type as follows:
// note the extends keyword below đ
export const Text = <C extends unknown>({
as,
children,
}: {
as?: C;
children: React.ReactNode;
}) => {
const Component = as || "span";
return <Component>{children}</Component>;
};
For now, Iâll stick to the second solution because itâs closer to our final solution. In most cases, though, I use the multiple generic syntax and just add a comma.
However, with our current solution, we get another TypeScript error:
JSX element type âComponentâ does not have any construct or call signatures. ts(2604)
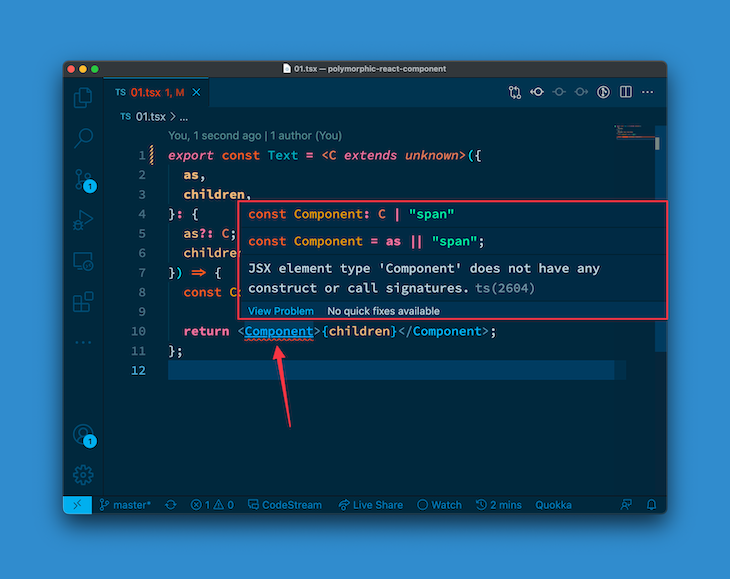
This is similar to the error we had when we worked with the echoLength
function. Just like accessing the length
property of an unknown variable type, the same may be said here: trying to render any generic type as a valid React component doesnât make sense.
We need to constrain the generic only to fit the mold of a valid React element type.
To achieve this, weâll leverage the internal React type: React.ElementType
, and make sure the generic is constrained to fit that type:
// look just after the extends keyword đ
export const Text = <C extends React.ElementType>({
as,
children,
}: {
as?: C;
children: React.ReactNode;
}) => {
const Component = as || "span";
return <Component>{children}</Component>;
};
Note that if youâre using an older version of React, you may have to import a newer React version as follows:
import React from 'react'
With this, we have no more errors!
Now, if you go ahead and use this component as follows, itâll work just fine:
<Text as="div">Hello Text world</Text>
However, if you pass an invalid as
prop, youâll now get an appropriate TypeScript error. Consider the example below:
<Text as="emmanuel">Hello Text world</Text>
And the error thrown:
Type ââemmanuelââ is not assignable to type âElementType | undefinedâ.
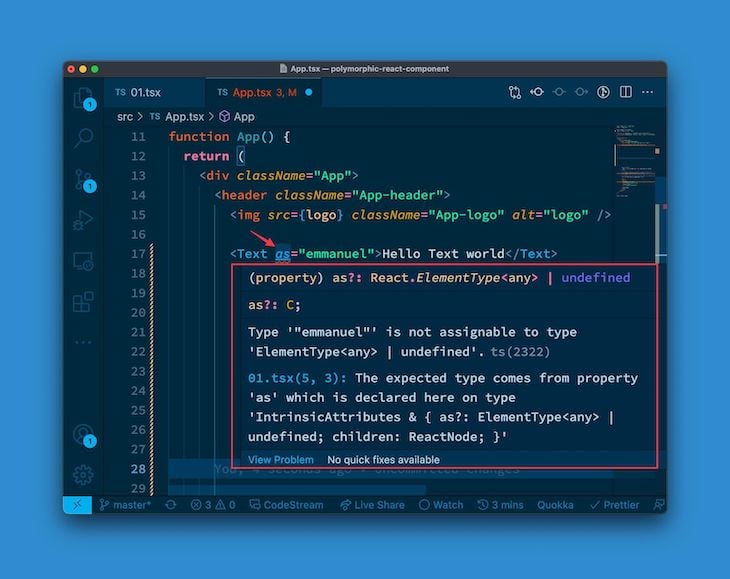
This is excellent! We now have a solution that doesnât accept gibberish for the as
prop and will also prevent against nasty typos, e.g., divv
instead of div
.
This is a much better developer experience!