How to draw a checkerboard
About 2 min
How to draw a checkerboard 관련
SwiftUI by Example
Back to Home
How to draw a checkerboard | SwiftUI by Example
How to draw a checkerboard
Updated for Xcode 15
SwiftUI’s paths don’t need to be continuous, isolated shapes, and can instead be multiple rectangles, ellipses, and more, all combined into one.
As an easy way of demonstrating this, we could write a shape that creates checkerboard patterns by building up a series of rectangles of a set number of rows and columns, like this:
struct Checkerboard: Shape {
let rows: Int
let columns: Int
func path(in rect: CGRect) -> Path {
var path = Path()
// figure out how big each row/column needs to be
let rowSize = rect.height / Double(rows)
let columnSize = rect.width / Double(columns)
// loop over all rows and columns, making alternating squares colored
for row in 0 ..< rows {
for column in 0 ..< columns {
if (row + column).isMultiple(of: 2) {
// this square should be colored; add a rectangle here
let startX = columnSize * Double(column)
let startY = rowSize * Double(row)
let rect = CGRect(x: startX, y: startY, width: columnSize, height: rowSize)
path.addRect(rect)
}
}
}
return path
}
}
// Create a checkerboard in a view
struct ContentView: View {
var body: some View {
Checkerboard(rows: 16, columns: 16)
.fill(.red)
.frame(width: 200, height: 200)
}
}
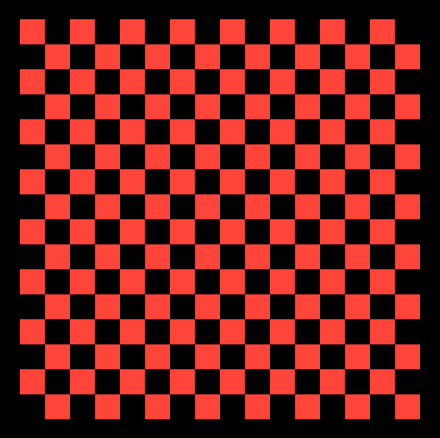
Similar solutions…
How to add Metal shaders to SwiftUI views using layer effects | SwiftUI by Example
How to add Metal shaders to SwiftUI views using layer effects
How to draw polygons and stars | SwiftUI by Example
How to draw polygons and stars
How to draw a border inside a view | SwiftUI by Example
How to draw a border inside a view
How to draw a custom path | SwiftUI by Example
How to draw a custom path
How to draw a shadow around a view | SwiftUI by Example
How to draw a shadow around a view