How to draw a custom path
How to draw a custom path 관련
Updated for Xcode 15
SwiftUI lets us draw custom paths by conforming to the Shape
protocol, so we can create our own shapes that work the same as Rectangle
, Capsule
, and Circle
. Conforming to this protocol isn’t hard, because all you need to do is support a path(in:)
method that accepts a CGRect
and returns a Path
. Even better, you can use any paths you’ve previously built using CGPath
or UIBezierPath
, then convert the result to a SwiftUI path.
If you want to use SwiftUI’s native Path
type, create a variable instance of it then add as many points or shapes as you need. Don’t think about colors, fills, or stroke widths – you’re focusing on the raw shape here, and those kinds of settings are provided when your custom path is used.
For example, we could make a creative effect by drawing a series of shrinking squares, then placing that shape into a SwiftUI view with a stroke and a size:
struct ShrinkingSquares: Shape {
func path(in rect: CGRect) -> Path {
var path = Path()
for i in stride(from: 1, through: 100, by: 5.0) {
let rect = CGRect(x: 0, y: 0, width: rect.width, height: rect.height)
let insetRect = rect.insetBy(dx: i, dy: i)
path.addRect(insetRect)
}
return path
}
}
struct ContentView: View {
var body: some View {
ShrinkingSquares()
.stroke()
.frame(width: 200, height: 200)
}
}
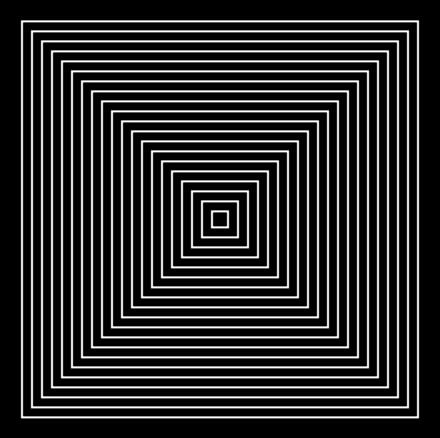