How to integrate SpriteKit using SpriteView
About 1 min
How to integrate SpriteKit using SpriteView 관련
SwiftUI by Example
Back to Home
How to integrate SpriteKit using SpriteView | SwiftUI by Example
How to integrate SpriteKit using SpriteView
Updated for Xcode 15
SwiftUI's SpriteView
lets us render any SKScene
subclass right inside SwiftUI, and it will even resize the scene if you request it.
To try it out, first add import SpriteKit
to your Swift file. Second, create some sort of game scene you want to render. For example, this will create falling boxes wherever you tap, adding a physics effect so they interact neatly:
// A simple game scene with falling boxes
class GameScene: SKScene {
override func didMove(to view: SKView) {
physicsBody = SKPhysicsBody(edgeLoopFrom: frame)
}
override func touchesBegan(_ touches: Set<UITouch>, with event: UIEvent?) {
guard let touch = touches.first else { return }
let location = touch.location(in: self)
let box = SKSpriteNode(color: .red, size: CGSize(width: 50, height: 50))
box.position = location
box.physicsBody = SKPhysicsBody(rectangleOf: CGSize(width: 50, height: 50))
addChild(box)
}
}
// A sample SwiftUI creating a GameScene and sizing it
// at 300x400 points
struct ContentView: View {
var scene: SKScene {
let scene = GameScene()
scene.size = CGSize(width: 300, height: 400)
scene.scaleMode = .fill
return scene
}
var body: some View {
SpriteView(scene: scene)
.frame(width: 300, height: 400)
.ignoresSafeArea()
}
}
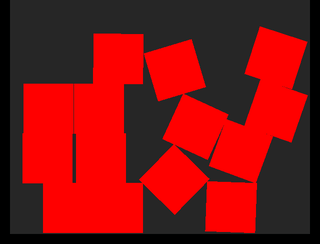
The game scene you create is fully interactive, so it works just like a regular SKView
would do in UIKit.