How to create and compose custom views
How to create and compose custom views 관련
Updated for Xcode 15
One of the core tenets of SwiftUI is composition, which means it’s designed for us to create many small views then combine them together to create something bigger. This allows us to re-use views on a massive scale, which means less work for us. Even better, combining small subviews has almost no runtime overhead, so we can use them freely.
The key is to start small and work your way up. For example, many apps have to work with employees that look something like this:
struct Employee {
var name: String
var jobTitle: String
var emailAddress: String
var profilePicture: String
}
If you want to have a consistent design for employee profile pictures in your app, you might create a 100x100 image view with a circular shape:
struct ProfilePicture: View {
var imageName: String
var body: some View {
Image(imageName)
.resizable()
.frame(width: 100, height: 100)
.clipShape(Circle())
}
}
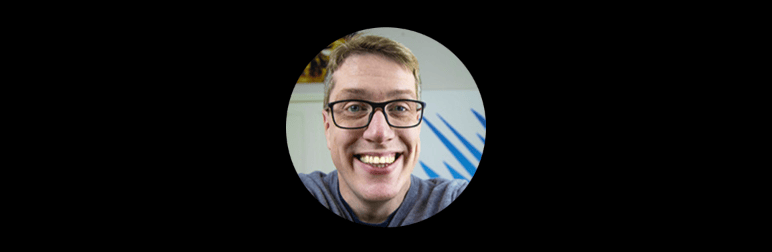
Your designer might tell you that whenever an email address is visible you should show a little envelope icon next to it as a visual hint, so you could make an EmailAddress
view:
struct EmailAddress: View {
var address: String
var body: some View {
HStack {
Image(systemName: "envelope")
Text(address)
}
}
}

When it comes to showing an employee’s details, you could create a view that has their name and job title formatted neatly, backed up by their email address using your EmailAddress
view, like this:
struct EmployeeDetails: View {
var employee: Employee
var body: some View {
VStack(alignment: .leading) {
Text(employee.name)
.font(.largeTitle)
.foregroundStyle(.primary)
Text(employee.jobTitle)
.foregroundStyle(.secondary)
EmailAddress(address: employee.emailAddress)
}
}
}
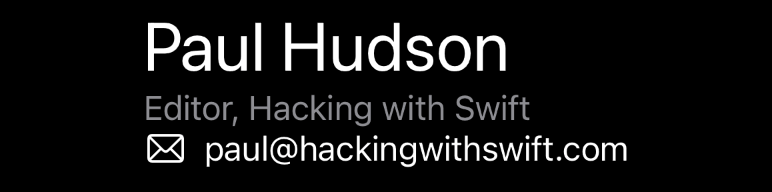
And you could even create a larger view that puts a ProfilePicture
next to a EmployeeDetails
to give a single visual representation of employees, like this:
struct EmployeeView: View {
var employee: Employee
var body: some View {
HStack {
ProfilePicture(imageName: employee.profilePicture)
EmployeeDetails(employee: employee)
}
}
}
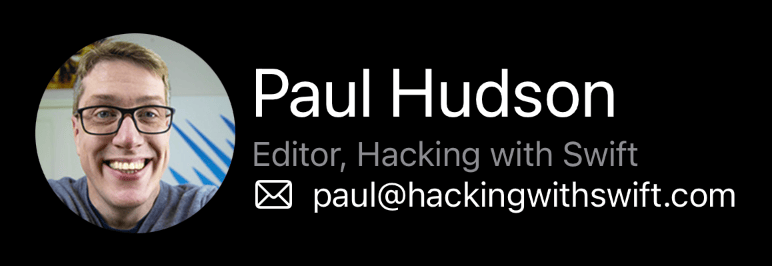
With this structure we now have several ways of showing employees:
- Just their picture
- Just their email address
- Just their job details
- Everything all at once
More importantly, it means that when it comes to using all this work, our main content views don’t have to worry about what employees look like or how they should be treated – all that work is baked into our smaller views. This means we can create a EmployeeView
with an example employee and have it just work.
To demonstrate all this together, here’s one code sample with all the smaller view structs, ending with a ContentView
struct that displays a single employee. To the user the result is the same, but we end up with a whole bunch of small views that do individual things, each of which can be recombined in any number of different ways.
Here’s the code:
struct Employee {
var name: String
var jobTitle: String
var emailAddress: String
var profilePicture: String
}
struct ProfilePicture: View {
var imageName: String
var body: some View {
Image(imageName)
.resizable()
.frame(width: 100, height: 100)
.clipShape(Circle())
}
}
struct EmailAddress: View {
var address: String
var body: some View {
HStack {
Image(systemName: "envelope")
Text(address)
}
}
}
struct EmployeeDetails: View {
var employee: Employee
var body: some View {
VStack(alignment: .leading) {
Text(employee.name)
.font(.largeTitle)
.foregroundStyle(.primary)
Text(employee.jobTitle)
.foregroundStyle(.secondary)
EmailAddress(address: employee.emailAddress)
}
}
}
struct EmployeeView: View {
var employee: Employee
var body: some View {
HStack {
ProfilePicture(imageName: employee.profilePicture)
EmployeeDetails(employee: employee)
}
}
}
struct ContentView: View {
let employee = Employee(name: "Paul Hudson", jobTitle: "Editor, Hacking with Swift", emailAddress: "paul@hackingwithswift.com", profilePicture: "paul-hudson")
var body: some View {
EmployeeView(employee: employee)
}
}