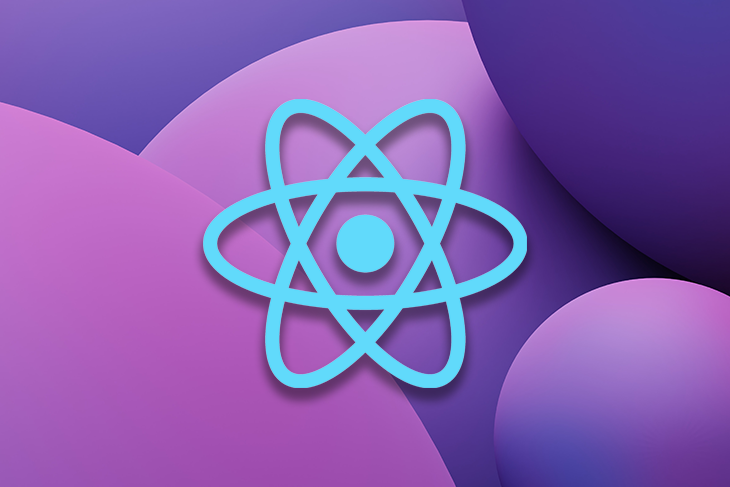
Displaying toast notifications using the `showToast` method
June 21, 2023About 1 min
Displaying toast notifications using the `showToast` method 관련
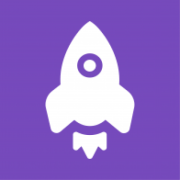
How to create a custom toast component with React
Create a toast component in your React app that is capable of displaying multiple notifications, customizing their position, and deleting them.

How to create a custom toast component with React
Create a toast component in your React app that is capable of displaying multiple notifications, customizing their position, and deleting them.
In the showToast
method, we will create a new object with the showToast
parameter and add it to the toasts using the setToasts
state method. Additionally, we will check for the autoClose
value, which is essentially a checkbox state. If enabled, the toast will be automatically removed after the specified autoCloseDuration
:
const showToast = (message, type) => {
const toast = {
id: Date.now(),
message,
type,
};
setToasts((prevToasts) => [...prevToasts, toast]);
if (autoClose) {
setTimeout(() => {
removeToast(toast.id);
}, autoCloseDuration * 1000);
}
};
Adding trigger buttons
In the JSX, we can use native HTML buttons and pass the showToast
method to their onClick
event handlers in order to trigger a specific type of toast with a designated message. Furthermore, we can include a button that, when clicked, removes all available toasts at once. Here’s an example to illustrate this functionality:
<div className="app-row app-row--group">
<button onClick={() => showToast("A success message", "success")}>
Show Success Toast
</button>
<button onClick={() => showToast("A failure message", "failure")}>
Show Error Toast
</button>
<button onClick={() => showToast("A warning message", "warning")}>
Show Warning Toast
</button>
<button onClick={removeAllToasts}>Clear Toasts</button>
</div>