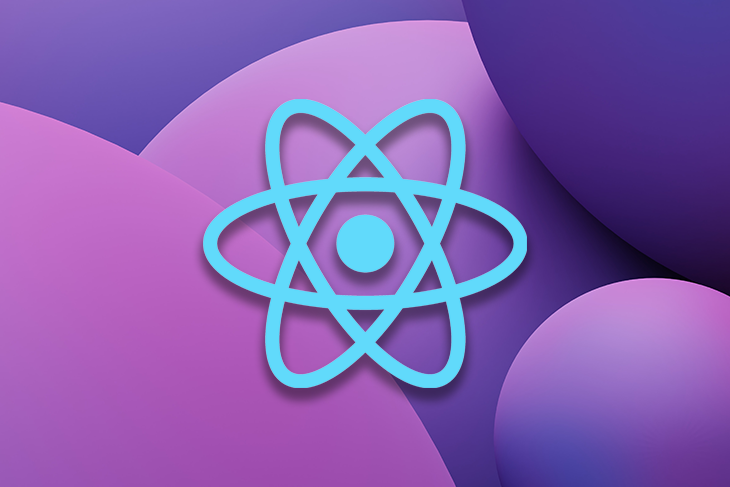
Positioning toast notifications
Positioning toast notifications 관련
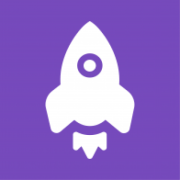

Once again, we can retrieve the position inputs from a select combo box (which we will define shortly) by utilizing the target
event property. Setting the current position requires just a single line of code:
const handlePositionChange = (event) => {
setPosition(event.target.value);
};
Adding the position input
We can define an object to iterate over and populate our select combo box, which is responsible for showing different positions for ToastList
:
const positions = {
"top-right": "Top-right",
"top-left": "Top-left",
"bottom-right": "Bottom-right",
"bottom-left": "Bottom-left",
};

Adding options to the select box is now easy by looping over the positions using the Object.keys
and providing the value as well as the label, as shown below:
<div className="app-row">
<label htmlFor="position">Position</label>
<select
id="position"
value={position}
onChange={handlePositionChange}
>
{Object.keys(positions).map((position) => (
<option key={position} value={position}>
{positions[position]}
</option>
))}
</select>
</div>
The state of the position combo box is handled by the position
state variable, while the change of this input is managed by the handlePositionChange
function, which we discussed in the last few steps.
Adding the ToastList
component
Finally, we conclude our app component file by adding ToastList
at the end. We’ll use the toast and position state variables that we declared in the app component above. Additionally, we’ll pass the removeToast
function as the removeToast
prop of ToastList
before exporting it as a default module:
const App = () => {
...
return (
<div className="app">
<h1 className="app-title">React Toast Component</h1>
...
<ToastList data={toasts} position={position} removeToast={removeToast} />
</div>
);
};
export default App;
Below is a live working demonstration of our final output. You can view it in a different tab using this link. Alternatively, you can also find all of the code on GitHub (c99rahul/react-toast
):