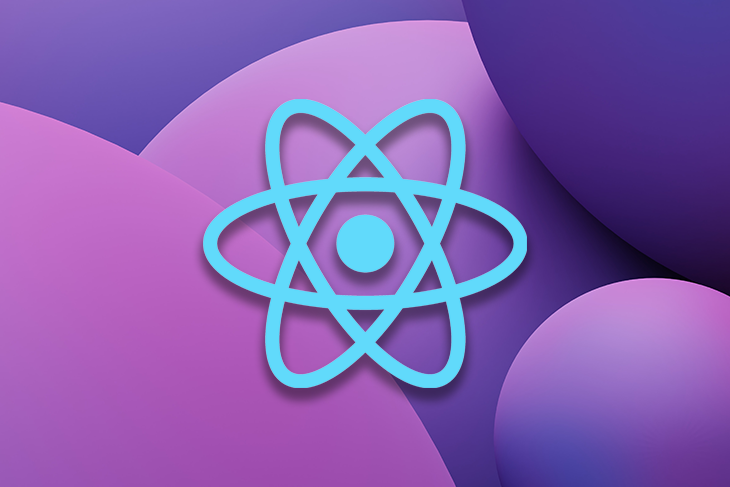
Structuring toast list components
Structuring toast list components 관련
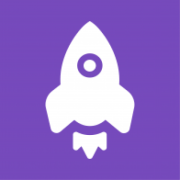

The toast notifications should be flexible enough to be put across all four corners of the viewport. We’ll have four different positions for the toast elements, which will be determined by a container list that will be wrapping up all our toast notifications:
- Top-right
- Bottom-right
- Top-left
- Bottom-left
The positions will be added dynamically, depending on the position props added to the toast list component that we are going to define in this segment.
The basic structure of our toast list will consist of a wrapper element that acts as a list of toast messages. It would look something like the following:
<div class="toast-list toast-list--top-left" aria-live="assertive">
<div class="toast ...">...</div>
<div class="toast ...">...</div>
<div class="toast ...">...</div>
<div class="toast ...">...</div>
</div>
If you notice, we are also using the [aria-live
attribute for our toast list, just to indicate to the screen reader that the list will update with time. Later on, we will use this structure to construct the JSX for the toast list component.
Styling the toast container
Let’s create another folder inside the components
directory named ToastList
. Inside it, create a CSS file named ToastList.css
, which is responsible for styling, structuring, and positioning our ToastList
component, as well as adding appropriate animations to the toast notifications.
First, let’s define some CSS custom properties to centralize the configuration and customization of various settings such as sizing, spacing, and more:
:root {
--toast-speed: 250ms;
--toast-list-scrollbar-width: 0.35em;
--toast-list-width: 400px;
--toast-list-padding: 1em;
}
The toast list needs to remain fixed to the viewport, regardless of the scroll position of the document contents. This is essential to ensure a consistent display of toast notifications to the user. The container should have its contents positioned slightly inward from the edges of the viewport, and its width should be limited on larger screens.
To avoid requiring the user to scroll the document to see the notifications, the toast list should be restricted to a maximum height equal to the viewport height. The list can be scrolled vertically to access the notifications as needed. The following CSS setup for the toast list can help us achieve all of that:
.toast-list {
position: fixed;
padding: var(--toast-list-padding);
width: 100%;
max-width: var(--toast-list-width);
max-height: 100vh;
overflow: hidden auto;
}
Next, we need to define various positional variations for the toast list. These variations can be dynamically added later, depending on the position prop in the React component to ensure its proper functioning:
.toast-list--top-left {
top: 0;
left: 0;
}
.toast-list--top-right {
top: 0;
right: 0;
}
.toast-list--bottom-left {
bottom: 0;
left: 0;
}
.toast-list--bottom-right {
bottom: 0;
right: 0;
}
The next CSS styles are for animations to slide the toast either left or right of the page based on the list’s position. We are going to use the CSS keyframes rule and the animation and transition properties to add the appropriate slide-in and slide-out animations:
@keyframes toast-in-right {
from {
transform: translateX(100%);
}
to {
transform: translateX(0);
}
}
@keyframes toast-in-left {
from {
transform: translateX(-100%);
}
to {
transform: translateX(0);
}
}
.toast-list--top-left .toast,
.toast-list--bottom-left .toast {
animation: toast-in-left var(--toast-speed);
}
.toast-list--top-right .toast,
.toast-list--bottom-right .toast {
animation: toast-in-right var(--toast-speed);
}
.toast-list .toast {
transition: transform var(--toast-speed), opacity var(--toast-speed),
box-shadow var(--toast-speed) ease-in-out;
}
For the additional scrollbar decorations, check out the full ToastList.css
file here (c99rahul/react-toast
).