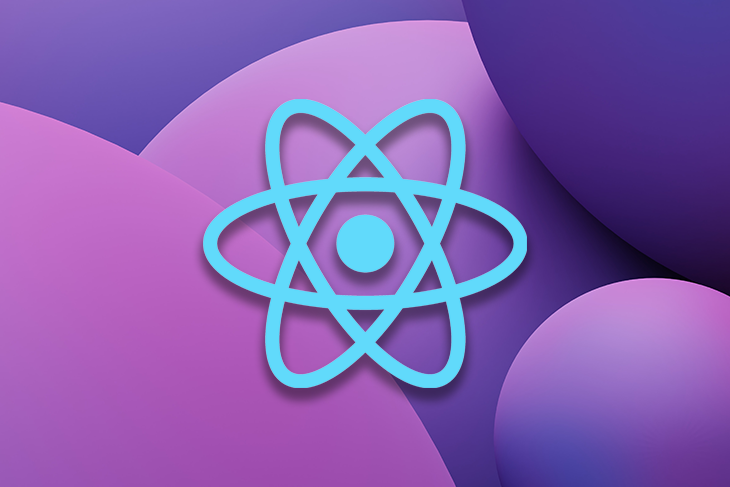
Selecting icons for our toast notifications
Selecting icons for our toast notifications 관련
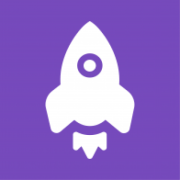

Before delving into the main part of the code, let’s plan the icons we will use for the toast notifications. We need SVG icons that correspond to the different types of notifications, as well as a close button for each toast notification.
To begin, we’ll create a new folder called Icons
within the components
directory. Inside this folder, we’ll create a React component named Icons.css
. This file controls the icon sizing and presentation and looks like this:
.icon {
width: 1em;
height: 1em;
fill: currentColor;
}
.icon--lg {
width: 1.5em;
height: 1.5em;
}
The .icon
class essentially maintains the default size of the SVG icons wrapped inside it at 1em
. Additionally, I’ve included a larger variant of the same class to size the notification icons a bit larger than this default size.
Next, create another file inside the Icons
directory and name it Icons.jsx
. Let’s import React and the above-defined CSS into it first:
import React from "react";
import "./Icons.css";
Now, let’s select some SVG icons from the FontAwesome free icon pack and enclose them within four different components. We will export these components by name.
Feel free to choose the icons you like from your favorite icon sources. Here is an example that demonstrates how the remaining portion of the Icons.jsx
file will appear:
const CloseIcon = () => (
<svg>...</svg>
);
const SuccessIcon = () => (
<svg>...</svg>
);
const FailureIcon = () => (
<svg>...</svg>
);
const WarningIcon = () => (
<svg>...</svg>
);
export { CloseIcon, SuccessIcon, FailureIcon, WarningIcon };
That’s it for the icons. You may view the finished Icons.jsx
file here. (c99rahul/react-toast
) In the next segment, we will learn how to create our toast component, import our icons into it, and use the .icon
class and its variant to size them appropriately.