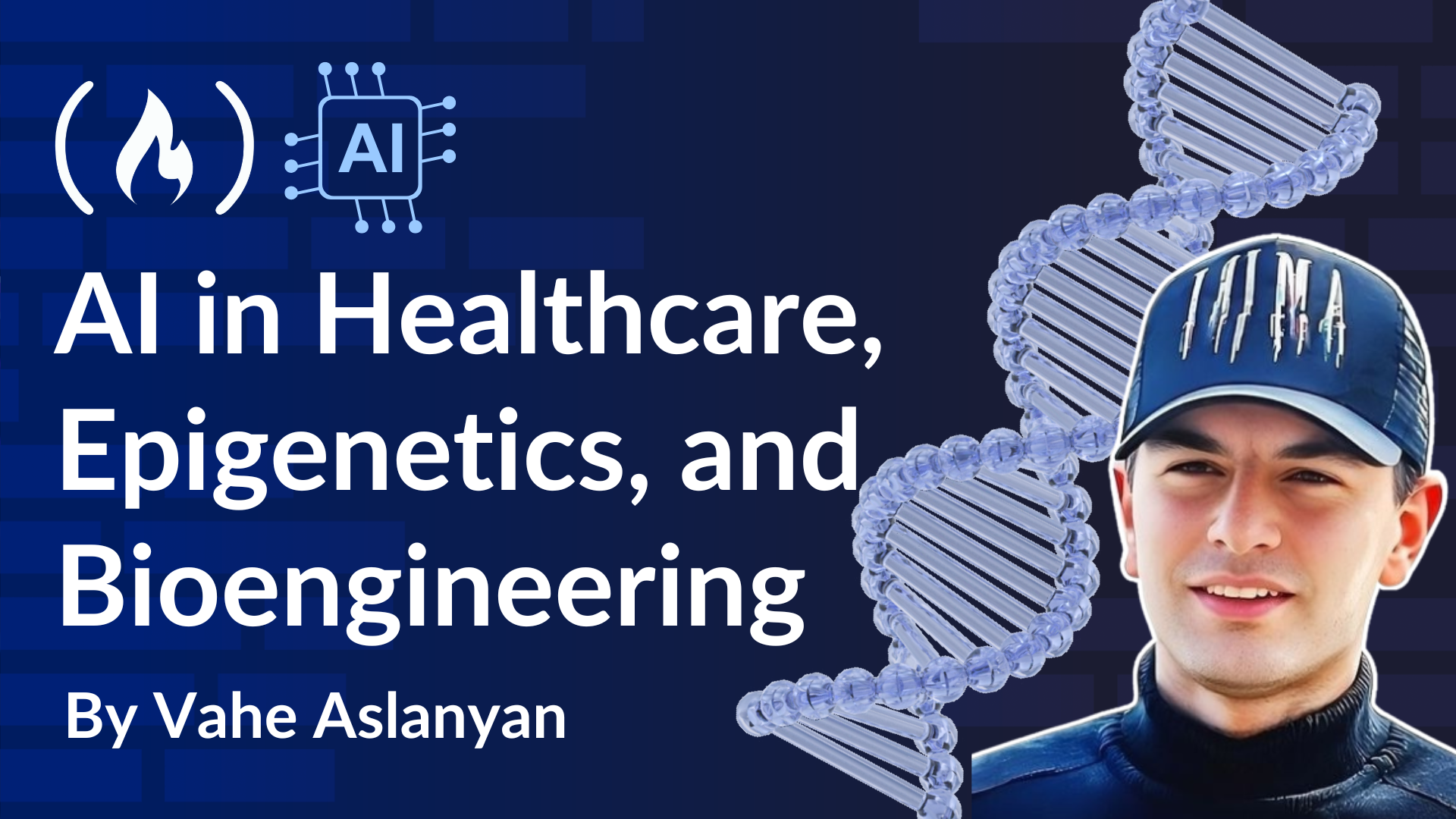
Integration of Bioengineering, Physical Devices, and Language Models
Integration of Bioengineering, Physical Devices, and Language Models 관련
The advent of sophisticated physical devices and the utilization of advanced language models have further propelled bioengineering into new realms of innovation and efficiency.
This chapter delves into the intersection of bioengineering, physical devices, and language models, providing 20 comprehensive code examples that illustrate their practical applications. These examples span data analysis, device control, simulation, and natural language processing, demonstrating how these technologies synergize to address complex challenges in bioengineering.
Data Analysis in Bioengineering
Data analysis is a cornerstone of bioengineering, enabling researchers to interpret vast datasets, identify patterns, and derive meaningful insights. Python, with its extensive libraries, is a preferred language for bioinformatics and data science tasks. Below are several code examples demonstrating data analysis techniques in bioengineering.
Genomic Data Visualization
Visualizing genomic data helps in understanding genetic variations and their implications. The following example uses pandas
and matplotlib
to visualize single nucleotide polymorphisms (SNPs) across different chromosomes.
import pandas as pd
import matplotlib.pyplot as plt
# Load SNP data
snp_data = pd.read_csv('snp_data.csv') # Columns: Chromosome, Position, SNP_Type
# Plot SNP distribution per chromosome
plt.figure(figsize=(12, 6))
for chromosome in snp_data['Chromosome'].unique():
chr_data = snp_data[snp_data['Chromosome'] == chromosome]
plt.scatter(chr_data['Position'], [chromosome]*len(chr_data), label=f'Chr {chromosome}', alpha=0.6)
plt.xlabel('Position')
plt.ylabel('Chromosome')
plt.title('SNP Distribution Across Chromosomes')
plt.legend(bbox_to_anchor=(1.05, 1), loc='upper left')
plt.tight_layout()
plt.show()
This script reads SNP data from a CSV file, filters the data by each chromosome, and plots the SNP positions using scatter plots. Each chromosome is represented on the y-axis, allowing for a clear visualization of SNP distribution across the genome.

Differential Gene Expression Analysis
Identifying differentially expressed genes between conditions is crucial in understanding disease mechanisms. This example uses the statsmodels
library to perform statistical analysis on gene expression data.
import pandas as pd
import statsmodels.api as sm
# Load gene expression data
expression_data = pd.read_csv('gene_expression.csv') # Rows: Genes, Columns: Samples
conditions = pd.read_csv('conditions.csv') # Columns: Sample, Condition
# Prepare design matrix
conditions_encoded = pd.get_dummies(conditions['Condition'], drop_first=True)
X = sm.add_constant(conditions_encoded)
results = {}
# Perform t-test for each gene
for gene in expression_data['Gene']:
y = expression_data[expression_data['Gene'] == gene].iloc[0, 1:]
model = sm.OLS(y, X).fit()
p_value = model.pvalues['Condition_Treated']
results[gene] = p_value
# Convert results to DataFrame
results_df = pd.DataFrame.from_dict(results, orient='index', columns=['p_value'])
significant_genes = results_df[results_df['p_value'] < 0.05]
print(f"Number of significantly differentially expressed genes: {len(significant_genes)}")
print(significant_genes)
This script performs differential gene expression analysis by fitting an Ordinary Least Squares (OLS) model for each gene, comparing treated versus control conditions. Genes with p-values below 0.05 are considered significantly differentially expressed.

Simulation and Modeling in Bioengineering
Simulating biological systems allows for the prediction of behaviors under various conditions without the need for extensive laboratory experiments. Tools like SciPy
and NumPy
are instrumental in building and solving biological models.
Modeling Enzyme Kinetics with Michaelis-Menten Equation
The Michaelis-Menten equation describes the kinetics of enzyme-mediated reactions. This example uses SciPy
to fit experimental data to the equation.
import numpy as np
import matplotlib.pyplot as plt
from scipy.optimize import curve_fit
# Define Michaelis-Menten equation
def michaelis_menten(S, Vmax, Km):
return (Vmax * S) / (Km + S)
# Experimental data: Substrate concentration (S) and reaction rate (V)
S = np.array([0.1, 0.5, 1, 2, 5, 10, 20, 50, 100])
V = np.array([0.05, 0.2, 0.35, 0.55, 0.8, 0.95, 1.1, 1.15, 1.2])
# Fit the model to data
popt, pcov = curve_fit(michaelis_menten, S, V, bounds=(0, np.inf))
Vmax, Km = popt
print(f"Estimated Vmax: {Vmax}")
print(f"Estimated Km: {Km}")
# Plot data and fitted curve
S_fit = np.linspace(0, 100, 500)
V_fit = michaelis_menten(S_fit, Vmax, Km)
plt.scatter(S, V, label='Experimental Data')
plt.plot(S_fit, V_fit, 'r-', label='Fitted Michaelis-Menten')
plt.xlabel('Substrate Concentration (mM)')
plt.ylabel('Reaction Rate (μM/min)')
plt.title('Enzyme Kinetics: Michaelis-Menten Fit')
plt.legend()
plt.show()

This script fits experimental substrate concentration and reaction rate data to the Michaelis-Menten equation using non-linear curve fitting. The estimated parameters Vmax
and Km
provide insights into the enzyme's efficiency and affinity for the substrate.
Population Growth Modeling with Logistic Equation
The logistic growth model describes population growth with a carrying capacity. This example simulates population growth over time using the logistic equation.
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
# Define logistic growth differential equation
def logistic_growth(P, t, r, K):
dPdt = r * P * (1 - P / K)
return dPdt
# Parameters
r = 0.3 # Growth rate
K = 1000 # Carrying capacity
P0 = 10 # Initial population
t = np.linspace(0, 30, 300) # Time
# Solve ODE
P = odeint(logistic_growth, P0, t, args=(r, K))
# Plot results
plt.plot(t, P, label='Population')
plt.axhline(y=K, color='r', linestyle='--', label='Carrying Capacity')
plt.xlabel('Time')
plt.ylabel('Population')
plt.title('Logistic Population Growth')
plt.legend()
plt.show()

This script models population growth using the logistic equation, which incorporates the carrying capacity K
to prevent indefinite growth. The solution is obtained using the odeint
function from SciPy
, and the population dynamics are visualized over time.
Control Systems in Bioengineering Devices
Control systems are vital in bioengineering devices to maintain desired states and respond to external stimuli. Python's control systems library (control
) facilitates the design and analysis of these systems.
Designing a PID Controller for a Temperature Regulation System
A Proportional-Integral-Derivative (PID) controller adjusts system inputs based on the error between desired and actual temperatures. This example designs a PID controller for a simulated temperature system.
import numpy as np
import matplotlib.pyplot as plt
import control as ctrl
# Define system parameters
K = 2.0 # Gain
tau = 5.0 # Time constant
system = ctrl.TransferFunction([K], [tau, 1])
# Define PID controller parameters
Kp = 3.0
Ki = 1.0
Kd = 0.5
controller = ctrl.TransferFunction([Kd, Kp, Ki], [1, 0])
# Closed-loop system
closed_loop = ctrl.feedback(controller*system, 1)
# Step response
t, y = ctrl.step_response(closed_loop)
# Plot response
plt.plot(t, y, label='Closed-loop Response')
plt.xlabel('Time (s)')
plt.ylabel('Temperature')
plt.title('PID Controller for Temperature Regulation')
plt.legend()
plt.grid(True)
plt.show()

This script models a temperature regulation system using a first-order transfer function. A PID controller is designed with specified proportional, integral, and derivative gains. The closed-loop system's step response illustrates the controller's effectiveness in achieving the desired temperature.
Stabilizing a Biological Oscillator
Biological oscillators, such as circadian rhythms, require stabilization to maintain consistent cycles. This example demonstrates stabilizing a simple oscillator using feedback control.
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
# Define oscillator with feedback control
def oscillator_with_feedback(state, t, alpha, beta, gamma):
x, y = state
dxdt = alpha * (y - x)
dydt = x * (gamma - x) - y
return [dxdt, dydt]
# Parameters
alpha = 10.0
beta = 28.0
gamma = 8.0/3.0
# Initial state
state0 = [1.0, 1.0]
# Time points
t = np.linspace(0, 50, 10000)
# Integrate ODE
states = odeint(oscillator_with_feedback, state0, t, args=(alpha, beta, gamma))
# Plot trajectory
plt.plot(states[:,0], states[:,1])
plt.xlabel('X')
plt.ylabel('Y')
plt.title('Stabilized Biological Oscillator')
plt.grid(True)
plt.show()

This script simulates a stabilized biological oscillator using a modified Lorenz system with feedback control parameters. By adjusting alpha
, beta
, and gamma
, the system can achieve stable oscillatory behavior, mimicking biological rhythms.
Machine Learning in Bioengineering
Machine learning techniques are increasingly employed in bioengineering for predictive modeling, classification, and pattern recognition. Libraries like scikit-learn
and TensorFlow
facilitate the implementation of these techniques.
Predicting Protein-Protein Interactions
Predicting protein-protein interactions (PPIs) is essential for understanding cellular functions. This example uses a Support Vector Machine (SVM) to classify potential PPIs based on feature vectors.
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.svm import SVC
from sklearn.metrics import classification_report, confusion_matrix
# Load dataset
data = pd.read_csv('ppi_data.csv') # Columns: Feature1, Feature2, ..., Label
# Features and labels
X = data.drop('Label', axis=1)
y = data['Label']
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Feature scaling
scaler = StandardScaler()
X_train_scaled = scaler.fit_transform(X_train)
X_test_scaled = scaler.transform(X_test)
# Train SVM classifier
svm = SVC(kernel='rbf', C=1.0, gamma='scale')
svm.fit(X_train_scaled, y_train)
# Predict
y_pred = svm.predict(X_test_scaled)
# Evaluation
print(confusion_matrix(y_test, y_pred))
print(classification_report(y_test, y_pred))

This script trains an SVM classifier to predict PPIs using labeled feature data. The dataset is split into training and testing sets, standardized, and then used to train and evaluate the SVM model. The confusion matrix and classification report provide insights into the model's performance.
Deep Learning for Cell Image Classification
Classifying cell images is crucial in medical diagnostics. This example uses a Convolutional Neural Network (CNN) with TensorFlow
and Keras
to classify cell images into different categories.
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense, Dropout
from tensorflow.keras.preprocessing.image import ImageDataGenerator
# Data preparation
train_datagen = ImageDataGenerator(rescale=1./255, horizontal_flip=True, rotation_range=20)
test_datagen = ImageDataGenerator(rescale=1./255)
train_generator = train_datagen.flow_from_directory(
'cell_images/train',
target_size=(64, 64),
batch_size=32,
class_mode='binary')
validation_generator = test_datagen.flow_from_directory(
'cell_images/validation',
target_size=(64, 64),
batch_size=32,
class_mode='binary')
# Build CNN model
model = Sequential([
Conv2D(32, (3,3), activation='relu', input_shape=(64, 64, 3)),
MaxPooling2D(pool_size=(2,2)),
Conv2D(64, (3,3), activation='relu'),
MaxPooling2D(pool_size=(2,2)),
Flatten(),
Dense(128, activation='relu'),
Dropout(0.5),
Dense(1, activation='sigmoid')
])
# Compile model
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# Train model
history = model.fit(
train_generator,
steps_per_epoch=100,
epochs=20,
validation_data=validation_generator,
validation_steps=50)
# Evaluate model
loss, accuracy = model.evaluate(validation_generator)
print(f'Validation Accuracy: {accuracy*100:.2f}%')

This script constructs a CNN for binary classification of cell images. The model includes convolutional layers for feature extraction, pooling layers for dimensionality reduction, and dense layers for classification. Data augmentation is applied to the training data to improve model generalization.
Natural Language Processing in Bioengineering
Natural Language Processing (NLP) facilitates the extraction of information from scientific literature and the automation of documentation tasks. Libraries like nltk
, spaCy
, and transformers
are commonly used in bioengineering applications.
Extracting Gene Names from Scientific Articles
Extracting gene names from text is essential for knowledge extraction and database population. This example uses spaCy
for Named Entity Recognition (NER) to identify gene names in scientific abstracts.
import spacy
# Load pre-trained spaCy model
nlp = spacy.load('en_core_web_sm')
# Sample abstract
abstract = """
The interaction between BRCA1 and RAD51 is critical for the repair of double-strand breaks in DNA.
Mutations in BRCA1 are associated with an increased risk of breast and ovarian cancers.
"""
# Process text
doc = nlp(abstract)
# Extract gene names (assuming they are tagged as ORG for this example)
gene_names = [ent.text for ent in doc.ents if ent.label_ == 'ORG']
print("Extracted Gene Names:", gene_names)

This script processes a scientific abstract to extract gene names using spaCy's NER capabilities. While spaCy's pre-trained models may not be optimized for gene names, this example demonstrates the approach. For more accurate gene name extraction, custom models or domain-specific libraries like BioBERT
can be employed.
Summarizing Biomedical Literature with Transformer Models
Summarizing biomedical literature helps researchers quickly grasp the essence of extensive research. This example uses the transformers
library to generate summaries of biomedical abstracts.
from transformers import pipeline
# Initialize summarization pipeline
summarizer = pipeline("summarization", model="facebook/bart-large-cnn")
# Sample abstract
abstract = """
The CRISPR-Cas9 system has revolutionized genome editing by allowing precise modifications to DNA sequences.
Its applications range from basic research to therapeutic interventions for genetic disorders.
Despite its potential, challenges such as off-target effects and delivery mechanisms remain.
Ongoing research focuses on improving specificity and developing efficient delivery vectors.
"""
# Generate summary
summary = summarizer(abstract, max_length=50, min_length=25, do_sample=False)
print("Summary:", summary[0]['summary_text'])

This script utilizes a pre-trained BART model to summarize a biomedical abstract. The pipeline
abstraction simplifies the process, allowing for easy integration of powerful transformer models in bioengineering workflows.
Security and Privacy in Bioengineering Data Handling
Handling sensitive bioengineering data necessitates robust security and privacy measures. This section provides code examples demonstrating encryption and secure data storage practices.
Encrypting Biomedical Data with Fernet
Encrypting biomedical data ensures that sensitive information remains confidential and protected from unauthorized access. This example uses the cryptography
library's Fernet module for symmetric encryption.
from cryptography.fernet import Fernet
import pandas as pd
# Generate encryption key
key = Fernet.generate_key()
cipher_suite = Fernet(key)
print(f"Encryption Key: {key.decode()}") # Store securely
# Load biomedical data
data = pd.read_csv('biomedical_data.csv')
# Convert DataFrame to bytes
data_bytes = data.to_csv(index=False).encode()
# Encrypt data
encrypted_data = cipher_suite.encrypt(data_bytes)
# Save encrypted data to file
with open('biomedical_data_encrypted.bin', 'wb') as file:
file.write(encrypted_data)
print("Data encrypted and saved successfully.")

This script generates a symmetric encryption key, encrypts a biomedical dataset, and saves the encrypted data to a binary file. Proper key management is crucial—the encryption key must be stored securely to allow data decryption when needed.
Secure Data Transmission with SSL/TLS
Transmitting biomedical data securely over networks is essential to prevent data breaches. This example demonstrates setting up a secure server-client communication using SSL/TLS in Python.
import socket
import ssl
# Create socket
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(('localhost', 8443))
server_socket.listen(5)
print("Server listening on port 8443...")
# Wrap socket with SSL
context = ssl.create_default_context(ssl.Purpose.CLIENT_AUTH)
context.load_cert_chain(certfile='server.crt', keyfile='server.key')
while True:
client_socket, addr = server_socket.accept()
conn = context.wrap_socket(client_socket, server_side=True)
print(f"Connection from {addr}")
data = conn.recv(1024)
if data:
print(f"Received: {data.decode()}")
conn.sendall(b"Data received securely.")
conn.close()

import socket
import ssl
# Create socket
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# Wrap socket with SSL
context = ssl.create_default_context()
context.check_hostname = False
context.verify_mode = ssl.CERT_NONE # For testing purposes only
conn = context.wrap_socket(client_socket, server_hostname='localhost')
# Connect to server
conn.connect(('localhost', 8443))
print("Connected to server.")
# Send data
message = "Sensitive biomedical data."
conn.sendall(message.encode())
# Receive response
response = conn.recv(1024)
print(f"Server Response: {response.decode()}")
conn.close()

This setup establishes a secure server-client communication channel using SSL/TLS. The server listens for incoming connections, wraps the socket with SSL using a certificate and key, and securely receives data from clients. The client connects to the server using an SSL context, sends sensitive data, and receives confirmation.
Note
In production, proper certificate verification should be enforced to ensure security.
Leveraging Cloud Computing for Bioengineering Applications
Cloud computing provides scalable resources for bioengineering applications, facilitating large-scale data processing and storage. This section includes examples of using cloud services for bioengineering tasks.
Uploading and Processing Data on AWS S3 with Boto3
Amazon Web Services (AWS) S3 offers scalable storage for bioengineering data. This example demonstrates how to upload a file to S3 and process it using AWS Lambda.
import boto3
from botocore.exceptions import NoCredentialsError
# Initialize S3 client
s3 = boto3.client('s3')
def upload_to_s3(file_name, bucket, object_name=None):
if object_name is None:
object_name = file_name
try:
s3.upload_file(file_name, bucket, object_name)
print(f"Uploaded {file_name} to {bucket}/{object_name}")
except FileNotFoundError:
print("The file was not found")
except NoCredentialsError:
print("Credentials not available")
# Upload file
upload_to_s3('biomedical_data.csv', 'my-bioengineering-bucket', 'data/biomedical_data.csv')
# Lambda function code (to be deployed on AWS Lambda)
"""
import json
import boto3
import pandas as pd
def lambda_handler(event, context):
s3 = boto3.client('s3')
bucket = event['Records'][0]['s3']['bucket']['name']
key = event['Records'][0]['s3']['object']['key']
# Download the file from S3
s3.download_file(bucket, key, '/tmp/biomedical_data.csv')
# Process data (example: compute mean of a column)
data = pd.read_csv('/tmp/biomedical_data.csv')
mean_values = data.mean().to_dict()
# Log results
print("Mean Values:", mean_values)
return {
'statusCode': 200,
'body': json.dumps('Data processed successfully!')
}
"""

This script uploads a biomedical data file to an AWS S3 bucket using the boto3
library. The accompanying AWS Lambda function (commented out) is triggered upon file upload, downloads the file, processes it (for example, computing mean values), and logs the results. This integration automates data processing workflows, leveraging cloud scalability and serverless computing.
Deploying a Bioinformatics Web Application on Heroku
Deploying bioinformatics tools as web applications enables broader accessibility and collaboration. This example outlines deploying a simple Flask-based bioinformatics tool on Heroku.
from flask import Flask, request, jsonify
import pandas as pd
import io
app = Flask(__name__)
@app.route('/upload', methods=['POST'])
def upload_file():
if 'file' not in request.files:
return jsonify({'error': 'No file provided'}), 400
file = request.files['file']
if file.filename == '':
return jsonify({'error': 'No selected file'}), 400
if file:
stream = io.StringIO(file.stream.read().decode("UTF8"), newline=None)
df = pd.read_csv(stream)
summary = df.describe().to_dict()
return jsonify({'summary': summary}), 200
if __name__ == '__main__':
app.run(debug=True)

Flask
pandas
gunicorn
web: gunicorn app:app
Deployment Steps:
Initialize a Git repository and commit the code.
Create a Heroku app:
heroku create bioinformatics-app
Deploy to Heroku:
git push heroku master
Access the deployed app via the provided Heroku URL.
This Flask application provides an endpoint to upload a CSV file containing bioinformatics data. Upon receiving the file, it processes the data using pandas
, generates statistical summaries, and returns the results as a JSON response. Deploying this application on Heroku makes it accessible to researchers worldwide, facilitating data analysis and collaboration.
Ethical Considerations in Bioengineering Technology Integration
Integrating advanced technologies in bioengineering raises ethical considerations related to privacy, consent, and the potential for misuse. This section emphasizes the importance of ethical practices and provides guidelines for responsible technology integration.
Ensuring Data Privacy and Security
Protecting sensitive biomedical data is paramount. Implementing robust encryption, access controls, and compliance with regulations like HIPAA ensures data privacy and security.
Example
Implementing role-based access control (RBAC) in a Flask application
from flask import Flask, request, jsonify
from functools import wraps
app = Flask(__name__)
# Simple user roles
users = {
'admin': {'password': 'adminpass', 'role': 'admin'},
'user1': {'password': 'user1pass', 'role': 'user'}
}
def check_auth(username, password):
if username in users and users[username]['password'] == password:
return users[username]['role']
return None
def requires_role(role):
def decorator(f):
@wraps(f)
def decorated(*args, **kwargs):
auth = request.authorization
if not auth or not check_auth(auth.username, auth.password):
return jsonify({'message': 'Authentication required'}), 401
user_role = check_auth(auth.username, auth.password)
if user_role != role and user_role != 'admin':
return jsonify({'message': 'Permission denied'}), 403
return f(*args, **kwargs)
return decorated
return decorator
@app.route('/admin/data', methods=['GET'])
@requires_role('admin')
def admin_data():
# Return sensitive data
return jsonify({'data': 'Sensitive admin data'}), 200
@app.route('/user/data', methods=['GET'])
@requires_role('user')
def user_data():
# Return user-specific data
return jsonify({'data': 'User-specific data'}), 200
if __name__ == '__main__':
app.run(debug=True)

This Flask application implements basic role-based access control (RBAC) to restrict access to sensitive data based on user roles. Only users with the 'admin' role can access admin-specific data, while regular users can access their own data.
Enhancing such mechanisms with more sophisticated authentication and authorization frameworks ensures robust data privacy and security.
Addressing Bias in Machine Learning Models
Machine learning models can inadvertently perpetuate biases present in training data. Ensuring diverse and representative datasets and implementing bias detection mechanisms are essential for fair and ethical AI applications in bioengineering.
Example
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import classification_report
import pandas as pd
# Load dataset with demographic information
data = pd.read_csv('patient_data.csv') # Includes features and 'Outcome'
# Check for bias
print(data['Demographic'].value_counts())
# Ensure balanced dataset
X = data.drop(['Outcome', 'Demographic'], axis=1)
y = data['Outcome']
demographics = data['Demographic']
X_train, X_test, y_train, y_test, dem_train, dem_test = train_test_split(
X, y, demographics, test_size=0.2, stratify=demographics, random_state=42)
# Train model
clf = RandomForestClassifier(n_estimators=100, random_state=42)
clf.fit(X_train, y_train)
# Predict
y_pred = clf.predict(X_test)
# Evaluate
print(classification_report(y_test, y_pred))
# Analyze performance across demographics
for group in dem_test.unique():
idx = dem_test == group
print(f"Performance for {group}:")
print(classification_report(y_test[idx], y_pred[idx]))

This script evaluates the performance of a Random Forest classifier across different demographic groups to detect potential biases. By stratifying the dataset and analyzing classification metrics for each group, bioengineers can identify and mitigate biases, ensuring equitable AI-driven decisions in healthcare applications.
Future Directions in Bioengineering Technology Integration
The integration of bioengineering with physical devices and language models is poised to drive significant advancements in healthcare, biotechnology, and environmental sustainability. Future directions include the development of intelligent biomedical devices, enhanced data interpretation systems, and collaborative platforms that leverage the strengths of each technology.
Intelligent Wearables for Personalized Health Monitoring
Wearable devices embedded with intelligent algorithms and language models can provide personalized health insights and proactive healthcare management. Future developments may include continuous monitoring of vital signs, real-time health analytics, and seamless communication with healthcare providers through natural language interfaces.
Example
Integrating wearable sensor data with a chatbot for health advice
import speech_recognition as sr
from transformers import pipeline
# Initialize speech recognizer and summarization pipeline
recognizer = sr.Recognizer()
command_processor = pipeline("text2text-generation", model="t5-small")
def listen_for_commands():
with sr.Microphone() as source:
print("Listening for commands...")
audio = recognizer.listen(source)
try:
command = recognizer.recognize_google(audio)
print(f"Recognized Command: {command}")
return command
except sr.UnknownValueError:
print("Could not understand audio")
return ""
except sr.RequestError as e:
print(f"Could not request results; {e}")
return ""
def process_command(command):
if "open hand" in command.lower():
action = "Opening hand"
elif "close hand" in command.lower():
action = "Closing hand"
else:
action = "Command not recognized"
return action
def main():
while True:
command = listen_for_commands()
if command:
action = process_command(command)
print(action)
# Here, integrate with prosthetic control system
# e.g., send_signal_to_prosthetic(action)
if __name__ == "__main__":
main()

This script captures verbal commands using a microphone, processes them with a speech recognizer, and interprets the commands using simple keyword matching. In a real-world scenario, the process_command
function could be enhanced with a language model to better understand and execute complex instructions, directly interfacing with the prosthetic's control system to perform actions like opening or closing the hand.
Collaborative Platforms for Bioengineering Research
Collaborative platforms that integrate bioengineering data, physical devices, and language models can facilitate interdisciplinary research and innovation. These platforms can support data sharing, joint analysis, and automated reporting, fostering a more integrated and efficient research environment.
Example
Building a collaborative dashboard with Streamlit
import streamlit as st
import pandas as pd
from transformers import pipeline
# Initialize summarization pipeline
summarizer = pipeline("summarization", model="facebook/bart-large-cnn")
st.title("Bioengineering Collaborative Dashboard")
# File upload
uploaded_file = st.file_uploader("Upload Bioengineering Data (CSV)", type="csv")
if uploaded_file:
data = pd.read_csv(uploaded_file)
st.write("Data Preview:")
st.dataframe(data.head())
# Data summary
if st.button("Generate Summary"):
summary = data.describe().to_string()
st.write("Data Summary:")
st.text(summary)
# Summarize summary using language model
lm_summary = summarizer(summary, max_length=100, min_length=50, do_sample=False)[0]['summary_text']
st.write("Automated Summary:")
st.write(lm_summary)

This Streamlit application allows researchers to upload bioengineering datasets, view data previews, generate statistical summaries, and obtain automated summaries using a language model. Such collaborative dashboards enhance data accessibility and streamline research workflows.
The integration of bioengineering with physical devices and language models represents a transformative frontier in scientific and medical advancements. By harnessing the capabilities of data analysis, simulation, machine learning, and natural language processing, bioengineers can develop intelligent systems that enhance healthcare outcomes, drive biotechnological innovations, and address environmental challenges.
Ethical considerations, security measures, and equitable access remain paramount in ensuring that these technologies are deployed responsibly and beneficially. As we move forward, the collaborative efforts of bioengineers, data scientists, and AI specialists will be crucial in shaping a future where technology and biology synergize to create a healthier and more sustainable world.