How to make a ScrollView snap with paging or between child views
How to make a ScrollView snap with paging or between child views 관련
Updated for Xcode 15
New in iOS 17
SwiftUI's ScrollView
moves smoothly by default, but with the scrollTargetLayout()
and scrollTargetBehavior()
modifiers we can make it automatically snap to either to specific child views or to whole pages.
As an example, this places 10 rounded rectangles in a horizontal scroll view, with each one being a scroll target. Because .scrollTargetBehavior()
is set to .viewAligned
, SwiftUI will automatically snap between each of the rounded rectangles.
struct ContentView: View {
var body: some View {
ScrollView(.horizontal) {
LazyHStack {
ForEach(0..<10) { i in
RoundedRectangle(cornerRadius: 25)
.fill(Color(hue: Double(i) / 10, saturation: 1, brightness: 1).gradient)
.frame(width: 300, height: 100)
}
}
.scrollTargetLayout()
}
.scrollTargetBehavior(.viewAligned)
.safeAreaPadding(.horizontal, 40)
}
}
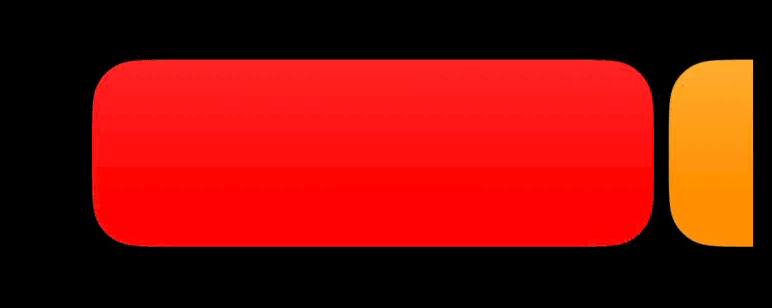
That uses scrollTargetLayout()
to make everything inside the layout be a scroll target. If you only want some child views to be snapped to, you should remove that and instead attach scrollTarget()
to individual views.
An alternative scroll targeting behavior is .paging
, which makes the ScrollView
move by exactly one screen width or height depending on your scroll direction:
ScrollView {
ForEach(0..<50) { i in
Text("Item \(i)")
.font(.largeTitle)
.frame(maxWidth: .infinity)
.frame(height: 200)
.background(.blue)
.foregroundStyle(.white)
.clipShape(.rect(cornerRadius: 20))
}
}
.scrollTargetBehavior(.paging)
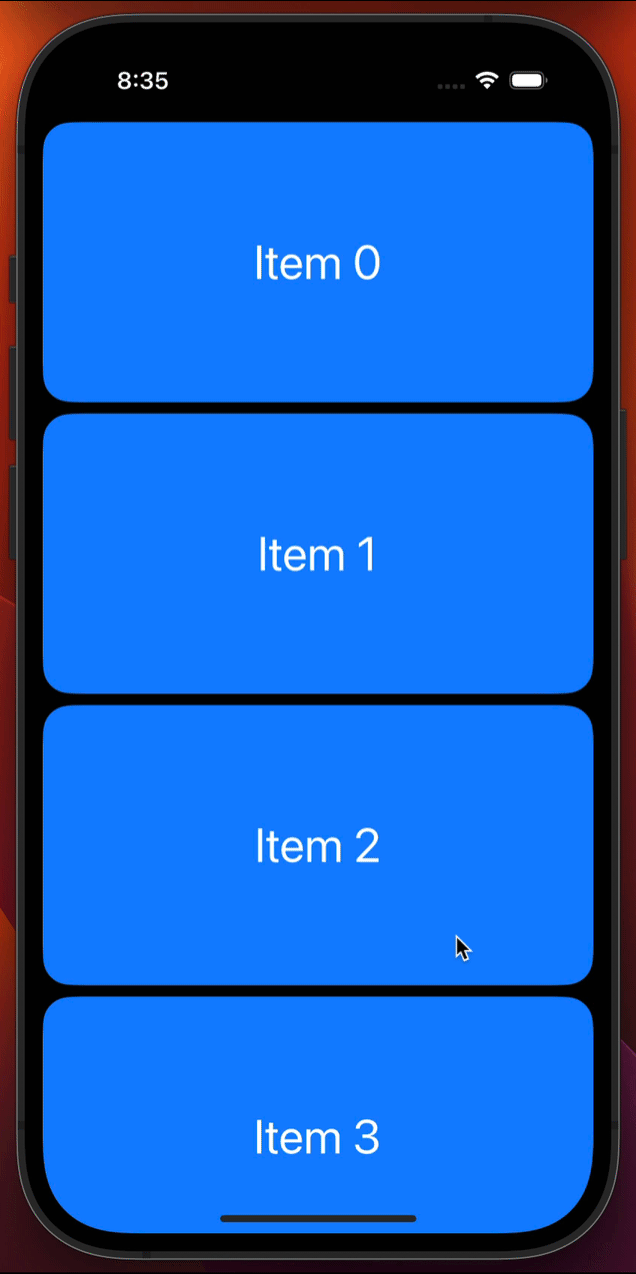