How to make two views the same width or height
How to make two views the same width or height êŽë š
Updated for Xcode 15
SwiftUI makes it easy to create two views that are the same size, regardless of whether you want the same height or the same width, by combining a frame()
modifier with fixedSize()
â there's no need for a GeometryReader
or similar.
On iOS, the key is to give each view you want to size an infinite maximum height or width, which will automatically make it stretch to fill all the available space. You then apply fixedSize()
to the container they are in, which tells SwiftUI those views should only take up the space they need. The result is that SwiftUI figures out the least amount of space the views need, then allows them to take up that full amount â the two views will always match their sizes no matter what they contain.
Here's an example showing how to make two text views have the same height even though they have very different text lengths. Thanks to the frame()
and fixedSize()
combination both text views are laid out at the same size:
HStack {
Text("This is a short string.")
.padding()
.frame(maxHeight: .infinity)
.background(.red)
Text("This is a very long string with lots and lots of text that will definitely run across multiple lines because it's just so long.")
.padding()
.frame(maxHeight: .infinity)
.background(.green)
}
.fixedSize(horizontal: false, vertical: true)
.frame(maxHeight: 200)

This approach works just as well when you want to make two views have the same width:
VStack {
Button("Log in") { }
.foregroundStyle(.white)
.padding()
.frame(maxWidth: .infinity)
.background(.red)
.clipShape(Capsule())
Button("Reset Password") { }
.foregroundStyle(.white)
.padding()
.frame(maxWidth: .infinity)
.background(.red)
.clipShape(Capsule())
}
.fixedSize(horizontal: true, vertical: false)
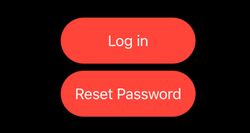
There are many other significantly more complex solutions to this same problem, which is quite strange given how well the simple solution works for most people. I first learned this solution from Becky Hansmeyer and now I use nothing else!