How to style text views with fonts, colors, line spacing, and more
How to style text views with fonts, colors, line spacing, and more êŽë š
Updated for Xcode 15
Not only do text views give us a predictably wide range of control in terms of how they look, they are also designed to work seamlessly alongside core Apple technologies such as Dynamic Type.
By default a Text view has a âBodyâ Dynamic Type style, but you can select from other sizes and weights by calling .font()
on it like this:
Text("This is an extremely long text string that will never fit even the widest of phones without wrapping")
.font(.largeTitle)
.frame(width: 300)
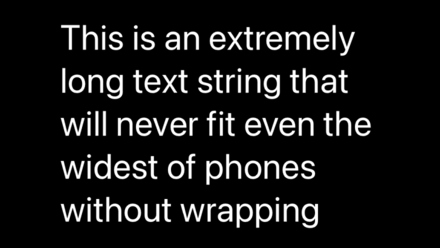
We can control the color of text using the .foregroundStyle()
modifier, like this:
Text("The best laid plans")
.foregroundStyle(.red)

For more complex text coloring, e.g. using a gradient, you should use foregroundStyle()
like this:
Text("The best laid plans")
.foregroundStyle(.blue.gradient)
You can also set the background color, but that uses .background()
because it's possible to use more advanced backgrounds than just a flat color. Anyway, to give our layout a yellow background color we would add this:
Text("The best laid plans")
.padding()
.background(.yellow)
.foregroundStyle(.white)
.font(.headline)
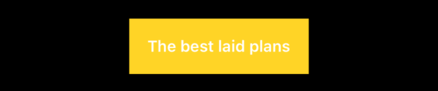
There are even more options. For example, we can adjust the line spacing in our text. The default value is 0, which means there is no extra line spacing applied, but you can also specify position values to add extra spacing between lines:
Text("This is an extremely long text string that will never fit even the widest of phones without wrapping")
.font(.largeTitle)
.lineSpacing(50)
.frame(width: 300)
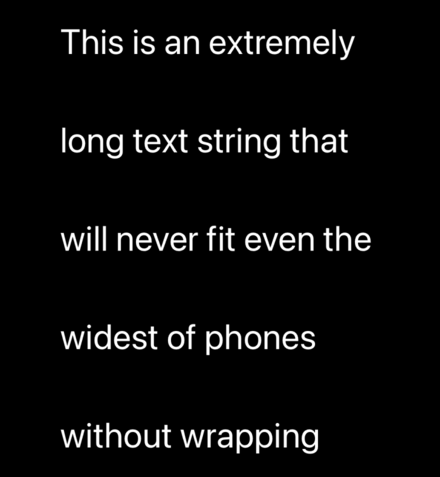
You can also use the fontDesign()
modifier to adjust only the style of the font without also adjusting its size, like this:
Text("Hello, world!")
.fontDesign(.serif)
Or use the fontWidth()
modifier to compress or expand the font, like this:
Text("Hello, world!")
.fontWidth(.condensed)
Note
You will find that fontWidth()
only works with fonts that have been designed to support it. If your font hasn't been designed with font width in mind, you'll just get the default width.