How to draw polygons and stars
About 3 min
How to draw polygons and stars 관련
SwiftUI by Example
Back to Home
How to draw polygons and stars | SwiftUI by Example
How to draw polygons and stars
Updated for Xcode 15
Once you understand SwiftUI’s basic path drawing system, you can add all sorts of shapes easily. For example, we could create a Star
shape that is capable of representing a variety of star shapes, or even other polygons, with just a little mathematics.
Here’s that in code:
struct Star: Shape {
// store how many corners the star has, and how smooth/pointed it is
let corners: Int
let smoothness: Double
func path(in rect: CGRect) -> Path {
// ensure we have at least two corners, otherwise send back an empty path
guard corners >= 2 else { return Path() }
// draw from the center of our rectangle
let center = CGPoint(x: rect.width / 2, y: rect.height / 2)
// start from directly upwards (as opposed to down or to the right)
var currentAngle = -CGFloat.pi / 2
// calculate how much we need to move with each star corner
let angleAdjustment = .pi * 2 / Double(corners * 2)
// figure out how much we need to move X/Y for the inner points of the star
let innerX = center.x * smoothness
let innerY = center.y * smoothness
// we're ready to start with our path now
var path = Path()
// move to our initial position
path.move(to: CGPoint(x: center.x * cos(currentAngle), y: center.y * sin(currentAngle)))
// track the lowest point we draw to, so we can center later
var bottomEdge: Double = 0
// loop over all our points/inner points
for corner in 0..<corners * 2 {
// figure out the location of this point
let sinAngle = sin(currentAngle)
let cosAngle = cos(currentAngle)
let bottom: Double
// if we're a multiple of 2 we are drawing the outer edge of the star
if corner.isMultiple(of: 2) {
// store this Y position
bottom = center.y * sinAngle
// …and add a line to there
path.addLine(to: CGPoint(x: center.x * cosAngle, y: bottom))
} else {
// we're not a multiple of 2, which means we're drawing an inner point
// store this Y position
bottom = innerY * sinAngle
// …and add a line to there
path.addLine(to: CGPoint(x: innerX * cosAngle, y: bottom))
}
// if this new bottom point is our lowest, stash it away for later
if bottom > bottomEdge {
bottomEdge = bottom
}
// move on to the next corner
currentAngle += angleAdjustment
}
// figure out how much unused space we have at the bottom of our drawing rectangle
let unusedSpace = (rect.height / 2 - bottomEdge) / 2
// create and apply a transform that moves our path down by that amount, centering the shape vertically
let transform = CGAffineTransform(translationX: center.x, y: center.y + unusedSpace)
return path.applying(transform)
}
}
// Draw our star shape in a view
struct ContentView: View {
var body: some View {
Star(corners: 5, smoothness: 0.45)
.fill(.red)
.frame(width: 200, height: 200)
.background(.green)
}
}
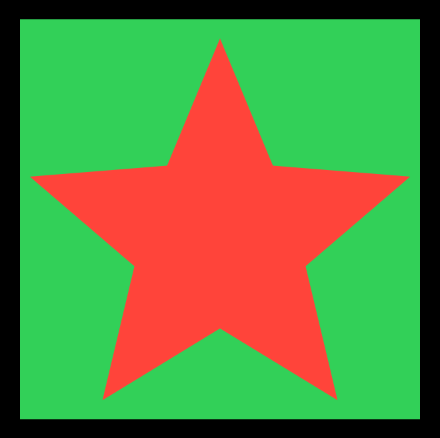
As stars are just polygons, if you increase smoothness to 1 you’ll find you can draw shapes like hexagons and octagons without having to change the code.
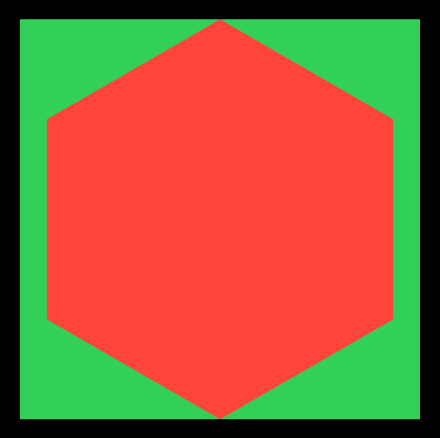
Similar solutions…
All SwiftUI property wrappers explained and compared | SwiftUI by Example
All SwiftUI property wrappers explained and compared
SwiftUI tips and tricks | SwiftUI by Example
SwiftUI tips and tricks
How to use Instruments to profile your SwiftUI code and identify slow layouts | SwiftUI by Example
How to use Instruments to profile your SwiftUI code and identify slow layouts
Building a menu using List | SwiftUI by Example
Building a menu using List
Answering the big question: should you learn SwiftUI, UIKit, or both? | SwiftUI by Example
Answering the big question: should you learn SwiftUI, UIKit, or both?