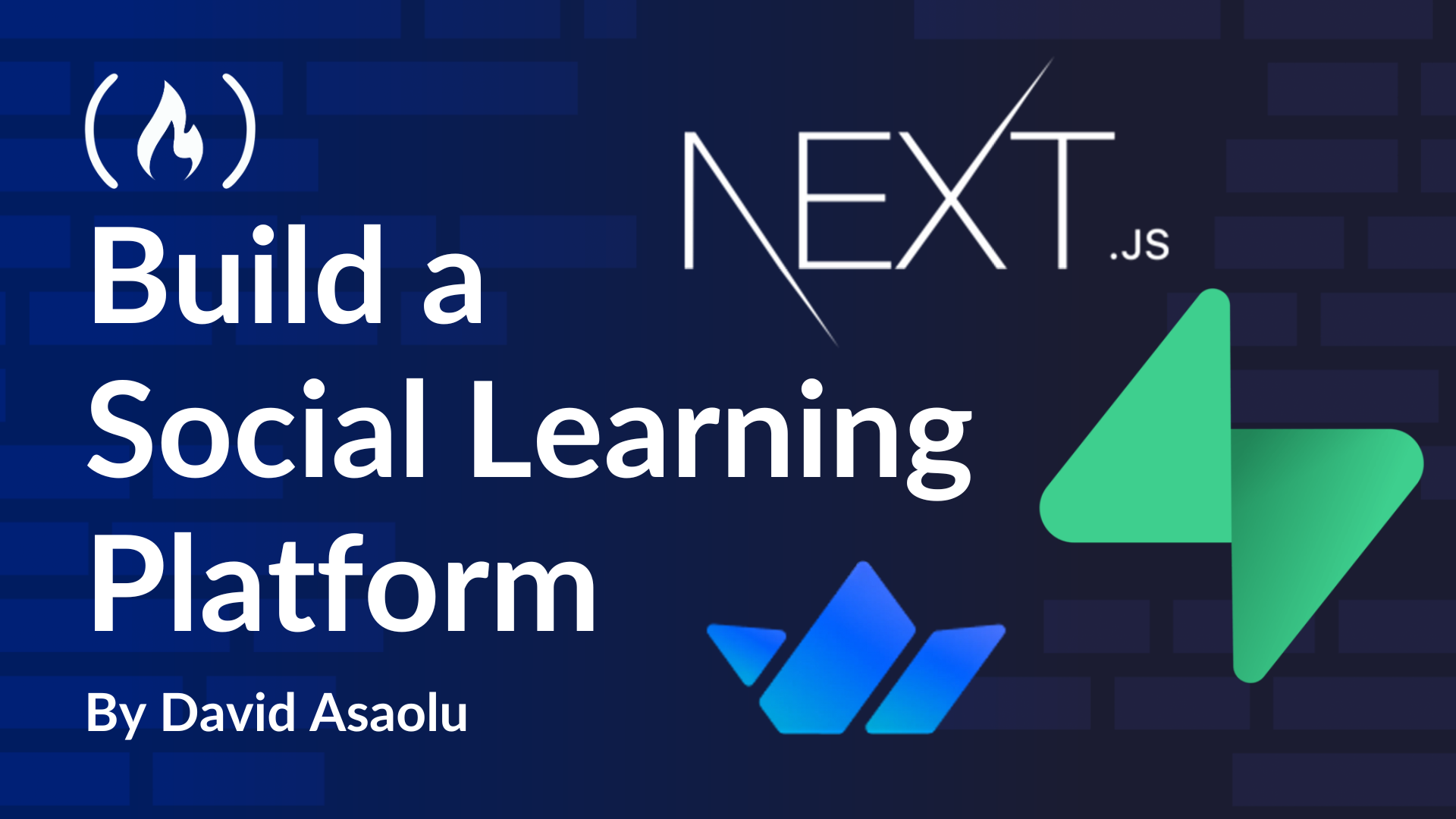
How to Integrate a Group Chat Feature Using Stream Chat Messaging
How to Integrate a Group Chat Feature Using Stream Chat Messaging κ΄λ ¨
In this section, you will learn how to integrate a community chat feature into the application. Each instructor will create a group chat for their followers (students). The chat will allow students to interact with one another and share documents, video links, text, images, and so on using the Stream Chat Messaging SDK.
Setting Up the Stream Chat SDK in Next.js
Add the following code snippet to the stream.action.ts
file:
import { StreamChat } from "stream-chat";
import { getUserSession } from "./auth";
//ππ» creates a StreamChat instance
const serverClient = StreamChat.getInstance(STREAM_API_KEY, STREAM_API_SECRET);
//ππ» creates a token
export async function createToken(): Promise<string> {
const { user } = await getUserSession();
if (!user) throw new Error("User is not authenticated");
return serverClient.createToken(user.id);
}
The code snippet above initializes a Stream Chat instance using its API key and secret key. It also includes a function that generates and returns a token based on the current user's ID.
To ensure that only instructors can create a community channel, follow these steps:
- Retrieve all the channels where the instructor is a member.
- If no channels are found (i.e., the returned array is empty), the instructor can create a new channel.
- An error message is displayed if a channel already exists, informing the instructor that they can only have one community channel.
export async function createChannel({
userId,
data,
}: {
userId: string;
data: { name: string; imageUrl: string };
}) {
try {
//ππ» retrieve channel list
const channels = await serverClient.queryChannels(
{
members: { $in: [userId] },
type: "messaging",
},
{ last_message_at: -1 }
);
//ππ» instructor already has a channel
if (channels.length > 0) {
return {
success: false,
error: "You already have an existing channel",
id: channels[0].id,
};
}
//ππ» declare channel type
const channel = serverClient.channel("messaging", `channel-${userId}`, {
name: data.name,
image: data.imageUrl,
members: [userId],
created_by_id: userId,
});
//ππ» create a channel
await channel.create();
return { success: true, error: null, id: channel.id };
} catch (err) {
return { success: false, error: "Failed to create channel", id: null };
}
}
The code snippet above creates a public channel, meaning anyone can join at any time. Also, the channel name is linked to the instructor's ID, ensuring it remains unique to that instructor.
To retrieve the instructor's channel link, add a function inside the stream.action.ts
file. This function should return the channel URL (channel ID), allowing members to access the channel whenever needed. Then, you can display this link on the instructor's profile for easy access.
export async function getInstructorChannel(userId: string) {
try {
const channels = await serverClient.queryChannels(
{
members: { $in: [userId] },
type: "messaging",
},
{ last_message_at: -1 }
);
return `/chat/${channels[0].id}`;
} catch (err) {
return null;
}
}
Finally, to grant users access to the channel page, check if the user is already a member. If not, add the student as a member before rendering the chat page. This ensures that only authorized users can participate in the conversation.
export async function addUserToChannel(channelId: string, userId: string) {
try {
//ππ» check if student is already a member
const channels = await serverClient.queryChannels(
{
members: { $in: [userId] },
type: "messaging",
id: channelId,
},
{ last_message_at: -1 }
);
//ππ» student already a member (success - show chat page)
if (channels.length > 0) {
return {
success: true,
message: "Already a member",
id: channels[0].id,
error: null,
};
}
//ππ» get channel by ID (student not a member)
const channel = serverClient.channel("messaging", channelId);
//ππ» add student to channel as a member
await channel.addMembers([userId]);
//ππ» student now a member (success - show chat page)
return {
success: true,
error: null,
id: channel.id,
message: "Member just added",
};
} catch (error) {
console.error("Error adding user to channel:", error);
return {
success: false,
error: "Failed to add user to channel",
id: null,
message: null,
};
}
}

Stream Chat UI Components
Inside the (stream)
folder, create a chat/[id]/
page.tsx
file. This page retrieves the channel ID from the page route and checks whether the user is already a channel member. If not, the user is automatically added as a member before displaying the chat interface.
Copy the following code snippet into the chat/[id]/
page.tsx
file:
"use client";
import { useCallback, useEffect, useState } from "react";
import StreamChat from "./../(components)/StreamChat";
import { useParams } from "next/navigation";
import { useRouter } from "next/navigation";
export default function ChatPage() {
const [userData, setUserData] = useState<UserData | null>(null);
const [joinChannel, setJoinChannel] = useState<boolean>(false);
const params = useParams<{ id: string }>();
const router = useRouter();
const fetchUserData = useCallback(async () => {
// ππ» get user object & channel id from useParams
// ππ» execute the addUserToChannel() function declared in the previous section
// ππ» update the joinChannel React state
}, [params.id, router]);
useEffect(() => {
fetchUserData();
}, [fetchUserData]);
if (!userData) {
return null;
}
return (
<>{joinChannel ? <StreamChat user={userData} /> : <ConfirmMember />}</>
);
}
function ConfirmMember() {
return (
<div className='flex flex-col items-center justify-center h-screen'>
<h1 className='text-2xl font-bold mb-4 text-blue-500'>
You are not a member of this channel
</h1>
<p className='text-lg mb-4'>
Please wait while we add you to the channel
</p>
<div className='loader'>
<Loader2 size={48} className='animate-spin' />
</div>
</div>
);
}
This code snippet ensures that a user is either already a member of the channel or is added before displaying the chat interface. The StreamChat
component is a custom React component that contains all the Stream Chat UI elements. The ConfirmMember
component shows a loading message while the user is added to the channel.
Create a StreamChat component and add the following imports to the file:
"use client";
import { useCallback } from "react";
//ππ» -- Stream chat UI components
import {
Chat,
Channel,
ChannelList,
Window,
ChannelHeader,
MessageList,
MessageInput,
useCreateChatClient,
} from "stream-chat-react";
// -- end of Stream chat UI components
//ππ» -- allows members to send emoji within the chat
import { EmojiPicker } from "stream-chat-react/emojis";
import { init, SearchIndex } from "emoji-mart";
import data from "@emoji-mart/data";
init({ data });
// -- end of emoji imports
//ππ» -- create token server action
import { createToken } from "../../../../../actions/stream.action";
Declare the StreamChat component as follows:
export default function StreamChat({ user }: { user: UserData }) {
const tokenProvider = useCallback(async () => {
return await createToken();
}, []);
const filters = { members: { $in: [user.id] }, type: "messaging" };
const options = { presence: true, state: true };
const client = useCreateChatClient({
apiKey: process.env.NEXT_PUBLIC_STREAM_API_KEY!,
tokenOrProvider: tokenProvider,
userData: { id: user.id, name: user.name, image: user.image },
});
if (!client) return <div>Loading...</div>;
return (
// -- Stream Chat UI components --
)
}
The useCreateChatClient
hook creates a Stream chat client using the Stream API key, the user's data, and the token created using the createToken()
function declared earlier in this section.
Finally, return the chat UI from the StreamChat component:
return (
<Chat client={client}>
<div className='chat-container'>
{/* -- Channel List -- */}
<div className='channel-list'>
<ChannelList
sort={{ last_message_at: -1 }}
filters={filters}
options={options}
/>
</div>
{/* -- Messages Panel -- */}
<div className='chat-panel'>
<Channel EmojiPicker={EmojiPicker} emojiSearchIndex={SearchIndex}>
<Window>
<ChannelHeader />
<MessageList />
<MessageInput />
</Window>
</Channel>
</div>
</div>
</Chat>
);
From the code snippet above:
- Chat component initializes the Stream Chat client and wraps the entire Chat page.
- ChannelList shows available chat channels.
- Channel sets up an active chat session.
- Window contains the message display and input areas.
- ChannelHeader, MessageList, and MessageInput provide a fully functional chat interface.

Congratulations! You've completed this tutorial. The source code for this article is also available on GitHub (dha-stix/stream-lms
).