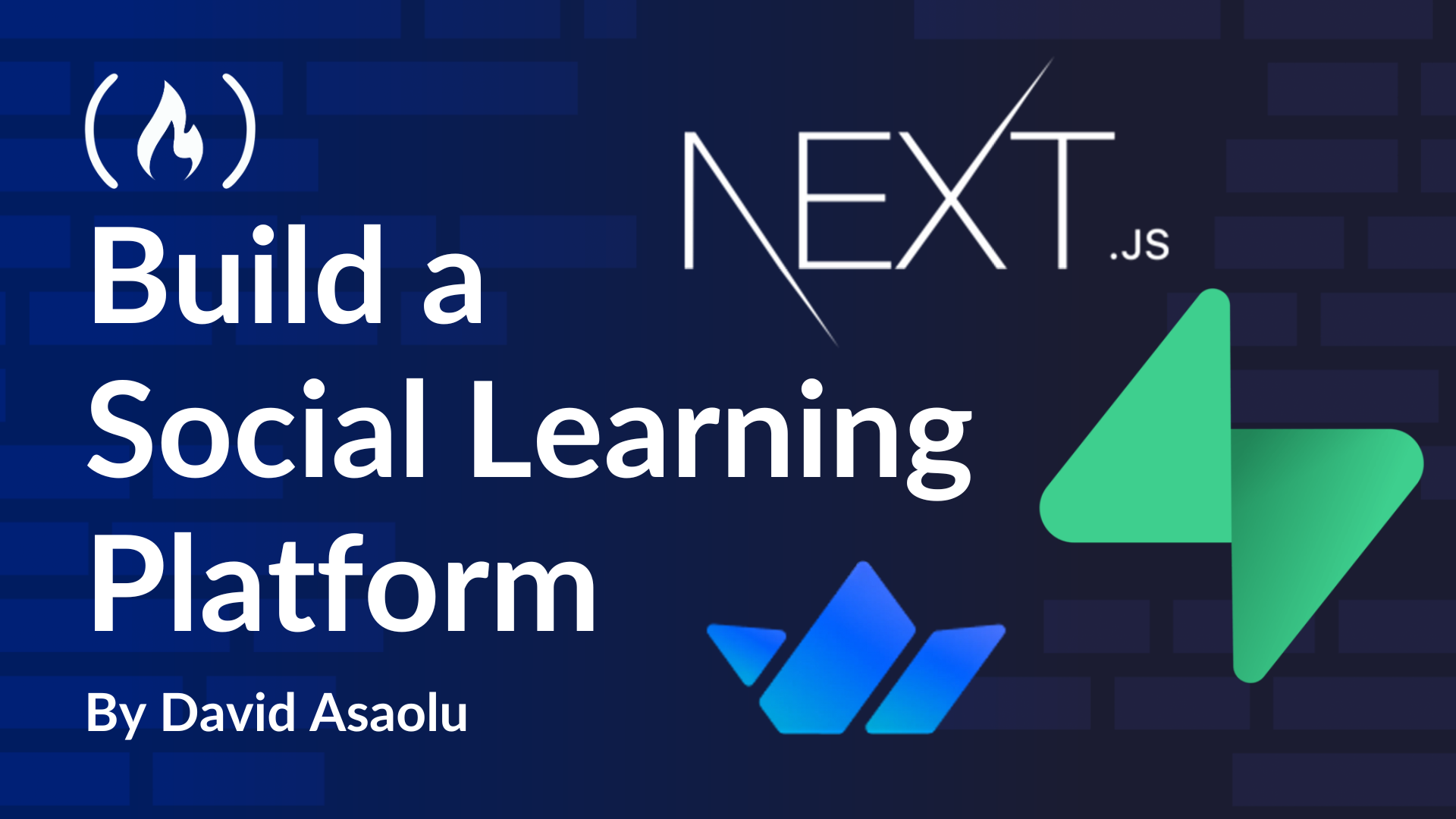
How to Set up Server-Side Authentication with Supabase
How to Set up Server-Side Authentication with Supabase κ΄λ ¨
Here, you'll learn how to configure Supabase, add server-side authentication, and protect pages from unauthorized users in a Next.js application. You'll also learn how to handle the authentication logic efficiently using Next.js server actions.
How to Configure Supabase Authentication in a Next.js application
First, create a Supabase account and an organization that will contain your various Supabase projects.

Add a new Supabase project to the organisation and copy the following credentials on your dashboard into a .env.local
file at the root of your project:
NEXT_PUBLIC_SUPABASE_ANON_KEY=<anon_key_from_Supabase_dashboard>
NEXT_PUBLIC_SUPABASE_URL=<supabase_project_url>
Create a utils/supabase
folder at the root of the Next.js project and add the following files to the folder: client.ts
, middleware.ts
, and server.ts
.
mkdir utils && cd utils
mkdir supabase && cd supabase
touch client.ts middleware.ts server.ts
Copy the following code into utils/supabase/
client.ts
. This initializes a Supabase browser client to interact with Supabase on client-side routes:
import { createBrowserClient } from "@supabase/ssr";
export function createClient() {
return createBrowserClient(
process.env.NEXT_PUBLIC_SUPABASE_URL!,
process.env.NEXT_PUBLIC_SUPABASE_ANON_KEY!
);
}
Next, copy the following code into utils/supabase/
server.ts
. This creates a Supabase server client for handling authentication and interacting with Supabase in server-side requests:
import { createServerClient } from "@supabase/ssr";
import { cookies } from "next/headers";
export async function createClient() {
const cookieStore = await cookies();
return createServerClient(
process.env.NEXT_PUBLIC_SUPABASE_URL!,
process.env.NEXT_PUBLIC_SUPABASE_ANON_KEY!,
{
cookies: {
getAll() {
return cookieStore.getAll();
},
setAll(cookiesToSet) {
try {
cookiesToSet.forEach(({ name, value, options }) =>
cookieStore.set(name, value, options)
);
} catch {
// The `setAll` method was called from a Server Component.
// This can be ignored if you have middleware refreshing
// user sessions.
}
},
},
}
);
}
Now, copy the following code into utils/supabase/
middleware.ts
. This middleware creates authentication cookies and protects pages from unauthorized access:
import { createServerClient } from "@supabase/ssr";
import { NextResponse, type NextRequest } from "next/server";
export async function updateSession(request: NextRequest) {
let supabaseResponse = NextResponse.next({
request,
});
//ππ» creates the Supabase cookie functions
const supabase = createServerClient(
process.env.NEXT_PUBLIC_SUPABASE_URL!,
process.env.NEXT_PUBLIC_SUPABASE_ANON_KEY!,
{
cookies: {
getAll() {
return request.cookies.getAll();
},
setAll(cookiesToSet) {
cookiesToSet.forEach(({ name, value }) =>
request.cookies.set(name, value)
);
supabaseResponse = NextResponse.next({
request,
});
cookiesToSet.forEach(({ name, value, options }) =>
supabaseResponse.cookies.set(name, value, options)
);
},
},
}
);
// ππ» placeholder for protected route controller
}
To enforce authentication, add the following code inside the placeholder in middleware.ts
. This checks if a user is signed in and redirects unauthenticated users to the login page:
//ππ» gets current user
const {
data: { user },
} = await supabase.auth.getUser();
//ππ» declares protected routes
if (
!user &&
request.nextUrl.pathname !== "/" &&
!request.nextUrl.pathname.startsWith("/instructor/auth") &&
!request.nextUrl.pathname.startsWith("/student/auth")
) {
//ππ» Redirect unauthenticated users to the login page
const url = request.nextUrl.clone();
url.pathname = "/student/auth/login"; // ππΌ redirect page
return NextResponse.redirect(url);
}
//ππ» returns Supabase response
return supabaseResponse;
Add another middleware.ts
file to the root of the Next.js project and copy the following code into the file:
import { type NextRequest } from "next/server";
import { updateSession } from "./utils/supabase/middleware";
export async function middleware(request: NextRequest) {
return await updateSession(request);
}
export const config = {
matcher: [
/*
* Match all request paths except for the ones starting with:
* - _next/static (static files)
* - _next/image (image optimization files)
* - favicon.ico (favicon file)
* Feel free to modify this pattern to include more paths.
*/
"/((?!_next/static|_next/image|favicon.ico|.*\.(?:svg|png|jpg|jpeg|gif|webp)$).*)",
],
};
Finally, create an auth/confirm
(dha-stix/stream-lms
) route and error (dha-stix/stream-lms
) page within the Next.js app folder.
You've successfully configured authentication in your Next.js project using Supabase.
Student Authentication with Supabase
In this section, you will learn how to create the signup and login functions for the students within the application.
First, create an actions
folder in the root of your Next.js project and add an auth.ts
file inside it. This file will contain all Supabase authentication functions.
Add the following imports to the top of the auth.ts
file:
"use server";
import { revalidatePath } from "next/cache";
import { redirect } from "next/navigation";
import { createClient } from "../utils/supabase/server";
Next, you need to create the server functions that accept form data from the client and sign users up or log them in as students.
Copy the following code snippet into the actions/
auth.ts
file to create the user sign-up function:
export async function studentSignUp(formData: FormData) {
const supabase = await createClient();
//ππ» Extract form data
const credentials = {
email: formData.get("email") as string,
password: formData.get("password") as string,
interest: formData.get("interest") as string,
name: formData.get("name") as string,
};
//ππ» Supabase sign up function (options attribute :- for user metadata)
const { data, error } = await supabase.auth.signUp({
email: credentials.email,
password: credentials.password,
options: {
data: {
interest: credentials.interest,
name: credentials.name,
},
},
});
//ππ» return user or error object
}
The code snippet above accepts the form credentials such as email, password, interest, and name, and signs the user up as a Supabase user.
Modify the function to return the user or error object.
export async function studentSignUp(formData: FormData) {
//...form inputs and supabase functions
if (error) {
return { error: error.message, status: error.status, user: null };
} else if (data.user?.identities?.length === 0) {
return { error: "User already exists", status: 409, user: null };
}
revalidatePath("/", "layout");
return { error: null, status: 200, user: data.user };
}
Create the student login function as shown below:
export async function studentLogIn(formData: FormData) {
const supabase = await createClient();
const credentials = {
email: formData.get("email") as string,
password: formData.get("password") as string,
};
const { data, error } = await supabase.auth.signInWithPassword(credentials);
if (error) {
return { error: error.message, status: error.status, user: null };
}
//ππ» only instructors have an image attribute
if (data && data.user.user_metadata.image) {
return { error: "You are not a student", status: 400, user: null };
}
//ππ» create a student row and add to the database
revalidatePath("/", "layout");
return { error: null, status: 200, user: data.user };
}
The code above takes the student's email and password to log them into the application.
- If an error occurs, it returns an error message.
- If the user object includes an image attribute (indicating that they are an instructor), they are prevented from logging in.
Once the student is signed in, you must store their details in a Supabase table. This allows you to add a following_list
column that tracks the instructors they follow. The list will be updated whenever the student follows or unfollows an instructor.
export async function studentLogIn(formData: FormData) {
//...other functions
const { data: existingUser } = await supabase
.from("students")
.select()
.eq("email", credentials.email)
.single();
//ππ» if student doesn't exist
if (!existingUser) {
const { error: insertError } = await supabase.from("students").insert({
email: credentials.email,
name: data.user.user_metadata.name,
interest: data.user.user_metadata.interest,
id: data.user.id,
following_list: [] as string[],
});
if (insertError) {
return { error: insertError.message, status: 500, user: null };
}
}
revalidatePath("/", "layout");
return { error: null, status: 200, user: data.user };
}
Every time a student logs in, the code checks if they already exist in the students
table.
- If the student is found, no new entry is created.
- If the student is not found, a new row with their details is added.
Each studentβs data includes two primary keys: id
and email
and additional columns: interest
, name
, and following_list
.

Instructor Authentication with Supabase
The instructor's user object is quite different from the student's. It includes data such as email, password, name, interest, occupation, bio, URL, and image.
Add the following function to actions/
auth.ts
to handle instructor sign-ups:
export async function instructorSignUp(formData: FormData) {
const supabase = await createClient();
//ππ» get user credentials from the form
const credentials = {
email: formData.get("email") as string,
password: formData.get("password") as string,
interest: formData.get("interest") as string,
name: formData.get("name") as string,
occupation: formData.get("occupation") as string,
bio: formData.get("bio") as string,
url: formData.get("url") as string,
image: formData.get("image") as File,
};
//ππ» following code snippet below
}
Next, upload the image to Supabase Storage and retrieve its download URL before signing up the user as an instructor. Update the instructorSignUp
function to show this:
export async function instructorSignUp(formData: FormData) {
//ππ» upload instructor's image
const { data: imageData, error: imageError } = await supabase.storage
.from("headshots")
.upload(`${crypto.randomUUID()}/image`, credentials.image);
if (imageError) {
return { error: imageError.message, status: 500, user: null };
}
//ππ» get the image URL
const imageURL = `${process.env.STORAGE_URL!}${imageData.fullPath}`;
//ππ» authenticate user as instructor
const { data, error } = await supabase.auth.signUp({
email: credentials.email,
password: credentials.password,
options: {
data: {
interest: credentials.interest,
name: credentials.name,
occupation: credentials.occupation,
bio: credentials.bio,
url: credentials.url,
image: imageURL,
},
},
});
//ππ» return user or error object
if (error) {
return { error: error.message, status: error.status, user: null };
}
revalidatePath("/", "layout");
return { error: null, status: 200, user: data.user };
}
Finally, an instructor login function (dha-stix/stream-lms
) that authenticates the user, similar to the student login function, should be created. It should check whether the instructor already exists in the instructors
table. If the instructor does not exist, execute the function to add the instructor's user object to the database table.
Here is the Supabase function for adding an instructor to the table:
const { error: insertError } = await supabase.from("instructors").insert({
email: credentials.email,
name: data.user.user_metadata.name,
occupation: data.user.user_metadata.occupation,
bio: data.user.user_metadata.bio,
url: data.user.user_metadata.url,
image: data.user.user_metadata.image,
id: data.user.id,
interest: data.user.user_metadata.interest,
followers: [],
});
The instructors
table includes an additional followers
attribute, which stores an array of student IDs following the instructor. You can find the complete code on GitHub (dha-stix/stream-lms
).
Additionally, authentication functions like getUserSession
(dha-stix/stream-lms
) and logOut
(dha-stix/stream-lms
) must be created. These functions will retrieve the current user's object and allow them to log out when necessary, such as when clicking a logout button.
