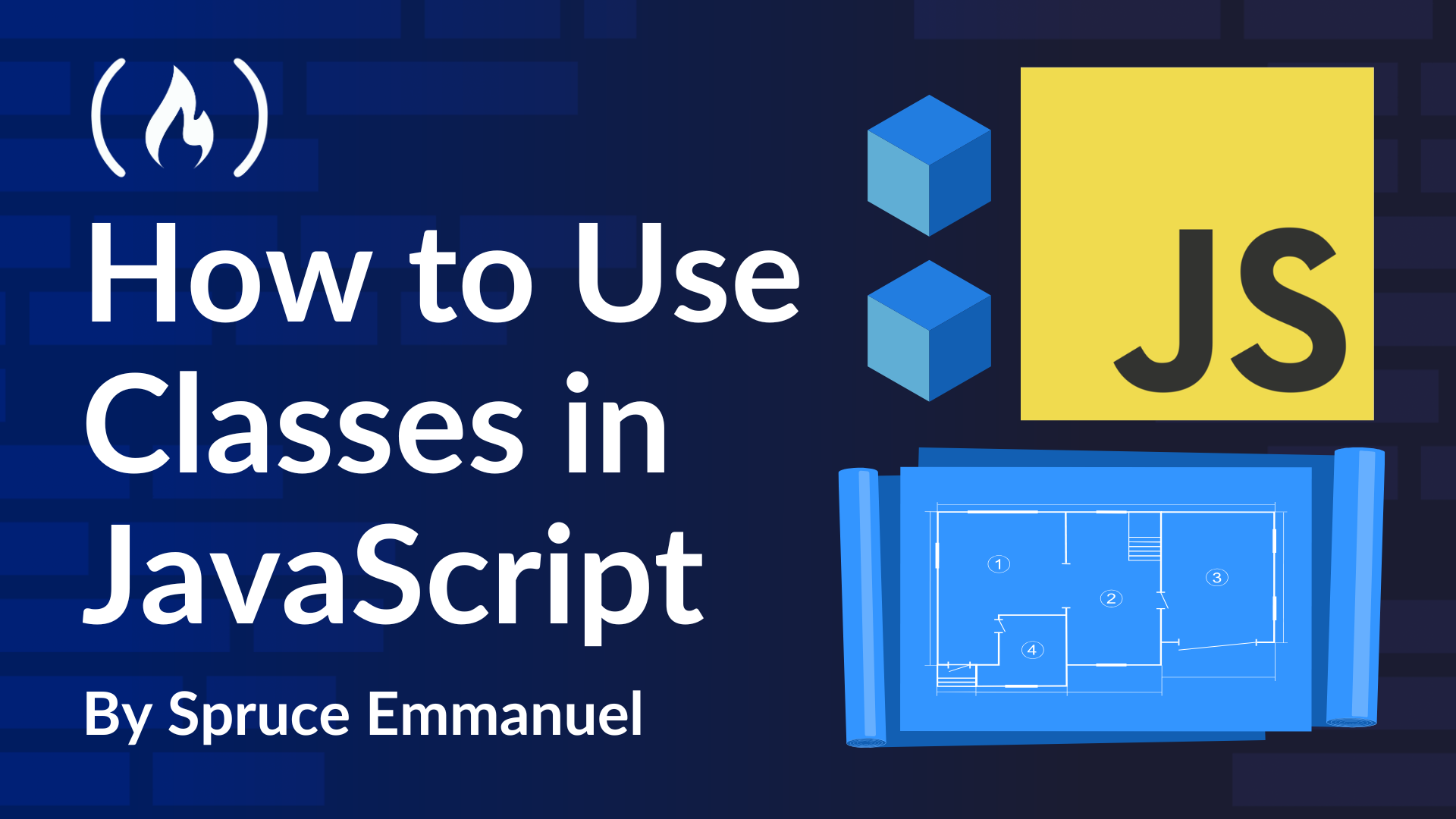
Constructor Functions: Great for Blueprints, but... Memory Waste?
Constructor Functions: Great for Blueprints, but... Memory Waste? 관련
Okay, so now that you know how to create object blueprints using constructor functions, and you understand what this
does, we can make lots of Person
objects.
But there's a little problem lurking in our PersonConstructor
:
function PersonConstructor(name, age) {
this.name = name;
this.age = age;
this.greet = function () {
// 😬 Look at this greet function!
console.log(`Hello, I'm ${this.name}`);
};
}
const person1 = new PersonConstructor("Alice", 25);
const person2 = new PersonConstructor("Bob", 30);
console.log(person1, person2);
//
// PersonConstructor {name: "Alice", age: 25, greet: function}
// PersonConstructor {name: "Bob", age: 30, greet: function}
Notice the greet
function inside the PersonConstructor
? Every time we create a new Person
object using new PersonConstructor()
, we're actually copying the entire greet
function to each and every object.
Imagine that we create one thousand Person
objects. We'd have a thousand identical greet
functions in memory. For a simple greet()
function, the memory impact might seem small. However, if you had more complex methods with lots of code, or if you were creating thousands or even millions of objects, duplicating these functions for every single object can become a significant waste of memory.
It also impacts performance as JavaScript has to manage all these duplicated functions. That's a lot of duplicated code, and it's not very memory-efficient, especially if the greet
function (or other methods) were more complex.