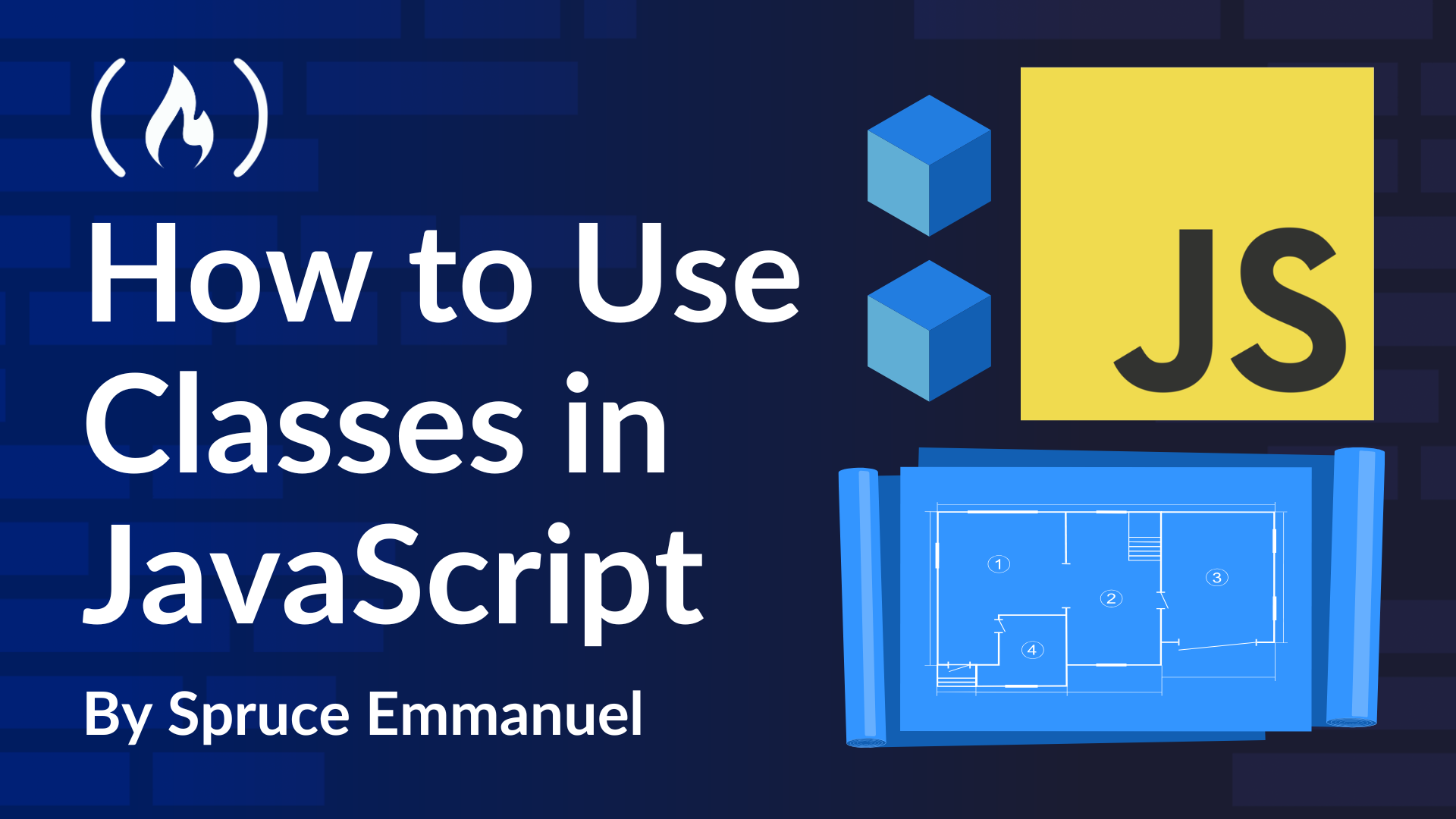
Prototypes to the Rescue (Again): Sharing Methods Efficiently
Prototypes to the Rescue (Again): Sharing Methods Efficiently 관련
Remember prototypes? We learned that objects can inherit properties and methods from their prototypes. Well, constructor functions have a built-in way to use prototypes to solve this memory-waste problem.
Instead of defining the greet
function inside the constructor and thus copying it to every instance, we can add it to the prototype
of the PersonConstructor
function.
Like this:
function PersonConstructor(name, age) {
this.name = name;
this.age = age;
}
// --- Add the greet method to the PROTOTYPE of PersonConstructor! ---
PersonConstructor.prototype.greet = function () {
console.log(`Hello, I'm ${this.name}`);
};
Now, the greet
method is defined only once on PersonConstructor.prototype
. But all objects created with PersonConstructor
can still use it. They inherit it from the prototype.
Let's test it:
const person1 = new PersonConstructor("Alice", 25);
const person2 = new PersonConstructor("Bob", 30);
person1.greet(); // Output: Hello, I'm Alice - Still works!
person2.greet(); // Output: Hello, I'm Bob - Still works!
console.log(person1.greet === person2.greet); // Output: false - They are NOT the same function object in memory
console.log(person1.__proto__.greet === person2.__proto__.greet); // Output: true - But they share the same prototype method!
person1.greet()
and person2.greet()
still work perfectly. But now, the greet
function is not copied for each object. It's shared through the prototype. This is much more efficient, especially when we're dealing with lots of objects and methods.