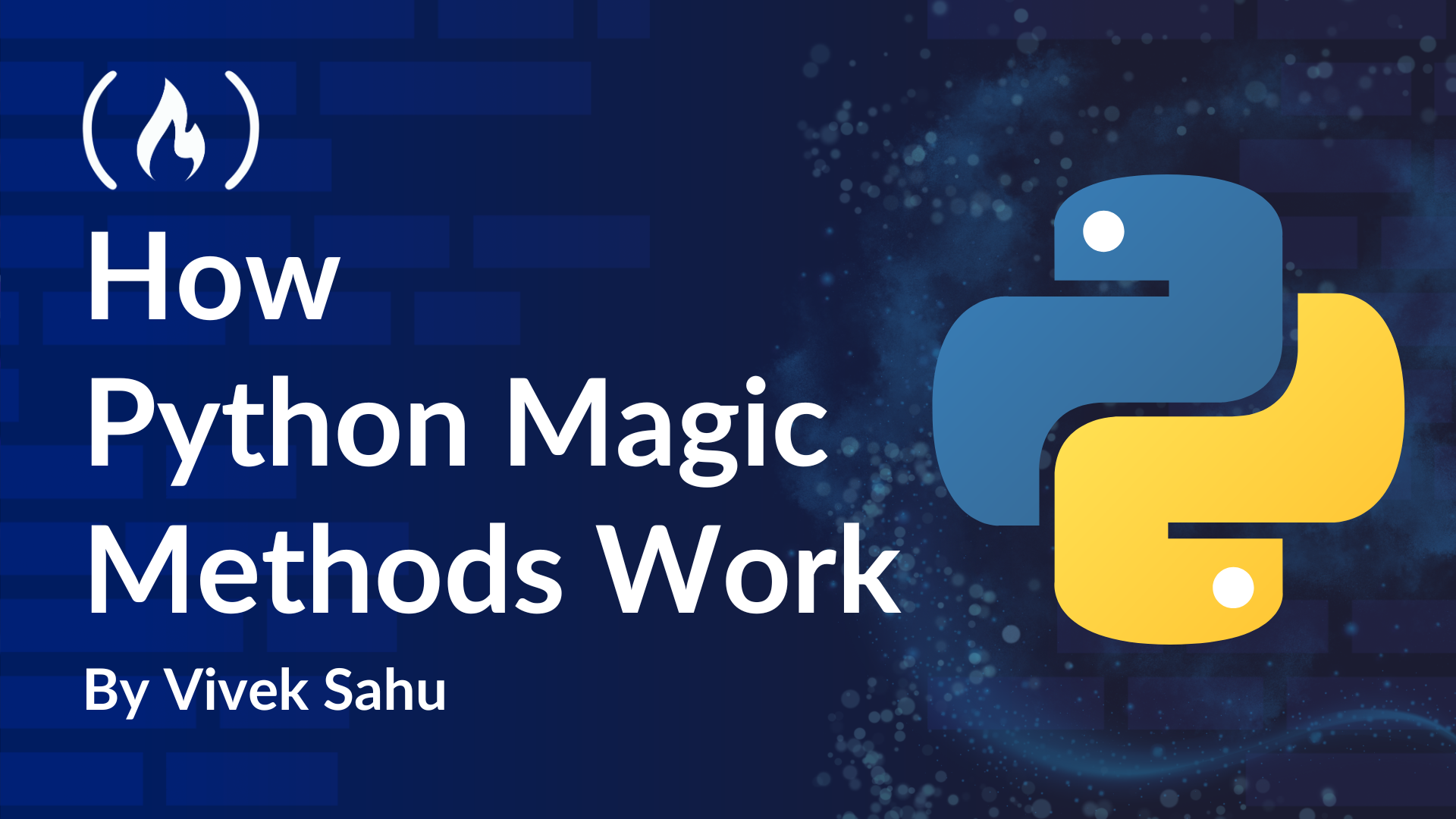
How Python Magic Methods Work: A Practical Guide
How Python Magic Methods Work: A Practical Guide 관련
Have you ever wondered how Python makes objects work with operators like +
or -
? Or how it knows how to display objects when you print them? The answer lies in Python's magic methods, also known as dunder (double under) methods.
Magic methods are special methods that let you define how your objects behave in response to various operations and built-in functions. They're what makes Python's object-oriented programming so powerful and intuitive.
In this guide, you'll learn how to use magic methods to create more elegant and powerful code. You'll see practical examples that show how these methods work in real-world scenarios.
Prerequisites
- Basic understanding of Python syntax and object-oriented programming concepts.
- Familiarity with classes, objects, and inheritance.
- Knowledge of built-in Python data types (lists, dictionaries, and so on).
- A working Python 3 installation is recommended to actively engage with the examples here.
Wrapping Up
Python's magic methods provide a powerful way to make your classes behave like built-in types, enabling more intuitive and expressive code. Throughout this guide, we've explored how these methods work and how to use them effectively.
Key Takeaways
- Object representation:
- Use
__str__
for user-friendly output - Use
__repr__
for debugging and development
- Use
- Operator overloading:
- Implement arithmetic and comparison operators
- Return
NotImplemented
for unsupported operations - Use
@total_ordering
for consistent comparisons
- Container behavior:
- Implement sequence and mapping protocols
- Consider performance for frequently used operations
- Handle edge cases appropriately
- Resource management:
- Use context managers for proper resource handling
- Implement
__enter__
and__exit__
for cleanup - Handle exceptions in
__exit__
- Performance optimization:
- Use
__slots__
for memory efficiency - Cache computed values when appropriate
- Minimize method calls in frequently used code
- Use
When to Use Magic Methods
Magic methods are most useful when you need to:
- Create custom data structures
- Implement domain-specific types
- Manage resources properly
- Add special behavior to your classes
- Make your code more Pythonic
When to Avoid Magic Methods
Avoid magic methods when:
- Simple attribute access is sufficient
- The behavior would be confusing or unexpected
- Performance is critical and magic methods would add overhead
- The implementation would be overly complex
Remember that with great power comes great responsibility. Use magic methods judiciously, keeping in mind their performance implications and the principle of least surprise. When used appropriately, magic methods can significantly enhance the readability and expressiveness of your code.
References and Further Reading
Official Python Documentation
Community Resources
Further Reading
If you enjoyed this article, you might find these Python-related articles on my personal blog useful: