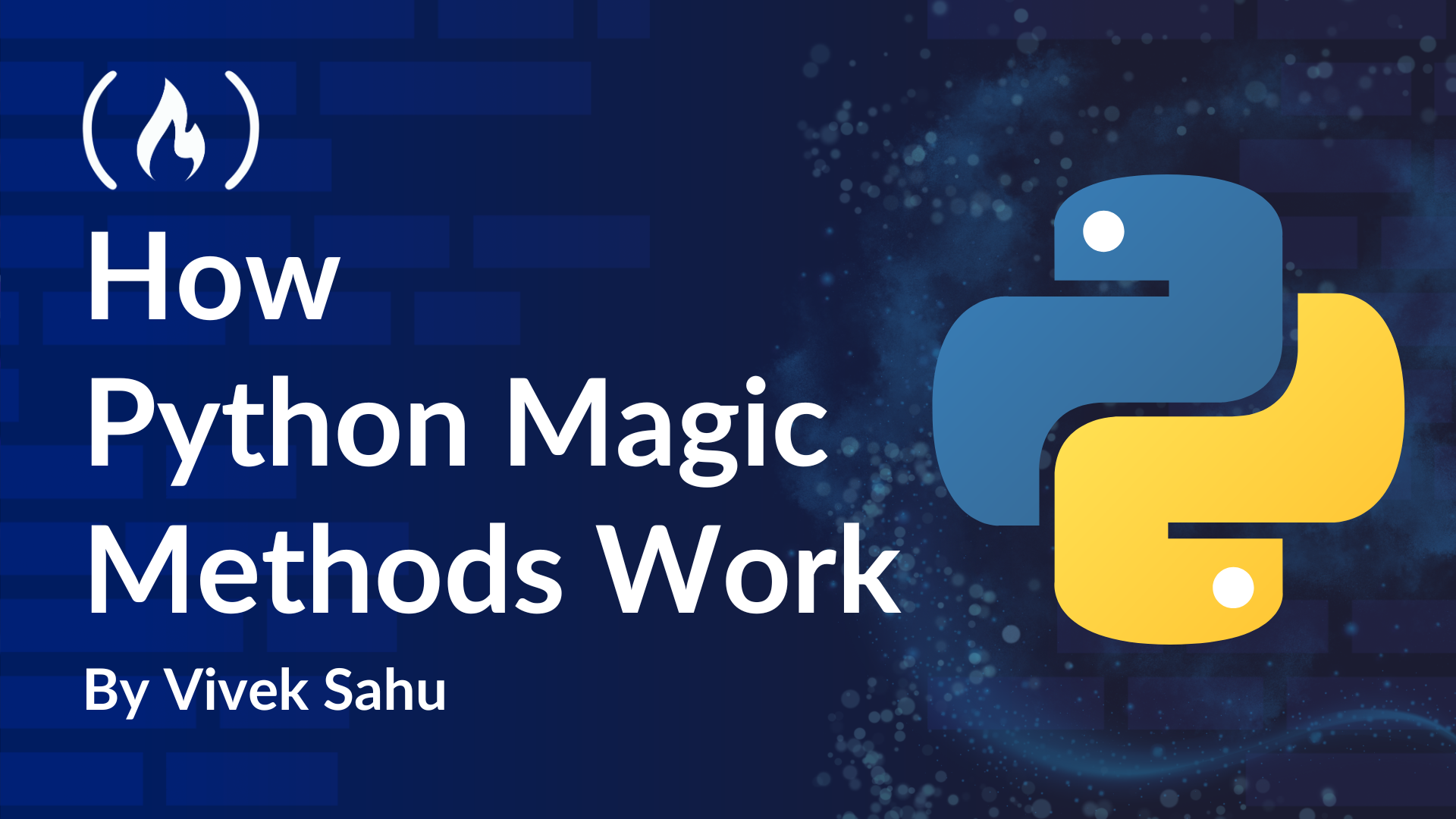
Performance Considerations
March 21, 2025About 2 min
Performance Considerations 관련
How Python Magic Methods Work: A Practical Guide
Have you ever wondered how Python makes objects work with operators like + or -? Or how it knows how to display objects when you print them? The answer lies in Python's magic methods, also known as dunder (double under) methods. Magic methods are spe...
How Python Magic Methods Work: A Practical Guide
Have you ever wondered how Python makes objects work with operators like + or -? Or how it knows how to display objects when you print them? The answer lies in Python's magic methods, also known as dunder (double under) methods. Magic methods are spe...
While magic methods are powerful, they can impact performance if you don’t use them carefully. Let's explore some common performance considerations and how to measure them.
Impact of Magic Methods on Performance
Different magic methods have different performance implications:
Attribute Access methods
__getattr__
,__getattribute__
,__setattr__
, and__delattr__
are called frequently- Complex operations in these methods can significantly slow down your code
Container methods
__getitem__
,__setitem__
, and__len__
are called often in loops- Inefficient implementations can make your container much slower than built-in types
Operator overloading
- Arithmetic and comparison operators are used frequently
- Complex implementations can make simple operations unexpectedly slow
Let's measure the performance impact of __getattr__
vs. direct attribute access:
import time
class DirectAccess:
def __init__(self):
self.value = 42
class GetAttrAccess:
def __init__(self):
self._value = 42
def __getattr__(self, name):
if name == "value":
return self._value
raise AttributeError(f"'{self.__class__.__name__}' object has no attribute '{name}'")
# Measure performance
direct = DirectAccess()
getattr_obj = GetAttrAccess()
def benchmark(obj, iterations=1000000):
start = time.time()
for _ in range(iterations):
x = obj.value
end = time.time()
return end - start
direct_time = benchmark(direct)
getattr_time = benchmark(getattr_obj)
print(f"Direct access: {direct_time:.6f} seconds")
print(f"__getattr__ access: {getattr_time:.6f} seconds")
print(f"__getattr__ is {getattr_time / direct_time:.2f}x slower")
Running this benchmark shows significant performance differences:
Direct access: 0.027714 seconds
__getattr__ access: 0.060646 seconds
__getattr__ is 2.19x slower
As you can see, using __getattr__
is more than twice as slow as direct attribute access. This might not matter for occasionally accessed attributes, but it can become significant in performance-critical code that accesses attributes in tight loops.
Optimization Strategies
Fortunately, there are various ways you can optimize magic methods.
- Use slots for memory efficiency: This reduces memory usage and improves attribute access speed. It’s best for classes with many instances.
- Cache computed values: You can store results of expensive operations and update the cache only when necessary. Use
@property
for computed attributes. - Minimize method calls: Make sure you avoid unnecessary magic method calls and use direct attribute access when possible. Consider using
__slots__
for frequently accessed attributes.