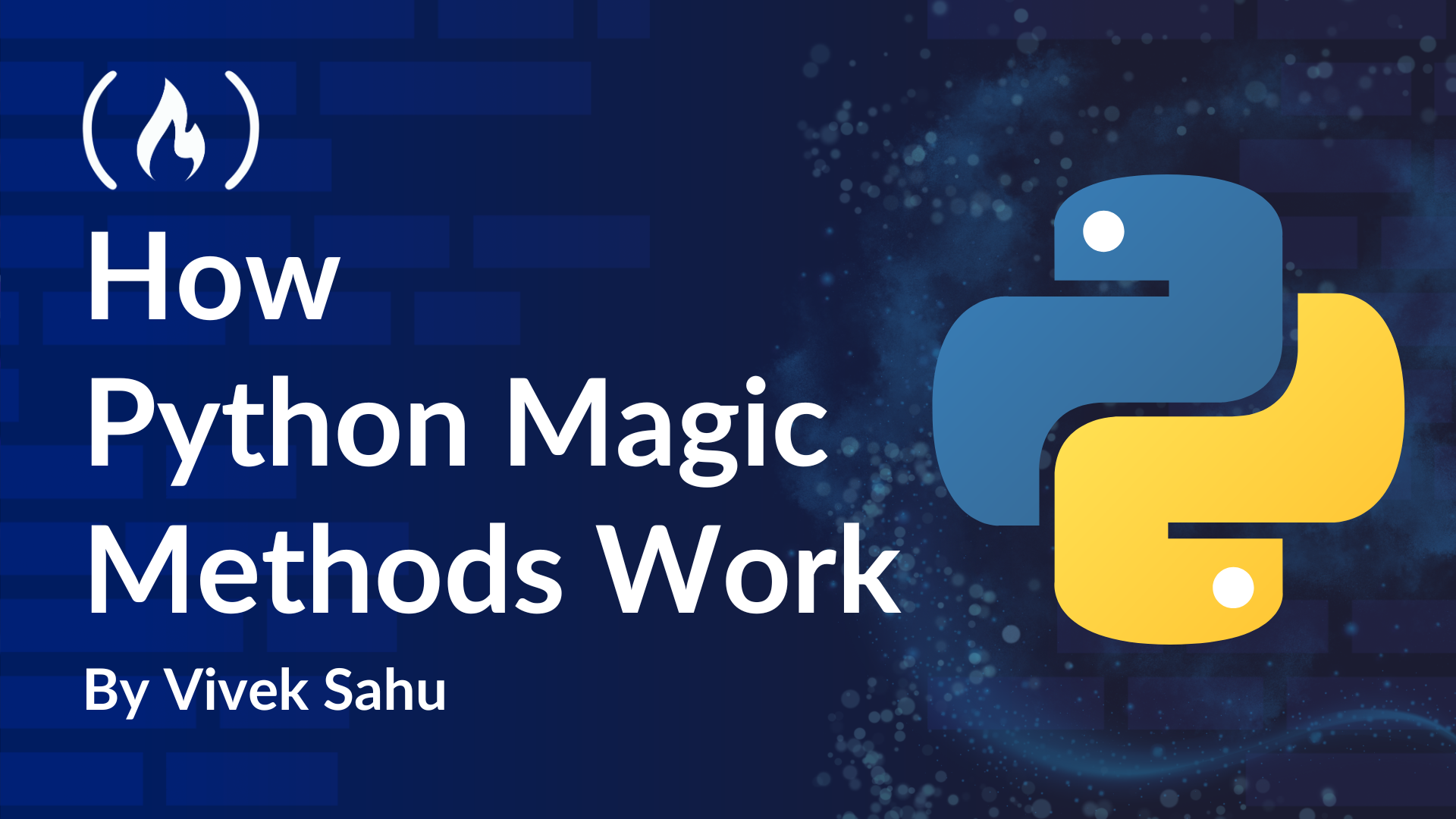
Operator Overloading
March 21, 2025About 3 min
Operator Overloading 관련
How Python Magic Methods Work: A Practical Guide
Have you ever wondered how Python makes objects work with operators like + or -? Or how it knows how to display objects when you print them? The answer lies in Python's magic methods, also known as dunder (double under) methods. Magic methods are spe...
How Python Magic Methods Work: A Practical Guide
Have you ever wondered how Python makes objects work with operators like + or -? Or how it knows how to display objects when you print them? The answer lies in Python's magic methods, also known as dunder (double under) methods. Magic methods are spe...
Operator overloading is one of the most powerful features of Python's magic methods. It lets you define how your objects behave when used with operators like +
, -
, *
, and ==
. This makes your code more intuitive and readable.
Arithmetic Operators
Python provides magic methods for all basic arithmetic operations. Here's a table showing which method corresponds to which operator:
Operator | Magic Method | Description |
---|---|---|
+ | __add__ | Addition |
- | __sub__ | Subtraction |
* | __mul__ | Multiplication |
/ | __truediv__ | Division |
// | __floordiv__ | Floor division |
% | __mod__ | Modulo |
** | __pow__ | Exponentiation |
Comparison Operators
Similarly, you can define how your objects are compared using these magic methods:
Operator | Magic Method | Description |
---|---|---|
== | __eq__ | Equal to |
!= | __ne__ | Not equal to |
< | __lt__ | Less than |
> | __gt__ | Greater than |
<= | __le__ | Less than or equal to |
>= | __ge__ | Greater than or equal to |
Practical Example: Money Class
Let's create a Money
class that handles currency operations correctly. This example shows how to implement multiple operators and handle edge cases:
from functools import total_ordering
from decimal import Decimal
@total_ordering # Implements all comparison methods based on __eq__ and __lt__
class Money:
def __init__(self, amount, currency="USD"):
self.amount = Decimal(str(amount))
self.currency = currency
def __add__(self, other):
if not isinstance(other, Money):
return NotImplemented
if self.currency != other.currency:
raise ValueError(f"Cannot add different currencies: {self.currency} and {other.currency}")
return Money(self.amount + other.amount, self.currency)
def __sub__(self, other):
if not isinstance(other, Money):
return NotImplemented
if self.currency != other.currency:
raise ValueError(f"Cannot subtract different currencies: {self.currency} and {other.currency}")
return Money(self.amount - other.amount, self.currency)
def __mul__(self, other):
if isinstance(other, (int, float, Decimal)):
return Money(self.amount * Decimal(str(other)), self.currency)
return NotImplemented
def __truediv__(self, other):
if isinstance(other, (int, float, Decimal)):
return Money(self.amount / Decimal(str(other)), self.currency)
return NotImplemented
def __eq__(self, other):
if not isinstance(other, Money):
return NotImplemented
return self.currency == other.currency and self.amount == other.amount
def __lt__(self, other):
if not isinstance(other, Money):
return NotImplemented
if self.currency != other.currency:
raise ValueError(f"Cannot compare different currencies: {self.currency} and {other.currency}")
return self.amount < other.amount
def __str__(self):
return f"{self.currency} {self.amount:.2f}"
def __repr__(self):
return f"Money({repr(float(self.amount))}, {repr(self.currency)})"
Let's break down the key features of this Money
class:
- Precision handling: We use
Decimal
instead offloat
to avoid floating-point precision issues with money calculations. - Currency safety: The class prevents operations between different currencies to avoid errors.
- Type checking: Each method checks if the other operand is of the correct type using
isinstance()
. - NotImplemented: When an operation doesn't make sense, we return
NotImplemented
to let Python try the reverse operation. @total_ordering
: This decorator automatically implements all comparison methods based on__eq__
and__lt__
.
Here's how to use the Money
class:
# Basic arithmetic
wallet = Money(100, "USD")
expense = Money(20, "USD")
remaining = wallet - expense
print(remaining) # Output: USD 80.00
# Working with different currencies
salary = Money(5000, "USD")
bonus = Money(1000, "USD")
total = salary + bonus
print(total) # Output: USD 6000.00
# Division by scalar
weekly_pay = salary / 4
print(weekly_pay) # Output: USD 1250.00
# Comparisons
print(Money(100, "USD") > Money(50, "USD")) # Output: True
print(Money(100, "USD") == Money(100, "USD")) # Output: True
# Error handling
try:
Money(100, "USD") + Money(100, "EUR")
except ValueError as e:
print(e) # Output: Cannot add different currencies: USD and EUR
This Money
class demonstrates several important concepts:
- How to handle different types of operands
- How to implement proper error handling
- How to use the
@total_ordering
decorator - How to maintain precision in financial calculations
- How to provide both string and representation methods