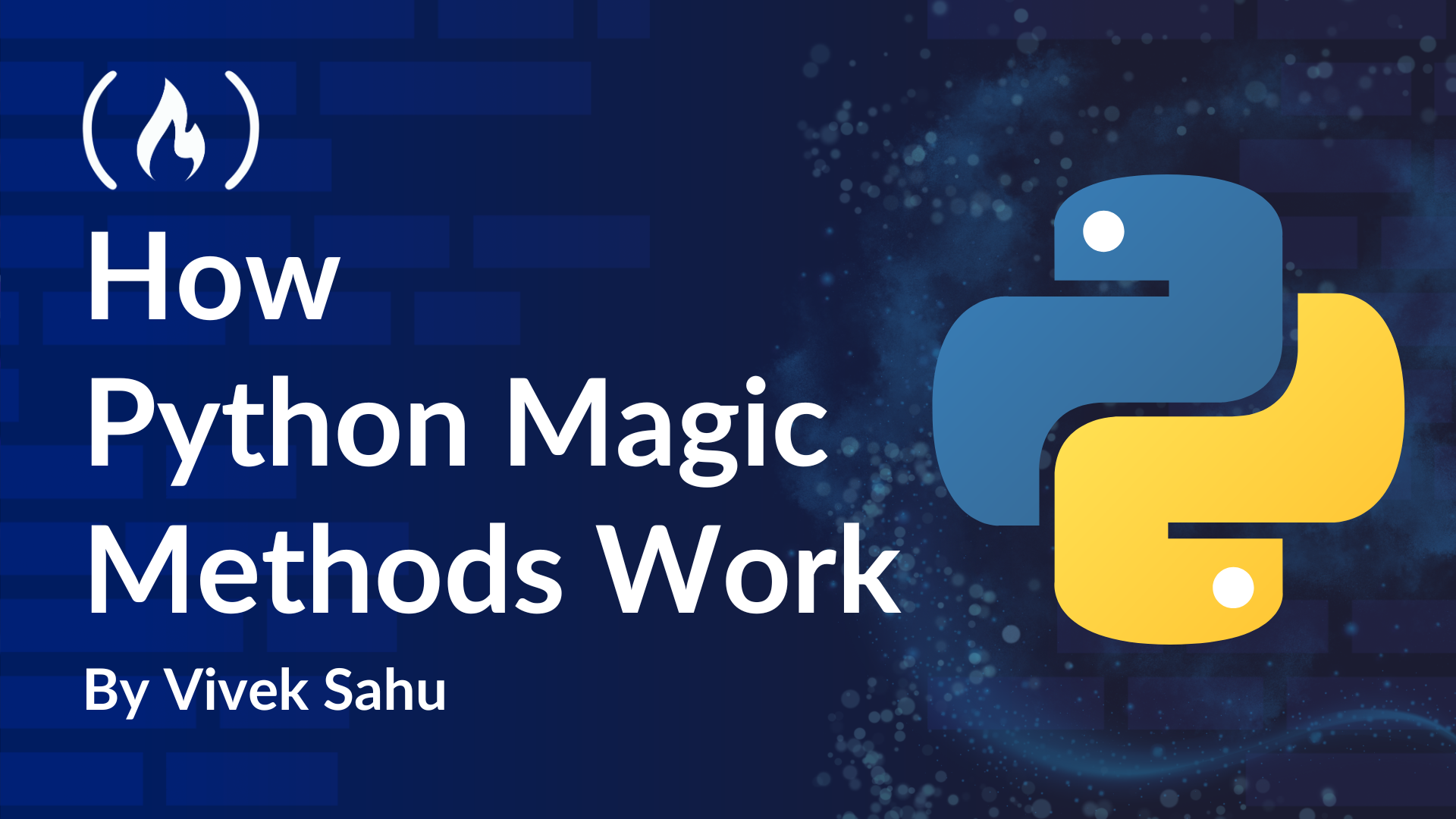
Attribute Access
Attribute Access 관련
Attribute access methods let you control how your objects handle getting, setting, and deleting attributes. This is particularly useful for implementing properties, validation, and logging.
getattr and getattribute
Python provides two methods for controlling attribute access:
__getattr__
: Called only when an attribute lookup fails (that is, when the attribute doesn't exist)__getattribute__
: Called for every attribute access, even for attributes that exist
The key difference is that __getattribute__
is called for all attribute access, while __getattr__
is only called when the attribute isn't found through normal means.
Here's a simple example showing the difference:
class AttributeDemo:
def __init__(self):
self.name = "Vivek"
def __getattr__(self, name):
print(f"__getattr__ called for {name}")
return f"Default value for {name}"
def __getattribute__(self, name):
print(f"__getattribute__ called for {name}")
return super().__getattribute__(name)
demo = AttributeDemo()
print(demo.name) # Output: __getattribute__ called for name
# Vivek
print(demo.age) # Output: __getattribute__ called for age
# __getattr__ called for age
# Default value for age
__setattr__
and __delattr__
Similarly, you can control how attributes are set and deleted:
__setattr__
: Called when an attribute is set__delattr__
: Called when an attribute is deleted
These methods let you implement validation, logging, or custom behavior when attributes are modified.
Practical Example: Auto-Logging Properties
Let's create a class that automatically logs all property changes. This is useful for debugging, auditing, or tracking object state changes:
import logging
# Set up logging
logging.basicConfig(
level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s'
)
class LoggedObject:
def __init__(self, **kwargs):
self._data = {}
# Initialize attributes without triggering __setattr__
for key, value in kwargs.items():
self._data[key] = value
def __getattr__(self, name):
if name in self._data:
logging.debug(f"Accessing attribute {name}: {self._data[name]}")
return self._data[name]
raise AttributeError(f"'{self.__class__.__name__}' object has no attribute '{name}'")
def __setattr__(self, name, value):
if name == "_data":
# Allow setting the _data attribute directly
super().__setattr__(name, value)
else:
old_value = self._data.get(name, "<undefined>")
self._data[name] = value
logging.info(f"Changed {name}: {old_value} -> {value}")
def __delattr__(self, name):
if name in self._data:
old_value = self._data[name]
del self._data[name]
logging.info(f"Deleted {name} (was: {old_value})")
else:
raise AttributeError(f"'{self.__class__.__name__}' object has no attribute '{name}'")
Let's break down how this class works:
- Storage: The class uses a private
_data
dictionary to store attribute values. - Attribute access:
__getattr__
: Returns values from_data
and logs debug messages__setattr__
: Stores values in_data
and logs changes__delattr__
: Removes values from_data
and logs deletions
- Special handling: The
_data
attribute itself is handled differently to avoid infinite recursion.
Here's how to use the class:
# Create a logged object with initial values
user = LoggedObject(name="Vivek", email="hello@wewake.dev")
# Modify attributes
user.name = "Vivek" # Logs: Changed name: Vivek -> Vivek
user.age = 30 # Logs: Changed age: <undefined> -> 30
# Access attributes
print(user.name) # Output: Vivek
# Delete attributes
del user.email # Logs: Deleted email (was: hello@wewake.dev)
# Try to access deleted attribute
try:
print(user.email)
except AttributeError as e:
print(f"AttributeError: {e}") # Output: AttributeError: 'LoggedObject' object has no attribute 'email'
This implementation provides several benefits:
- Automatic logging of all attribute changes
- Debug-level logging for attribute access
- Clear error messages for missing attributes
- Easy tracking of object state changes
- Useful for debugging and auditing