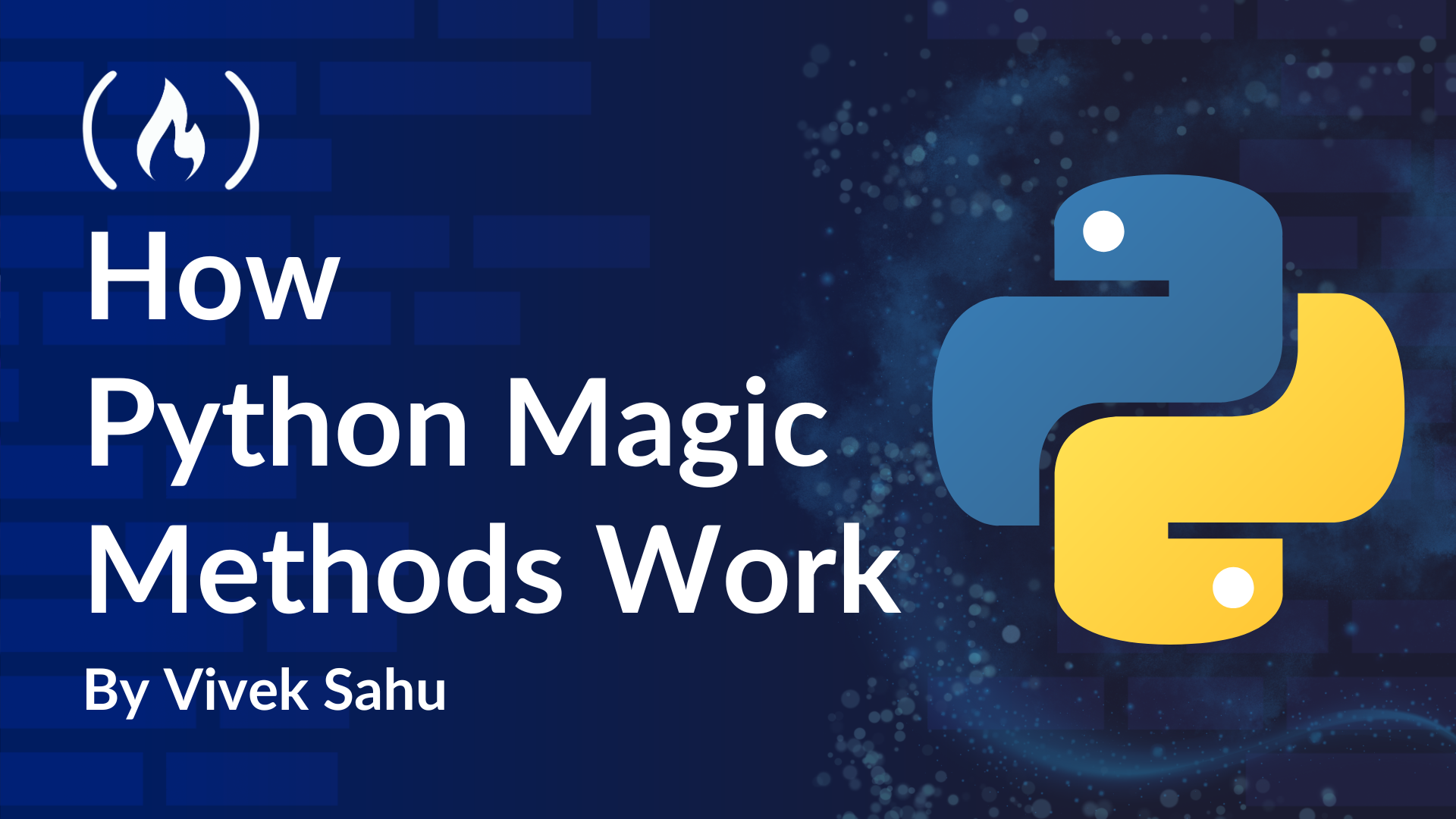
Callable Objects
March 21, 2025About 2 min
Callable Objects 관련
How Python Magic Methods Work: A Practical Guide
Have you ever wondered how Python makes objects work with operators like + or -? Or how it knows how to display objects when you print them? The answer lies in Python's magic methods, also known as dunder (double under) methods. Magic methods are spe...
How Python Magic Methods Work: A Practical Guide
Have you ever wondered how Python makes objects work with operators like + or -? Or how it knows how to display objects when you print them? The answer lies in Python's magic methods, also known as dunder (double under) methods. Magic methods are spe...
The __call__
magic method lets you make instances of your class behave like functions. This is useful for creating objects that maintain state between calls or for implementing function-like behavior with additional features.
__call__
The __call__
method is called when you try to call an instance of your class as if it were a function. Here's a simple example:
class Multiplier:
def __init__(self, factor):
self.factor = factor
def __call__(self, x):
return x * self.factor
# Create instances that behave like functions
double = Multiplier(2)
triple = Multiplier(3)
print(double(5)) # Output: 10
print(triple(5)) # Output: 15
This example shows how __call__
lets you create objects that maintain state (the factor) while being callable like functions.
Practical Example: Memoization Decorator
Let's implement a memoization decorator using __call__
. This decorator will cache function results to avoid redundant computations:
import time
import functools
class Memoize:
def __init__(self, func):
self.func = func
self.cache = {}
# Preserve function metadata (name, docstring, etc.)
functools.update_wrapper(self, func)
def __call__(self, *args, **kwargs):
# Create a key from the arguments
# For simplicity, we assume all arguments are hashable
key = str(args) + str(sorted(kwargs.items()))
if key not in self.cache:
self.cache[key] = self.func(*args, **kwargs)
return self.cache[key]
# Usage
@Memoize
def fibonacci(n):
"""Calculate the nth Fibonacci number recursively."""
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
# Measure execution time
def time_execution(func, *args, **kwargs):
start = time.time()
result = func(*args, **kwargs)
end = time.time()
print(f"{func.__name__}({args}, {kwargs}) took {end - start:.6f} seconds")
return result
# Without memoization, this would be extremely slow
print("Calculating fibonacci(35)...")
result = time_execution(fibonacci, 35)
print(f"Result: {result}")
# Second call is instant due to memoization
print("\nCalculating fibonacci(35) again...")
result = time_execution(fibonacci, 35)
print(f"Result: {result}")
Let's break down how this memoization decorator works:
- Initialization:
- Takes a function as an argument
- Creates a cache dictionary to store results
- Preserves the function's metadata using
functools.update_wrapper
- Call method:
- Creates a unique key from the function arguments
- Checks if the result is in the cache
- If not, computes the result and stores it
- Returns the cached result
- Usage:
- Applied as a decorator to any function
- Automatically caches results for repeated calls
- Preserves function metadata and behavior
The benefits of this implementation include:
- Better performance, as it avoids redundant computations
- Better, transparency, as it works without modifying the original function
- It’s flexible, and can be used with any function
- It’s memory efficient and caches results for reuse
- It maintains function documentation