How to load a remote image from a URL
How to load a remote image from a URL 관련
Updated for Xcode 15
New in iOS 15
SwiftUI has a dedicated AsyncImage
for downloading and displaying remote images from the internet. In its simplest form you can just pass a URL, like this:
AsyncImage(url: URL(string: "https://hws.dev/paul.jpg"))
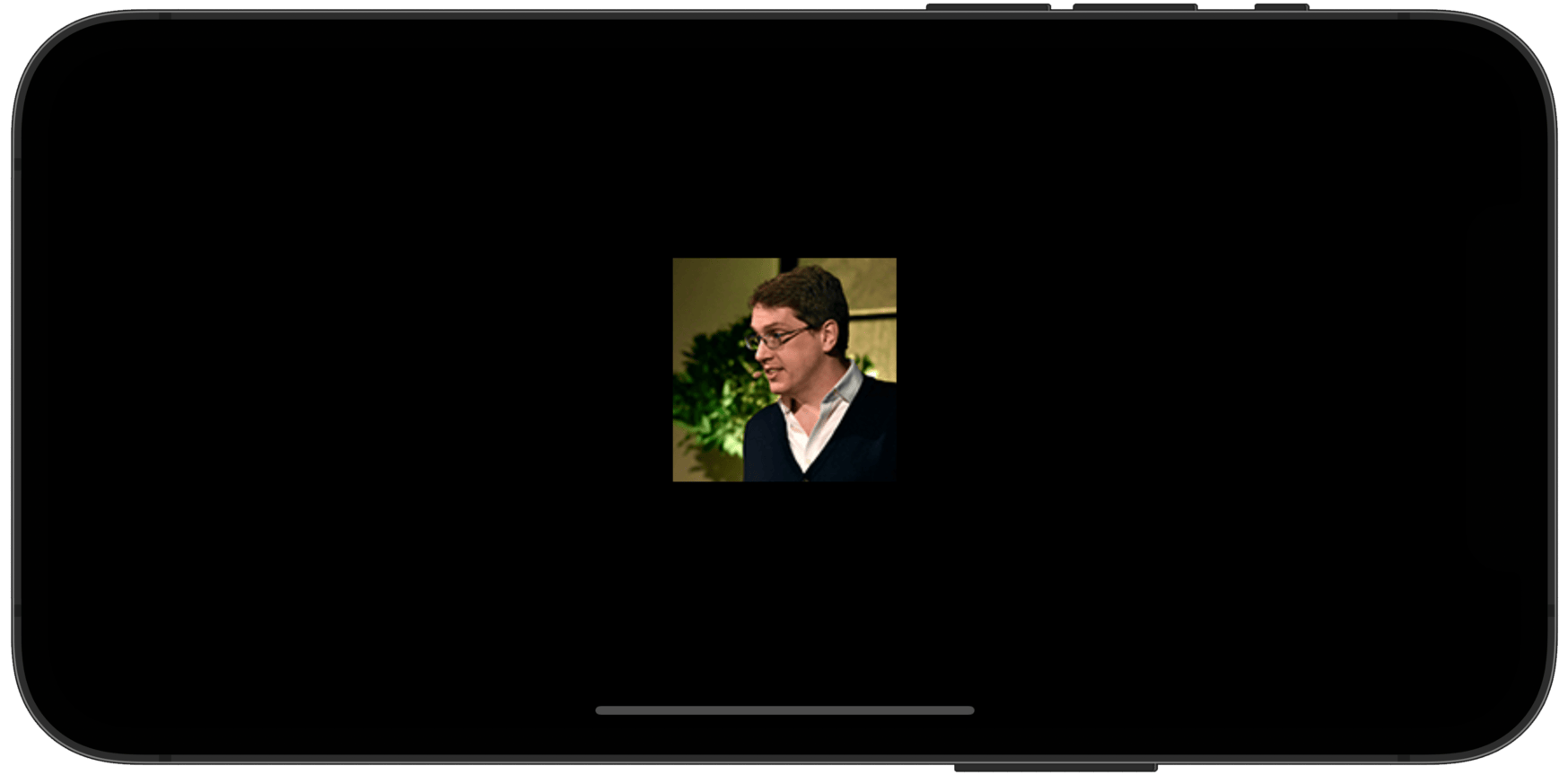
Note how the URL is optional – the AsyncImage
will simply show a default gray placeholder if the URL string is invalid. And if the image can't be loaded for some reason – if the user is offline, or if the image doesn't exist – then the system will continue showing the same placeholder image.
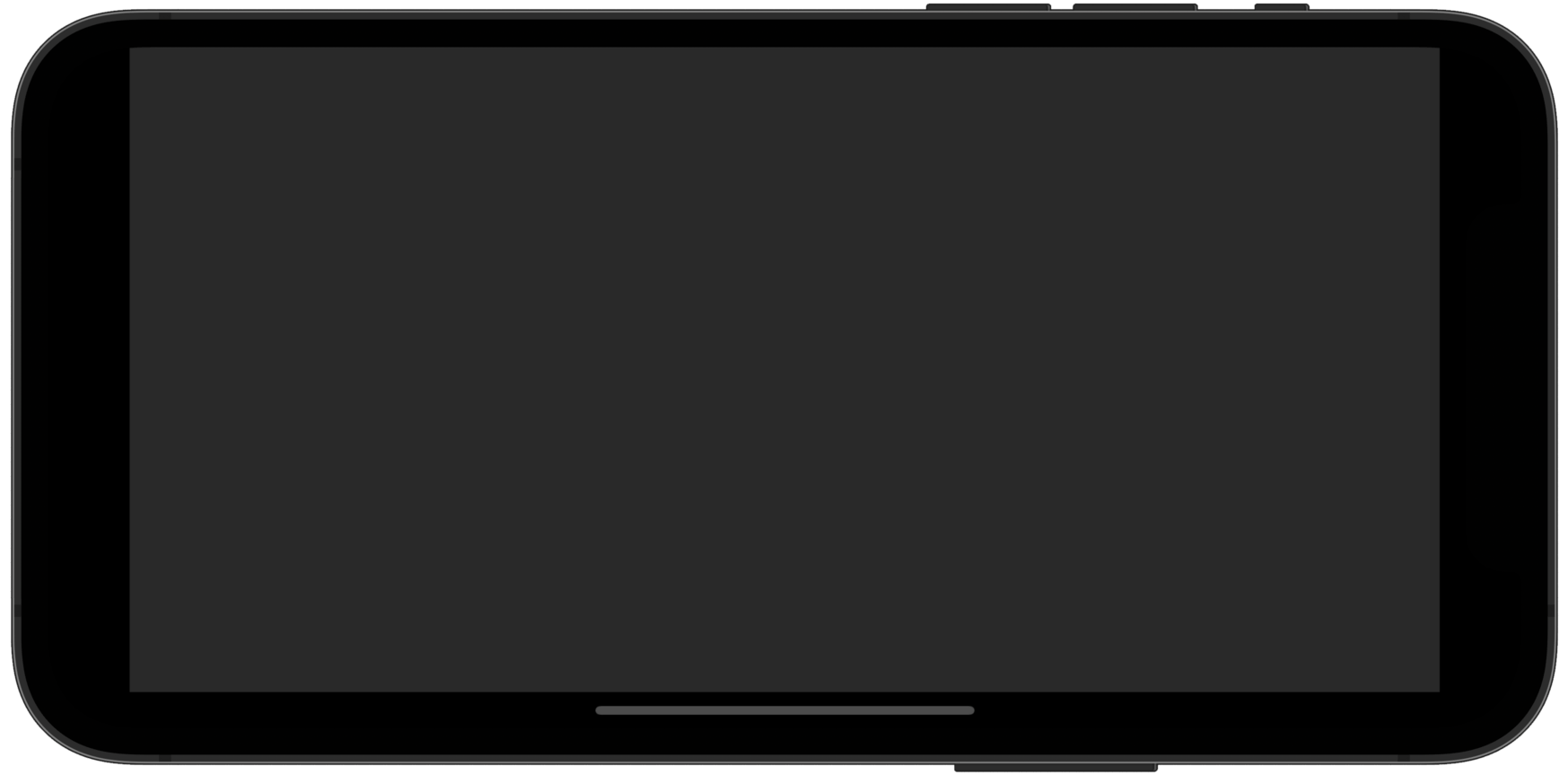
Because SwiftUI has no idea how big the downloaded image is going to be, by default AsyncImage
has a flexible width and height while it's loading. As a result, unless you specify otherwise it will take up a lot of space in your UI while the image loads, then jump to the correct size as soon as the image is loaded.
Although you can attach a frame to your image, it will only affect the placeholder by default – if your finished image arrives at a different size, your UI will have to adapt to fit it.
A better solution is to add functions to control how the resulting image is shown and what kind of placeholder you want. For example, this fetches our image and makes it resizable, but while it's loading uses a red placeholder color:
AsyncImage(url: URL(string: "https://hws.dev/paul.jpg")) { image in
image.resizable()
} placeholder: {
Color.red
}.frame(width: 128, height: 128)
.clipShape(RoundedRectangle(cornerRadius: 25))
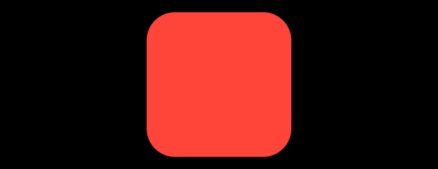
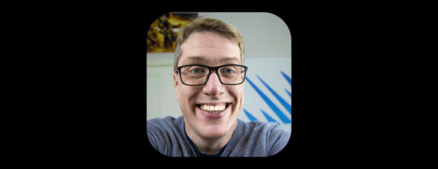
Because both the resulting image and the placeholder color are now resizable, the frame()
modifier is able to make sure our AsyncImage
stays at the correct size the entire time. Before you ask: no, there is no resizable()
modifier available directly on AsyncImage
.
Note
By default the image is assumed to have a scale of 1, meaning designed for non-retina screens. However, you can also control the scale with a second parameter if you already know the correct scale:
AsyncImage(url: URL(string: "https://hws.dev/paul.jpg"), scale: 2)
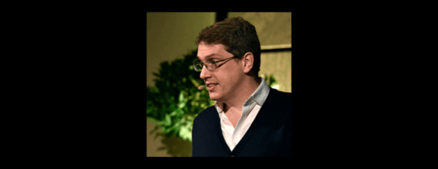
For full control over your AsyncImage
, you should use a single-closure variant of AsyncImage
that handles the loading phase. This approach gives you complete control over the image loading process, allowing you to show one thing when the image is loaded, another thing if the load failed, and of course the image itself when it succeeded.
This can be .empty
because loading hasn't completed yet, .failure
if the image load failed, success
with the image ready if it worked, and an unknown default case in case Apple add more options in the future.
For example, this shows a spinner, a placeholder error picture, or the actually loaded picture depending on how things went:
struct ContentView: View {
var body: some View {
AsyncImage(url: URL(string: "https://hws.dev/paul.jpg")) { phase in
switch phase {
case .failure:
Image(systemName: "photo")
.font(.largeTitle)
case .success(let image):
image.resizable()
default: ProgressView()
}
}
.frame(width: 256, height: 256)
.clipShape(RoundedRectangle(cornerRadius: 25))
}
}