How to lazy load views using LazyVStack and LazyHStack
How to lazy load views using LazyVStack and LazyHStack 관련
Updated for Xcode 15
By default, SwiftUI's VStack
and HStack
load all their contents up front, which is likely to be slow if you use them inside a scroll view. If you want to load content lazily – i.e., only when it scrolls into view, you should use LazyVStack
and LazyHStack
as appropriate.
For example, this will display 1000 text views lined up vertically, making sure each one is created only as it's scrolled into view:
struct ContentView: View {
var body: some View {
ScrollView {
LazyVStack {
ForEach(1...100, id: \.self) { value in
Text("Row \(value)")
}
}
}
.frame(height: 300)
}
}
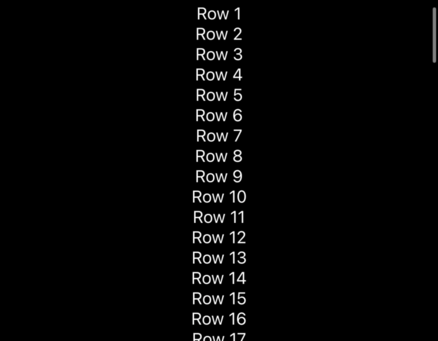
Warning
These lazy stacks automatically have a flexible preferred width, so they will take up free space in a way that regular stacks do not. To see the difference, try the code above and you'll find you can drag around using the whitespace around the text, but if you switch to a regular VStack
you'll see you need to scroll using the text itself.
When using lazy stacks, SwiftUI will automatically create a view when it gets shown for the first time. After that the view will remain in memory, so be careful how much you show.
If you want to see how the lazy loading works in practice, try this example in an iOS app:
struct SampleRow: View {
let id: Int
var body: some View {
Text("Row \(id)")
}
init(id: Int) {
print("Loading row \(id)")
self.id = id
}
}
struct ContentView: View {
var body: some View {
ScrollView {
LazyVStack {
ForEach(1...100, id: \.self, content: SampleRow.init)
}
}
.frame(height: 300)
}
}
If you run that in Xcode, you'll see the “Loading row…” messages bring printed out in the console as you scroll around.