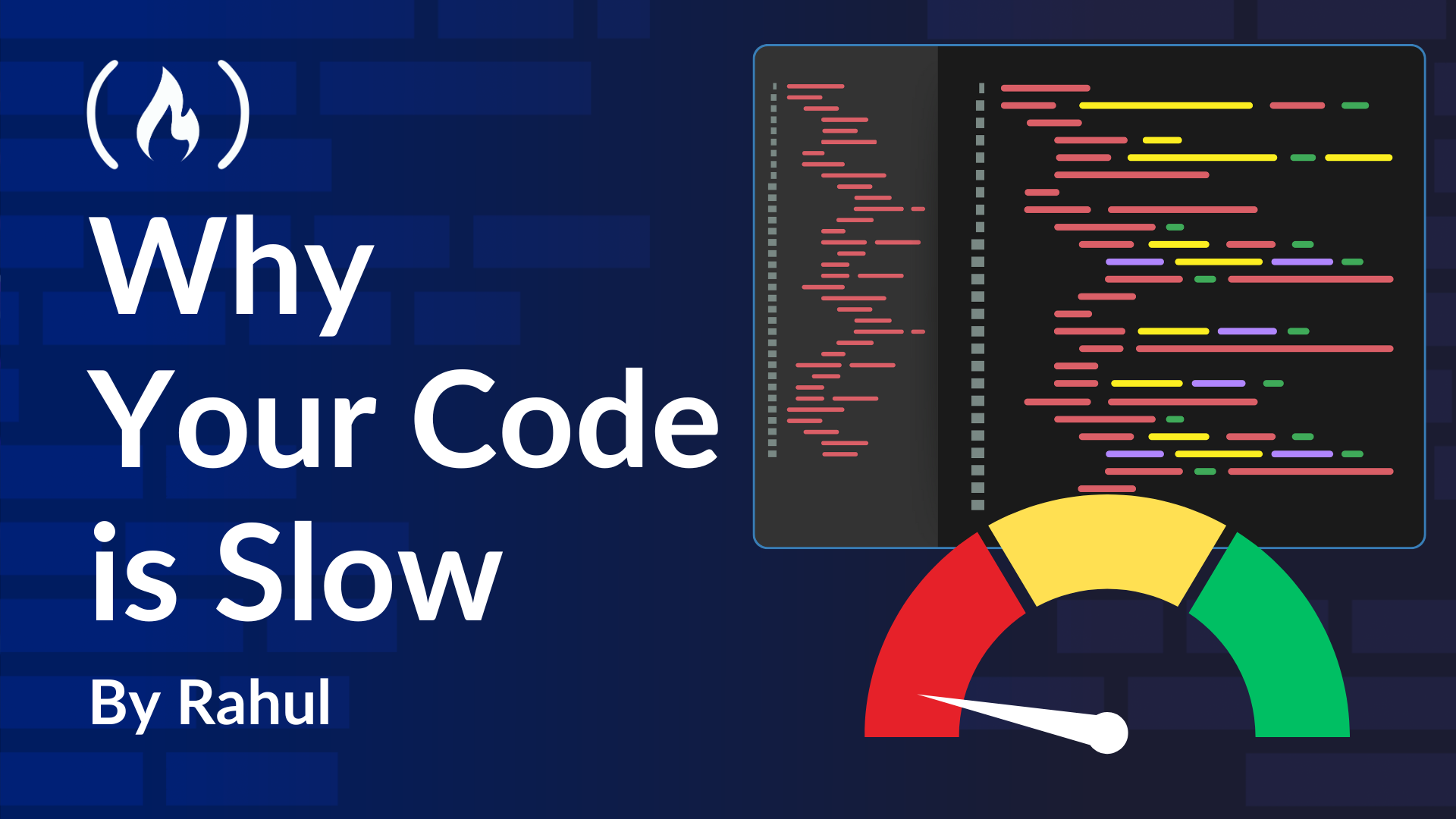
How Do Pro Developers Write Faster Code?
How Do Pro Developers Write Faster Code? 관련
Most beginners think "fast code" just means writing cleaner syntax or using a different framework. But in reality, performance isn't just about what language or framework you use—it's about how you think.
Pro developers don’t just write code. They measure, test, and optimize it**.** Here’s how they do it.
1. They Profile Their Code Instead of Guessing
🔥 Beginners: “This function feels slow… maybe I should rewrite it?”
💡 Pros: “Let’s profile it and see what’s actually slow.”
Instead of randomly rewriting code, pro developers measure first using profiling tools.
Example
In Python, you can use cProfile
to analyze where your code is spending the most time:
pythonCopyEditimport cProfile
def slow_function():
total = 0
for i in range(10**6):
total += i
return total
cProfile.run('slow_function()')
👀 What this tells you:
- Which function takes the longest
- How many times is a function being called
- Where is the actual bottleneck
✅ Takeaway: Before optimizing, always profile your code. You can’t fix what you don’t measure.
Other useful tools:
- Python:
cProfile
,line_profiler
- JavaScript: Chrome DevTools Performance Tab
- Java: JProfiler
- General:
perf
,Valgrind
2. They Avoid Premature Optimization
🔥 Beginners: “I’ll spend hours optimizing this loop before testing it.”
💡 Pros: “I’ll make it work first, then optimize only what matters.”
Donald Knuth famously said, "Premature optimization is the root of all evil." Many beginners waste time optimizing things that aren’t actually slow.
Example
A beginner might spend hours optimizing a loop that runs in 0.001 seconds, while the real slowdown is an extra database query that takes 500ms.
✅ Takeaway:
- First, make your code work.
- Then, profile and optimize only what’s slow.
3. They Pick the Right Data Structures (Not Just What’s Familiar)
🔥 Beginners: “I’ll just use a list.”
💡 Pros: “Which data structure is optimal for this task?”
Most slowdowns happen because of bad data structure choices. Pro developers pick the right tool instead of just going with the default.
Example: Fast lookups
❌ Slow (List - O(n))
pythonCopyEditusers = ["alice", "bob", "charlie"]
if "bob" in users: # Searches the entire list
print("Found")
✅ Fast (Set - O(1))
pythonCopyEditusers = {"alice", "bob", "charlie"}
if "bob" in users: # Uses a hash table for instant lookup
print("Found")
✅ Takeaway: When performance matters, choose the right data structure, not just the most familiar one.
4. They Automate Performance Checks
🔥 Beginners: “I’ll check for performance issues when I feel like it.”
💡 Pros: “I’ll use tools to automatically catch performance bottlenecks.”
Instead of manually looking for slow code, pro developers rely on automated tools that flag inefficiencies.
Example
- Python:
py-spy
(lightweight sampling profiler) - JavaScript: Chrome DevTools Performance Monitoring
- Java: JMH (Java Microbenchmark Harness)
- AI-assisted code reviews: There are tools like CodeAnt that analyze and auto fix your code automatically when you push on GitHub(or anywhere) and suggest performance improvements.
✅ Takeaway: Set up automated checks so you catch performance issues early—before they hit production.
5. They Think About Performance From Day One
🔥 Beginners: “I’ll optimize later.”
💡 Pros: “I’ll write efficient code from the start.”
While premature optimization is bad, writing slow code from the start is worse. Pro developers avoid common pitfalls before they become real problems.
Example: Writing efficient loops from the start
❌ Slow (Unnecessary .append()
)
pythonCopyEditresult = []
for i in range(10**6):
result.append(i * 2) # This is slow
✅ Fast (List Comprehension - Optimized from the Start)
pythonCopyEditresult = [i * 2 for i in range(10**6)] # Faster, more efficient
✅ Takeaway: Small choices add up. Think about performance as you write, rather than fixing it later.