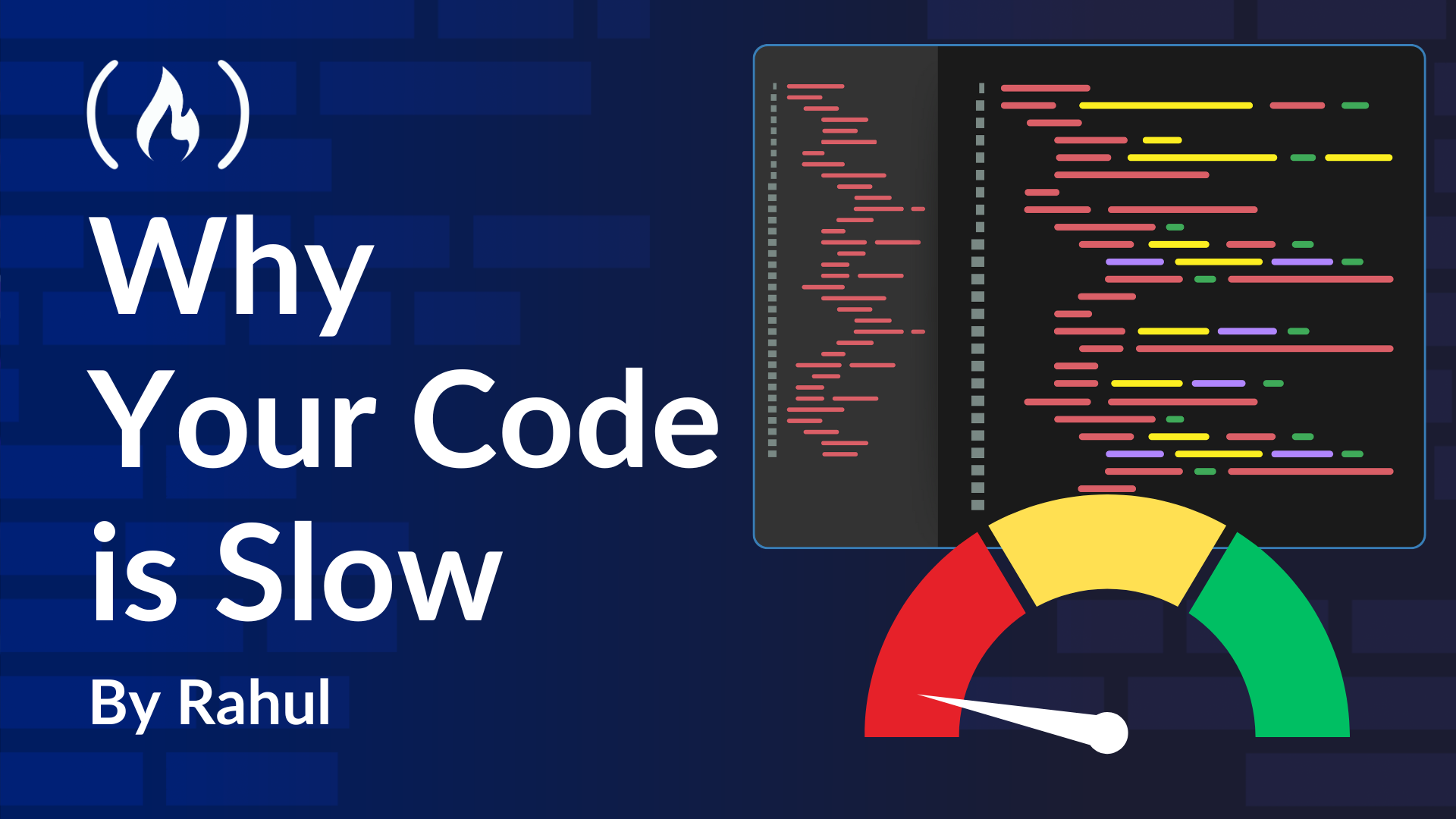
Mistake #2: Using the Wrong Loops (When There’s a Faster Alternative)
Mistake #2: Using the Wrong Loops (When There’s a Faster Alternative) 관련
Why This is a Problem
Loops are one of the first things you learn in programming, and for loops feel natural—they give you control, they’re easy to understand, and they work everywhere. That’s why beginners tend to reach for them automatically.
But just because something works doesn’t mean it’s the best way. In Python, for loops can be slow—especially when there’s a built-in alternative that does the same job faster and more efficiently.
This isn’t just a Python thing. Most programming languages have optimized ways to handle loops under the hood—whether it's vectorized operations in NumPy, functional programming in JavaScript, or stream processing in Java. Knowing when to use them is key to writing fast, clean code.
Example
Let’s say you want to square a list of numbers. A beginner might write this:
numbers = [1, 2, 3, 4, 5]
squared = []
for num in numbers:
squared.append(num ** 2)
Looks fine, right? But there are two inefficiencies here:
- You're manually looping when Python has a better, built-in way to handle this.
- You're making repeated
.append()
calls, which add unnecessary overhead.
In small cases, you won’t notice a difference. But when processing large datasets, these inefficiencies add up fast.
The Better, Faster Way
Python has built-in optimizations that make loops run faster. One of them is list comprehensions, which are optimized in C and run significantly faster than manual loops. Here’s how you can rewrite the example:
pythonCopyEdit# Much faster and cleaner
squared = [num ** 2 for num in numbers]
Why this is better:
- It’s faster. List comprehensions run in C under the hood, meaning they don’t have the overhead of Python function calls like
.append()
. - It eliminates extra work. Instead of growing a list dynamically (which requires resizing in memory), Python pre-allocates space for the entire list. This makes the operation much more efficient.
- It’s more readable. The intent is clear: "I’m creating a list by squaring each number"—no need to scan through multiple lines of code.
- It’s less error-prone. Since everything happens in a single expression, there’s less chance of accidentally modifying the list incorrectly (for example, forgetting to
.append()
).
When to Use For Loops vs. List Comprehensions
For loops still have their place. Use them when:
- You need complex logic inside the loop (for example, multiple operations per iteration).
- You need to modify existing data in place rather than create a new list.
- The operation involves side effects, like logging, file writing, or network requests.
Otherwise, list comprehensions should be your default choice for simple transformations. They’re faster, cleaner, and make your Python code more efficient.