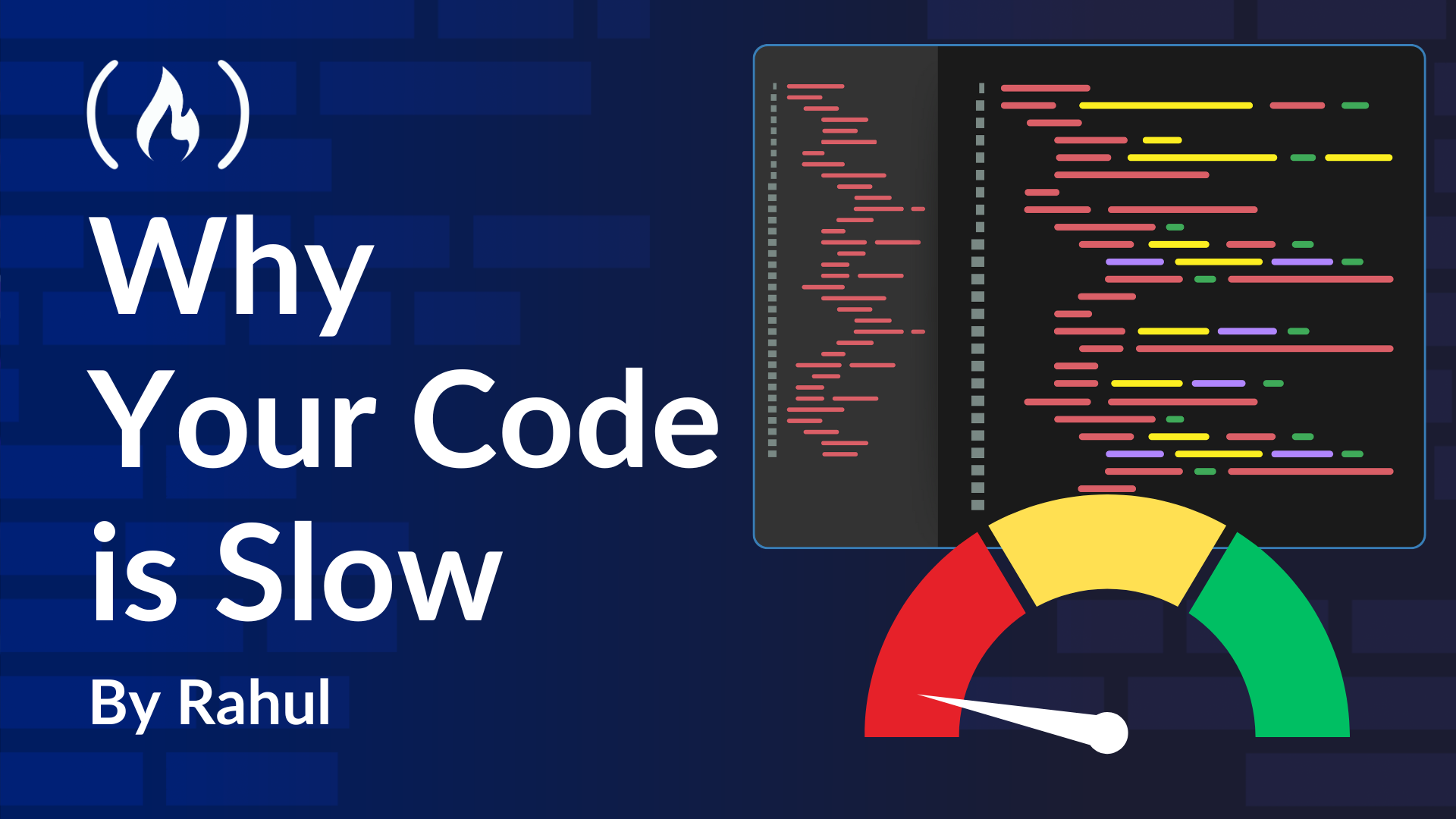
Mistake #7: The Copy-Paste Trap
Mistake #7: The Copy-Paste Trap êŽë š
Youâd never download 10 copies of the same movie. But in code? Youâre probably cloning data all the time without realizing it. Hereâs how invisible copies turn your app into a bloated, slow messâand how to fix it.
Problem 1: The Ghost Copies in âHarmlessâ Operations
âI sliced a listâitâs just a reference, right?â
In many languages, slicing creates a full copy of the data. Do this with large datasets, and youâre silently doubling memory usage and CPU work.
Python Example
# A 1GB list of data
big_data = [ ... ] # 1,000,000 elements
# Accidentally cloning the entire list
snippet = big_data[:1000] # Creates a copy (harmless, right?)
# Better: Use a view (if possible)
import numpy as np
big_array = np.array(big_data)
snippet = big_array[:1000] # A view, not a copy (0MB added)
Why this hurts
- Copying 1GB â 2GB of RAM used.
- If this happens in a loop, your program could crash with
MemoryError
.
The Fix:
- Use memory views (
numpy
,memoryview
in Python) or lazy slicing (Pandas.iloc
). - In JavaScript,
slice()
copies arraysâreplace withTypedArray.subarray
for buffers.
Problem 2: The Hidden Cost of âFunctionalâ Code
âIâll chain array methods for clean, readable code!â
Every map
, filter
, or slice
creates a new array. Chain three operations? Youâve cloned your data three times.
JavaScript Example:
// A 10,000-element array
const data = [ ... ];
// Slow: Creates 3 copies (original â filtered â mapped â sliced)
const result = data
.filter(x => x.active)
.map(x => x.value * 2)
.slice(0, 100);
// Faster: Do it in one pass
const result = [];
for (let i = 0; i < data.length; i++) {
if (data[i].active) {
result.push(data[i].value * 2);
if (result.length === 100) break;
}
}
Why this hurts:
- 10,000 elements â 30,000 operations + 3x memory.
- Functional programming is elegant but can be expensive.
The Fix:
- Use generators (Python
yield
, JSfunction*
) for lazy processing. - Replace method chains with single-pass loops in hot paths.
Problem 3: The âIâll Just Modify a Copyâ Mistake
âI need to tweak this object. Iâll duplicate it to avoid side effects.â
Deep cloning complex objects (especially in loops) is like photocopying a dictionary every time you edit a word.
Python Example:
import copy
config = {"theme": "dark", "settings": { ... }} # Nested data
# Slow: Deep-copying before every edit
for user in users:
user_config = copy.deepcopy(config) # Copies entire nested structure
user_config["theme"] = user.preference
# ...
# Faster: Reuse the base config, overlay changes
for user in users:
user_config = {"theme": user.preference, **config} # Shallow merge
# ...
Why this hurts:
deepcopy
is 10-100x slower than shallow copies.- Multiplied by 1,000 users, youâre wasting minutes.
The Fix:
- Use immutable patterns: Create new objects by merging instead of cloning.
- For big data, use structural sharing (libraries like
immutables
in Python).
How to Escape the Copy-Paste hell?
- Ask: âDo I need a copy?â: 90% of the time, you donât. Use views, generators, or in-place edits.
- Profile memory usage: Tools like
memory_profiler
(Python) or Chrome DevTools (JS) show copy overhead. - Learn your languageâs quirks:
- Python: Slicing lists copies, slicing NumPy arrays doesnât.
- JavaScript:
[...array]
clones,array.subarray
(TypedArray) doesnât.