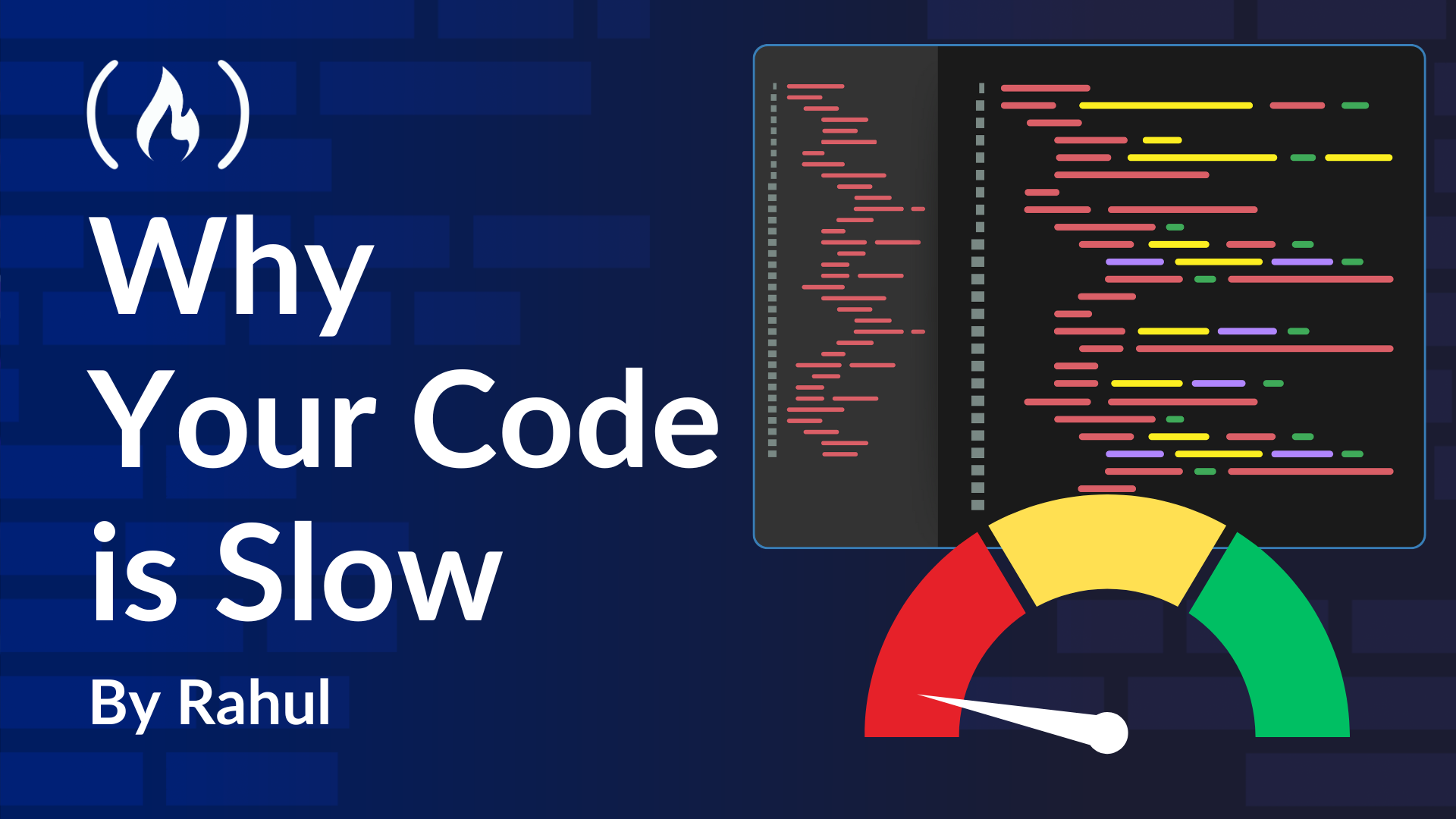
Mistake #5: Memory Fragmentation
Mistake #5: Memory Fragmentation êŽë š
Youâve optimized your algorithms. Youâve nailed Big O. Yet your app still crashes with âout of memoryâ errors or slows to a crawl over time. The culprit? Memory fragmentationâa ghost in the machine that most developers ignore until itâs too late.
Whatâs Happening Under the Hood
When your code allocates and frees memory blocks of varying sizes, it leaves behind a patchwork of free and used spaces. Over time, this creates a Swiss cheese effect in your RAM: plenty of total free memory, but no contiguous blocks for new allocations.
Example
Imagine a C++ server that handles requests by allocating buffers of random sizes:
void process_request() {
// Allocate a buffer of random size between 1â1024 bytes
char* buffer = new char[rand() % 1024 + 1];
// ... process ...
delete[] buffer;
}
After millions of requests, your memory looks like this:
[USED][FREE][USED][FREE][USED][FREE]...
Now, when you try to allocate a 2KB buffer, it failsânot because thereâs no space, but because no single free block is large enough.
How to Fix it:
Use a memory pool to allocate fixed-size blocks:
class MemoryPool {
public:
MemoryPool(size_t block_size) : block_size_(block_size) {}
void* allocate() { /* get a pre-allocated block */ }
void deallocate(void* ptr) { /* return block to pool */ }
};
// All requests use buffers of fixed size (1024 bytes)
MemoryPool pool(1024);
void process_request() {
char* buffer = static_cast<char*>(pool.allocate());
// ... process ...
pool.deallocate(buffer);
}
By standardizing block sizes, you eliminate fragmentation.
The Autoboxing Trap (Java, C#, and so on)
Whatâs Happening?
In languages that mix primitives (like int
, float
) and objects (like Integer
, Double
), converting a primitive to its object wrapper is called autoboxing. It feels harmless, but in hot loops, itâs a performance disaster.
Example
// Slow: Creates 1,000,000 Integer objects (and garbage!)
List<Integer> list = new ArrayList<>();
for (int i = 0; i < 1_000_000; i++) {
list.add(i); // Autoboxing 'i' to Integer
}
Why this hurts performance:
- Memory overhead: Each
Integer
object adds 16â24 bytes of extra memory (object headers, pointers). With 1,000,000 numbers, thatâs an extra 16â24MB wasted just on overhead. - Garbage collection (GC) pressure: Since objects are allocated on the heap, the GC constantly cleans up old
Integer
objects, leading to latency spikes. - CPU cache inefficiency: Primitives like
int
are tightly packed in memory, butInteger
objects are scattered across the heap with extra indirection, wrecking cache locality.
The Fix: Use Primitive Collections
To avoid autoboxing, use data structures that store raw primitives instead of objects. In Java, Eclipse Collections provides primitive-friendly lists like IntList
that store raw int
values directly.
Example: The Faster Version (Primitive Collections)
// Import primitive-friendly collection
import org.eclipse.collections.api.list.primitive.IntList;
import org.eclipse.collections.impl.list.mutable.primitive.IntArrayList;
// Use IntArrayList to store raw ints
IntList list = new IntArrayList();
for (int i = 0; i < 1_000_000; i++) {
list.add(i); // No autoboxing! Stores raw 'int'
}
How this fix works:
- Stores raw
int
values instead ofInteger
objects, eliminating memory overhead. - Avoids heap allocations, so the garbage collector doesnât get involved.
- Keeps numbers tightly packed in memory, improving CPU cache efficiency.
The Fix for C#
In C#, you can avoid unnecessary heap allocations by using struct
s and Span<T>
, which keep data on the stack or in contiguous memory rather than the heap.
// Span<T> avoids heap allocations
Span<int> numbers = stackalloc int[1_000_000];
for (int i = 0; i < numbers.Length; i++) {
numbers[i] = i; // No boxing, no heap allocation
}
No object wrappers. No GC pressure. Just performance.