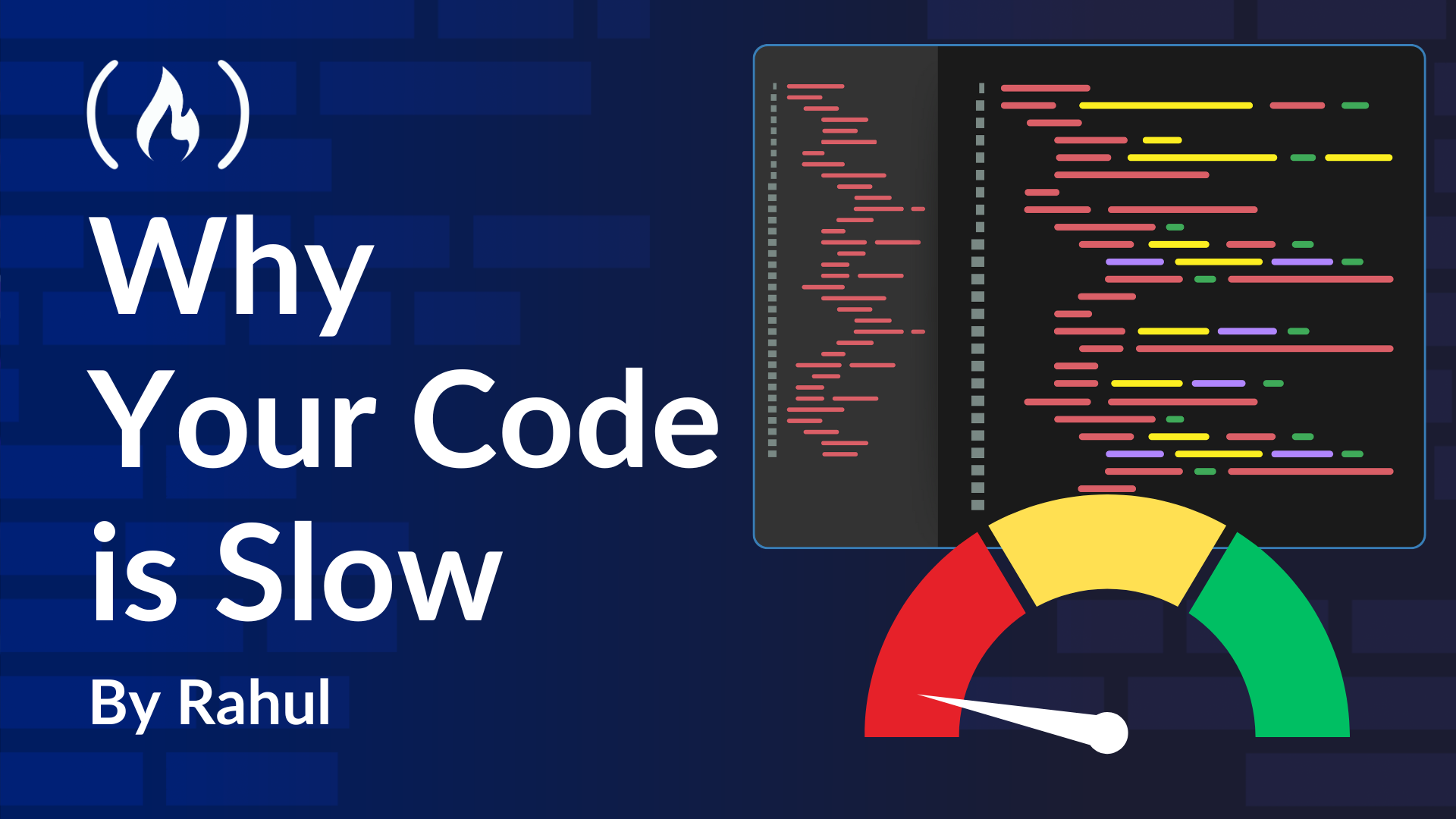
Mistake #1: Logging Everything in Production (Without Realizing It)
Mistake #1: Logging Everything in Production (Without Realizing It) 관련
Mistake #1: Logging Everything in Production (Without Realizing It)
Logging is supposed to help you understand what’s happening in your code—but if you’re logging everything, you’re actually slowing it down. A common beginner mistake is leaving print()
statements everywhere or enabling verbose logging even in production, where performance matters most.
Instead of logging only what’s useful, they log every function call, every input, every output, and sometimes even entire request bodies or database queries. This might seem harmless, but in a live application handling thousands of operations per second, excessive logging can cause major slowdowns.
Why This is a Problem
Logging isn’t free. Every log message, whether printed to the console or written to a file, adds extra processing time. If logging is done synchronously (which it often is by default), your application can pause execution while waiting for the log to be recorded.
It also wastes disk space. If every request gets logged in detail, log files can grow rapidly, eating up storage and making it harder to find useful information when debugging.
Here’s an example:
def process_data(data):
print(f"Processing data: {data}") # Logging every input
result = data * 2
print(f"Result: {result}") # Logging every result
return result
If this function is running inside a loop handling 10,000+ operations, those print statements are slowing things down massively.
How to Fix It
Instead of logging everything, focus on logging only what actually matters. Good logging helps you diagnose real issues without cluttering your logs or slowing down your app.
For example, let’s say you're processing user transactions. You don’t need to log every step of the calculation, but logging when a transaction starts, succeeds, or fails is valuable.
# ✅ Bad logging
logging.info(f"Received input: {data}")
logging.info(f"Processing transaction for user {user_id}")
logging.info(f"Transaction intermediate step 1 result: {some_var}")
logging.info(f"Transaction intermediate step 2 result: {another_var}")
logging.info(f"Transaction completed: {final_result}")
# ✅ Better logging
logging.info(f"Processing transaction for user {user_id}")
logging.info(f"Transaction successful. Amount: ${amount}")
Next, make sure debugging logs are turned off in production. Debug logs (logging.debug()
) are great while developing because they show detailed information, but they shouldn’t be running on live servers.
You can control this by setting the logging level to INFO
or higher:
import logging
logging.basicConfig(level=logging.INFO) # Only logs INFO, WARNING, ERROR, CRITICAL messages
def process_data(data):
logging.debug(f"Processing data: {data}") # Won't show up in production
return data * 2
Finally, for high-performance applications, consider using asynchronous logging. By default, logging operations can block execution, meaning your program waits until the log message is written before continuing. This can be a bottleneck, especially if you're logging to a file or a remote logging service.
Asynchronous logging solves this by handling logs in the background. Here’s how you can set it up with Python’s QueueHandler
:
import logging
import logging.handlers
import queue
log_queue = queue.Queue()
queue_handler = logging.handlers.QueueHandler(log_queue)
logger = logging.getLogger()
logger.addHandler(queue_handler)
logger.setLevel(logging.INFO)
logger.info("This log is handled asynchronously!")