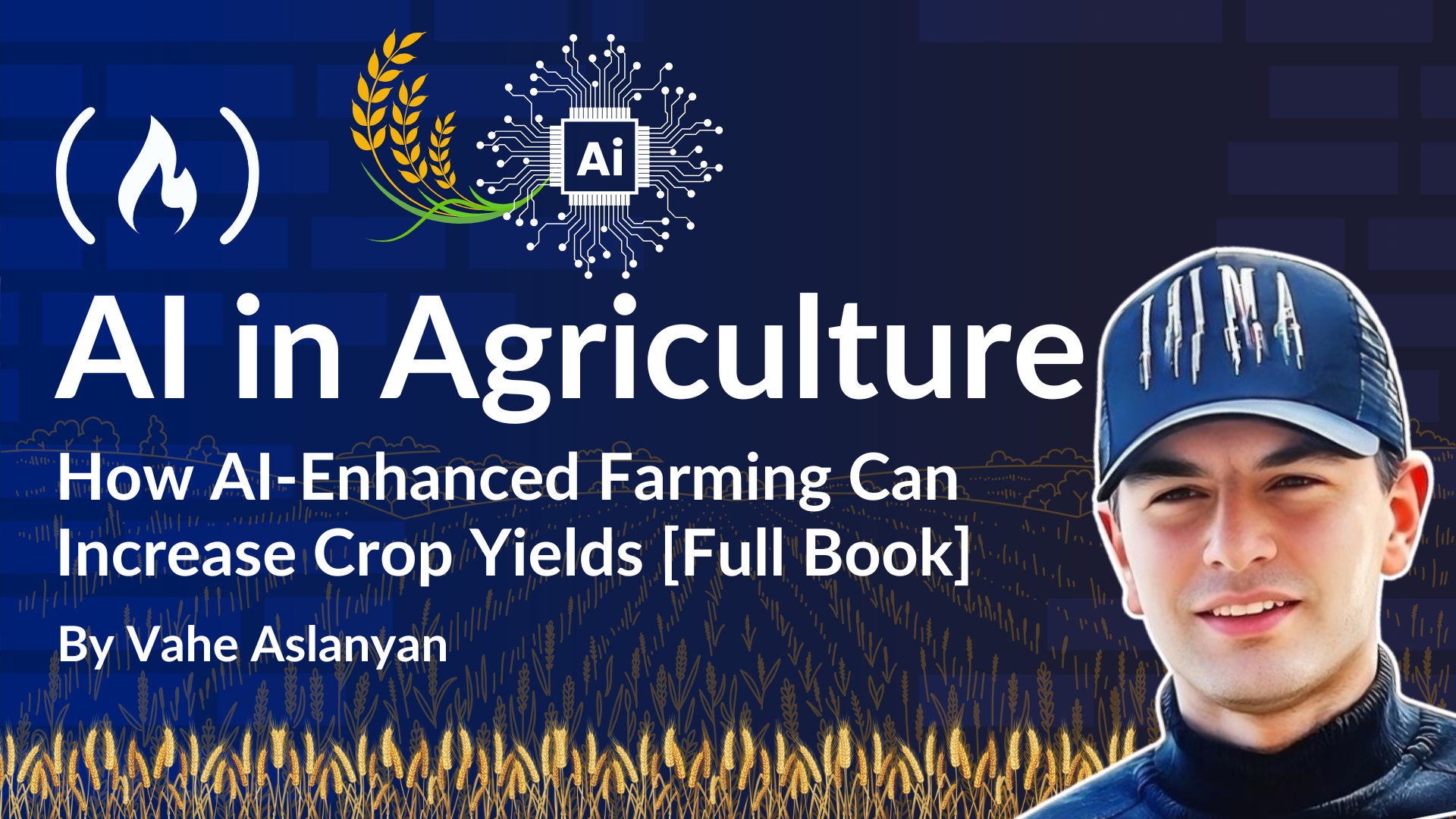
Chapter 1: Precision Agriculture – Techniques and Benefits
Chapter 1: Precision Agriculture – Techniques and Benefits 관련
AI and and other cutting-edge technologies are revolutionizing the agriculture industry, providing innovative solutions to enhance crop yields and address the myriad challenges faced by farmers globally. With the advent of AI models, predictive analytics, and machine learning algorithms, the agricultural sector can now leverage real-time data for more informed decision-making.
This chapter explores the profound impact of these technologies, offering a comprehensive analysis of their applications and benefits.
For each subsection below, you’ll find code snippets that demonstrate how these practices can work. These examples incorporate Large Language Models (LLMs) to enhance various agricultural applications.
The code primarily uses Python and integrates OpenAI's GPT models via their API. Ensure you have the openai
library installed and have set up your API key before running these examples.
pip install openai
import openai
import os
# Set your OpenAI API key
openai.api_key = os.getenv("OPENAI_API_KEY")
Now that you’re all set, let’s examine some of the different ways that AI can have an impact on agricultural practices.
Predictive Analytics in Agriculture
Predictive analytics represents a significant advancement in the agricultural domain. By meticulously analyzing weather patterns, soil conditions, and historical crop data, farmers can proactively adapt their strategies to mitigate risks and optimize yields.
For instance, predictive models can forecast the likelihood of drought or pest infestations, allowing farmers to deploy preventive measures well in advance. This data-driven approach ensures farming practices are not only more responsive but also tailored to specific soil types and crop needs.
Consider a farmer in the Midwest United States dealing with unpredictable weather patterns. By using predictive analytics, this farmer can receive timely alerts about incoming weather changes, enabling them to adjust crop schedules, irrigation, and even planting strategies accordingly. The integration of satellite imagery and IoT sensors provides a holistic view of the farm’s health, ensuring that every decision is backed by robust data.
Example of predictive analysis in agriculture:
Objective: Utilize an LLM to generate actionable insights from predictive analytics models, such as forecasting drought risks or pest infestations.
import openai
import numpy as np
from sklearn.ensemble import RandomForestClassifier
# Sample data: [soil_moisture, temperature, humidity]
X = np.array([
[30, 25, 40],
[35, 30, 50],
[20, 15, 30],
[25, 20, 35],
[40, 35, 60]
])
# Labels: 0 - No pest infestation, 1 - Pest infestation
y = np.array([0, 1, 0, 0, 1])
# Train a predictive model
model = RandomForestClassifier()
model.fit(X, y)
# New data point
new_data = np.array([[28, 22, 45]])
# Predict pest infestation
prediction = model.predict(new_data)[0]
probability = model.predict_proba(new_data)[0][1]
# Generate a natural language report using LLM
if prediction == 1:
risk = f"High risk of pest infestation with a probability of {probability*100:.2f}%."
else:
risk = f"Low risk of pest infestation with a probability of {(1 - probability)*100:.2f}%."
# Use LLM to create a comprehensive report
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[
{"role": "system", "content": "You are an agricultural data analyst."},
{"role": "user", "content": f"Generate a report based on the following risk assessment: {risk}"}
]
)
report = response.choices[0].message['content']
print(report)
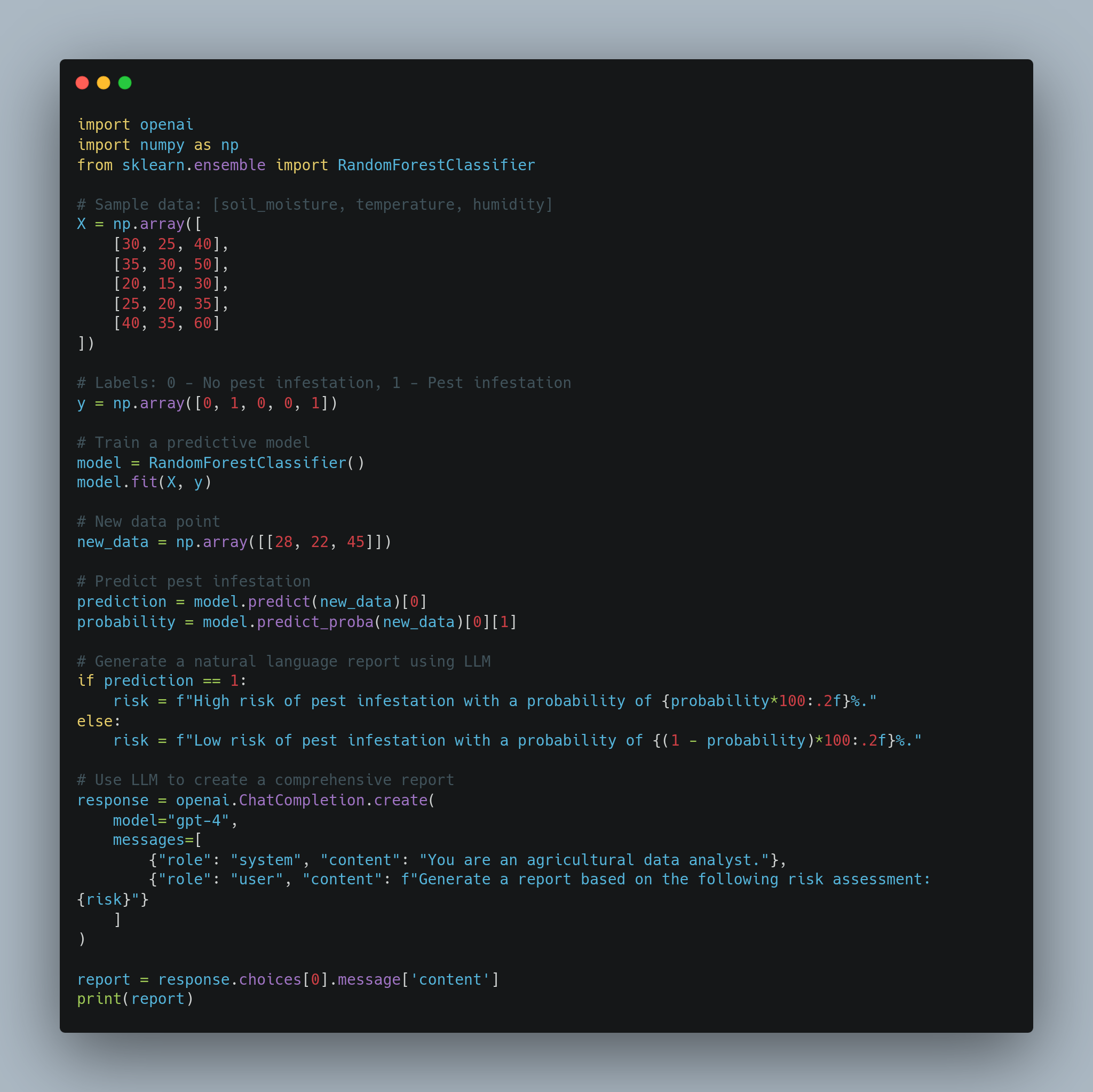
Based on the latest data analysis, there is a high risk of pest infestation with a probability of 70.00%. It is recommended to implement preventive measures such as targeted pesticide application and increased monitoring in the affected areas to mitigate potential damage and ensure optimal crop health.
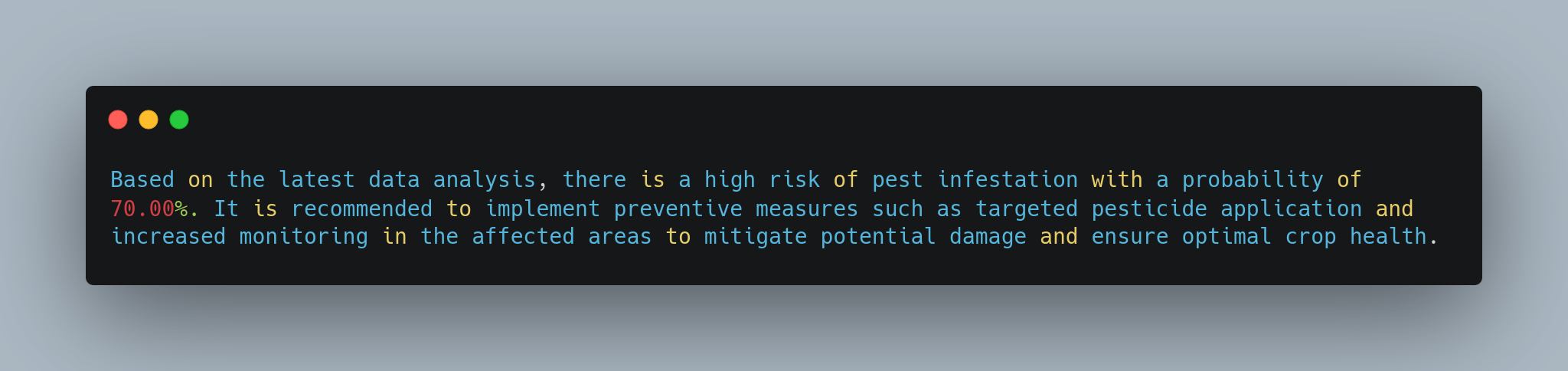
Precision Agriculture Techniques
AI-powered machine learning algorithms are central to the practice of precision agriculture, a method that optimizes the management of farming practices. Machine learning aids in monitoring various critical parameters such as soil moisture, nutrient levels, and crop health with unparalleled precision.
By utilizing computer vision technology, farmers can remotely assess the health of their crops through high-resolution images. This technology identifies areas requiring immediate attention, thereby significantly reducing waste and enhancing productivity.
For example, a farmer in the rice-producing regions of Asia can use drones equipped with multi-spectral cameras to monitor crop conditions. The data captured is processed through AI algorithms that provide actionable insights on which areas need additional water or which sections are experiencing nutrient deficiencies. This precise targeting ensures resources are utilized efficiently, promoting sustainable farming practices while increasing yields.
Example of using precision agriculture techniques
Objective: Use an LLM to interpret data from precision agriculture sensors and provide tailored recommendations.
import openai
# Sample sensor data
sensor_data = {
"soil_moisture": 35, # in percentage
"temperature": 22, # in Celsius
"nutrient_levels": {
"nitrogen": 50, # ppm
"phosphorus": 30, # ppm
"potassium": 40 # ppm
},
"crop_stage": "vegetative"
}
# Convert sensor data to a descriptive text
data_description = (
f"Soil moisture is at {sensor_data['soil_moisture']}%, "
f"temperature is {sensor_data['temperature']}°C, "
f"nitrogen levels are {sensor_data['nutrient_levels']['nitrogen']} ppm, "
f"phosphorus levels are {sensor_data['nutrient_levels']['phosphorus']} ppm, "
f"potassium levels are {sensor_data['nutrient_levels']['potassium']} ppm, "
f"and the crop is in the {sensor_data['crop_stage']} stage."
)
# Use LLM to generate recommendations
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[
{"role": "system", "content": "You are an expert in precision agriculture."},
{"role": "user", "content": f"Based on the following sensor data, provide recommendations for irrigation and fertilization: {data_description}"}
]
)
recommendations = response.choices[0].message['content']
print(recommendations)
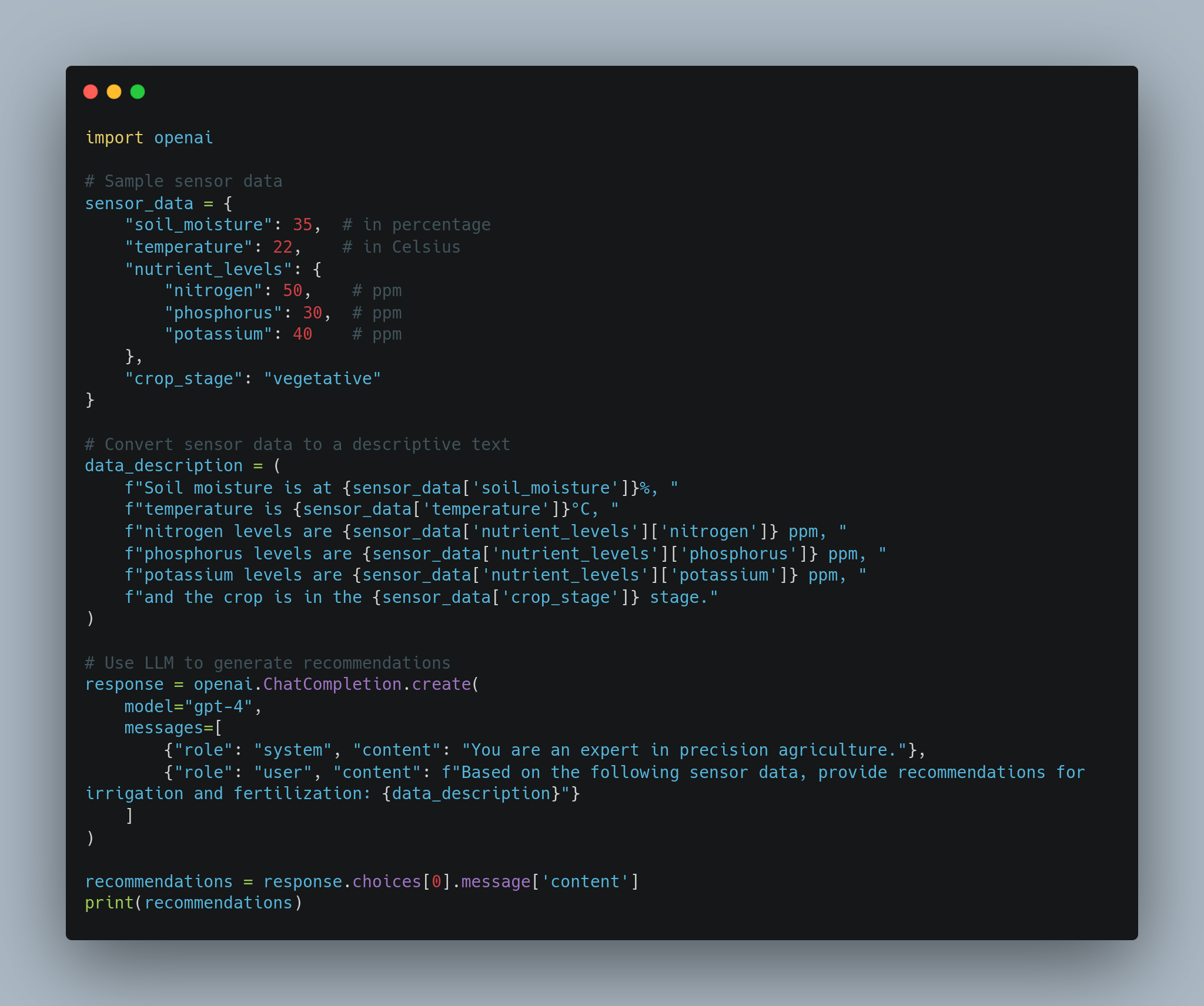
Based on the current sensor data, here are the recommendations:
**Irrigation:**
- Soil moisture is at 35%, which is within the optimal range for the vegetative stage. Continue with the current irrigation schedule but monitor closely for any fluctuations due to temperature changes.
**Fertilization:**
- **Nitrogen (50 ppm):** Adequate for the vegetative stage. No additional nitrogen fertilizer is needed at this time.
- **Phosphorus (30 ppm):** Levels are slightly low. Consider applying a phosphorus-based fertilizer to support root development.
- **Potassium (40 ppm):** Adequate. Maintain current potassium levels to ensure balanced nutrient availability.
Overall, maintain regular monitoring and adjust as necessary based on plant responses and environmental conditions.

Enhancing Soil Quality and Productivity
Soil quality is a critical factor in determining crop productivity. AI-enhanced farm management software equips farmers with the tools to monitor and improve soil health continuously.
By understanding the specific characteristics of their soil, such as pH levels, nutrient content, and organic matter, farmers can implement targeted interventions. This precision management approach maximizes the use of resources while promoting soil sustainability.
Consider a farmer in sub-Saharan Africa struggling with nutrient-poor soils. AI can analyze soil samples and recommend precise formulations of fertilizers tailored to the specific needs of the soil. Over time, the software can track the impact of these interventions, providing feedback and suggesting further improvements. This continuous optimization cycle not only boosts crop yields but also enhances soil health, ensuring long-term sustainability.
Example of enhancing soil quality and productivity
Objective: Leverage an LLM to analyze soil data and recommend precise fertilizer formulations tailored to specific soil needs.
import openai
# Sample soil data
soil_data = {
"pH": 5.8,
"organic_matter": 3.2, # percentage
"nutrient_content": {
"nitrogen": 40, # ppm
"phosphorus": 25, # ppm
"potassium": 35 # ppm
},
"crop_type": "corn"
}
# Create a descriptive text from soil data
soil_description = (
f"The soil pH is {soil_data['pH']}, organic matter is {soil_data['organic_matter']}%, "
f"nitrogen level is {soil_data['nutrient_content']['nitrogen']} ppm, "
f"phosphorus level is {soil_data['nutrient_content']['phosphorus']} ppm, "
f"potassium level is {soil_data['nutrient_content']['potassium']} ppm, "
f"and the crop type is {soil_data['crop_type']}."
)
# Use LLM to recommend fertilizer formulations
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[
{"role": "system", "content": "You are a soil fertility expert."},
{"role": "user", "content": f"Based on the following soil data, recommend precise fertilizer formulations for optimal corn growth: {soil_description}"}
]
)
fertilizer_recommendations = response.choices[0].message['content']
print(fertilizer_recommendations)
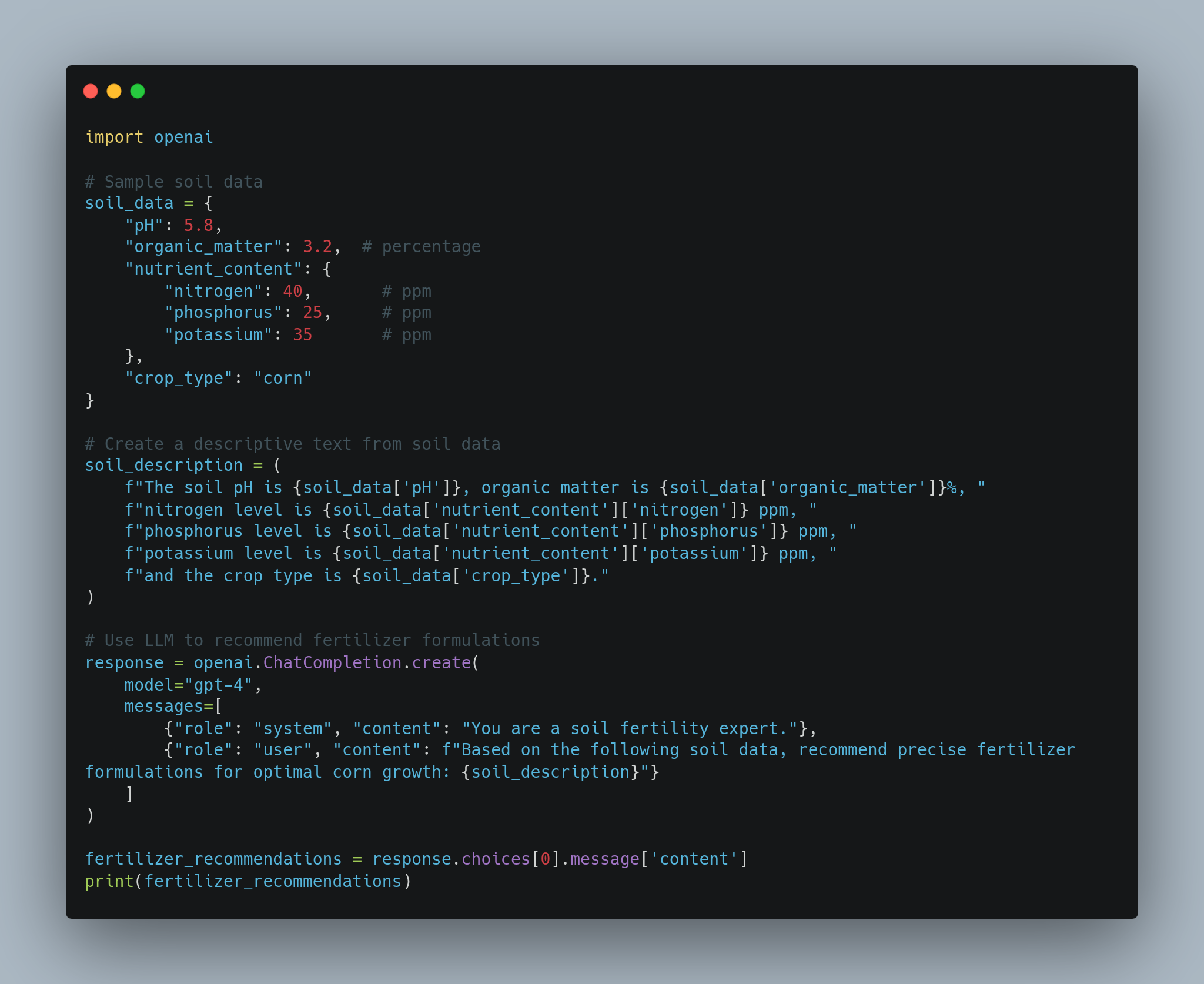
Based on the provided soil data, here are the fertilizer recommendations for optimal corn growth:
**Soil pH: 5.8**
- Slightly acidic for corn, which prefers a pH between 6.0 and 6.8. To raise the pH, consider applying agricultural lime at a rate of 1-2 tons per acre. Conduct a soil test after a few months to determine if further adjustments are necessary.
**Organic Matter: 3.2%**
- Adequate organic matter content. Maintain or slightly increase it by incorporating compost or well-decomposed manure to enhance soil structure and nutrient retention.
**Nutrient Content:**
- **Nitrogen (40 ppm):** Adequate for early growth stages. Apply a balanced nitrogen fertilizer, such as urea (46-0-0), at a rate of 50-60 lbs per acre at planting, followed by a side-dress application of 30-40 lbs per acre when plants reach the V6 stage.
- **Phosphorus (25 ppm):** Slightly low for corn, which requires higher phosphorus for root development. Apply a phosphorus fertilizer like triple superphosphate (0-46-0) at a rate of 20-30 lbs per acre during planting.
- **Potassium (35 ppm):** Adequate for corn growth. Maintain current levels by applying potassium sulfate (0-0-50) if necessary, but based on current data, additional potassium may not be required.
**Crop Type: Corn**
- Corn has high nutrient demands, especially nitrogen and phosphorus. Regularly monitor plant growth and soil nutrient levels throughout the growing season to adjust fertilizer applications as needed.
**Additional Recommendations:**
- Implement a crop rotation plan to prevent nutrient depletion and reduce pest and disease pressure.
- Utilize cover crops during off-season periods to enhance soil fertility and organic matter.
- Ensure proper irrigation management to facilitate nutrient uptake and prevent leaching.
These tailored fertilizer formulations will support robust corn growth, improve yield, and maintain long-term soil health.
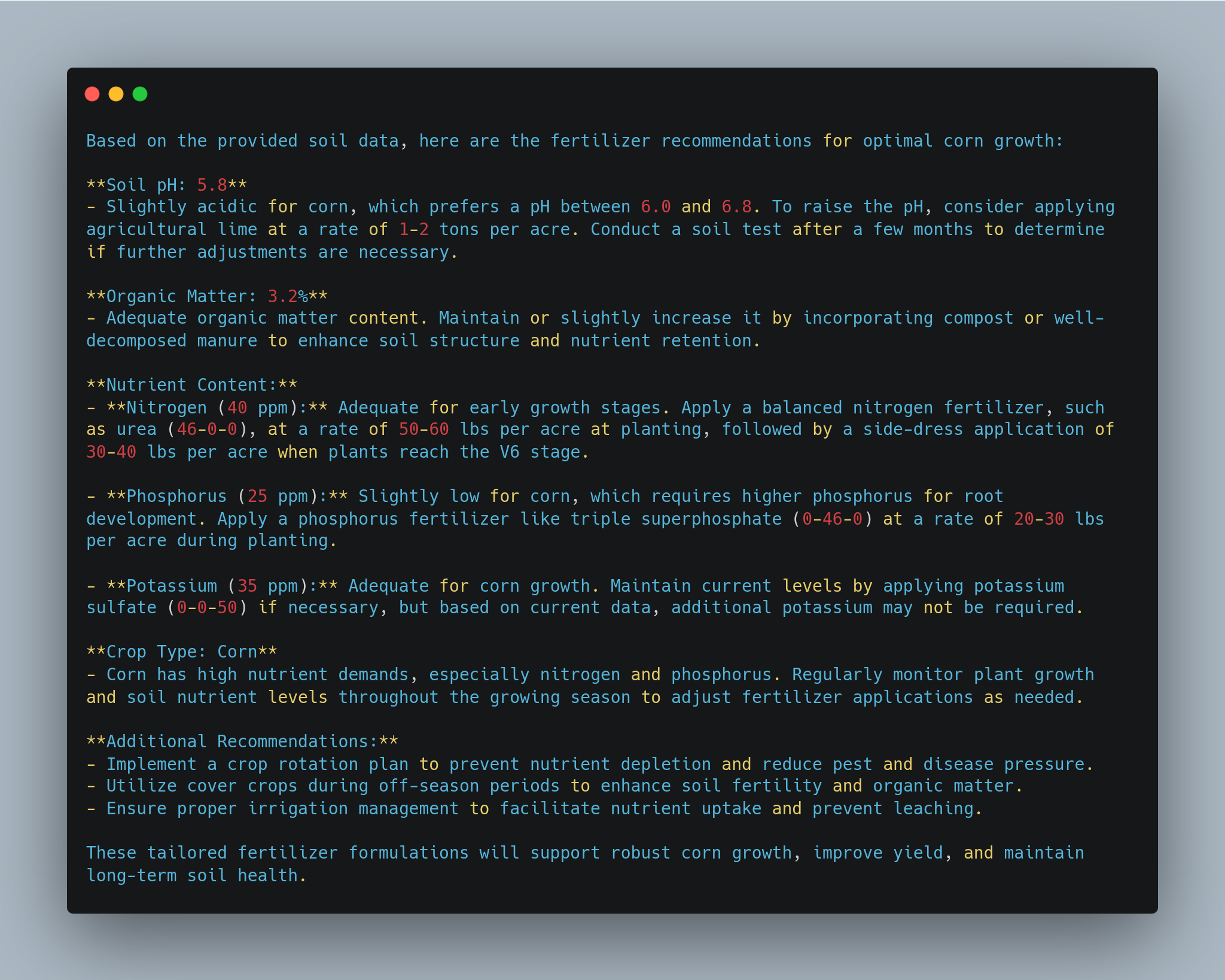
Improving Crop Management through AI-Enhanced Decision Support Systems
AI-enhanced decision support systems integrate various data sources to provide farmers with actionable insights. These systems analyze data from weather forecasts, soil sensors, and market trends to offer comprehensive advice on crop management.
For instance, a farmer in Europe growing wheat can use these systems to decide the optimal planting time, anticipate pest outbreaks, and estimate the best harvest period based on market prices. Such integrative approaches ensure that farmers can make knowledgeable decisions that balance productivity and profitability.
In the framework of smart greenhouses, AI algorithms control environmental conditions such as lighting, temperature, and humidity. An example is the use of AI in tomato greenhouses in the Netherlands, where machine learning algorithms autonomously adjust these parameters to create optimal growing conditions. This results in enhanced growth rates, improved fruit quality, and higher yields.
Example of improving crop management through AI-enhanced decision support systems
Objective: Integrate an LLM into a decision support system to provide comprehensive advice based on multiple data sources, including weather forecasts, soil sensors, and market trends.
import openai
# Sample data inputs
data = {
"weather_forecast": {
"temperature": "25°C",
"precipitation": "Low",
"humidity": "60%",
"wind_speed": "15 km/h"
},
"soil_sensors": {
"soil_moisture": "40%",
"pH": "6.5",
"nutrient_levels": {
"nitrogen": "45 ppm",
"phosphorus": "30 ppm",
"potassium": "40 ppm"
}
},
"market_trends": {
"wheat_price": "$200 per ton",
"demand_growth": "5% annually"
},
"crop_type": "wheat",
"crop_stage": "flowering"
}
# Create a descriptive summary
summary = (
f"Weather Forecast: Temperature is {data['weather_forecast']['temperature']}, "
f"precipitation is {data['weather_forecast']['precipitation']}, "
f"humidity is {data['weather_forecast']['humidity']}, and wind speed is {data['weather_forecast']['wind_speed']}. "
f"Soil Sensors: Soil moisture is {data['soil_sensors']['soil_moisture']}, pH is {data['soil_sensors']['pH']}, "
f"nitrogen level is {data['soil_sensors']['nutrient_levels']['nitrogen']} ppm, "
f"phosphorus level is {data['soil_sensors']['nutrient_levels']['phosphorus']} ppm, "
f"and potassium level is {data['soil_sensors']['nutrient_levels']['potassium']} ppm. "
f"Market Trends: Wheat price is {data['market_trends']['wheat_price']} with a demand growth of {data['market_trends']['demand_growth']}. "
f"Crop Type: {data['crop_type']} in the {data['crop_stage']} stage."
)
# Use LLM to generate decision support advice
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[
{"role": "system", "content": "You are an AI-powered agricultural decision support system."},
{"role": "user", "content": f"Provide comprehensive advice based on the following data: {summary}"}
]
)
advice = response.choices[0].message['content']
print(advice)
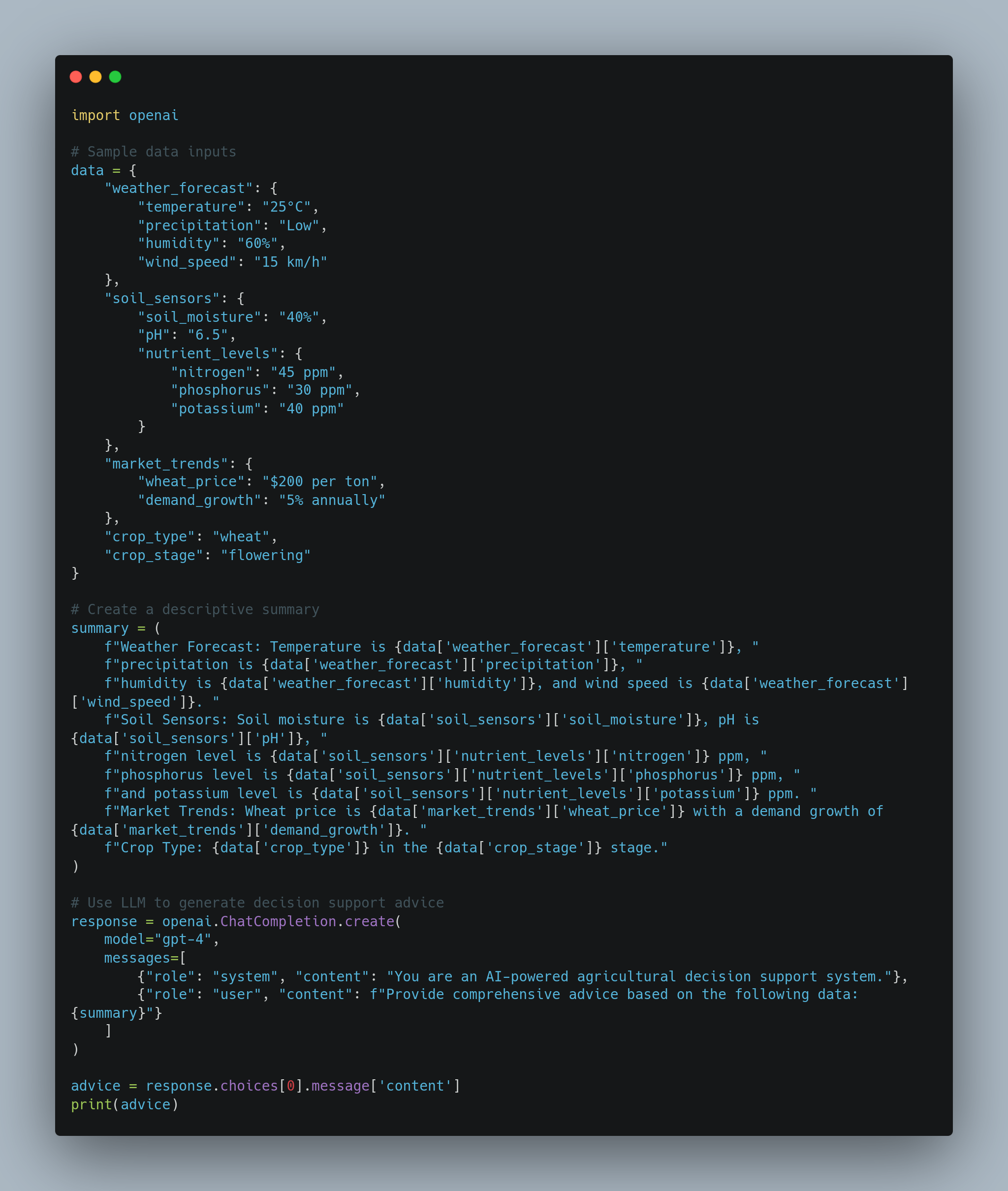
**Comprehensive Crop Management Advice for Wheat in the Flowering Stage**
**Weather Considerations:**
- **Temperature (25°C):** Optimal for wheat flowering. Maintain current irrigation levels to support continued growth.
- **Precipitation (Low):** Monitor soil moisture closely. Consider implementing supplemental irrigation if forecasts indicate prolonged dry periods.
- **Humidity (60%):** Moderate humidity levels are conducive to wheat health. Ensure adequate air circulation to prevent fungal diseases.
- **Wind Speed (15 km/h):** Manage wind exposure to reduce the risk of lodging (plants falling over). Implement windbreaks if necessary.
**Soil Management:**
- **Soil Moisture (40%):** Adequate moisture levels. Continue regular irrigation to sustain optimal growth.
- **pH (6.5):** Ideal pH for wheat. No immediate adjustments needed.
- **Nutrient Levels:**
- **Nitrogen (45 ppm):** Sufficient for the flowering stage. Avoid over-fertilization to prevent lodging.
- **Phosphorus (30 ppm):** Adequate. Continue monitoring to ensure availability for grain development.
- **Potassium (40 ppm):** Optimal levels. Maintains plant health and stress resistance.
**Market Trends:**
- **Wheat Price ($200 per ton):** Favorable market conditions. Maximize yield and quality to capitalize on high prices.
- **Demand Growth (5% annually):** Positive outlook. Invest in strategies that enhance yield and sustainability to meet growing demand.
**Recommendations:**
1. **Irrigation Management:**
- Maintain current irrigation schedules.
- Prepare for potential supplemental irrigation if dry conditions persist.
2. **Pest and Disease Control:**
- With moderate humidity, remain vigilant for signs of fungal diseases such as powdery mildew.
- Implement preventive measures, including appropriate fungicide applications if necessary.
3. **Nutrient Management:**
- Continue with balanced fertilization practices.
- Avoid excess nitrogen to prevent lodging; consider applying a controlled-release fertilizer if additional nutrients are needed.
4. **Mechanical Practices:**
- Assess fields for signs of lodging and take corrective actions if required.
- Ensure harvesting equipment is calibrated to minimize grain loss and maintain quality.
5. **Harvest Planning:**
- Monitor wheat maturity closely to determine the optimal harvest window.
- Coordinate harvesting activities to align with favorable market prices and minimize weather-related risks.
6. **Sustainability Practices:**
- Implement crop rotation strategies to maintain soil health.
- Utilize cover crops post-harvest to prevent soil erosion and enhance organic matter content.
By adhering to these recommendations, you can optimize wheat yield and quality, capitalize on favorable market conditions, and ensure sustainable farming practices for future growth.
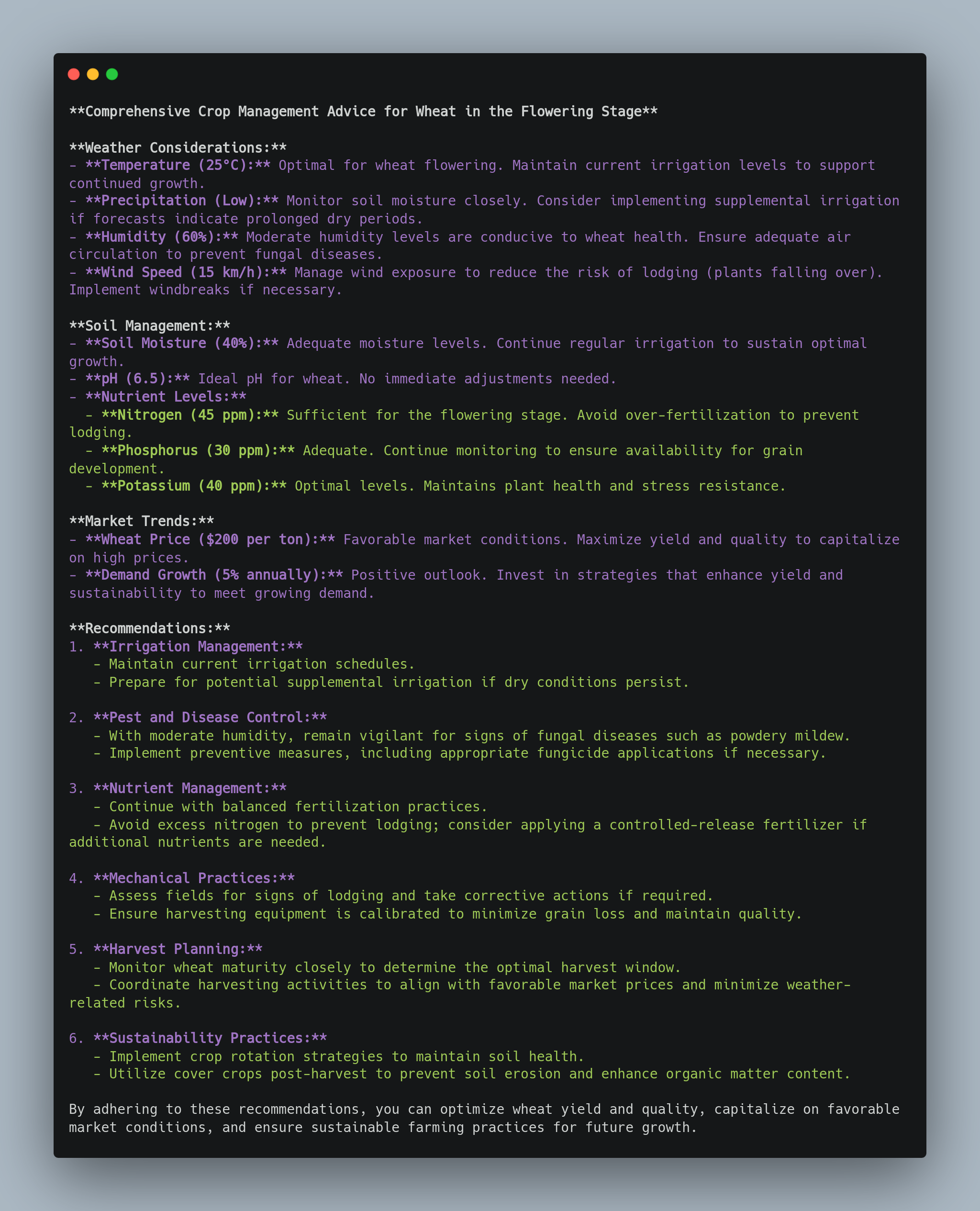
Addressing Global Agricultural Challenges with AI
AI technologies are not just limited to enhancing yields but are also pivotal in addressing global challenges such as climate change, food security, and sustainable resource management.
In regions prone to climate variability, AI models can predict and simulate different climate scenarios and recommend adaptive strategies for resilient farming. In doing so, AI helps secure food production against the changing climate.
For instance, in India, where farmers are heavily dependent on monsoon rains, AI-based systems can provide early warnings about deficient rainfalls. This allows farmers to switch to more drought-resistant crop varieties or alter their cropping patterns, thus safeguarding their livelihoods.
Example of addressing global agricultural challenges with AI
Objective: Use an LLM to generate adaptive farming strategies based on climate predictions and other global challenges.
import openai
# Sample climate data
climate_data = {
"region": "India",
"climate_challenge": "Deficient monsoon rains",
"current_crop": "rice",
"alternative_crops": ["millet", "sorghum", "pulses"],
"forecast": "El Niño event expected to reduce rainfall by 30% in the upcoming season."
}
# Create a descriptive summary
climate_summary = (
f"Region: {climate_data['region']}. "
f"Climate Challenge: {climate_data['climate_challenge']}. "
f"Current Crop: {climate_data['current_crop']}. "
f"Alternative Crops: {', '.join(climate_data['alternative_crops'])}. "
f"Forecast: {climate_data['forecast']}."
)
# Use LLM to recommend adaptive strategies
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[
{"role": "system", "content": "You are an expert in sustainable agriculture and climate adaptation."},
{"role": "user", "content": f"Given the following climate data, suggest adaptive farming strategies: {climate_summary}"}
]
)
strategies = response.choices[0].message['content']
print(strategies)

**Adaptive Farming Strategies for India Amidst Deficient Monsoon Rains**
**1. Crop Diversification:**
- **Shift to Drought-Resistant Crops:** Transition from rice to more drought-tolerant crops such as millet, sorghum, and pulses. These crops require less water and can thrive under reduced rainfall conditions.
- **Intercropping:** Implement intercropping practices by planting multiple crop species simultaneously. This enhances resource utilization and reduces the risk of total crop failure.
**2. Water Management:**
- **Rainwater Harvesting:** Construct rainwater harvesting systems to capture and store residual rainfall during the monsoon for use during dry periods.
- **Drip Irrigation:** Adopt efficient irrigation techniques like drip or sprinkler systems to minimize water wastage and ensure targeted water delivery to crops.
- **Soil Moisture Conservation:** Use mulching and cover cropping to retain soil moisture and reduce evaporation rates.
**3. Soil Health Improvement:**
- **Organic Amendments:** Incorporate organic matter such as compost or manure to improve soil structure, enhance water retention, and increase nutrient availability.
- **Conservation Tillage:** Practice conservation tillage methods to reduce soil erosion, maintain soil moisture, and promote microbial activity.
**4. Climate-Resilient Practices:**
- **Agroforestry:** Integrate trees and shrubs into agricultural landscapes to provide shade, reduce wind speed, and improve microclimates for crops.
- **Weather Forecasting Utilization:** Leverage advanced weather forecasting tools to make informed decisions about planting, irrigation, and harvesting schedules.
**5. Financial and Policy Support:**
- **Subsidies for Drought-Resistant Varieties:** Advocate for government subsidies and incentives for farmers adopting drought-resistant crop varieties and water-efficient technologies.
- **Insurance Schemes:** Promote crop insurance schemes that protect farmers against losses due to climate-induced risks.
**6. Community Engagement and Education:**
- **Training Programs:** Organize training sessions to educate farmers about climate-resilient farming techniques and the benefits of crop diversification.
- **Collaborative Platforms:** Foster community-based platforms for knowledge sharing, enabling farmers to learn from each other's experiences and adopt best practices.
**7. Technological Integration:**
- **IoT and Sensors:** Deploy IoT devices and soil moisture sensors to monitor environmental conditions in real-time, allowing for timely interventions.
- **AI-Driven Decision Support:** Utilize AI-powered tools to analyze climate data and provide personalized recommendations for crop management and resource allocation.
**8. Market Adaptation:**
- **Value Addition:** Explore value-added products and alternative markets for drought-resistant crops to enhance profitability.
- **Supply Chain Optimization:** Improve supply chain logistics to reduce post-harvest losses and ensure timely access to markets despite climatic challenges.
Implementing these adaptive strategies will help mitigate the adverse effects of deficient monsoon rains, ensure sustained agricultural productivity, and enhance the resilience of farming communities in India.
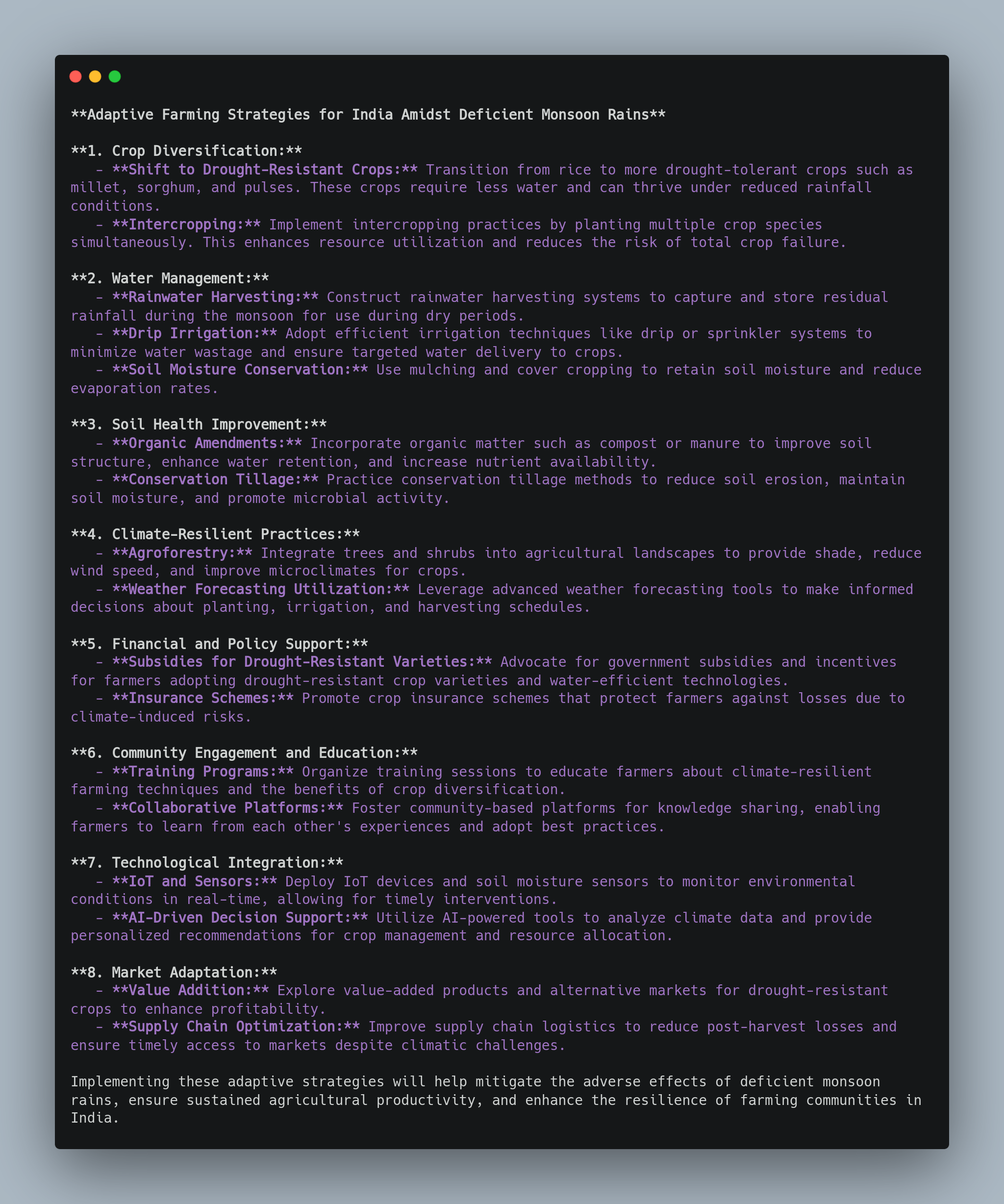
Advancing Agricultural Research through AI
AI is also making significant inroads into agricultural research. By fostering the development of new crop varieties, AI accelerates the breeding process. Machine learning models analyze vast datasets to identify traits associated with disease resistance, drought tolerance, and higher nutritional content. These insights expedite the breeding programs, leading to the development of superior crop varieties in record time.
For instance, in the quest to develop a rust-resistant wheat variety, researchers can use AI to sift through genetic data and pinpoint the genes responsible for resistance. This targeted approach not only saves time but also increases the likelihood of successful trait incorporation.
Example of advancing agricultural research through AI
Objective: Employ an LLM to assist in analyzing genetic data for breeding programs aimed at developing disease-resistant or drought-tolerant crop varieties.
import openai
# Sample genetic data summary
genetic_data = {
"crop": "wheat",
"goal": "develop rust-resistant variety",
"current_breeding_data": {
"gene_X": "associated with leaf rust resistance",
"gene_Y": "no significant association",
"gene_Z": "linked to stem rust resistance"
},
"existing_varieties": ["Variety_A", "Variety_B"],
"desired_traits": ["high yield", "drought tolerance"]
}
# Create a descriptive summary
genetic_summary = (
f"Crop: {genetic_data['crop']}. "
f"Goal: {genetic_data['goal']}. "
f"Current Breeding Data: {', '.join([f'{gene}: {desc}' for gene, desc in genetic_data['current_breeding_data'].items()])}. "
f"Existing Varieties: {', '.join(genetic_data['existing_varieties'])}. "
f"Desired Traits: {', '.join(genetic_data['desired_traits'])}."
)
# Use LLM to analyze genetic data and suggest next steps
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[
{"role": "system", "content": "You are a geneticist specializing in crop breeding."},
{"role": "user", "content": f"Analyze the following genetic data and suggest next steps for developing a rust-resistant wheat variety with high yield and drought tolerance: {genetic_summary}"}
]
)
analysis = response.choices[0].message['content']
print(analysis)
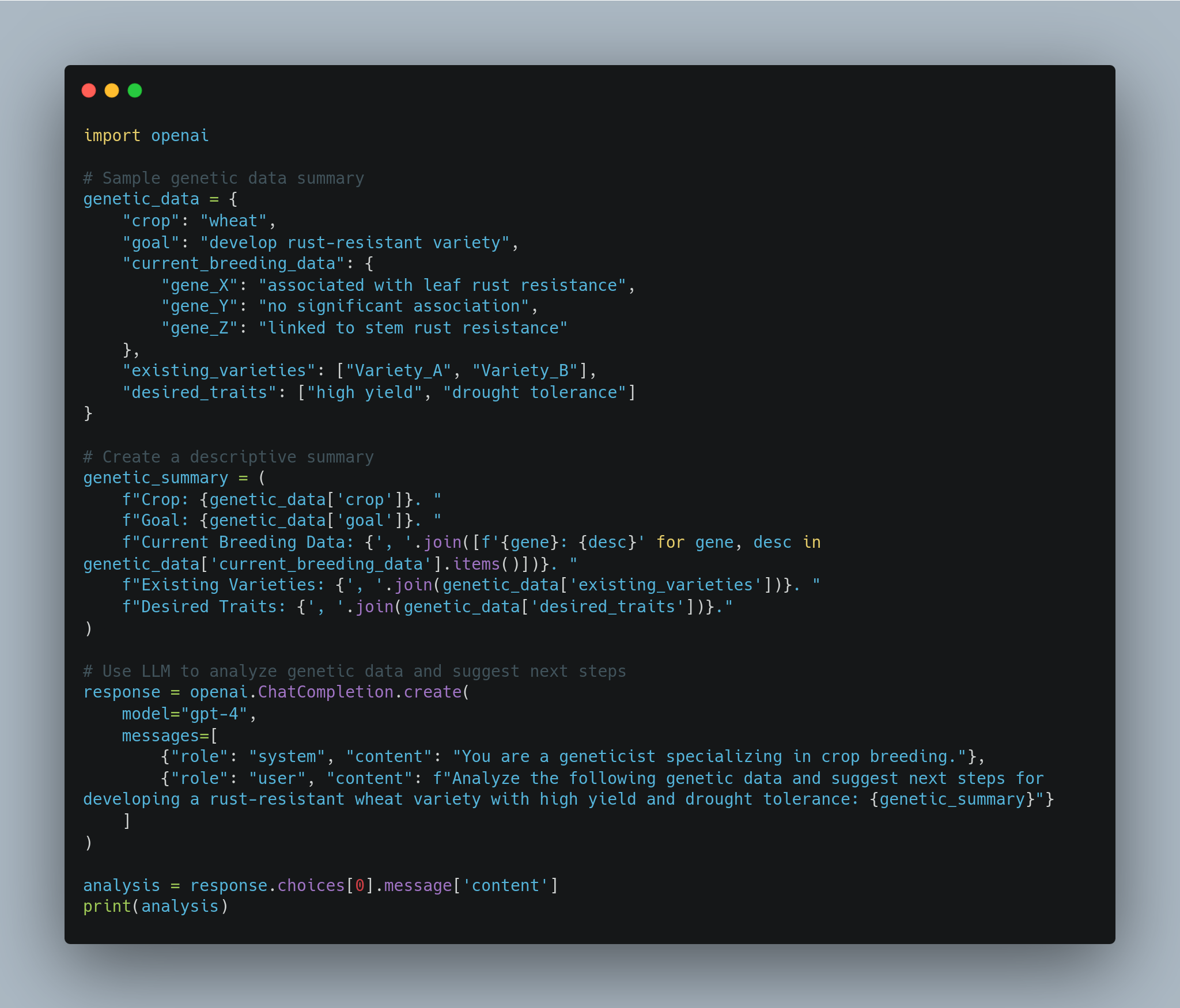
**Analysis and Recommendations for Developing a Rust-Resistant Wheat Variety with High Yield and Drought Tolerance**
**1. Genetic Analysis:**
- **Gene X:** Associated with leaf rust resistance. This gene shows promise for enhancing the plant's ability to withstand foliar rust infections.
- **Gene Y:** No significant association with rust resistance. It may be deprioritized in the breeding program.
- **Gene Z:** Linked to stem rust resistance. Incorporating this gene can provide comprehensive rust resistance, targeting both leaf and stem infections.
**2. Breeding Strategy:**
- **Marker-Assisted Selection (MAS):** Utilize molecular markers linked to Gene X and Gene Z to facilitate the selection of individuals carrying these resistance genes. This approach accelerates the breeding process by enabling the identification of desired traits at the seedling stage.
- **Pyramiding Resistance Genes:** Combine Gene X and Gene Z within a single genotype to ensure broad-spectrum rust resistance. This strategy reduces the likelihood of rust pathogens overcoming resistance through mutation.
- **Incorporate Desired Traits:**
- **High Yield:** Select parent lines known for their high-yield potential. Ensure that these lines are compatible with the rust-resistant varieties to maintain yield performance.
- **Drought Tolerance:** Integrate genes or quantitative trait loci (QTLs) associated with drought tolerance. This can be achieved through traditional breeding methods or by employing genomic selection techniques.
**3. Crossbreeding Plan:**
- **Parent Selection:** Choose existing varieties (e.g., Variety_A and Variety_B) that exhibit high yield and possess either Gene X or Gene Z.
- **Hybridization:** Perform crosses between these parent lines to combine rust resistance with high yield traits.
- **Progeny Evaluation:** Assess the offspring for rust resistance, yield performance, and drought tolerance through phenotypic screening and molecular assays.
**4. Genomic Tools and Techniques:**
- **Genomic Selection:** Implement genomic selection models to predict the performance of breeding lines based on their genetic makeup. This enhances the accuracy of selecting superior genotypes.
- **CRISPR-Cas9 Gene Editing:** Consider utilizing gene editing technologies to precisely insert or enhance Gene X and Gene Z in elite wheat varieties, reducing the time required for conventional breeding.
**5. Field Trials and Validation:**
- **Multi-Location Trials:** Conduct field trials across different environments to evaluate the stability and effectiveness of rust resistance and drought tolerance under varying conditions.
- **Pathogen Monitoring:** Continuously monitor rust pathogen populations to ensure that the resistance conferred by Gene X and Gene Z remains effective over time.
**6. Collaboration and Data Sharing:**
- **Research Partnerships:** Collaborate with research institutions and agricultural organizations to share genetic data, breeding lines, and best practices.
- **Data Management:** Maintain a comprehensive database of genetic markers, phenotypic traits, and breeding outcomes to inform future breeding decisions and track progress.
**7. Sustainability and Farmer Adoption:**
- **Seed Distribution:** Develop a strategy for the distribution of the new rust-resistant, high-yield, and drought-tolerant wheat varieties to farmers.
- **Training and Support:** Provide training to farmers on the benefits and cultivation practices of the new varieties to ensure successful adoption and maximize impact.
**Conclusion:**
By integrating Gene X and Gene Z through marker-assisted selection and genomic tools, and by incorporating high yield and drought tolerance traits, the breeding program can successfully develop a robust wheat variety. This variety will not only resist rust pathogens but also thrive under drought conditions, ensuring food security and enhancing agricultural sustainability.
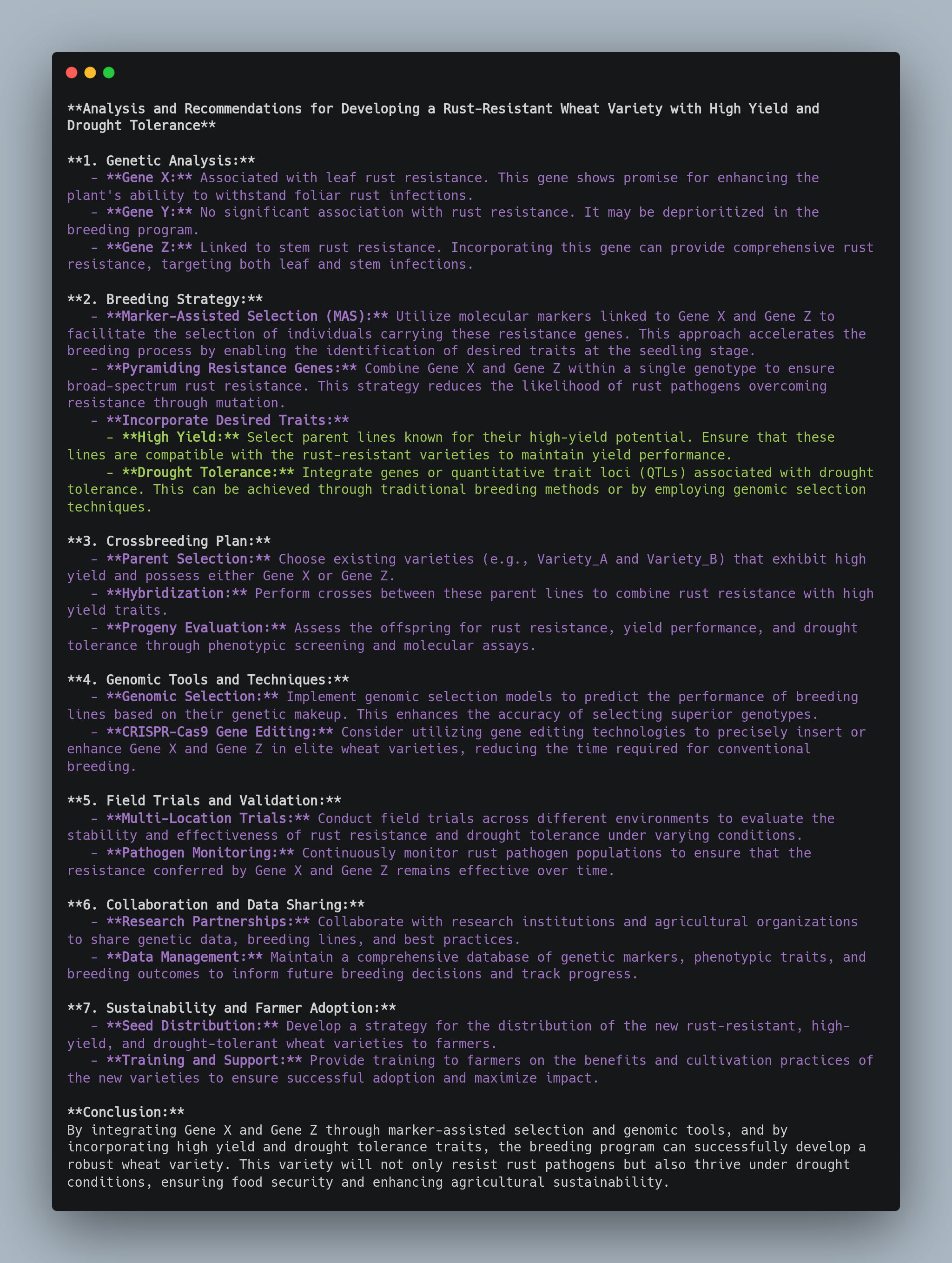
These examples demonstrate how Large Language Models (LLMs) like OpenAI's GPT-4 can be integrated into various agricultural applications to enhance decision-making, provide actionable insights, and support sustainable farming practices.
Just a quick note: make sure you handle API keys securely and comply with OpenAI's usage policies when implementing these solutions.
These strategies represent a paradigm shift towards more resilient, efficient, and sustainable farming practices. By enabling predictive analytics, precision agriculture, and enhanced soil management, AI empowers farmers to make smarter decisions, optimize resource use, and achieve higher yields. T