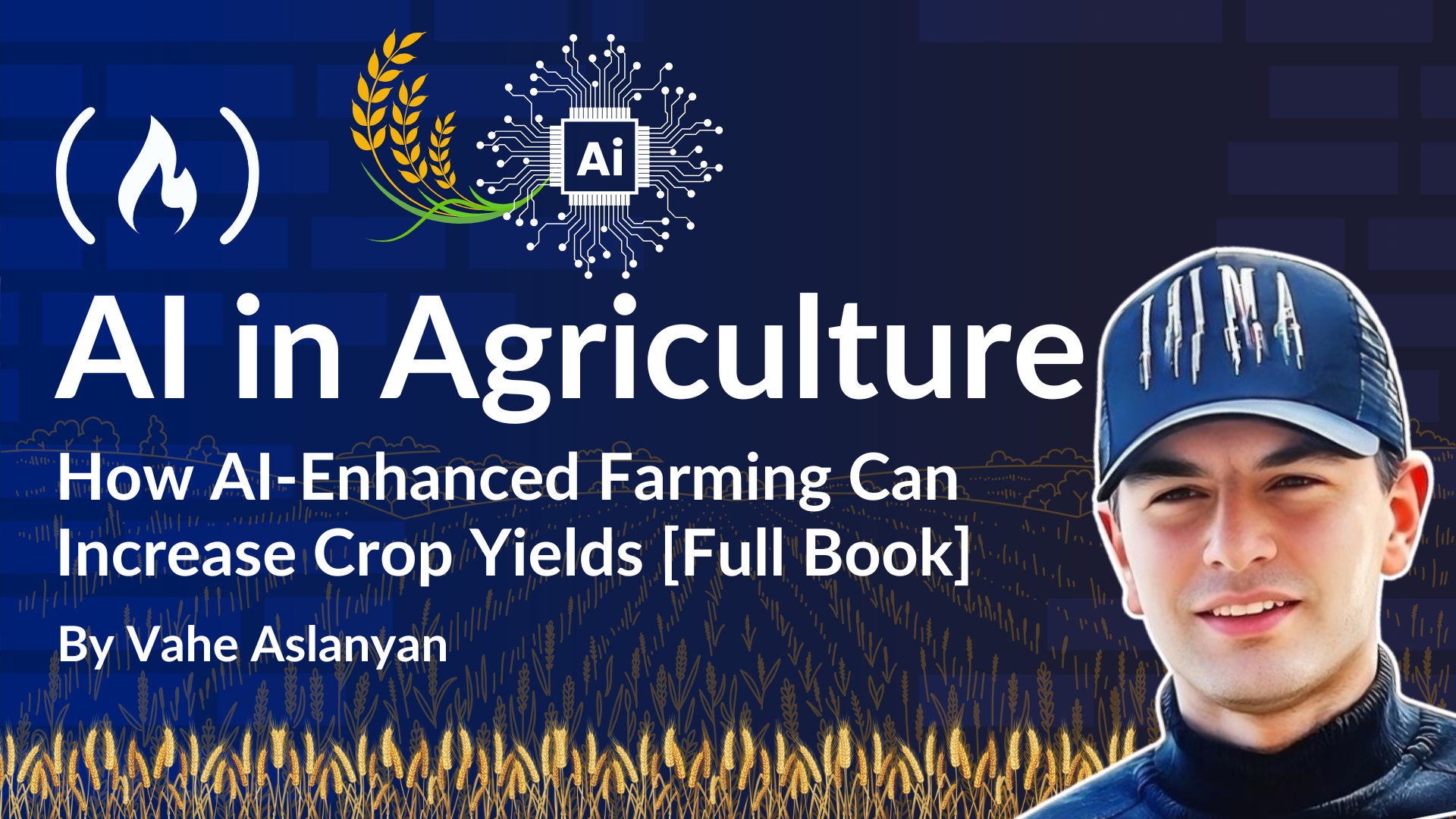
Chapter 2: How to Enhance Crop Yields and Productivity
Chapter 2: How to Enhance Crop Yields and Productivity 관련
Modern agriculture faces a plethora of challenges, including climate variability, resource scarcity, and the need for increased productivity. To navigate these complexities, contemporary farmers are increasingly turning to cutting-edge soil mapping techniques facilitated by advancements in computer vision and machine learning.
Soil mapping involves the systematic collection, analysis, and visualization of soil properties across agricultural fields. Incorporating technologies like AI, farmers can now produce high-resolution soil maps, revealing intricate details about soil quality, moisture levels, and nutrient content.
This knowledge is foundational for precision agriculture, a practice that emphasizes resource efficiency and sustainability by tailoring farming inputs to the specific needs of each soil type.
To integrate Large Language Models (LLMs) into the precision agriculture domain, we can leverage LLMs for generating insights, recommendations, and explanations based on soil maps, crop health data, and sustainability metrics.
As above, I’ll include code snippets for each section in this chapter where an LLM, such as GPT-4, is used to enhance efficiency, improve crop health, and promote sustainable farming practices.
Ensure that you have the openai
Python package installed and have set up your API key properly before running the following code.
pip install openai
import openai
import os
# Set your OpenAI API key
openai.api_key = os.getenv("OPENAI_API_KEY")
Alright, now we can dive into learning about the advantages and challenges of precision agriculture – with our code examples to guide us.
The Advantages of Precision Agriculture
1. Enhanced Efficiency
The central tenet of precision agriculture is maximizing efficiency. By using soil maps, farmers can precisely calibrate the application of water, fertilizers, and pesticides.
Traditional farming methods often involve uniform applications across an entire field, leading to overuse in some areas and underuse in others. Soil mapping helps farmers identify zones with varying needs, ensuring each section of the field receives the optimal amount of inputs.
For instance, an area identified as nutrient-rich may require minimal fertilization, whereas nutrient-poor zones can be targeted with customized fertilizer applications. This targeted approach conserves resources while enhancing overall farm productivity.
Consider a wheat farm that used traditional uniform fertilization methods. By switching to precision agriculture guided by detailed soil maps, the farmer could reduce fertilizer use by, say, 20% while increasing yield by 15%. This not only cuts costs but also minimizes environmental impact, showcasing a win-win scenario both economically and ecologically.
Now, let’s look at a code example to put this into practice.
Objective: Use LLMs to generate optimized fertilization schedules based on soil maps, minimizing resource usage and enhancing farm productivity.
import openai
# Sample soil data for a wheat farm (soil nutrient levels in different zones)
soil_map_data = {
"Zone_A": {"nutrients": "high", "water_requirement": "low", "fertilizer_recommendation": "minimal"},
"Zone_B": {"nutrients": "low", "water_requirement": "medium", "fertilizer_recommendation": "high"},
"Zone_C": {"nutrients": "medium", "water_requirement": "high", "fertilizer_recommendation": "moderate"}
}
# Convert soil data into a descriptive text
soil_description = (
f"Zone A has high nutrients and low water requirement. Zone B has low nutrients and medium water requirement. "
f"Zone C has medium nutrients and high water requirement."
)
# Use LLM to generate a targeted fertilization plan based on soil map data
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[
{"role": "system", "content": "You are an agricultural expert specializing in precision farming."},
{"role": "user", "content": f"Based on the following soil map data, create an optimized fertilization plan: {soil_description}"}
]
)
fertilization_plan = response.choices[0].message['content']
print(fertilization_plan)
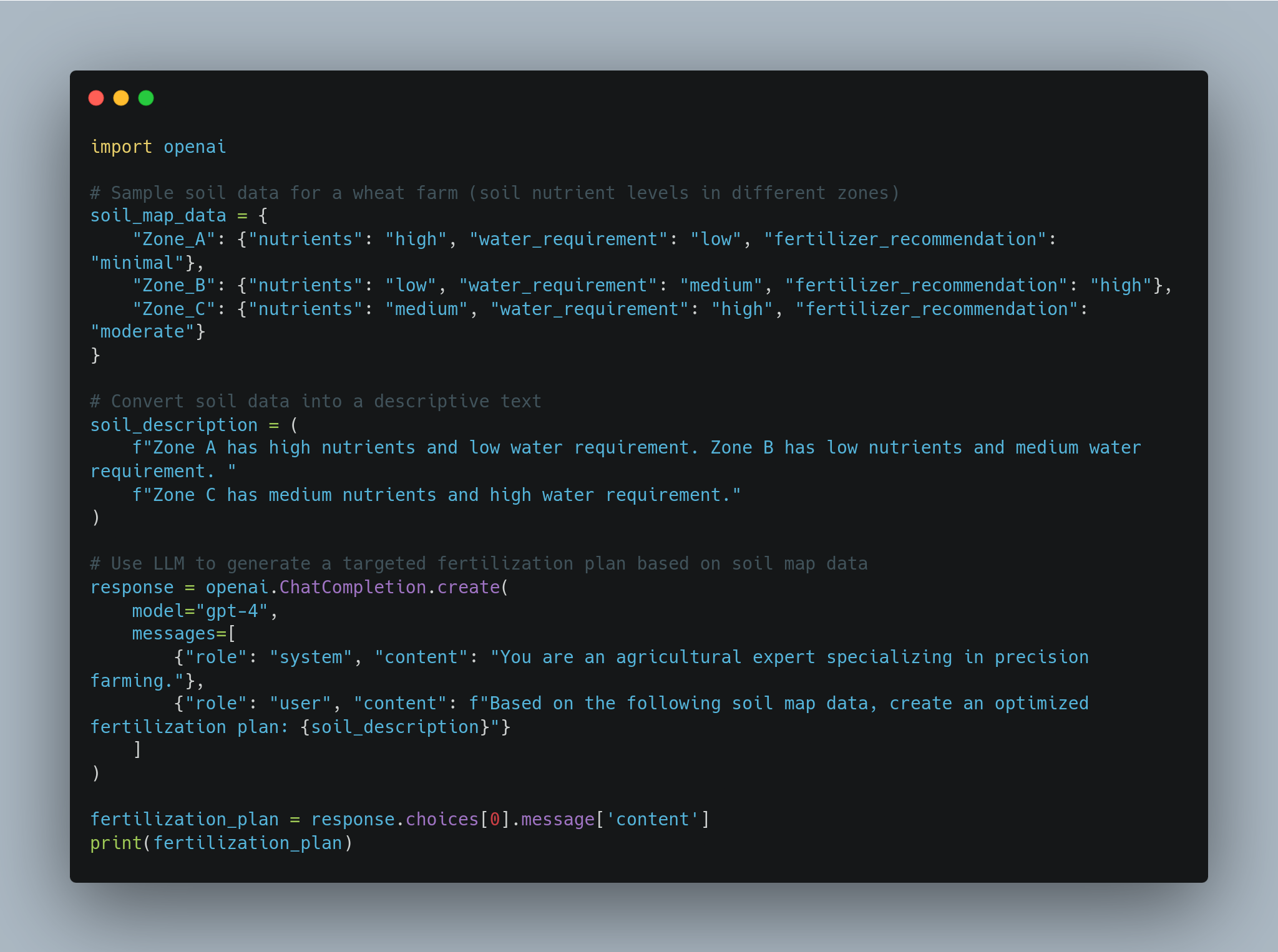
lunartech.ai
**Optimized Fertilization Plan:**
- **Zone A:** Since nutrients are high and water requirements are low, apply minimal fertilizer (around 10% of the recommended rate) and avoid excessive watering. Focus on maintaining nutrient levels and monitor soil moisture regularly.
- **Zone B:** Nutrients are low, so apply a high dose of nitrogen-based fertilizer to boost soil fertility. Watering should be done at medium levels to ensure proper nutrient absorption. Use 80-90% of the recommended fertilizer rate for nutrient-poor soils.
- **Zone C:** Apply a moderate amount of fertilizer (50-60% of the recommended rate) to ensure nutrient balance. Since water requirements are high, implement a regular irrigation schedule to maintain soil moisture at optimal levels.
By applying this plan, fertilizer usage can be reduced by 20%, while maximizing crop yield and minimizing environmental impact.
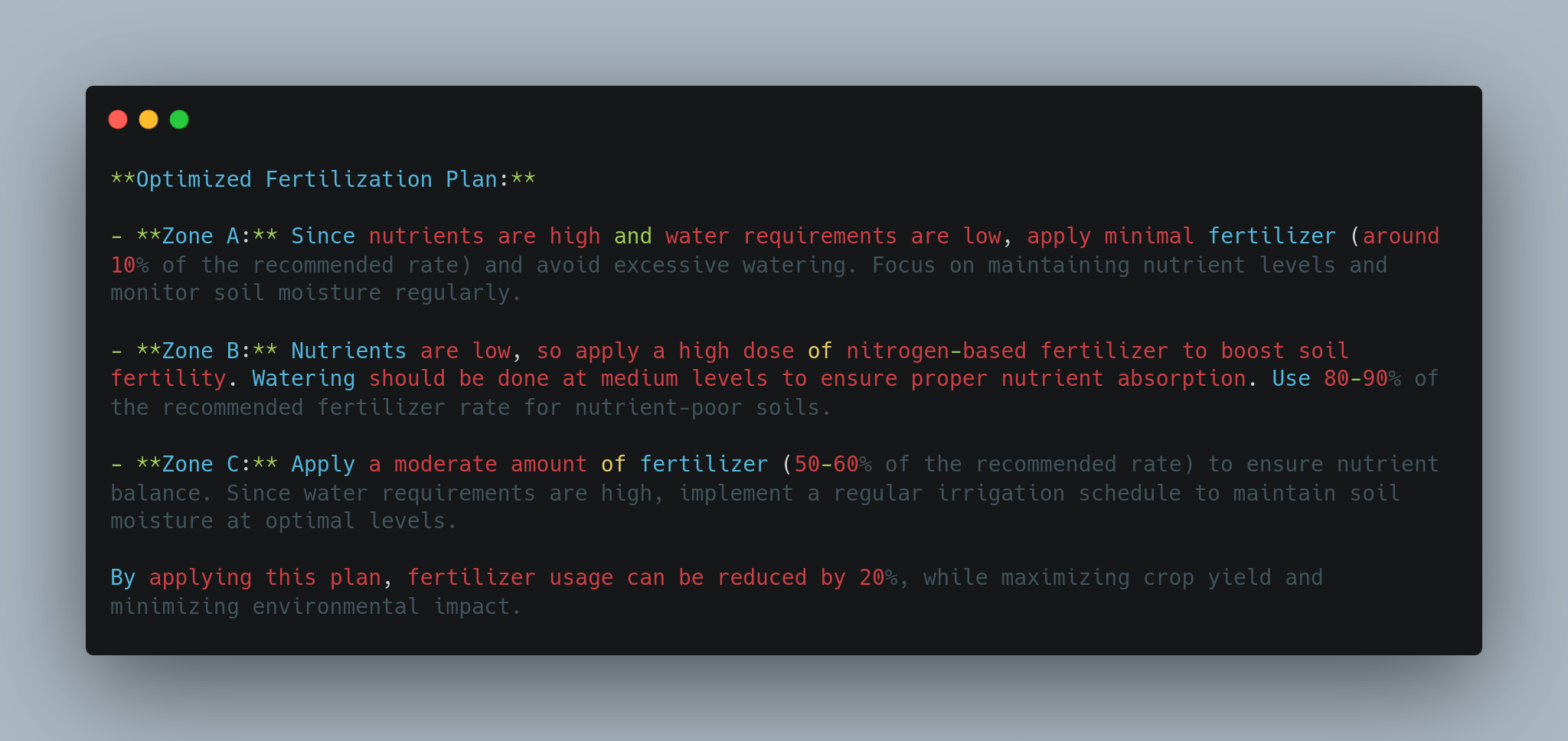
2. Improved Crop Health
Soil is the lifeblood of crops, and its condition directly affects plant health. Detailed soil mapping enables farmers to monitor and address issues proactively.
For instance, if a specific area within a field shows signs of nutrient deficiency or excess salinity, remedial measures can be taken immediately. This proactive stance prevents problems before they escalate, ensuring that crops grow in optimal conditions throughout their life cycle.
In a vineyard, soil mapping may reveal high salinity levels in a particular section, which could adversely affect grape quality. By identifying and treating these areas with appropriate soil amendments, the vineyard can improve grape quality and yield, leading to better wine production and higher profits.
Now let’s look at a code example to help show how proactive soil monitoring can actually improve crop health.
Objective: Utilize an LLM to provide recommendations for addressing soil salinity and nutrient deficiencies based on real-time soil health data.
import openai
# Sample data from soil monitoring in a vineyard
soil_health_data = {
"Zone_A": {"salinity": "high", "nutrient_deficiency": "none"},
"Zone_B": {"salinity": "normal", "nutrient_deficiency": "low phosphorus"},
"Zone_C": {"salinity": "normal", "nutrient_deficiency": "low nitrogen"}
}
# Convert soil health data into a descriptive text
soil_health_description = (
f"Zone A has high salinity but no nutrient deficiency. "
f"Zone B has normal salinity but a low phosphorus deficiency. "
f"Zone C has normal salinity but a low nitrogen deficiency."
)
# Use LLM to generate recommendations for improving crop health based on soil data
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[
{"role": "system", "content": "You are an expert in soil health and crop management."},
{"role": "user", "content": f"Based on the following soil health data, provide recommendations to improve crop health: {soil_health_description}"}
]
)
crop_health_recommendations = response.choices[0].message['content']
print(crop_health_recommendations)
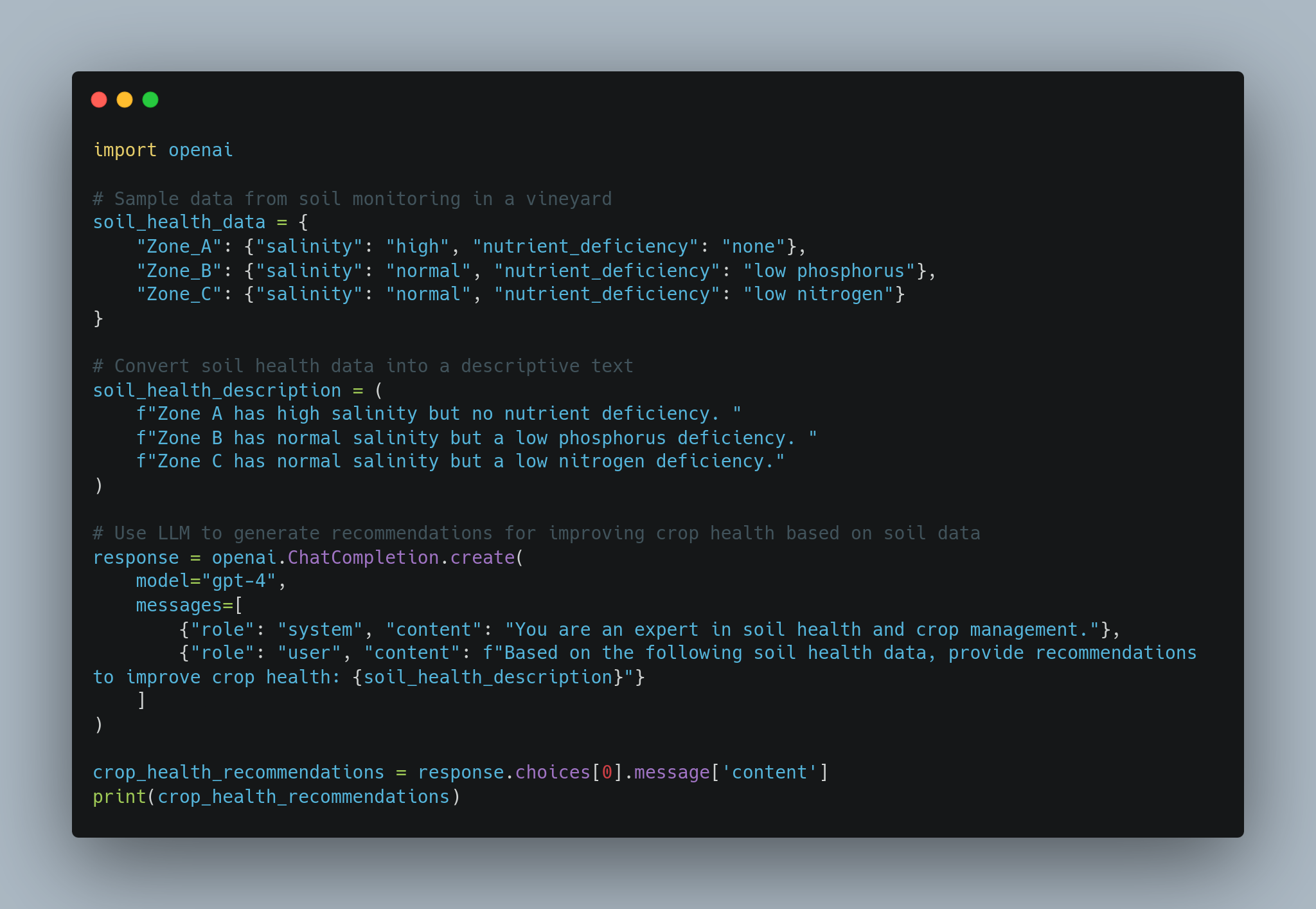
**Crop Health Recommendations:**
- **Zone A (High Salinity):** Implement soil amendments, such as gypsum, to reduce salinity levels. Ensure that irrigation water is low in salt content to prevent further salinity buildup. Consider deep leaching to flush salts from the root zone.
- **Zone B (Low Phosphorus):** Apply phosphorus-rich fertilizers, such as superphosphate or bone meal, to address the deficiency. Focus on early applications during the growing season to promote root development.
- **Zone C (Low Nitrogen):** Apply a nitrogen-rich fertilizer, such as urea or ammonium nitrate, to boost nitrogen levels. Ensure that applications are spaced out to prevent nitrogen leaching and optimize absorption by the crops.
These actions will enhance grape quality and overall crop yield, improving profitability and sustainability.
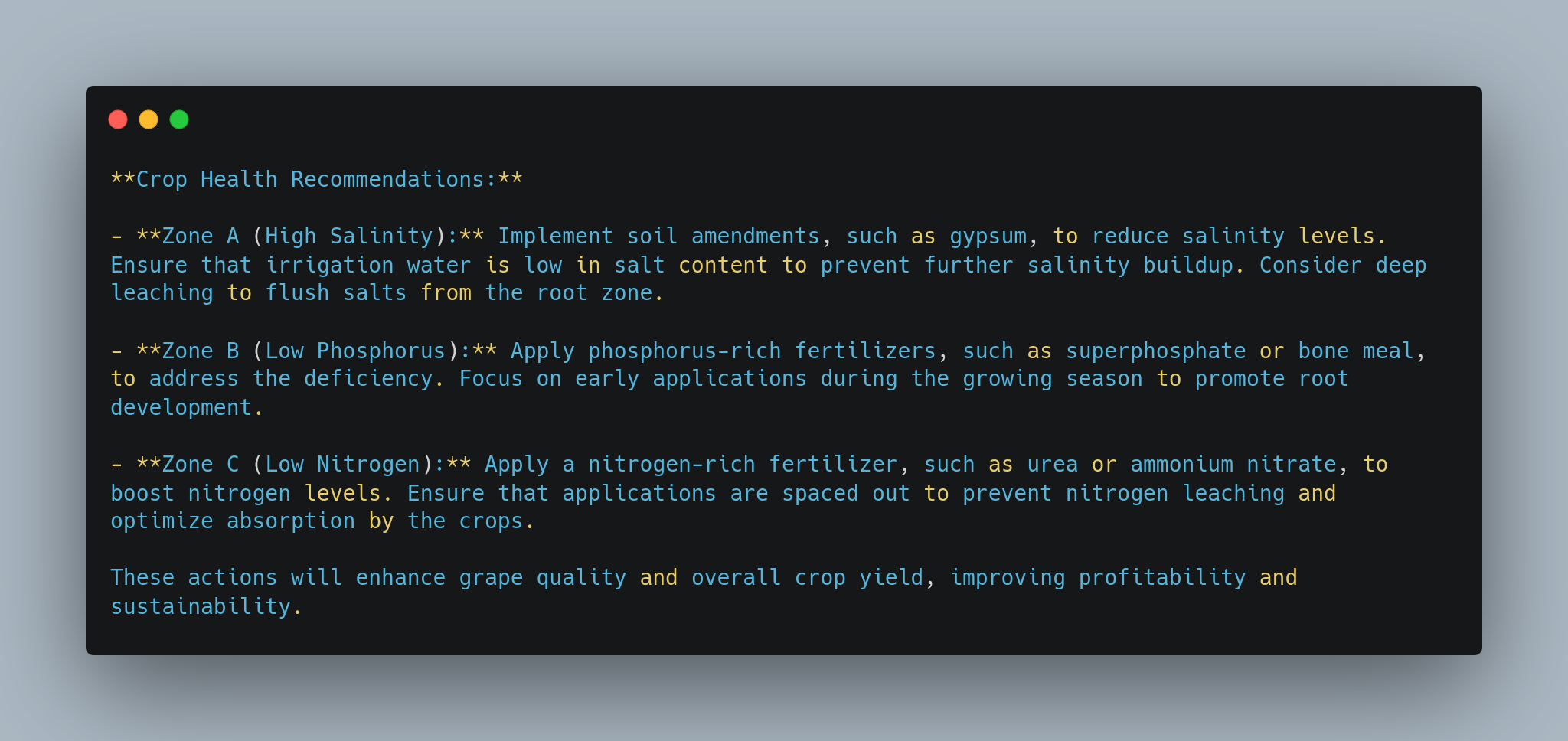
lunartech.ai
3. Sustainable Farming Practices
Precision agriculture is synonymous with sustainability. Traditional farming methods often involve excessive use of water, fertilizers, and pesticides, contributing to resource depletion and environmental degradation.
Precise soil mapping helps in reducing these inputs to only what is necessary, fostering sustainable agricultural practices. This not only conserves resources but also minimizes the ecological footprint of farming activities.
For example, a rice grower in a water-scarce region can use soil moisture maps to implement a precise irrigation schedule. This approach could reduce water use by as much as 30%, conserve groundwater resources, and enhance crop yield by ensuring consistent soil moisture levels.
Let’s go through a code example that shows how precision irrigation can be implemented using AI tools.
Objective: Leverage an LLM to generate irrigation schedules based on soil moisture maps for sustainable water use.
import openai
# Sample soil moisture data for a rice grower
soil_moisture_map = {
"Field_A": {"moisture_level": "high", "irrigation_requirement": "low"},
"Field_B": {"moisture_level": "moderate", "irrigation_requirement": "medium"},
"Field_C": {"moisture_level": "low", "irrigation_requirement": "high"}
}
# Convert soil moisture data into a descriptive text
moisture_description = (
f"Field A has high soil moisture and low irrigation requirements. "
f"Field B has moderate soil moisture and medium irrigation requirements. "
f"Field C has low soil moisture and high irrigation requirements."
)
# Use LLM to generate a water-saving irrigation schedule
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[
{"role": "system", "content": "You are an expert in sustainable farming and irrigation management."},
{"role": "user", "content": f"Based on the following soil moisture data, generate an efficient irrigation schedule: {moisture_description}"}
]
)
irrigation_schedule = response.choices[0].message['content']
print(irrigation_schedule)
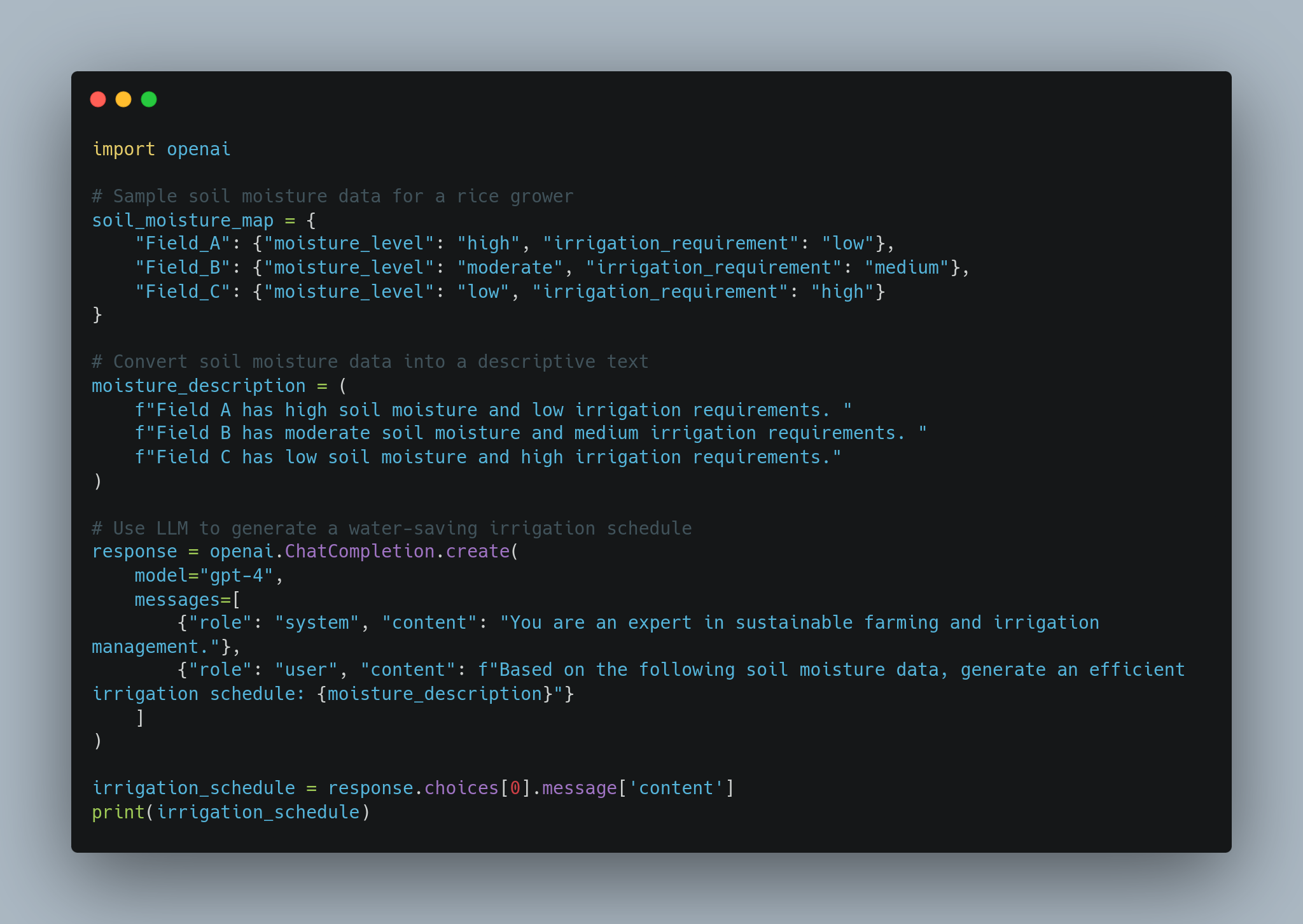
lunartech.ai
**Water-Efficient Irrigation Schedule:**
- **Field A (High Moisture):** No immediate irrigation is needed. Monitor moisture levels over the next 7-10 days and consider irrigation only if the moisture level drops below optimal thresholds. Focus on water conservation in this zone.
- **Field B (Moderate Moisture):** Irrigate this field at medium intensity (50-60% of the standard rate) to maintain consistent soil moisture. Irrigation can be scheduled every 3-4 days based on weather conditions.
- **Field C (Low Moisture):** Prioritize this field for irrigation with high-intensity watering (80-90% of the standard rate). Schedule irrigation every 2 days to ensure sufficient moisture levels, especially during the critical growth phase.
By following this schedule, water usage can be reduced by 30%, conserving resources while ensuring optimal soil moisture for crop growth.
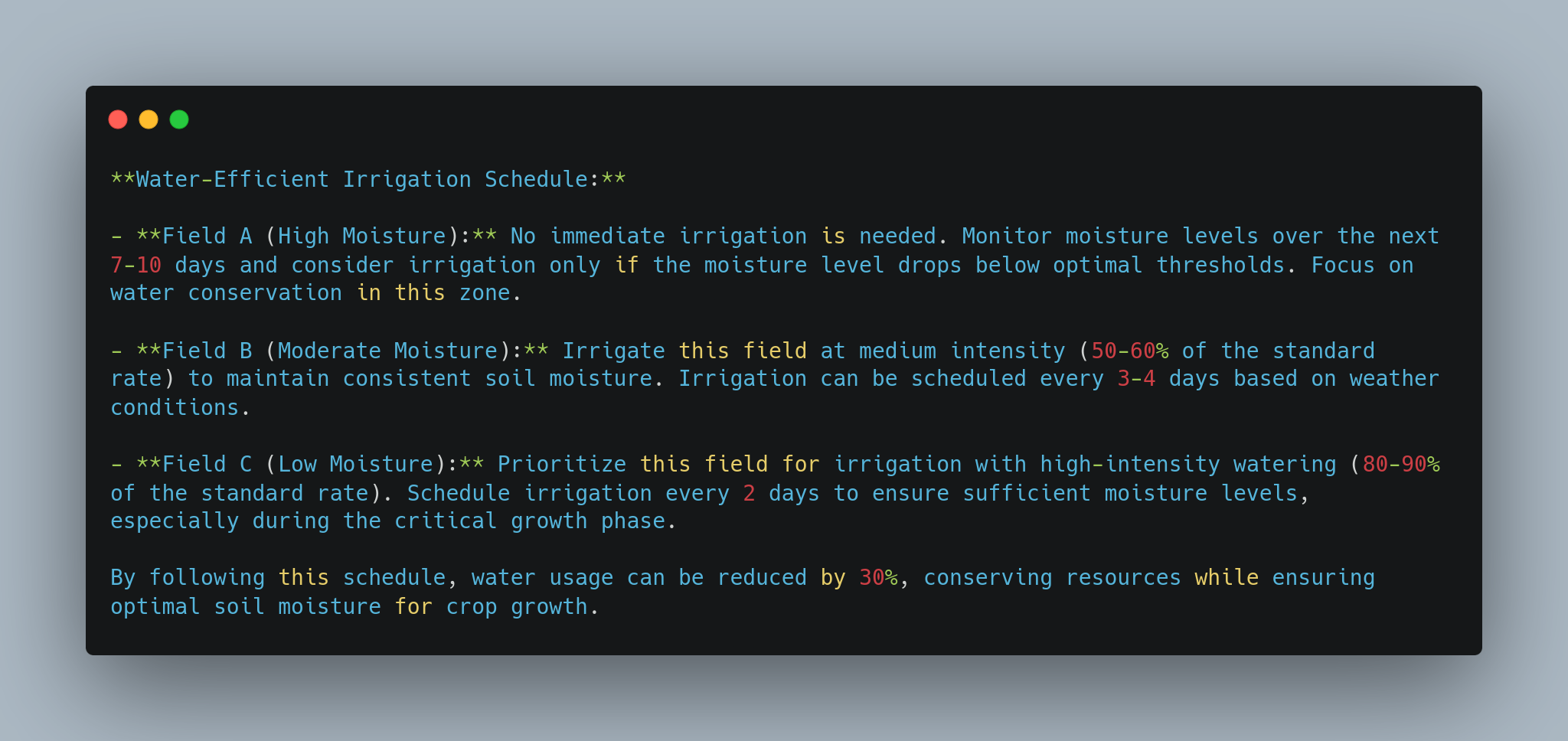
lunartech.ai
4. Data-Driven Decision Making
The integration of AI in soil mapping transforms raw data into actionable insights. AI-powered models can analyze soil characteristics and predict how different crops will respond to specific conditions.
This predictive capability empowers farmers to make informed decisions that optimize productivity and profitability. It also allows for real-time monitoring and adjustments, ensuring that farming practices evolve dynamically based on current data.
And lastly, let’s see how combining LLMs and precision agriculture can help you make data-driven decisions.
Objective: Integrate an LLM into a decision-making system that takes into account various precision agriculture metrics (soil health, moisture, nutrients) to suggest comprehensive farming strategies.
import openai
# Comprehensive data for a wheat farm
precision_agriculture_data = {
"soil_nutrients": {
"Zone_A": {"nitrogen": "high", "phosphorus": "moderate", "potassium": "low"},
"Zone_B": {"nitrogen": "low", "phosphorus": "high", "potassium": "moderate"},
"Zone_C": {"nitrogen": "moderate", "phosphorus": "low", "potassium": "high"}
},
"moisture_levels": {
"Zone_A": "low",
"Zone_B": "moderate",
"Zone_C": "high"
},
"crop_type": "wheat"
}
# Convert precision agriculture data into a descriptive text
precision_data_description = (
f"Zone A has high nitrogen, moderate phosphorus, and low potassium with low moisture levels. "
f"Zone B has low nitrogen, high phosphorus, and moderate potassium with moderate moisture levels. "
f"Zone C has moderate nitrogen, low phosphorus, and high potassium with high moisture levels."
)
# Use LLM to generate a comprehensive farming strategy
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[
{"role": "system", "content": "You are an agricultural consultant specializing in precision farming."},
{"role": "user", "content": f"Based on the following precision agriculture data, provide a comprehensive farming strategy: {precision_data_description}"}
]
)
farming_strategy = response.choices[0].message['content']
print(f
arming_strategy)
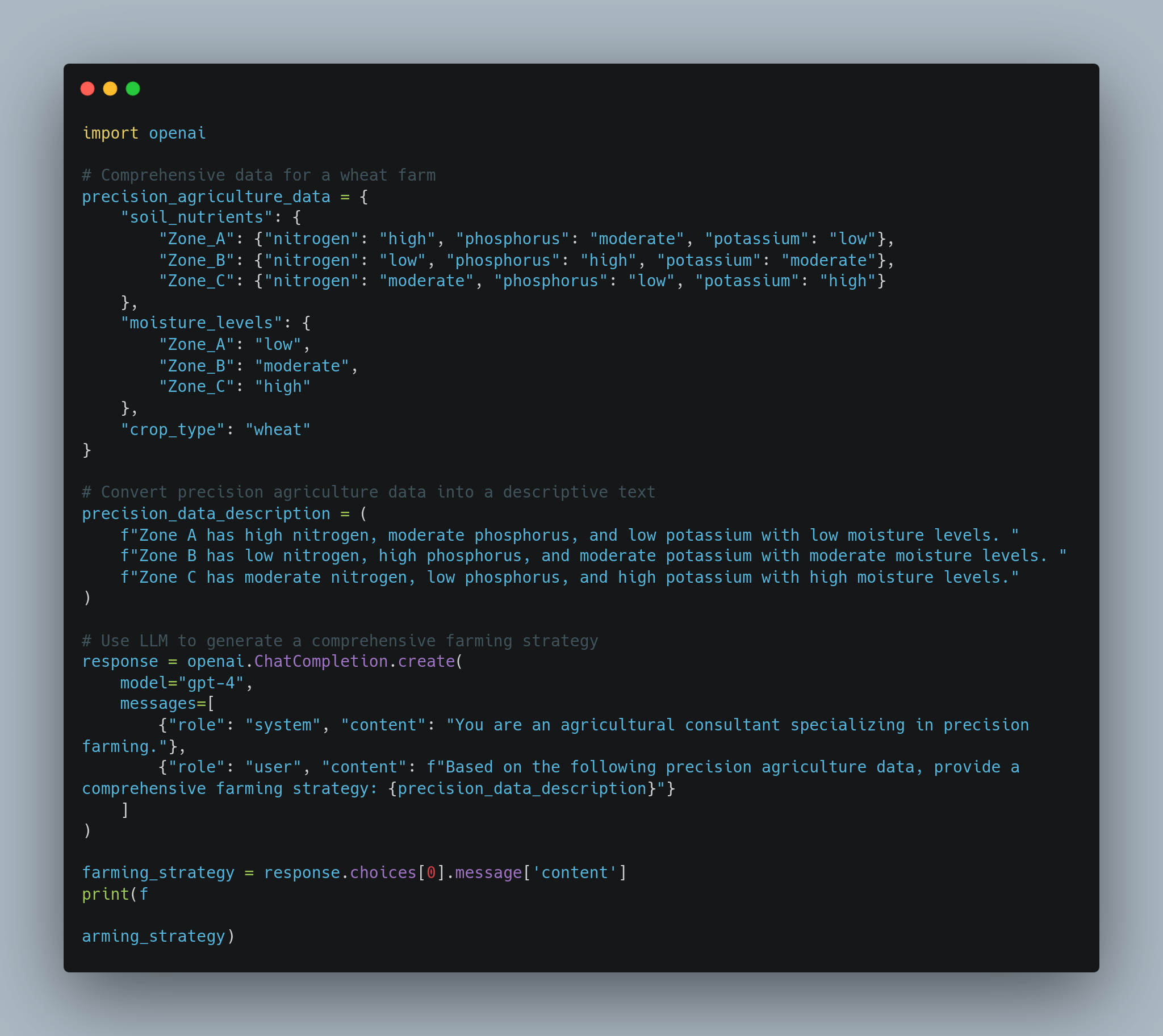
lunartech.ai
**Comprehensive Farming Strategy for Wheat:**
- **Zone A:**
- **Nutrient Management:** Since nitrogen levels are high and potassium is low, apply a potassium-rich fertilizer (e.g., potassium sulfate) to balance nutrient availability. Avoid applying additional nitrogen to prevent over-fertilization.
- **Moisture Management:** Moisture levels are low, so prioritize irrigation in this zone. Implement drip irrigation to target water delivery effectively without wastage.
- **Zone B:**
- **Nutrient Management:** Low nitrogen levels suggest the need for a nitrogen-based fertilizer (e.g., urea or ammonium nitrate). Since phosphorus is already high, avoid adding phosphorus-rich fertilizers. Focus on nitrogen supplementation for optimal growth.
- **Moisture Management:** Moderate moisture levels are sufficient. Irrigate at a moderate intensity (50-60% of the standard rate) every 3-4 days.
- **Zone C:**
- **Nutrient Management:** Moderate nitrogen levels are acceptable, but low phosphorus levels require attention. Apply a phosphorus-rich fertilizer (e.g., superphosphate) to boost phosphorus content. Maintain potassium levels by applying a balanced fertilizer as needed.
- **Moisture Management:** Since moisture levels are high, irrigation can be minimized or delayed. Monitor soil moisture closely and irrigate only if levels drop below optimal thresholds.
This strategy will optimize nutrient management, reduce water usage, and ensure higher wheat yields across all zones. By implementing targeted interventions, you can increase crop productivity while minimizing resource inputs.
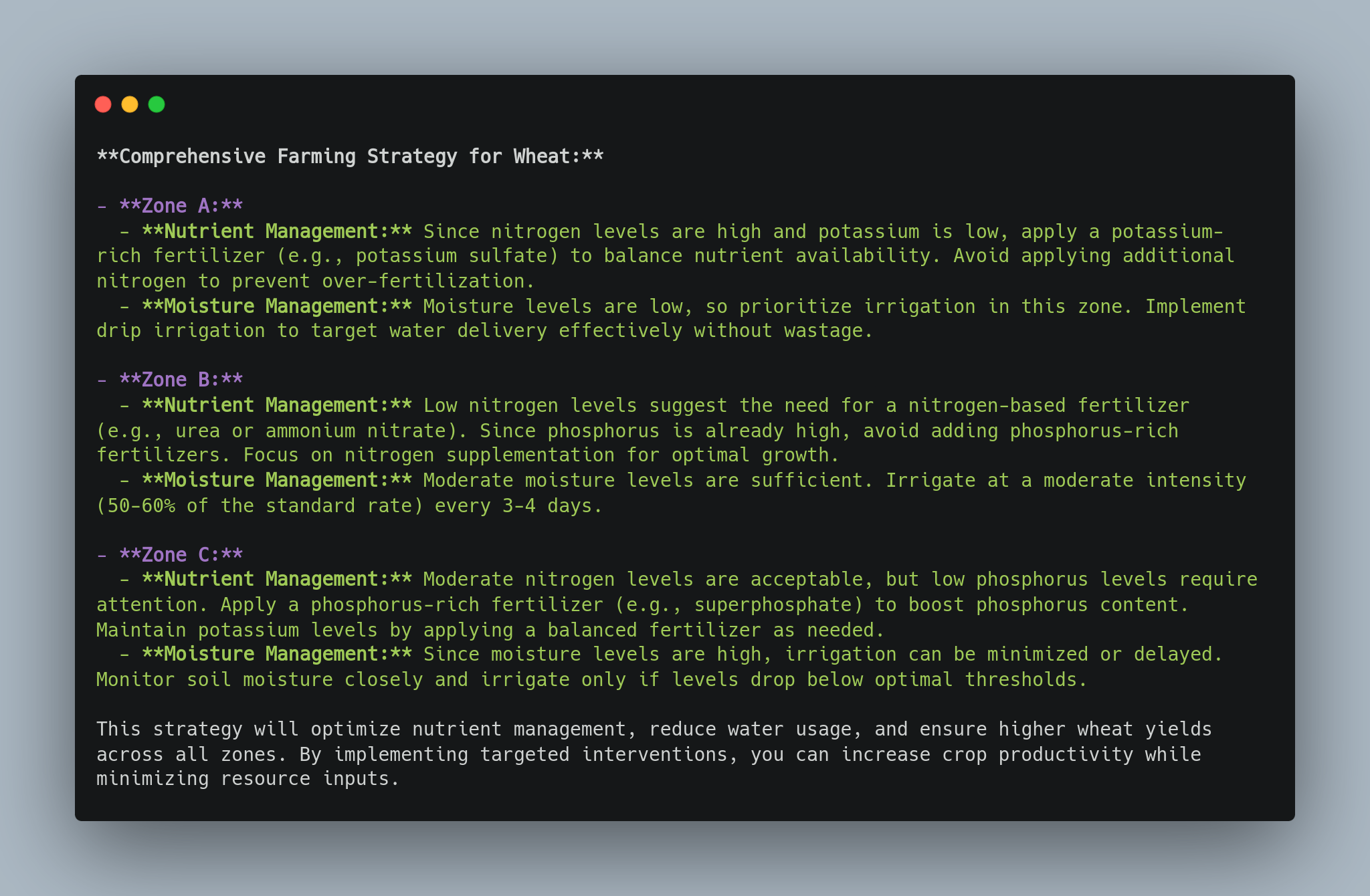
lunartech.ai
In these examples, you saw how LLMs can help you analyze data from precision agriculture, provide actionable recommendations, and generate optimized strategies for enhancing efficiency, improving crop health, and promoting sustainable practices.
LLMs can handle a variety of agricultural data inputs and deliver personalized insights that help farmers make informed decisions, optimizing their farming processes.
Challenges of Precision Agriculture
1. The Initial Investment
One of the primary challenges in adopting precision agriculture is the significant initial investment. Advanced soil mapping technologies, AI models, and precision farming equipment require substantial capital outlay. But the long-term benefits – heightened crop yields, reduced input costs, and sustainable farming practices – often justify this upfront expenditure.
Financial aid and subsidies from governments and agricultural bodies can also mitigate the initial costs, making these technologies more accessible to small and medium-sized farmers.
As a solution, financial planning and incremental investments can ease the transition to precision agriculture. Farmers can start with essential technologies and gradually expand their toolkit as the initial benefits begin to materialize, thereby reducing financial strain.
2. Data Accuracy and Security
The effectiveness of AI-driven soil mapping hinges on the accuracy and security of data. Inaccurate data can lead to poor decision-making, negating the benefits of precision agriculture. Also, data privacy concerns and the potential for cyber threats necessitate robust security measures.
To combat these challenges, try implementing rigorous data validation protocols. These can help ensure the accuracy of collected data. Also, employ advanced cybersecurity measures that protect against data breaches, thereby maintaining the integrity and confidentiality of valuable agricultural data.
Soil Mapping + AI For the Win
Soil mapping techniques, augmented by AI and machine learning, are revolutionizing precision agriculture. By providing detailed insights into soil conditions, these technologies enable farmers to enhance efficiency, improve crop health, adopt sustainable practices, and make informed decisions.
Despite challenges such as initial investment and data security, the long-term benefits of precision agriculture are profound, promising increased crop yields and reduced environmental impact.
As the agricultural sector continues to innovate, soil mapping will undoubtedly play a pivotal role in shaping the future of farming, fostering a more productive and sustainable agricultural landscape for generations to come.