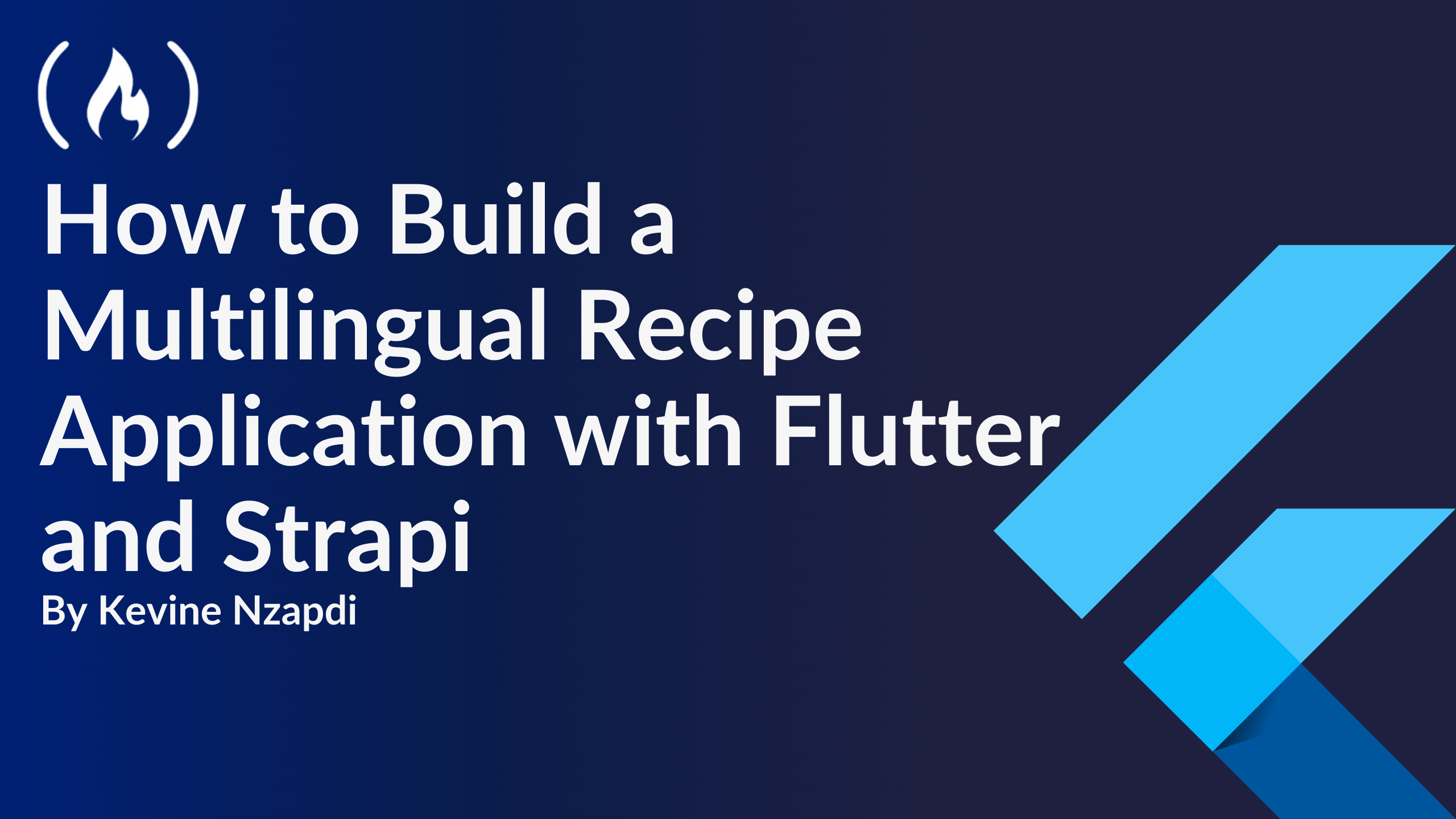
Create User Profile Screen
Create User Profile Screen 관련
In the lib/screens/
profile.dart
file, add the code below:
import 'package:easy_localization/easy_localization.dart';
import 'package:flutter/material.dart';
import 'package:flutter_recipe_app/screens/requestRecipe.dart';
import '../models/recipe.dart';
import '../utils/server2.dart';
class ProfileScreen extends StatefulWidget {
_ProfileScreenState createState() => _ProfileScreenState();
}
class _ProfileScreenState extends State<ProfileScreen> {
late Future<List<RecipeRequest>> _requestedRecipesFuture;
void initState() {
super.initState();
_requestedRecipesFuture = ApiService().fetchUserRequestedRecipes();
}
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(tr('profile')),
),
body: Column(
children: [
Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
SizedBox(height: 10),
Text(
tr('request_list'),
style: TextStyle(fontSize: 16, color: Colors.grey[600]),
),
SizedBox(height: 20),
ElevatedButton(
onPressed: () {
Navigator.pop(context);
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => RecipeRequestScreen(),
),
);
},
child: Text(tr('request_new_recipe')),
),
],
),
),
Expanded(
child: FutureBuilder<List<RecipeRequest>>(
future: _requestedRecipesFuture,
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.waiting) {
return Center(child: CircularProgressIndicator());
} else if (snapshot.hasError) {
return Center(child: Text('Error: ${snapshot.error.toString()}'));
} else if (snapshot.data == null || snapshot.data!.isEmpty) {
return Center(child: Text(tr('no_request_found')));
}
return ListView.builder(
itemCount: snapshot.data!.length,
itemBuilder: (context, index) {
RecipeRequest request = snapshot.data![index];
String fullDescription = request.description
.map((d) => d.children.map((t) => t.text).join('\n'))
.join('\n\n');
return Padding(
padding: const EdgeInsets.symmetric(horizontal: 40.0),
child: ListTile(
title: Text(
request.title.toUpperCase(),
style: const TextStyle(fontWeight: FontWeight.bold),
),
subtitle: Text(fullDescription),
),
);
},
);
},
),
),
],
),
);
}
}
The ProfileScreen
class in this Flutter application represents a user's profile page where they can view their requested recipes. When the screen is initialized, it fetches a list of recipes requested by the user by calling the fetchUserRequestedRecipes
method from the ApiService
. This data is then stored in the _requestedRecipesFuture
variable, which is a Future
that will eventually hold the list of requested recipes.
In the build
method, the screen is constructed using a Scaffold
widget.
The main part of the screen is an Expanded
widget containing a FutureBuilder
. The FutureBuilder
widget waits for the _requestedRecipesFuture
to complete and then builds the list of requested recipes. If the data is still loading, it shows a CircularProgressIndicator
. If there's an error, it displays an error message. And if there are no recipes, it shows a "no request found" message. Otherwise, it displays the list of requested recipes, each rendered as a ListTile
with the recipe title and description.