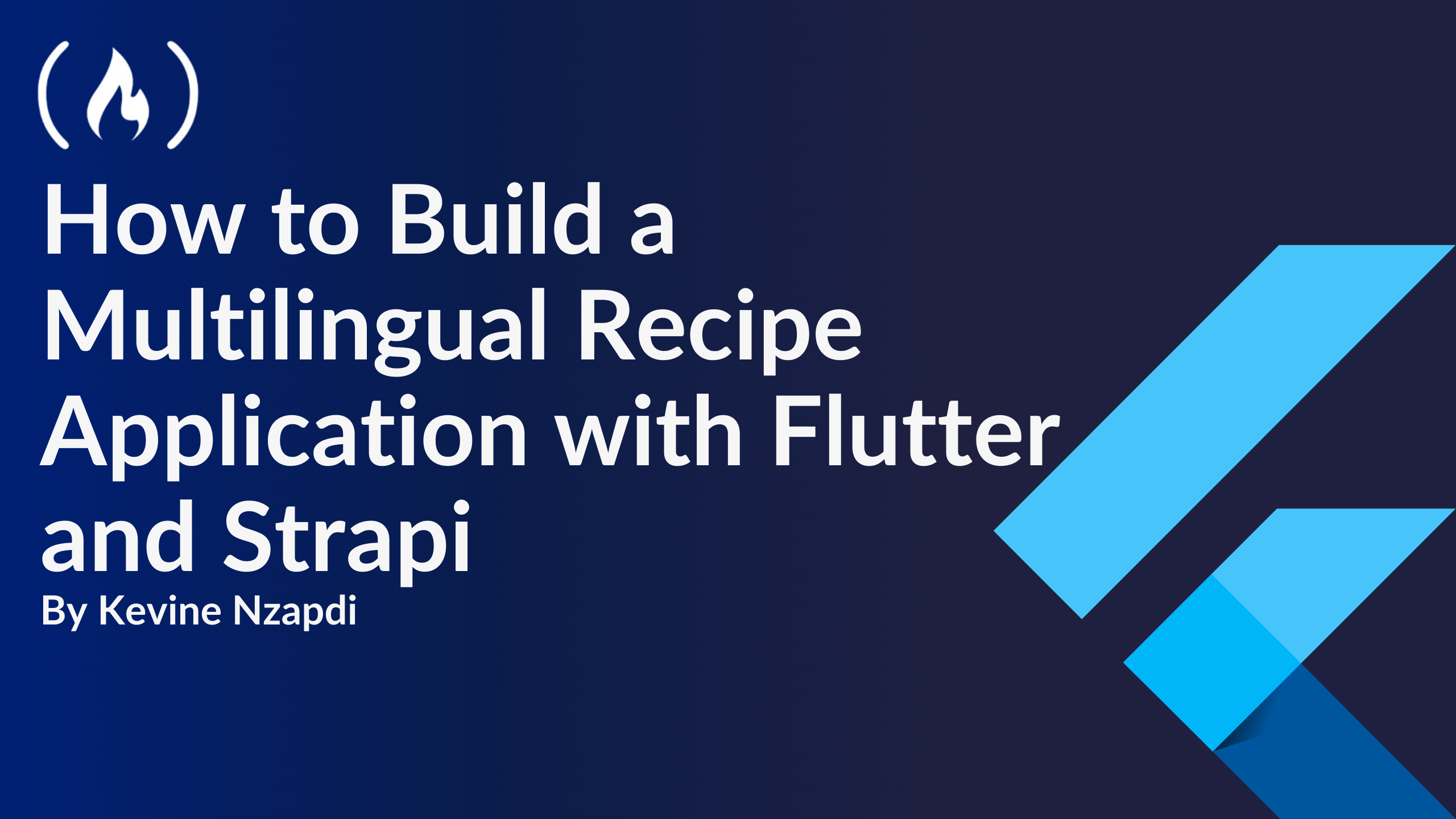
Install Packages
Install Packages 관련
In this tutorial, we’re using five main packages:
flutter_dotenv
: to manage environment variableshttp
: to handle HTTP requests and interact with Strapi REST APIshared_preferences
: persists key-value data on the device like user login tokensprovider
: for state management and updating your UI reactively when the underlying state changeseasy_localization
: for managing translations and locale data. It supports both JSON and YAML file formats for defining translations.
In your pubspec.yaml
file, add the following lines:
dependencies:
flutter:
...
flutter_dotenv: ^5.1.0
http: ^1.1.0
shared_preferences: ^2.2.2
provider: ^6.1.2
easy_localization: ^3.0.7
Then run the command below to install the packages:
flutter pub get
Add Assets
Add the path to your assets in your pubspec.yaml
file found at the root of your project:
flutter:
uses-material-design: true
assets:
- .env
- assets/translations/
- assets/images/
The translations folder contains the list of your translations while the images folder hosts the photos of your application.
Taking a look at main.dart
In the main.dart
file, you need to set up your localization, load environment variables, and a list of providers for dependency injection:
import 'package:easy_localization/easy_localization.dart';
import 'package:flutter/material.dart';
import 'package:flutter_recipe_app/screens/home.dart';
import 'package:flutter_recipe_app/screens/login.dart';
import 'package:flutter_recipe_app/screens/requestRecipe.dart';
import 'package:flutter_recipe_app/screens/signUp.dart';
import 'package:flutter_recipe_app/utils/server.dart';
import 'package:provider/provider.dart';
import 'package:flutter_dotenv/flutter_dotenv.dart';
Future<void> main() async{
// Ensure all bindings are initialized
WidgetsFlutterBinding.ensureInitialized();
await EasyLocalization.ensureInitialized();
// Load environment variables
await dotenv.load(fileName: ".env");
runApp(EasyLocalization(
supportedLocales: const [
Locale('en'),
Locale('fr', 'FR'),
Locale('ja', 'JP')],
path: 'assets/translations', //
fallbackLocale: Locale('en'),
child: MyApp(),
));
}
class MyApp extends StatelessWidget {
Widget build(BuildContext context) {
return MultiProvider(
providers: [
Provider(create: (_) => ApiService()),
],
child: MaterialApp(
title: tr('app_description'),
localizationsDelegates: context.localizationDelegates,
supportedLocales: context.supportedLocales,
locale: context.locale,
initialRoute: '/home',
routes: {
'/request': (context) => RecipeRequestScreen(),
'/login': (context) => LoginScreen(),
'/register': (context) => RegisterScreen(),
'/home': (context) => HomeScreen(), // Implement HomeScreen
},
),
);
}
}
From the code snippet above, the WidgetsFlutterBinding.ensureInitialized()
ensures that all Flutter bindings are initialized before any other operations and the EasyLocalization.ensureInitialized()
initializes the EasyLocalization package to handle translations.
Load the environment variables with dotenv.load(fileName: ".env")
to read variables from the .env
file. The runApp
function wraps the MyApp
widget with the EasyLocalization
widget, which is configured to support English (en
), French (fr_FR
), and Japanese (ja_JP
) locales. The path for translation files is set to 'assets/translations'
, and the fallback locale is set to English.
It also creates the main routes of the recipe application and sets home
as the initial route.