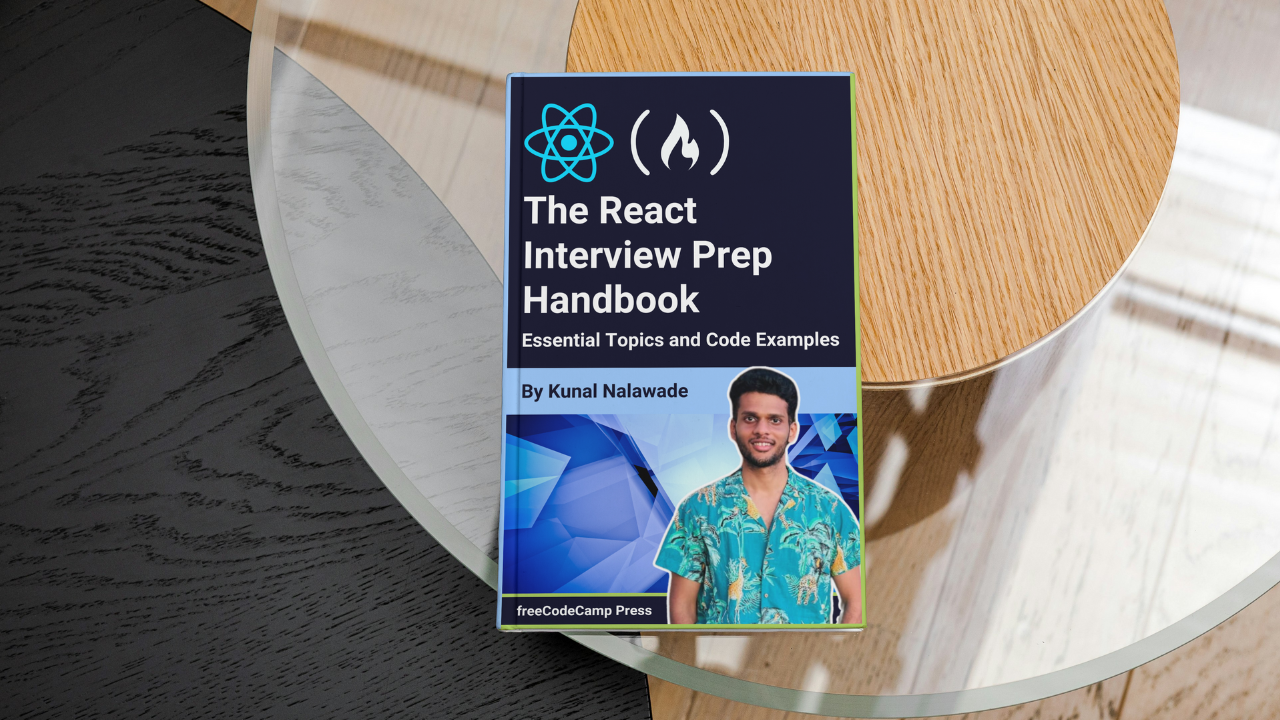
React Redux
React Redux κ΄λ ¨
Redux is a state-management library that helps manage complex application state. It is a powerful library for managing state in large React applications.
In the context of React, letβs look at the Hooks Redux provides:
useSelector
A selector function accepts a Redux state object as an argument and returns a part of that state. The useSelector
Hook is used to call the selector function. Let's take the following example:
// example state for e-commerce app
const initialState = {
users: { ... },
products: { ... },
cart: { ... },
orders: {
ordersList: [
{ id: 101, status: "Shipped" },
{ id: 102, status: "Processing" },
...
]
}
}
Let's say you want to display list of orders on a page. We can't access this state directly from the component as itβs part of the redux store. So, we use selector functions.
const selectAllOrders = (state) => state.orders.ordersList
To call this selector function, we use the useSelector
Hook:
const OrdersList = () => {
const orders = useSelector(selectAllOrders);
return (
// display orders
);
};
The main advantage of using selectors is that you get access to the Redux state object, allowing you to access any slice of the state.
useDispatch
The useDispatch
Hook returns a function that you can use to dispatch actions, such as updating state and calling APIs. This function takes an action object as an argument and performs the corresponding action. This function is known as a dispatch function.
Let's take an example. Weβll work with the same state and update one of the orderβs status:
function App() {
const dispatch = useDispatch();
const handleUpdateStatus = () => {
dispatch({type: 'ORDER_UPDATE_STATUS', payload: {
id: 102,
status: "Shipped"
}});
};
return (
<div>
<h2>Update Order Status</h2>
<button onClick={handleUpdateStatus}>Mark as Delivered</button>
</div>
);
}
Here, the action ORDER_UPDATE_STATUS
will be dispatched with the corresponding payload. This action will be mapped to a reducer that will perform the state update.
The advantage of using dispatch is that you can just specify the action type and pass the payload and the state update logic will be handled by the reducer, instead of the component itself.
Others
I have just listed two Hooks that React provides to work with Redux. However, if you are not familiar with Redux, you should check out the docs to get started.
Redux is much more than just these two Hooks. Make sure you are clear on the core concepts: store, slices, reducers, actions, selectors, dispatch. Redux Sagas is another major concept you should learn. They are mainly used for async operations.