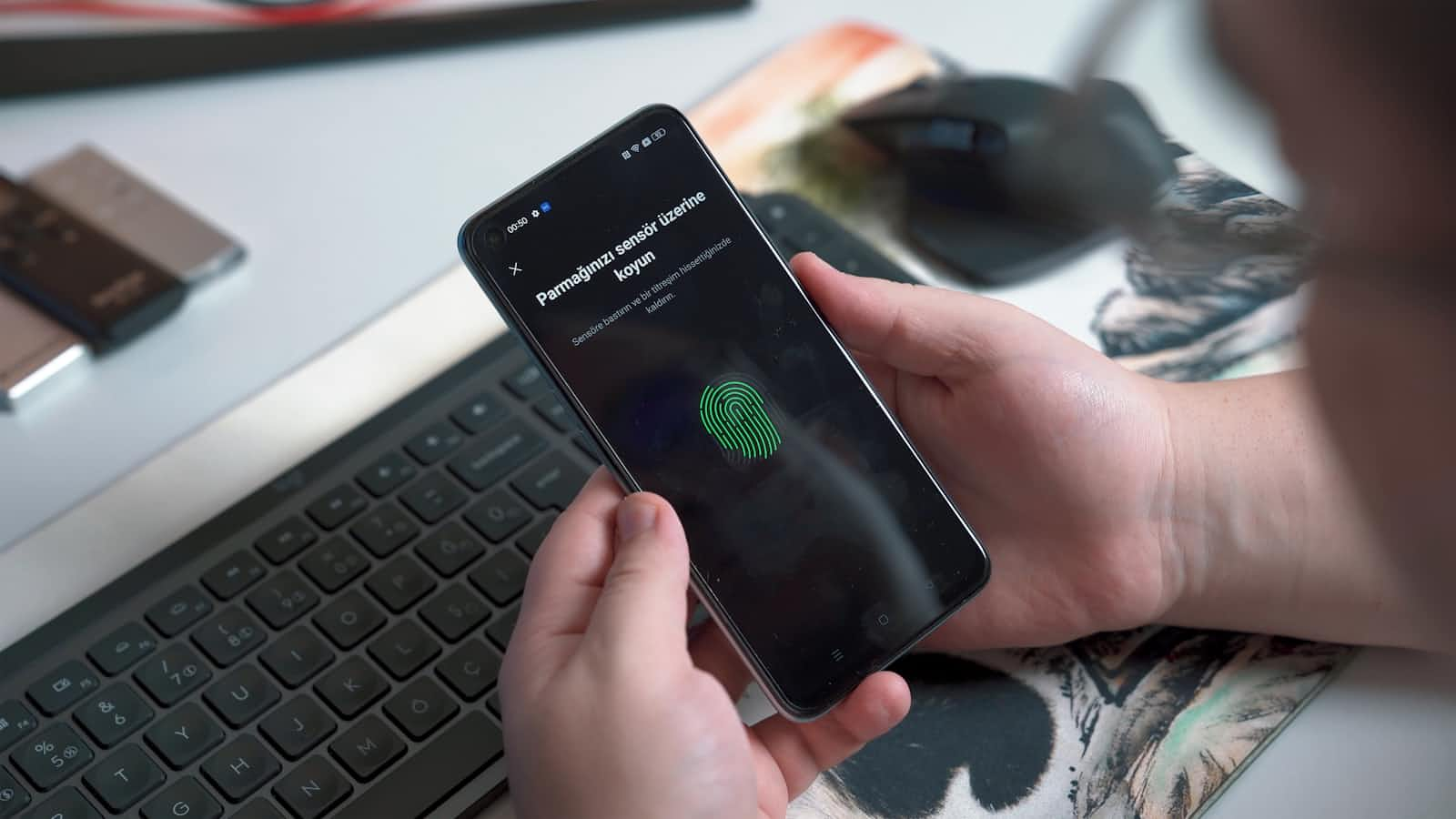
How to Authenticate Your React App Using Firebase
How to Authenticate Your React App Using Firebase 관련
Authentication is a fundamental aspect of modern web and mobile applications. It ensures that users can securely access an app while protecting their data.
Firebase, a platform developed by Google, offers a simple and efficient way to add authentication to your app.
In this article, I’ll walk you through the steps to authenticate your app using Firebase. Whether you're working on a web or mobile application, Firebase provides a straightforward way to integrate various authentication methods.
By the end of this article, you'll have a fully functional authentication system that allows users to sign up, sign in, and manage their accounts securely.
Prerequisites
Before we begin, you need to have the following:
- A Google Account: Firebase is a Google product, and you need a Google account to access the Firebase Console and use Firebase services. If you don’t have a Google account, you can create one here.
Why Use Firebase for Authentication?
Firebase Authentication provides backend services and easy-to-use SDKs to authenticate users to your app. It supports various authentication methods, including:
- Email and password authentication
- Google, Facebook, Twitter, and GitHub Authentication
- Phone Number Authentication
- Anonymous Authentication
These features make Firebase an excellent choice for developers who want to implement secure and reliable authentication without dealing with the complexities of building a custom authentication system.
Let’s get started with the setup!
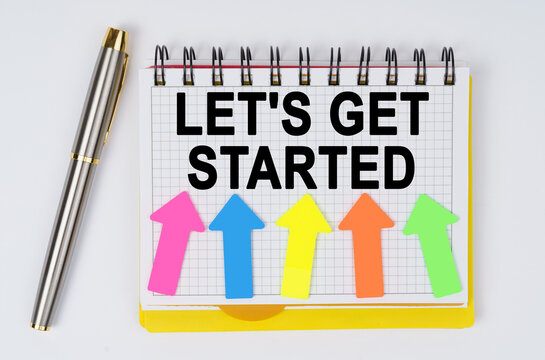
Step 1: How to Set Up a Firebase Project
Before using Firebase Authentication, you need to set up a Firebase project.
Go to the Firebase Console.
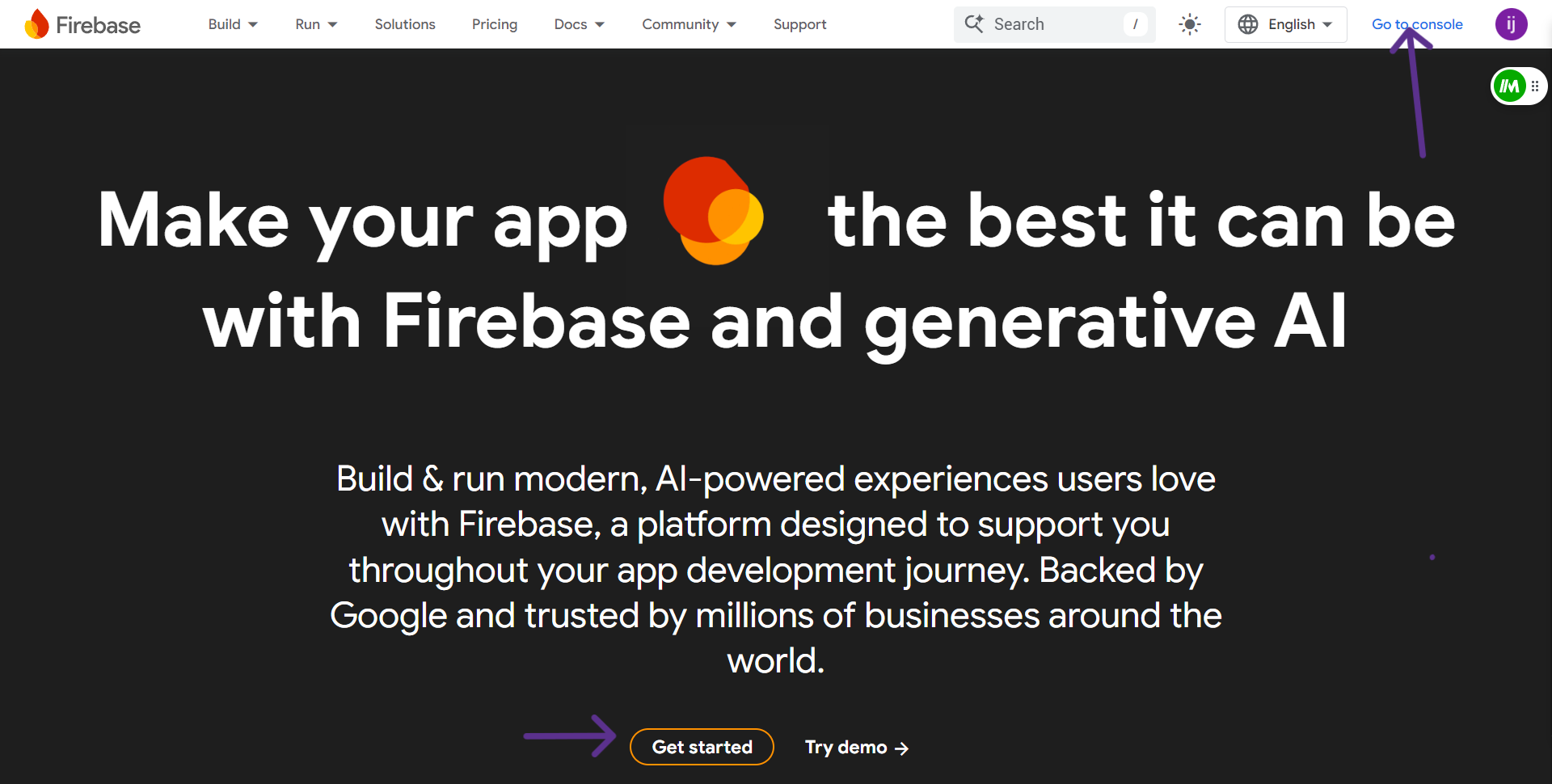
Click "Add Project" and follow the on-screen instructions to create a new project.
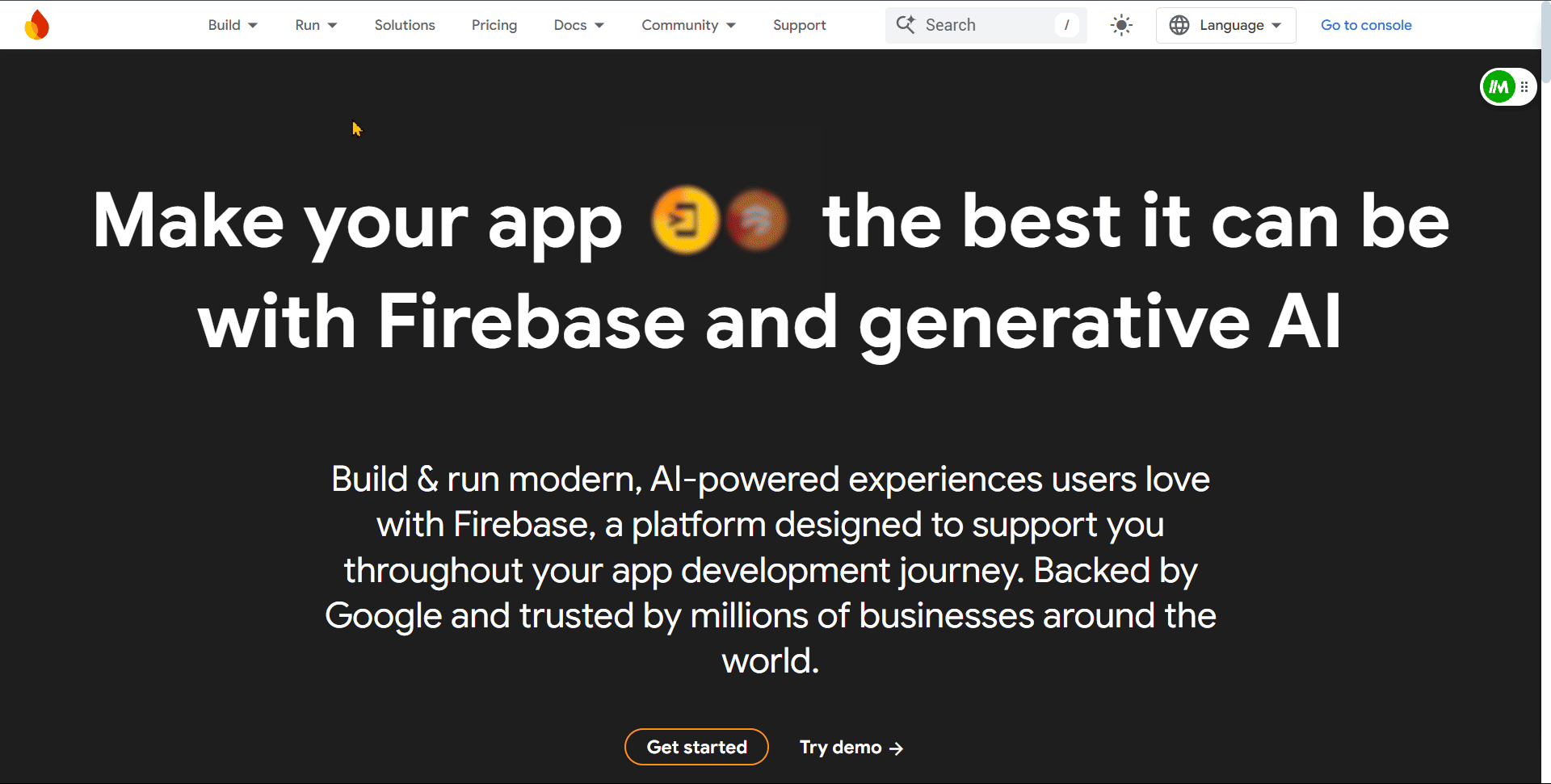
Once your project is created, you’ll be directed to the Firebase project dashboard.
- In the Firebase console, click on the "Web" icon (
</>
) to add a web app to your Firebase project. - Register your app with a nickname, and click "Register app."
- You will be provided with a Firebase SDK snippet (Software Development Kit), which you'll need to add to your app.
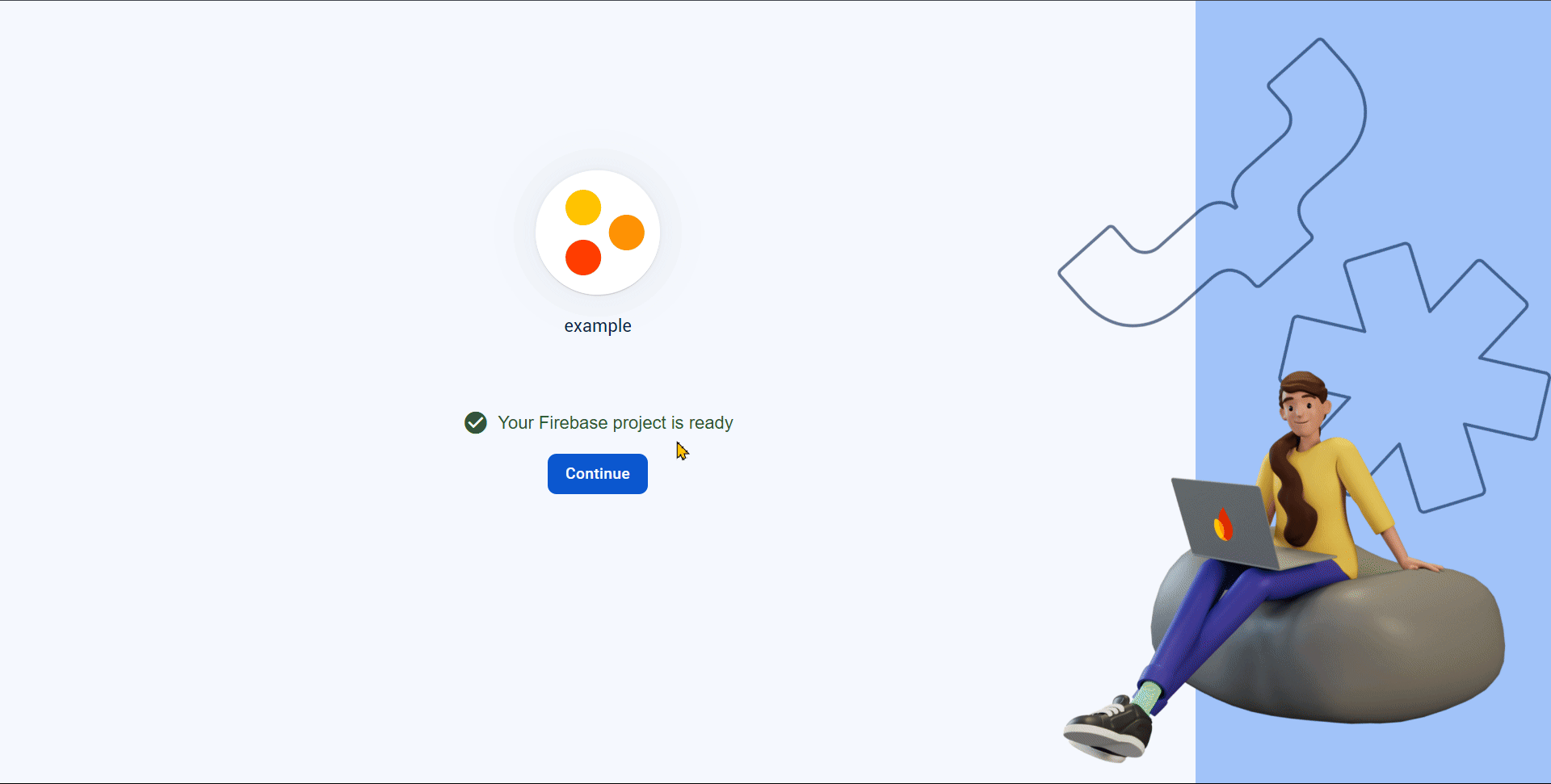
Step 2: How to Install Firebase in Your Project
To start with Firebase Authentication, you'll first need to install Firebase in your project. Here's how you can do it:
- In your code editor, open the terminal for your project.
- Run the following command to install Firebase:
npm install firebase
This command will add Firebase to your project, allowing you to use its authentication and other features.
Step 3: How to Initialize Firebase in Your App
After installing Firebase, the next step is to initialize it in your project using the configuration snippet provided in the Firebase console, commonly referred to as the Firebase SDK snippet.
To set this up:
- Create a folder named config in your project directory.
- Inside the folder, create a file called
firebase.js
. - Paste the SDK snippet you obtained from the Firebase console into the
firebase.js
file.
Here’s what your project setup should look like:
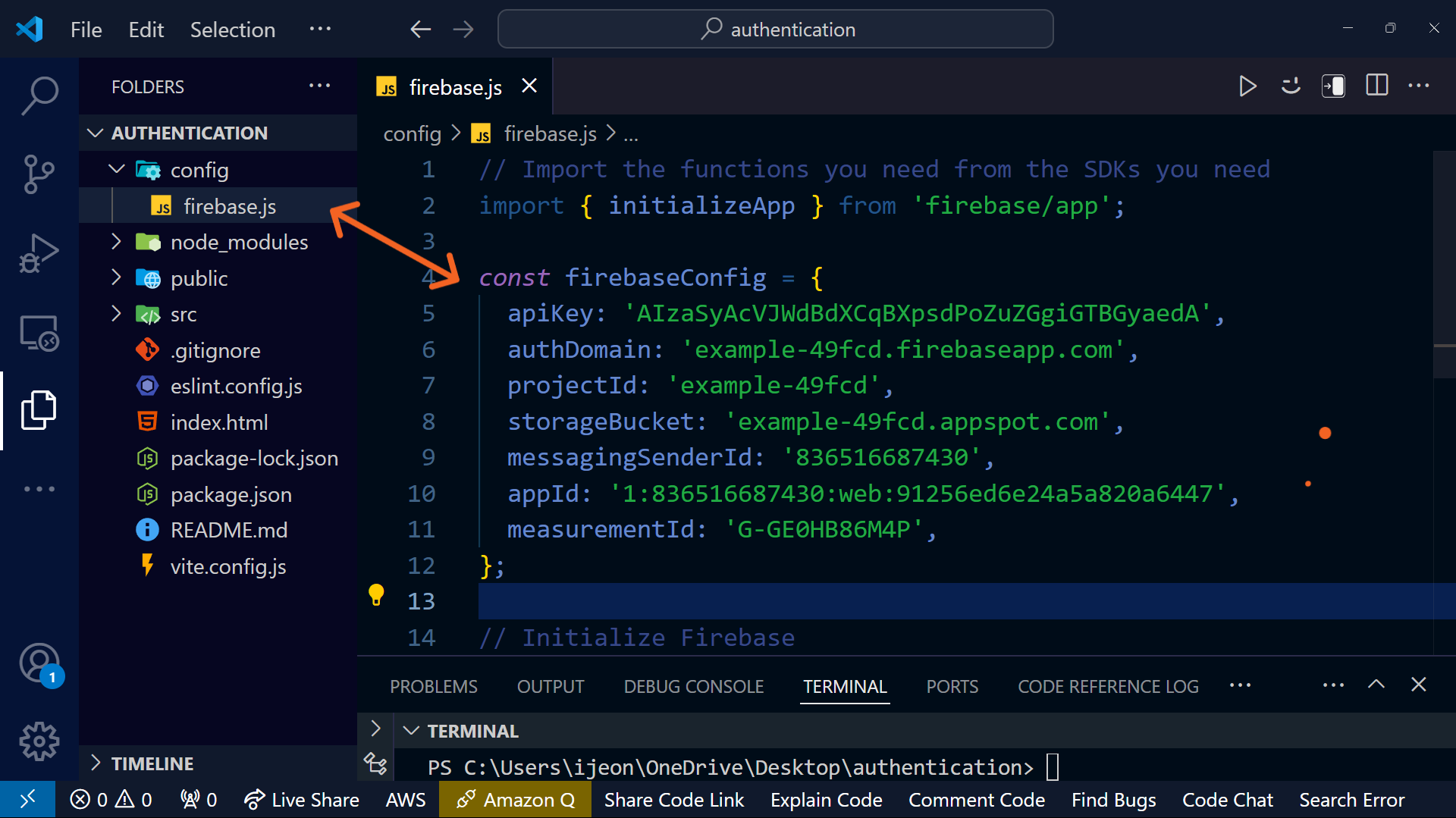
This code initializes Firebase in your app, enabling you to utilize Firebase authentication and other services, such as Firebase storage, for managing your data.
Note
Ensure you generate your unique application key for your application to function correctly.
Step 4: How to Set Up Authentication Methods
Firebase supports multiple authentication methods, like using Google, Facebook, GitHub, and so on.
But let’s set up email and password authentication as an example:
- Go to "Authentication" in the left-hand menu in the Firebase console.
- Click on the "Sign-in method" tab.
- Enable "Email/Password" under the "Sign-in providers" section.
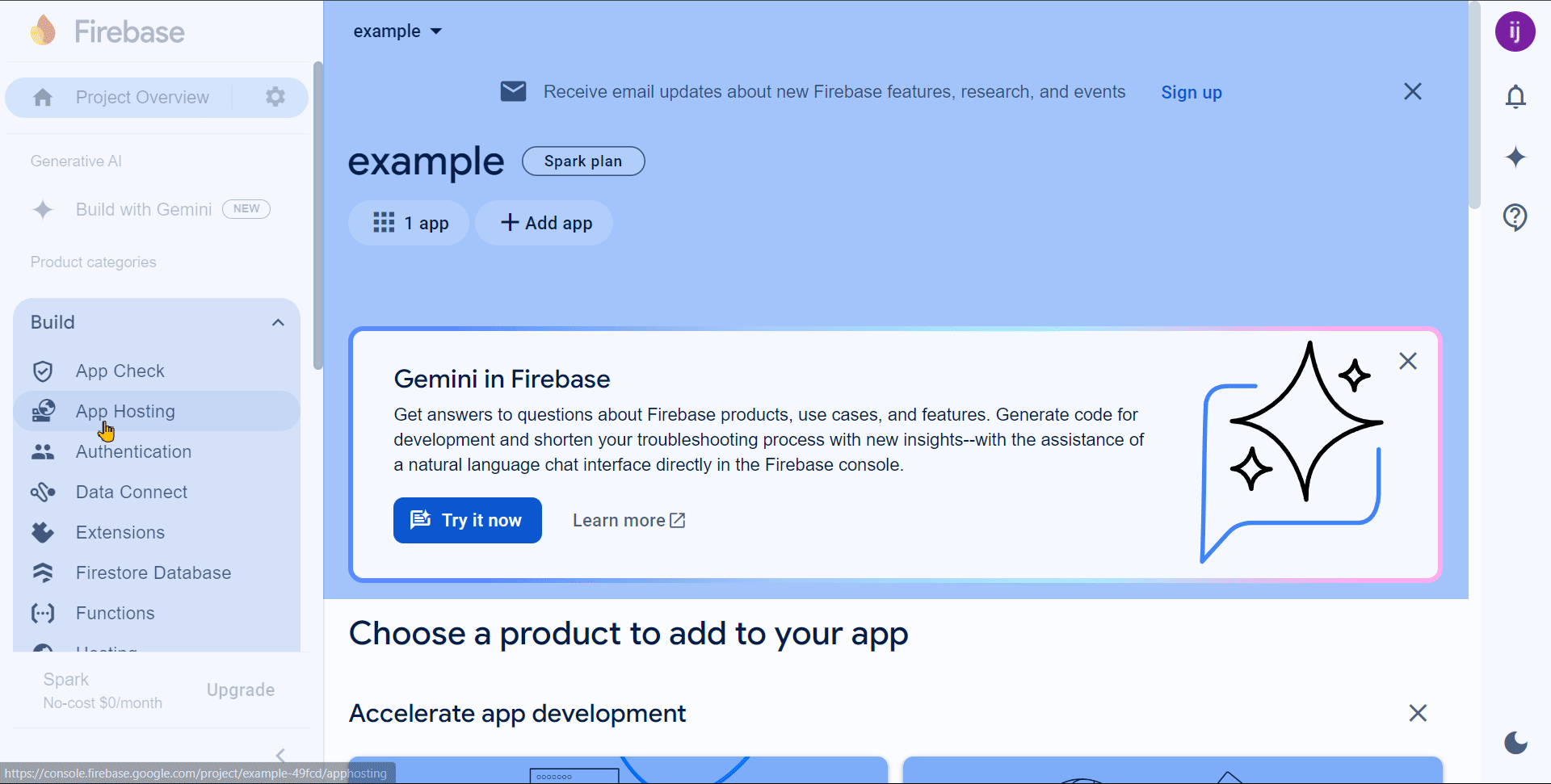
Now that you've enabled email/password authentication, you can create a sign-up and a sign-in function in your app.
Let’s create a working example of a sign-up function:
- In your project, create a file named sign-up.jsx.
- Import the function needed to create a user from Firebase. The function you'll use to create a user is
createUserWithEmailAndPassword
. - Before creating a user, make sure to import the auth instance that is initialized in firebase.js into the sign-up.jsx file.
import { auth } from '../../../config/firebase';
import { createUserWithEmailAndPassword } from 'firebase/auth';
const SignUp = () => {
// To create the user with email and password
const handleUser = async (e) => {
e.preventDefault();
try {
await createUserWithEmailAndPassword(auth, email, password);
alert('User created successfully');
} catch (err) {
console.error(err);
}
};
// ... (rest of your SignUp component)
};
In the return statement, I will use a form, so we need to import the useState()
Hook to manage and track changes in the form's input fields.
<div>
<h2>Register your Account</h2>
<form onSubmit={handleCreateUser}>
<div>
<label>Name</label>
<input
type="text"
id="name"
name="name"
value={name}
onChange={(e) => setName(e.target.value)}
/>
</div>
<div>
<label htmlFor="email">Email</label>
<input
type="email"
id="email"
name="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
/>
</div>
<div>
<label htmlFor="password">Password</label>
<input
type="password"
id="password"
name="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
/>
</div>
<div>
<label htmlFor="confirm_password" className={styles.label}>
Confirm Password
</label>
<input
type="password"
id="confirm_password"
name="confirm_password"
value={confirmPassword}
onChange={(e) => setConfirmPassword(e.target.value)}
/>
</div>
<div>
<div>
<input type="checkbox" id="terms" name="terms" className="mr-2" />
<label htmlFor="terms">
I agree to the <a href="#">Terms and Conditions</a>
</label>
</div>
</div>
<button type="submit">Register</button>
</form>
</div>
Putting all code together (Sign-up.jsx
):
import { useState } from 'react';
import { auth } from '../../config/firebase';
import { createUserWithEmailAndPassword } from 'firebase/auth';
const SignUp = () => {
const [name, setName] = useState('');
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const [confirmPassword, setConfirmPassword] = useState('');
const handleCreateUser = async (e) => {
e.preventDefault();
try {
await createUserWithEmailAndPassword(auth, email, password);
alert('User created successfully');
} catch (error) {
console.log(error);
}
};
return (
<div>
<h2>Register your Account</h2>
<form onSubmit={handleCreateUser}>
<div>
<label>Name</label>
<input
type='text'
id='name'
name='name'
value={name}
onChange={(e) => setName(e.target.value)}
/>
</div>
<div>
<label htmlFor='email'>Email</label>
<input
type='email'
id='email'
name='email'
value={email}
onChange={(e) => setEmail(e.target.value)}
/>
</div>
<div>
<label htmlFor='password'>Password</label>
<input
type='password'
id='password'
name='password'
value={password}
onChange={(e) => setPassword(e.target.value)}
/>
</div>
<div>
<label htmlFor='confirm_password'>
Confirm Password
</label>
<input
type='password'
id='confirm_password'
name='confirm_password'
value={confirmPassword}
onChange={(e) => setConfirmPassword(e.target.value)}
/>
</div>
<div>
<div>
<input
type='checkbox'
id='terms'
name='terms'
className='mr-2'
/>
<label htmlFor='terms'>
I agree to the{' '}
<a href='#'>
Terms and Conditions
</a>
</label>
</div>
</div>
<button type='submit'>Register</button>
</form>
</div>
);
};
export default SignUp;
Now that you've created the sign-up function, it's time to add a sign-in function so users can log into your app.
Here's how to create a simple sign-in function:
- In your project, create a new file named
sign-in.jsx
. - Import the initialized
auth
instance fromfirebase.js
intosign-in.jsx
. - Use the
signInWithEmailAndPassword
function from Firebase to allow users to sign in.
Here’s the structure for the sign-in function:
import { useState } from 'react';
import { auth } from '../../config/firebase';
import { signInWithEmailAndPassword } from 'firebase/auth';
const SignIn = () => {
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const handleSignIn = async (e) => {
e.preventDefault();
try {
await signInWithEmailAndPassword(auth, email, password);
alert('Signed in successfully');
} catch (error) {
console.error(error);
}
};
return (
<div>
<h2>Sign In</h2>
<form onSubmit={handleSignIn}>
<div>
<label htmlFor="email">Email</label>
<input
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
/>
</div>
<div>
<label htmlFor="password">Password</label>
<input
type="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
/>
</div>
<button type="submit">Sign In</button>
</form>
</div>
);
};
export default SignIn;
The visual display of the result from the code above both sign-up and sign-in
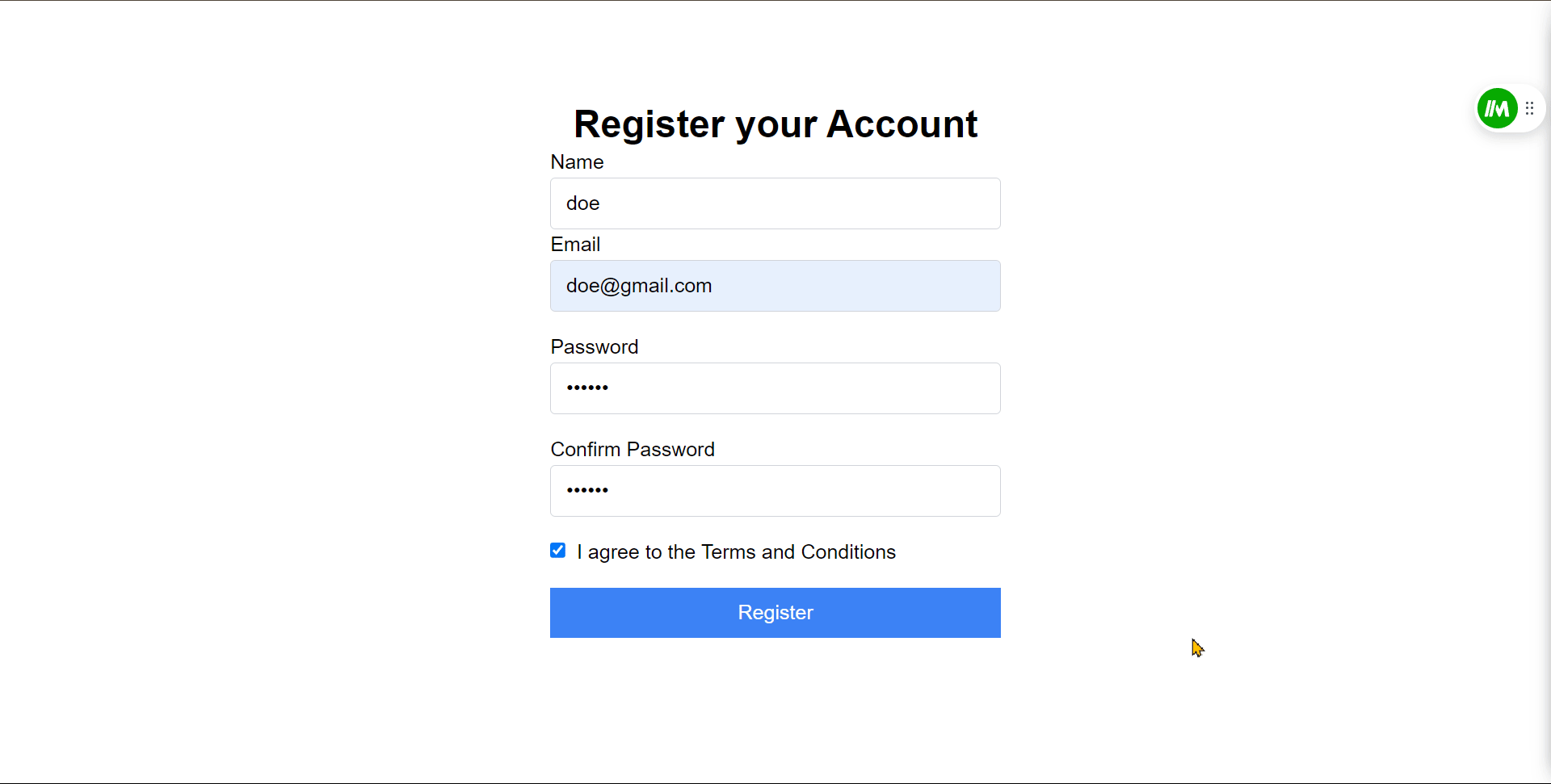
Authentication Method Using Google
As mentioned earlier, you can collect users' emails directly through a form before they use your app and other ways to authenticate the users.
To use Google authentication:
- In the Firebase console, navigate to "Authentication" in the left-hand menu.
- Click on the "Sign-in method" tab.
- Enable "Google" under the "Sign-in providers" section (for this tutorial, we'll stick with Google, though you can choose other providers).
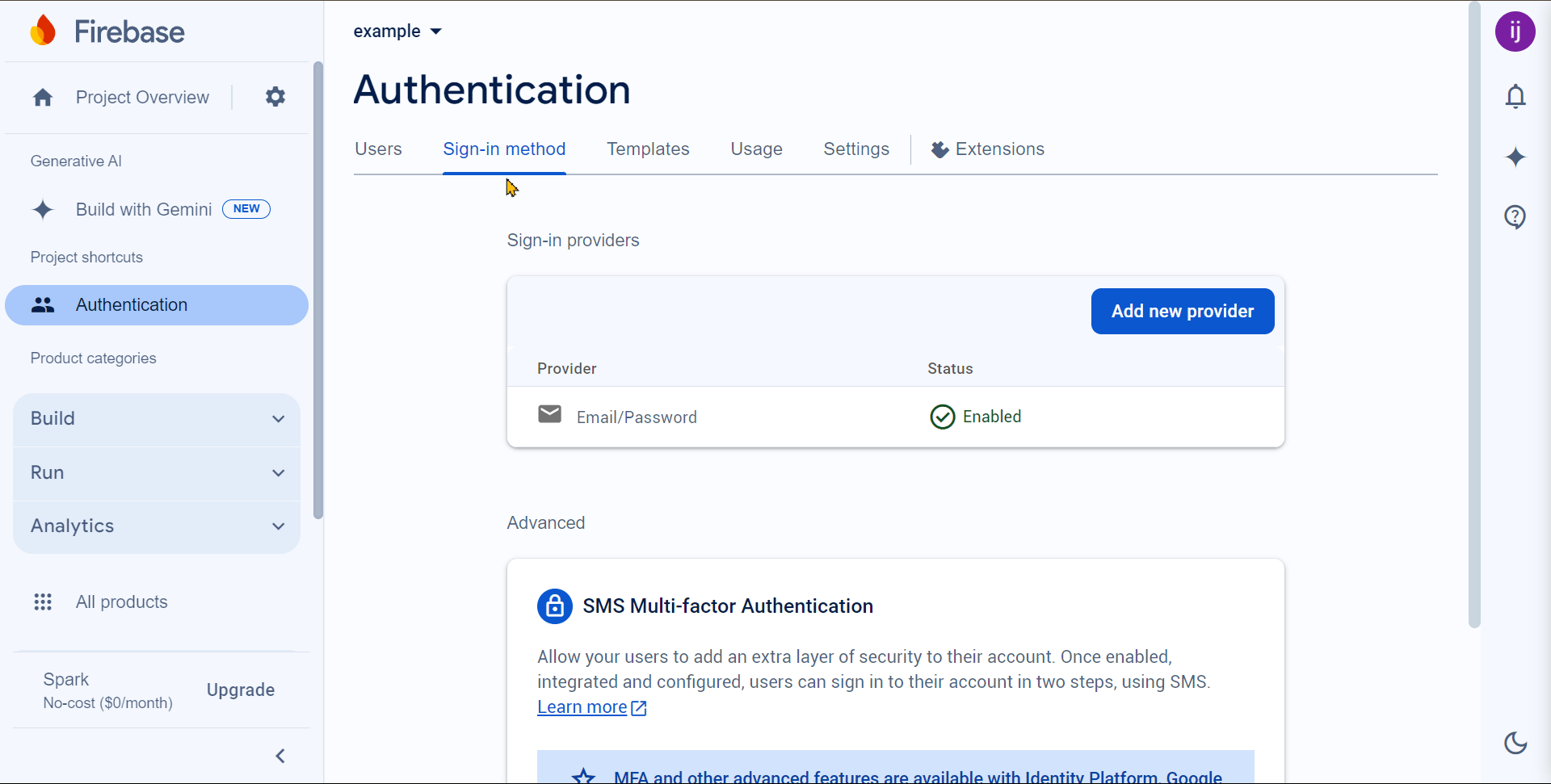
Now that you've enabled Google authentication, you can create a Google sign-up and sign-in function for your app.
Let's go through how to set up a Google sign-up function:
- First, create a file named
Google.jsx
in your project. - Import
auth
andGoogleAuthProvider
from thefirebase.js
file
// Import the functions you need from the SDKs you need
import { initializeApp } from 'firebase/app';
import { getAuth, GoogleAuthProvider } from 'firebase/auth';
const firebaseConfig = {
apiKey: ....,
authDomain: ....,
projectId:.... ,
storageBucket: .... ,
messagingSenderId: .... ,
appId: ....,
measurementId: ....,
};
// Initialize Firebase
const app = initializeApp(firebaseConfig);
export const auth= getAuth(app);
export const googleProvider = new GoogleAuthProvider(app);
- Initialize the Google provider and export it for use in other parts of your application.
import { auth, googleProvider } from './firebase'; // Adjust the path to your Firebase config file
import { signInWithPopup } from 'firebase/auth';
- Import the necessary Firebase function to authenticate a user. Use the
signInWithPopup
method to authenticate users with Google.
While there are other authentication methods available, signInWithPopup
is preferable as it keeps users within the app, avoiding the need to open a new browser tab.
const signInWithGoogle = async () => {
try {
await signInWithPopup(auth, googleProvider);
alert('Signed in successfully with Google');
} catch (error) {
console.error('Error signing in with Google', error);
}
};
- In your return statement, create a button to trigger the Google sign-in.
return (
<div>
<button onClick={signInWithGoogle}>Sign in with Google</button>
</div>
);
The visual display of the result from the code above:
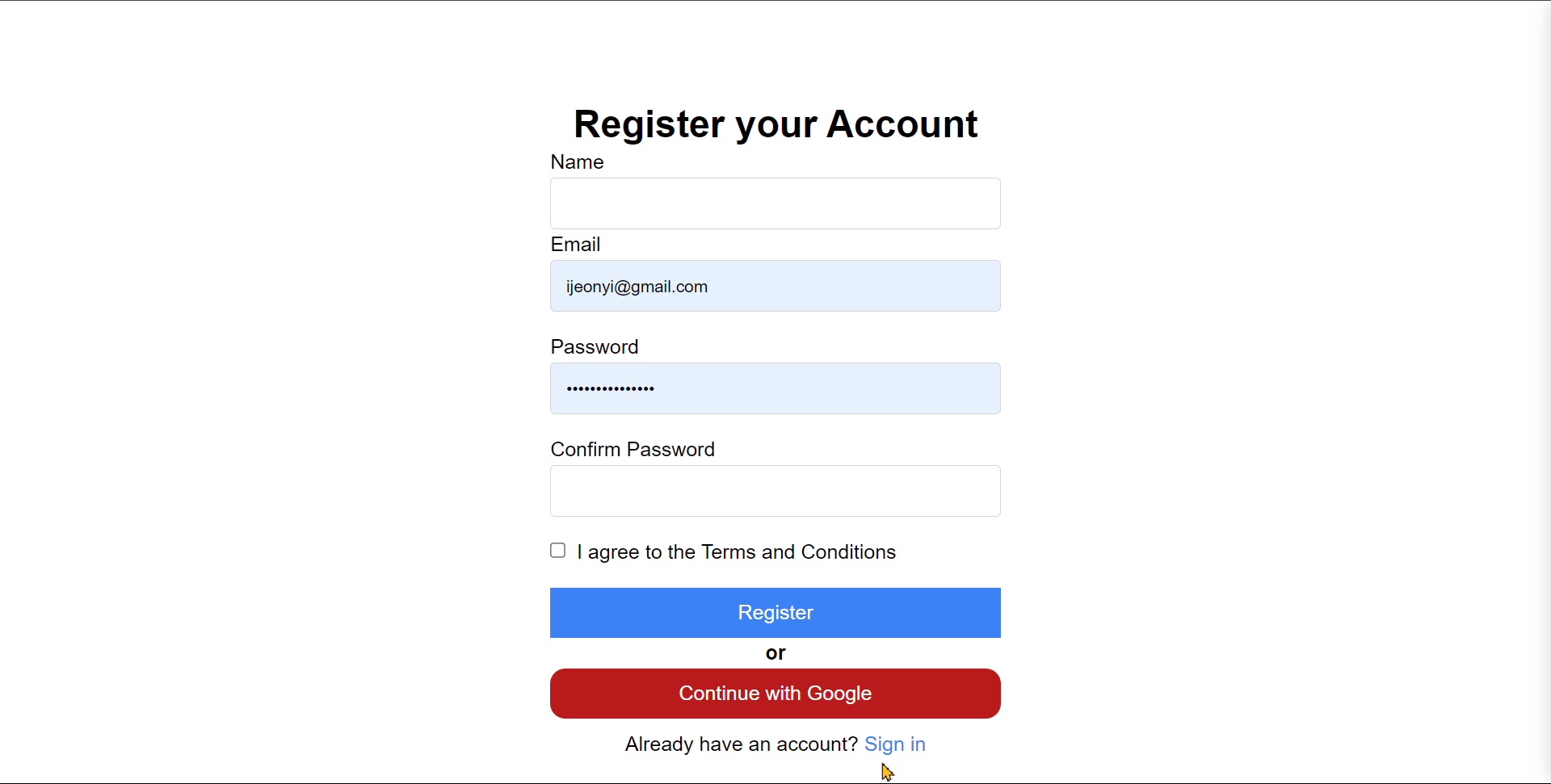
signInWithPop()
Firebase allows you to sign users out of your application easily. Here's how you can implement a sign-out function:
- First, import the
signOut
function from Firebase. - Once imported, you can call
signOut
to log the user out of the app.
Here’s a simple example:
import { auth } from './config/firebase'; // Adjust the path based on your file structure
import { signOut } from 'firebase/auth';
const handleSignOut = async () => {
try {
await signOut(auth);
alert('User signed out successfully');
} catch (error) {
console.error('Error signing out:', error);
}
};
With this function, users can easily log out of the app.
In the return statement, you'll typically have a button that triggers the handleSignOut
function when clicked.
return (
<div>
<h2>Welcome to the app!</h2>
<button onClick={handleSignOut}>Sign Out</button>
</div>
)
The visual display of the result from the code above
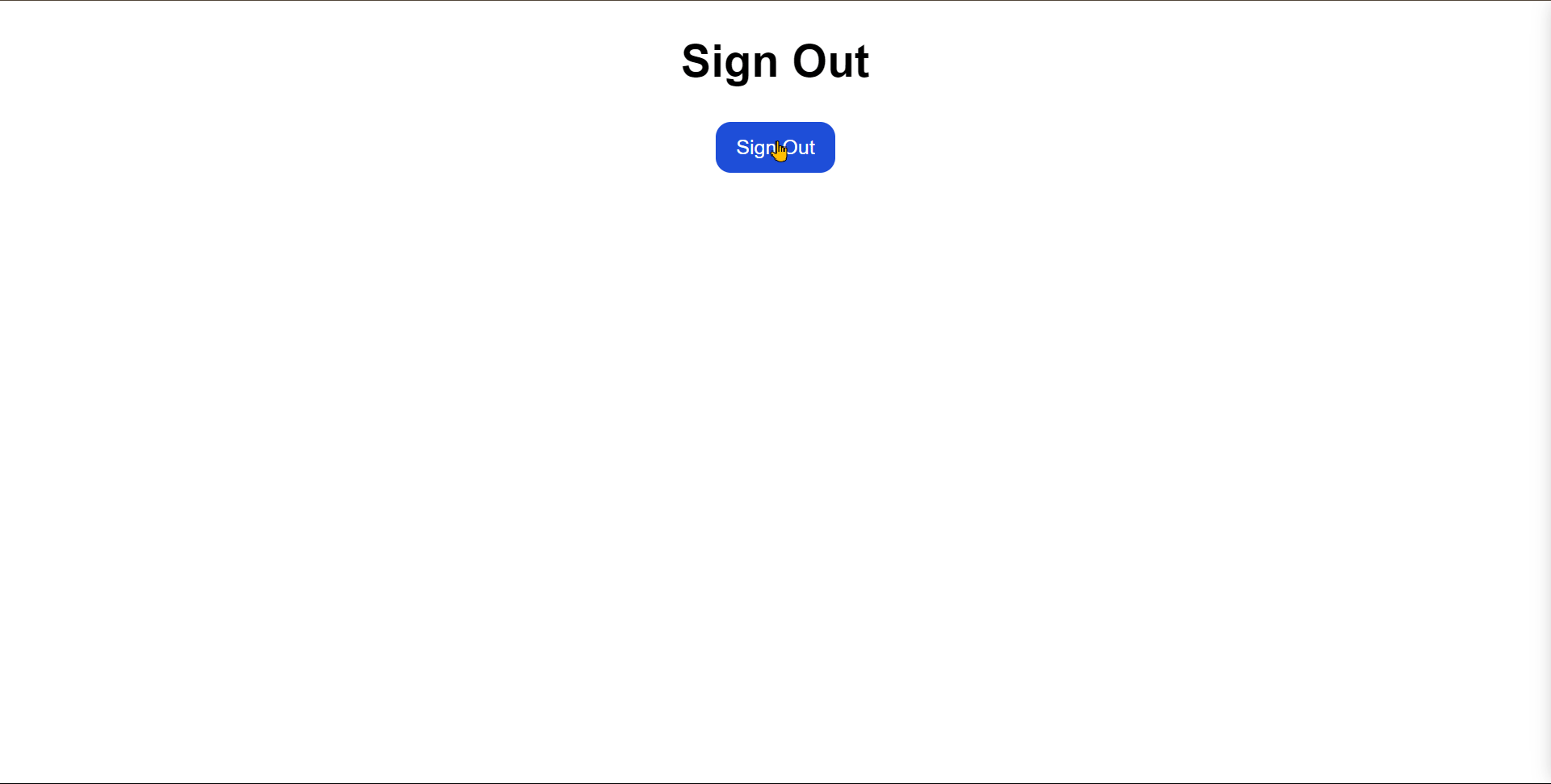
signOut()
visual displayMake sure your Firebase project is configured to handle authentication correctly, including Google sign-in, to ensure a smooth sign-in and sign-out experience.
Step 5: How to Upload to GitHub
Before pushing your project to GitHub, make sure to store your Firebase API key in an environment variable to keep it secure. This will prevent sensitive information from being exposed in your shared code.
Creating a .env
file
- At the root of your application, create a
.env
file.
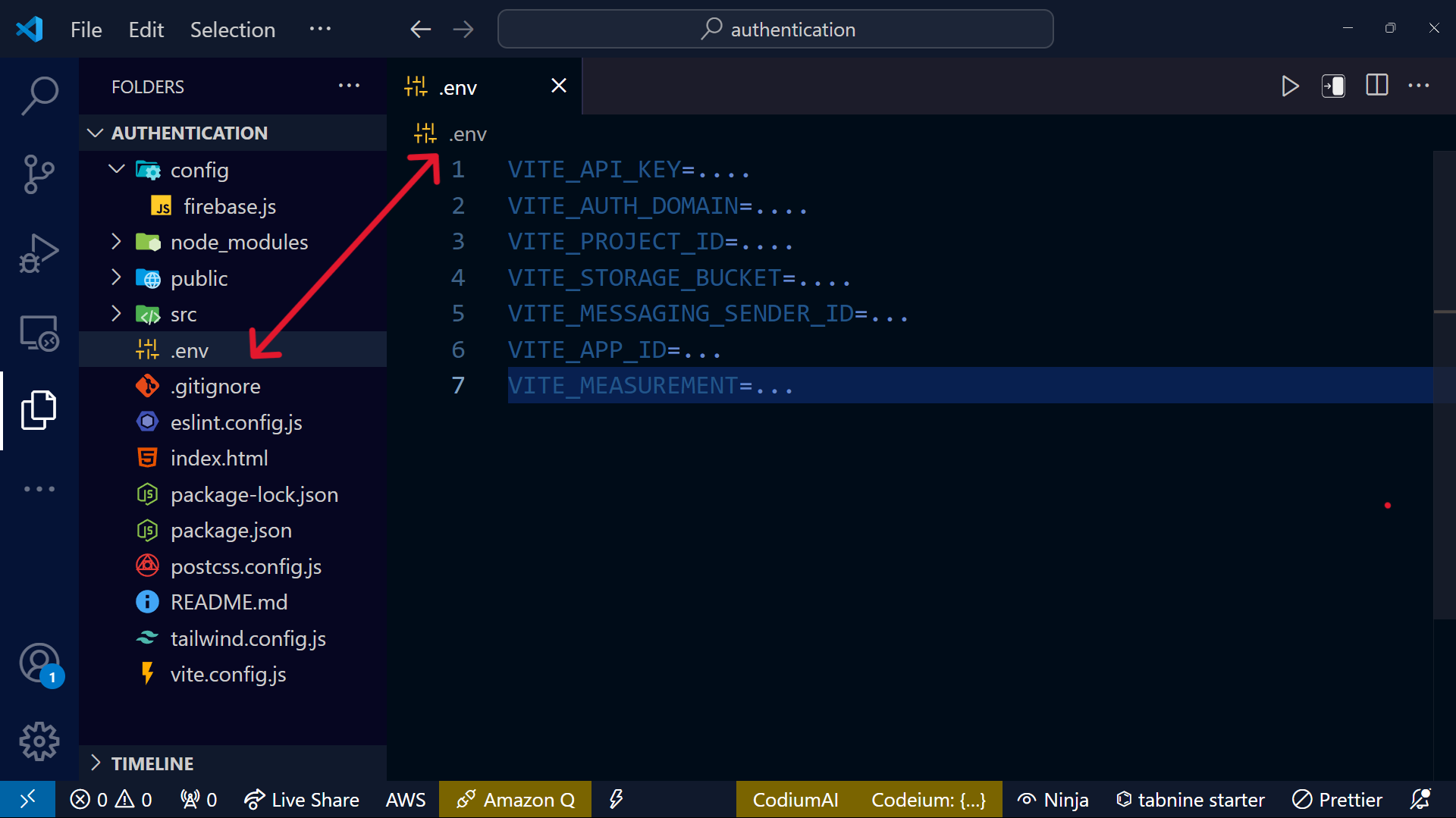
.env
file- Add your Firebase API key to the
firebase.js
file. - Use
import
orprocess.env
to access your Firebase API key. Since the app was created with Vite, I usedimport
.
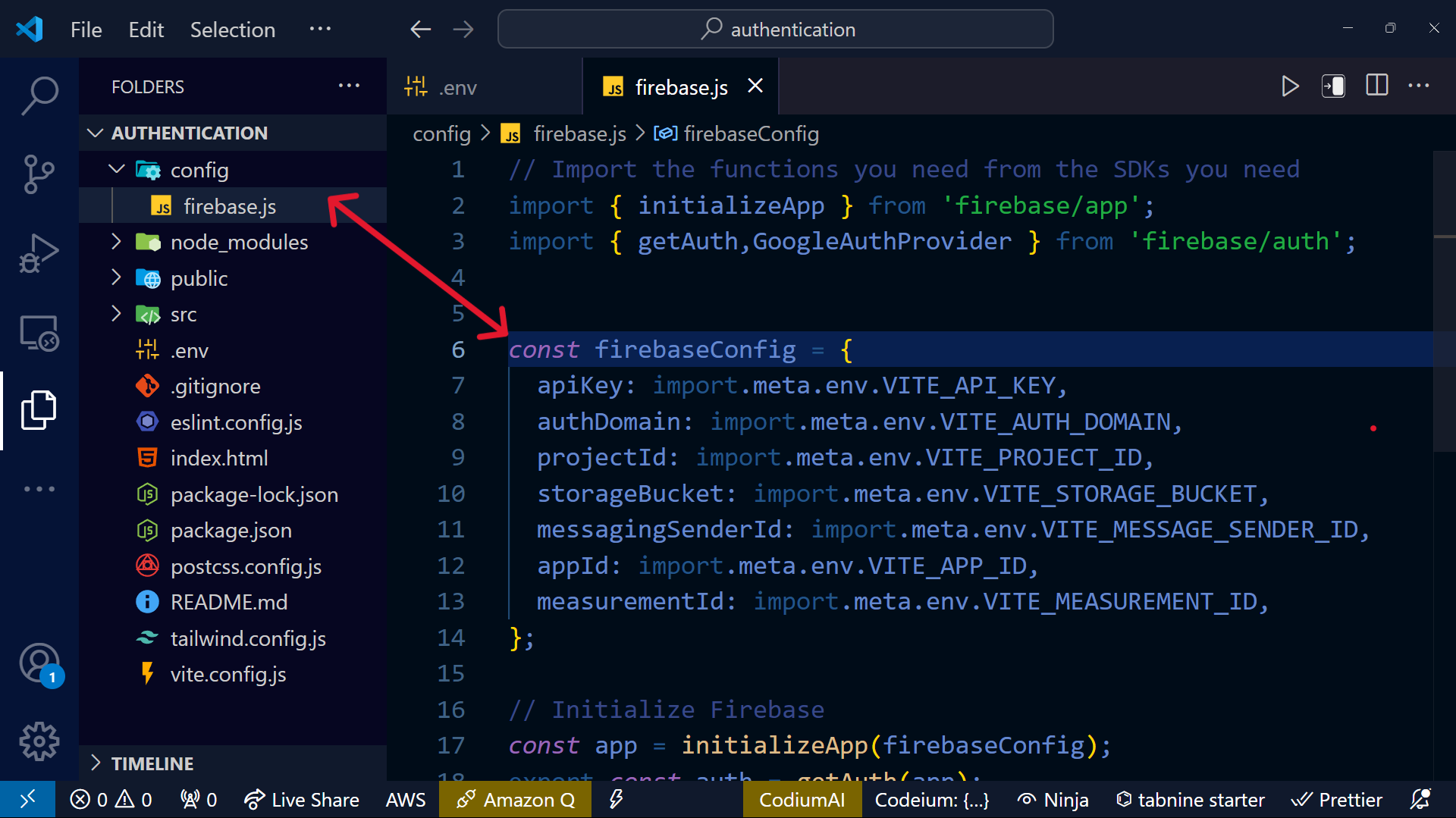
- Finally, update your .gitignore file to include the
.env
file. This step also protects other sensitive files and directories, likenode_modules
.
# Logs
logs
node_modules
.env
Conclusion
In conclusion, this guide explains how to integrate Firebase Authentication into your app. Firebase simplifies adding authentication features such as email/password and Google login.
By setting up a Firebase project, installing, and initializing it in your app, you can efficiently build secure user sign-up and sign-in functionalities without the need to start from scratch or set up a server.
If you found this article helpful, share it with others who may also find it interesting.
Stay updated with my projects by following me on Twitter (ijaydimples
), LinkedIn (ijeoma-igboagu
) and GitHub (ijayhub
).
The code I used for this tutorial article can be found on my GitHub (ijayhub/authentication-example-tutorial
).
Thank you for reading.