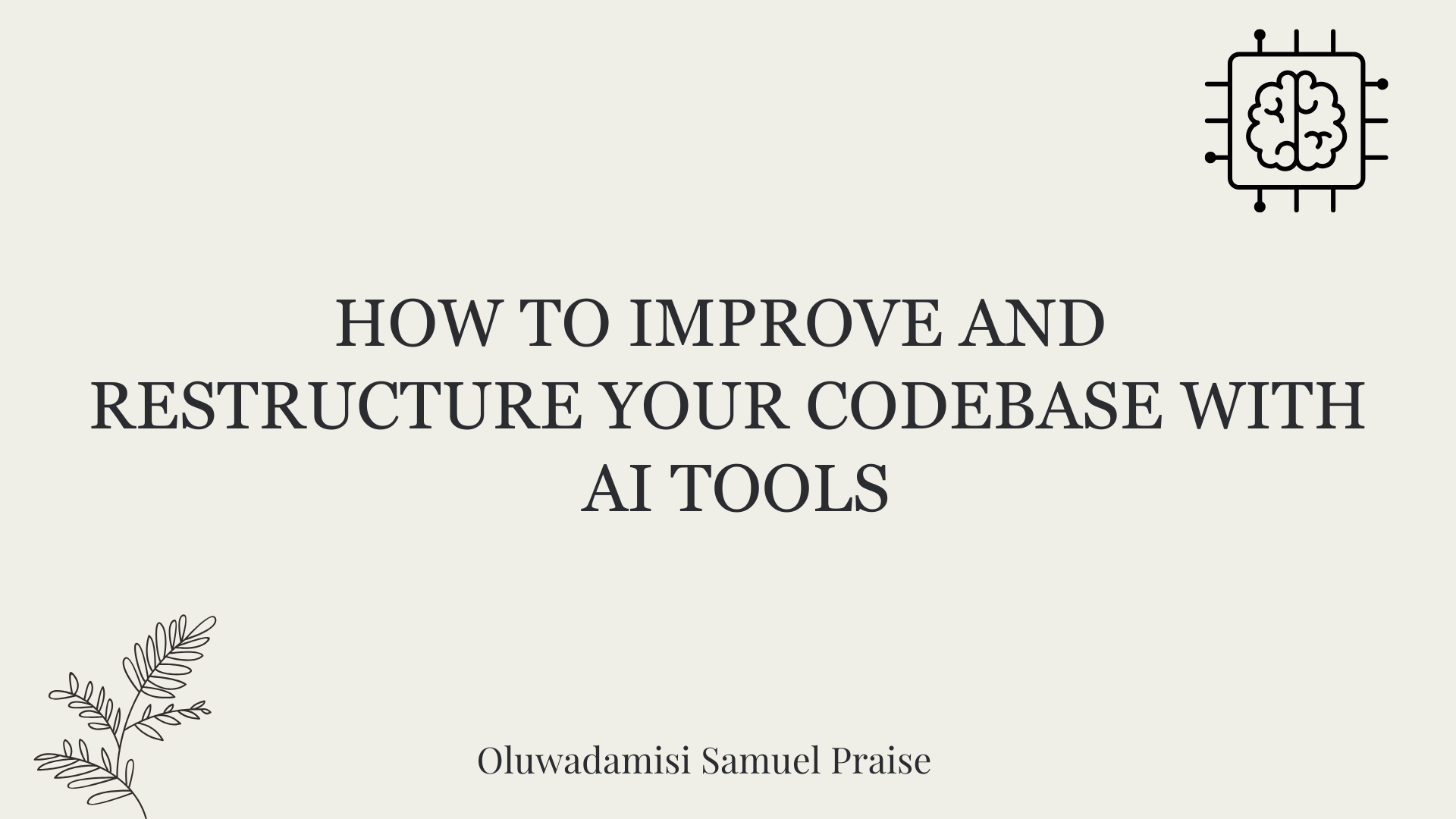
How to Improve and Restructure Your Codebase with AI Tools & Version Control
How to Improve and Restructure Your Codebase with AI Tools & Version Control 관련
A codebase can become messy and hard to manage over time. This happens because of quick fixes, outdated features, or just not enough time to clean things up.
When code becomes difficult to read or change, it slows down progress and can even cause bugs. To keep a codebase healthy and easy to work with, you’ll need to take care of it.
Improving and organizing old code can feel like a big task, but there are tools and methods that can make it easier. This guide will show how to refresh your codebase step by step which will make it simpler to work with and less likely to cause issues.
How to Review Your Code Effectively
Code reviews are essential for catching issues early, improving readability, and ensuring long-term maintainability. Reviewing your own code or someone else’s involves more than just scanning for errors – you’ll also want to make sure each part is clear, efficient, and follows good practices.
Here’s a step-by-step approach to help you review code effectively, with practical strategies, tools, and what to look for during the process.
Strategies for Effective Code Review
- Break Down the Review Process: Reviewing code all at once can be overwhelming, especially in large projects. Focus on small sections of the codebase at a time, such as individual functions or modules. This approach helps you examine each part closely and avoids missing issues that could be overlooked in a quick scan.
- Review for Clarity and Simplicity: Good code should be easy to read and understand. When reading through the code:
- Variable and Function Names: Are variable names descriptive enough to convey their purpose? Long, unclear names make code harder to follow.
- Function Length: Keep functions short and focused on one task. Long functions are harder to debug and maintain.
- Comments and Documentation: Comments should explain why something is done rather than what is happening, which should be clear from the code itself. For instance, avoid excessive commenting on trivial lines and focus on complex logic or business rules.
- Check for Code Reusability and Modularity: Look for repeated code or functions performing multiple tasks. By modularizing code, you make it easier to test, update, and reuse. In a review, look for:
- Duplicate Code: Repeated code can often be refactored into a function.
- Single Responsibility: Each function should handle one task, making it easier to maintain and update.
- Examine Error Handling and Edge Cases: Robust code should handle unexpected inputs or errors gracefully. During a review, think about potential edge cases that could break the code:
- Null or Undefined Values: Does the code check for undefined values where needed?
- Out-of-Range Errors: Ensure array indexes and calculations won’t produce errors with edge cases.
- Error Messages: Make sure error handling is meaningful, with clear error messages where applicable.
- Look for Performance Issues: Performance may not always be critical, but it’s good to check for potential bottlenecks. Look for:
- Loop Optimization: Avoid deeply nested loops or repeated work inside loops.
- Database Queries: Minimize unnecessary database calls.
- Heavy Computation in the Main Thread: Move any heavy processing outside the main application thread if possible.
- Ensure Consistency with Coding Standards: Following a consistent coding style improves readability across the team. Many teams use linters or style guides to enforce these standards. Look for:
- Code Format: Consistent indentation, spacing, and use of braces.
- Naming Conventions: Follow agreed naming conventions (camelCase, snake_case, and so on) consistently.
Tools to Assist with Code Reviews
There are a number of tools out there that can help streamline your code reviews, whether you’re checking your own code or collaborating with others:
1. Linters (like ESLint and Pylint)
Linters check for syntax errors, code smells, and style guide violations. They are especially useful for catching minor issues, like inconsistent formatting or unused variables. We will discuss ESLint more in an upcoming section.
npx eslint src/
2. Static Analysis Tools (like SonarQube)
These tools analyze code for deeper issues like security vulnerabilities, code duplication, and complex functions that might need refactoring.
sonar.projectKey=my_project
sonar.sources=src
sonar.host.url=http://localhost:9000
sonar.login=my_token
3. Automated Testing Tools
Running tests can verify that code changes don’t introduce new bugs. Use testing frameworks like Jest for JavaScript, PyTest for Python, or JUnit for Java to confirm your code behaves as expected.
Example of Refactoring During Code Review
Let’s say you encounter a long function with multiple responsibilities. The goal is to split it into smaller, focused functions. Here’s how you can do that:
// Original: A single function that handles everything
function processOrder(order) {
// Calculate total price
let total = 0;
order.items.forEach(item => {
total += item.price * item.quantity;
});
// Apply discount
if (order.discountCode) {
total = total * 0.9; // 10% discount
}
// Send order confirmation email
sendEmail(order.customerEmail, 'Order Confirmation', 'Your order total is ' + total);
}
// Improved: Break into smaller functions for readability and reusability
function calculateTotal(order) {
return order.items.reduce((sum, item) => sum + item.price * item.quantity, 0);
}
function applyDiscount(total, discountCode) {
return discountCode ? total * 0.9 : total;
}
function sendConfirmationEmail(email, total) {
sendEmail(email, 'Order Confirmation', 'Your order total is ' + total);
}
function processOrder(order) {
let total = calculateTotal(order);
total = applyDiscount(total, order.discountCode);
sendConfirmationEmail(order.customerEmail, total);
}
Breaking down the process into smaller functions makes the code cleaner, more readable, and easier to test. Each function now has a single responsibility, which helps reduce bugs and makes future updates simpler.
How to Identify Technical Debt and Problem Areas in Code
Technical debt refers to the accumulation of issues within a codebase that arise when development shortcuts are taken, often to meet tight deadlines or speed up releases. While these shortcuts may enable quicker progress initially, they lead to complications down the line.
Technical debt requires proactive management. If you leave it unchecked, it can reduce productivity, create bugs, and slow down development.
Think of technical debt like financial debt: taking on debt can be helpful in the short term, but failing to address it or pay it down will lead to greater challenges.
Common causes of technical debt include:
- Rushed development cycles: When teams prioritize quick delivery over thorough design and testing, they may produce incomplete or hastily written code.
- Lack of planning for future changes: Sometimes, code is written without accounting for scalability, leading to issues as the project grows.
- Insufficient documentation or testing: Without proper documentation and test coverage, codebases become difficult to understand and validate over time.
- Outdated frameworks and dependencies: When frameworks or libraries aren’t updated, they can become incompatible with newer components or security standards, introducing risk and hindering future updates.
Types of Technical Debt
Technical debt manifests in different ways. Here are some common examples:
Repeated code across multiple places within a project can lead to inconsistencies, as fixing an issue or updating a feature in one area may not carry over to others. Refactoring duplicate code into reusable functions or components is an effective way to reduce this debt.
Example: In a web application, you might find similar code for user authentication scattered across different modules. Instead, centralizing this logic into a single authentication module ensures consistent updates.
Using old libraries or frameworks can slow down development and introduce security vulnerabilities. Over time, dependencies may lose support or become incompatible with new features, making them costly to maintain.
Solution: Regularly update libraries and frameworks, and monitor for deprecations or vulnerabilities. This can be streamlined by using dependency managers, which help check for updates and security patches.
Large, complex functions that handle multiple tasks are difficult to understand, test, and modify. Known as “God functions,” these make debugging cumbersome and increase the risk of introducing new bugs.
Solution: Follow the Single Responsibility Principle (SRP). This means that each function or method should accomplish one task. Breaking down large functions into smaller, focused units makes the code easier to read and test.
Example: Instead of having a single processUserRequest
function that handles authentication, logging, and database queries, split it into three functions: authenticateUser
, logRequest
, and queryDatabase
.
Code that lacks proper error handling can lead to bugs and unexpected behavior, especially in larger systems. Without clear error messages, diagnosing and fixing issues can be challenging.
Solution: Include comprehensive error handling and ensure that meaningful error messages are displayed. Log errors in a way that helps developers track and diagnose issues.
Hardcoding values directly into code makes it difficult to adjust settings without modifying the source code. For example, using fixed URLs or credentials directly in the codebase can create security risks and maintenance headaches.
Solution: Use configuration files or environment variables to store values that might change. This improves security and allows for easy updates.
Documentation and testing are often neglected when time is short. But without proper documentation and test coverage, the code becomes challenging to understand and validate, slowing down development and increasing the risk of bugs.
Solution: Implement test-driven development (TDD) or include time in the development cycle for creating documentation and writing tests. Aim for at least basic test coverage for critical paths and functions.
How to Identify and Manage Technical Debt
Identifying technical debt is crucial if you want to address and improve it. Here are some strategies you can follow:
- Code Reviews: Regular peer reviews help uncover areas of potential debt. In reviews, team members can flag complex code, lack of tests, or unclear logic, helping address these issues early.
- Automated Static Code Analysis: Tools like SonarQube, Code Climate, and ESLint (for JavaScript) analyze codebases for code smells, vulnerabilities, and complexity. They’re effective for spotting issues like duplicate code, long functions, and outdated dependencies.
- Regular Refactoring Sessions: Scheduling dedicated time for refactoring allows the team to improve existing code quality. During these sessions, focus on simplifying code, breaking down large functions, and removing duplicates.
- Technical Debt Backlog: Track technical debt items in a backlog, prioritizing them alongside feature development. This backlog helps balance feature work with debt reduction and keeps everyone aware of existing debt.
How to Deal with Technical Debt in Code
Here’s a practical example to demonstrate how refactoring can help address technical debt, specifically by removing code duplication.
Example: Removing Duplicate Code
Let’s say we have two functions that send different types of emails but use repeated code:
# Duplicate code example
def send_welcome_email(user):
send_email(user.email, "Welcome!", "Thanks for joining!")
def send_password_reset_email(user):
send_email(user.email, "Password Reset", "Click here to reset your password.")
Each function has a similar structure, so refactoring can make the code cleaner and reduce duplication.
# Refactored code
def send_email_to_user(user, subject, message):
send_email(user.email, subject, message)
# Use the refactored function
send_email_to_user(new_user, "Welcome!", "Thanks for joining!")
send_email_to_user(existing_user, "Password Reset", "Click here to reset your password.")
This example demonstrates how consolidation can reduce repetition and make the code more flexible.
How to Avoid Technical Debt
Proactively managing technical debt helps reduce it over time. Here are ways to avoid accumulating more debt:
- Establish Code Standards: Create and enforce coding standards within the team. Consistent practices reduce complexity, improve readability, and make it easier to identify issues early.
- Refactor Regularly: Rather than waiting for debt to accumulate, make minor improvements during routine work. A “leave it better than you found it” approach ensures code quality remains high over time.
- Encourage Thorough Testing: Strong test coverage identifies potential problems early, reducing the likelihood of code with hidden issues. Testing tools like Jest for JavaScript or PyTest for Python make it easy to add tests to each function and module.
- Plan for Scalability: Think about future needs when designing code. Avoid shortcuts that might restrict scalability and performance as the application grows.
- Limit Workarounds and Temporary Fixes: If temporary fixes are necessary, document them and prioritize removing them as soon as possible. Keeping track of these “quick fixes” ensures they don’t become long-term issues.
How to Measure Code Quality with Code Analysis Tools
Code quality tools can help you find issues that might not be obvious. They can point out things like unused variables, code that’s hard to read, or security problems. Popular tools include ESLint
for JavaScript
, Pylint
for Python
, and SonarQube
for different programming languages.
Here’s how to set up a simple code check with ESLint:
npm install eslint --save-dev
npx eslint --init
This command will prompt you to answer a few configuration questions. You can choose your preferred style guide and select a few options about your environment and file format.
Here’s a sample JavaScript file (example.js
) with a few common issues:
//
var x = 10; // Unused variable
let y = 5;
const z = 'Hello World'
function calculateSum(a, b) {
return a + b
}
calculateSum(3, 4);
// Missing semicolon and inconsistent indentation
if (y > 3) {
console.log("Y is greater than 3")
}
npx eslint example.js
After running this command, ESLint will analyze example.js
and report any issues based on the configured rules.
ESLint provides detailed feedback about the issues it detects:
/path/to/example.js
1:5 warning 'x' is assigned a value but never used no-unused-vars
3:12 error Missing semicolon semi
6:25 error Missing semicolon semi
10:1 error Expected indentation of 4 spaces but found 3 indent
11:26 error Missing semicolon semi
✖ 5 problems (4 errors, 1 warning)
Here’s a breakdown of each issue detected by ESLint:
- Unused Variable: ESLint identifies that
x
is declared but never used (no-unused-vars
rule). - Missing Semicolons: ESLint flags lines where semicolons are missing at the end of statements (
semi
rule). - Inconsistent Indentation: ESLint notices that line 10 doesn’t follow consistent indentation (
indent
rule).
Based on ESLint’s feedback, here’s the corrected code:
// example.js
let y = 5;
const z = 'Hello World';
function calculateSum(a, b) {
return a + b;
}
calculateSum(3, 4);
if (y > 3) {
console.log("Y is greater than 3");
}
- We removed the unused variable
x
. - We added missing semicolons.
- And we adjusted indentation for consistent spacing.
After making these changes, you can run npx eslint example.js
again to confirm that there are no remaining issues. ESLint will return no output if everything is now clean, confirming that the code adheres to the configured standards.
Additional Tip: Auto-Fixing with ESLint
ESLint can automatically fix some issues for you. To do this, use the --fix
flag:
npx eslint example.js --fix
This command will automatically correct issues like indentation, unused variables, and missing semicolons where possible. But it’s important to review the changes to ensure they align with your intended functionality.
Reviewing code, spotting technical debt, and using quality tools help keep the codebase healthy. If you follow these steps, your project will be easier to manage and less likely to break.
AI Tools to Help You Improve Your Code
Using AI tools to restructure code makes improving code quality much faster and easier. These tools help find issues, suggest changes, and can even automate some parts of the refactoring process.
I'll share some AI tools that can help you with code analysis, refactoring, and dependency management, based on my own experience and what I've found useful.
Best AI Tools for Code Restructuring
AI-powered tools are becoming more common, and they offer different ways to boost code quality and simplify refactoring. Here are some I've found helpful:
1. GitHub Copilot
GitHub Copilot is like a coding assistant that provides smart suggestions as you write code. It can complete code snippets, suggest new functions, and help rework existing code to make it more efficient. I’ve found it useful for writing repetitive code blocks or coming up with quick refactorings.
For example, let’s say you need to rewrite a function to be more efficient:
# Original function that checks if a number is prime
def is_prime(n):
if n < 2:
return False
for i in range(2, n):
if n % i == 0:
return False
return True
GitHub Copilot might suggest optimizing the function like this:
# Optimized version suggested by Copilot
def is_prime(n):
if n < 2:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
The updated version checks factors only up to the square root of n
, making it faster for large numbers.
2. QodoGen
QodoGen provides automated suggestions for refactoring and can detect common code issues, like unused variables or large functions doing too many tasks. It also helps split complex code into smaller, more manageable pieces and can explain sections of the code base or the entire codebase which will facilitate the restructuring process.
This tool is capable of doing this because, unlike other AI assistants and general purpose code generation tools, Qodo focuses on code integrity, while generating tests that help you understand how your code behaves. This can help you discover edge cases and suspicious behaviors, and make your code more robust.
For example, if you have a function handling multiple tasks, QodoGen might suggest breaking it down:
# Before refactoring
def handle_user_data(user_data):
validate_data(user_data)
save_to_database(user_data)
send_welcome_email(user_data)
# After refactoring
def handle_user_data(user_data):
validated_data = validate_data(user_data)
save_data(validated_data)
notify_user(validated_data)
Separating the steps makes the code easier to maintain and test.
3. ChatGPT for Code Assistance*
ChatGPT can act as a helpful companion when working on code restructuring tasks. Arguably the most used coding assistant, it provides advice on refactoring strategies, explains how to implement changes, or offers example snippets. It’s like having an expert to consult whenever you need guidance or ideas.
For instance, if you’re unsure how to optimize a function or restructure a class, ChatGPT can provide sample code or describe best practices. You can also ask it for help with understanding errors or fixing specific problems in your code.
Just make sure you double-check the code it provides (same goes for all these AI assistants) as it can hallucinate and make mistakes.
Automated Tools for Refactoring and Analysis
AI tools not only assist with writing code but also with analyzing it for quality improvements:
1. SonarQube
SonarQube scans the code to detect bugs, vulnerabilities, and code smells. It generates reports with suggestions on what to fix, helping maintain a healthy codebase.
# Sample SonarQube configuration
sonar.projectKey=my_project
sonar.sources=src
sonar.host.url=http://localhost:9000
sonar.login=my_token
2. ReSharper
This tool integrates with Visual Studio and offers automatic refactoring options. It highlights code that can be simplified or cleaned up and suggests ways to optimize the codebase.
3. DepCheck for Dependency Management
AI tools like DepCheck help find unused dependencies in JavaScript projects, keeping package files clean.
# Running DepCheck to find unused dependencies
npx depcheck
How These Tools Help with Code Restructuring
Using AI tools like GitHub Copilot, QodoGen, and ChatGPT speeds up the process of code restructuring. They provide suggestions that save time and catch issues early, making the code easier to maintain.
Combining these tools with automated analysis tools like SonarQube and ReSharper ensures all aspects of the codebase are covered, from quality checks to refactoring.
These AI tools have other features that facilitate this process: for example, they all have a chat feature that lets you ask questions and get replies about your code and any best practices you should be following. Also, QodoGen allows you to add parts of or the whole codebase for context with the click of a button, along with other features for test generation and pull request reviews.
When restructuring your codebase, having a variety of AI tools can make the process smoother and more efficient. This is AI usage at its best.
Version Control Best Practices for Code Changes
Version control keeps track of code changes, making it easier to manage updates, collaborate with others, and fix issues. Following some best practices can help maintain a clean and organized codebase.
Let’s look at how to manage code changes, track updates, and ensure quality through code reviews.
Using Git Branching Strategies to Manage Code Changes
Git branching helps keep different versions of the code separate, allowing multiple developers to work without affecting the main codebase. Here are some common strategies:
1. Feature Branching
Feature branches allow developers to work on a new feature without changing the main codebase. Each feature gets its own branch, and once complete, it can be merged into the main branch.
# Creating a new feature branch
git checkout -b feature/new-login-page
# Working on the new feature and then committing changes
git add .
git commit -m "Added login page UI"
# Merging the feature branch into the main branch
git checkout main
git merge feature/new-login-page
2. GitFlow Strategy
This strategy involves using multiple branches for different stages of development, such as feature, develop, and release. It separates development work and allows smoother integration and deployment.
- Main Branch: Contains production-ready code.
- Develop Branch: Holds the latest completed work, ready for the next release.
- Feature Branches: Created from the develop branch for new features.
Example:
# Switch to the develop branch
git checkout develop
# Create a new branch for a feature
git checkout -b feature/upgrade-search
# Commit changes and push the feature branch
git add .
git commit -m "Improved search feature"
git push origin feature/upgrade-search
How to Track and Document Code Updates
Documenting code changes helps keep the team informed and makes it easier to understand what was done later. Here are some tips for tracking updates:
1. Writing Clear Commit Messages
Commit messages should explain what was changed and why. A clear message helps others know the purpose of each update.
Example:
# Good commit message
git commit -m "Fixed bug that caused login failure on mobile devices"
# Bad commit message
git commit -m "Fixed bug"
2. Using Tags to Mark Releases
Tags can be used to label important points in the project’s history, such as release versions. This makes it easier to find stable versions of the code.
# Create a tag for version 1.0
git tag v1.0
# Push the tag to the remote repository
git push origin v1.0
3. Creating and Using Changelogs
A changelog lists the changes made in each version, helping developers and users see what was updated or fixed.
Example format for a changelog:
---
## [1.0.1] - 2024-10-01
### Added
- New login feature
### Fixed
- Resolved search issue on homepage
### Changed
- Updated user dashboard layout
Importance of Code Reviews in Maintaining Code Quality
Code reviews help catch errors, share knowledge, and ensure code stays clean and maintainable. Here are some practices to follow for effective code reviews:
1. Keep Code Changes Small
Smaller changes are easier to review, making it more likely to spot mistakes. Large changes can be broken down into smaller parts.
2. Use Pull Requests for Reviews
Pull requests create a space for discussion around changes. Team members can review the changes, suggest improvements, and approve the updates.
# Push the feature branch to the remote repository
git push origin feature/new-feature
# Create a pull request on GitHub, GitLab, or Bitbucket
3. Provide Constructive Feedback
Code reviews should aim to improve the code without discouraging the developer. Suggest better ways to solve problems and explain the reasoning.
Example comments during a code review:
- "Consider using a list instead of a dictionary for this data structure, as it simplifies the code."
- "This function is doing multiple tasks. It might be clearer if we split it into two separate functions."
Using these practices helps ensure code changes are managed effectively, updates are well-documented, and the quality of the codebase remains high. Regular code reviews and proper branching strategies make it easier for teams to collaborate and keep the project on track.
Conclusion
Reviving and restructuring a codebase can seem like a big task, but taking small, planned steps makes it manageable. Start by checking the current state of the code and making a list of areas that need work. Set clear goals and create a plan to improve the code, step by step.
Using the tools we discussed here can help find issues, suggest changes, and even automate some tasks. Version control practices, such as branching strategies and code reviews, keep changes organized and ensure the quality stays high.
With a solid approach, even the messiest codebase can become clean, efficient, and easier to work with.
Resources
- AI tools have been developed to assist with the Git branching, Pull Request reviews and approval. Check out this article (
oluwadamisisamuel1
) to read more on one of my favorites. - If you want a step by step tutorial on how to revive and refactor your code, check out this youtube video.
- Check out this freecodecamp article on code restructuring to dive deeper.
Connect with me on LinkedIn (samuel-oluwadamisi-01b3a4236
), X (Data_Steve_
), and my peronal blog (dashboard
) if you found this helpful.